
This code holds the complete demo set for the sx1280: PingPong, PER and Ranging Outdoor demo application. >>>>> This code MUST run on the mbed library release 127 or everything will be painfully slow.
Dependencies: mbed SX1280Lib DmTftLibrary
main.cpp
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: Main program 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #include "mbed.h" 00016 #include "Timers.h" 00017 #include "Menu.h" 00018 #include "Eeprom.h" 00019 #include "GpsMax7.h" 00020 #include "SX9306.h" 00021 #include "sx1280-hal.h" 00022 #include "main.h" 00023 #include "app_config.h" 00024 00025 00026 /*! 00027 * \brief Define IO for Unused Pin 00028 */ 00029 DigitalOut F_CS( D6 ); // MBED description of pin 00030 DigitalOut SD_CS( D8 ); // MBED description of pin 00031 DigitalIn userButton( USER_BUTTON ); 00032 00033 void PrintCompileSupportMessage( void ); 00034 00035 /*! 00036 * \brief Specify serial datarate for UART debug output 00037 */ 00038 void baud( int baudrate ) 00039 { 00040 Serial s( USBTX, USBRX ); 00041 s.baud( baudrate ); 00042 } 00043 00044 extern SX1280Hal Radio; 00045 00046 int main( ) 00047 { 00048 uint8_t currentPage = START_PAGE; 00049 uint8_t demoStatusUpdate = 0; // used for screen display status 00050 00051 baud( 115200 ); 00052 00053 F_CS = 1; 00054 SD_CS = 1; 00055 00056 printf( "Starting SX1280DevKit %s (%s)\n\r", FIRMWARE_VERSION, FIRMWARE_DATE ); 00057 PrintCompileSupportMessage(); 00058 00059 EepromInit( ); 00060 00061 if( userButton == 0 ) 00062 { 00063 FactoryReset( ); 00064 } 00065 00066 InitDemoApplication( ); 00067 MenuInit( ); 00068 00069 #if defined(HAS_GPS_SENSOR) || defined (HAS_PROXIMITY_SENSOR) 00070 TimersInit( ); 00071 #endif 00072 #if defined(HAS_GPS_SENSOR) 00073 Max7GpsInit( ); 00074 #endif 00075 #if defined(HAS_PROXIMITY_SENSOR) 00076 SX9306ProximityInit( ); 00077 #endif 00078 00079 printf( "Radio version: 0x%x\n\r", Radio.GetFirmwareVersion( ) ); 00080 00081 while( 1 ) 00082 { 00083 currentPage = MenuHandler( demoStatusUpdate ); 00084 00085 switch( currentPage ) 00086 { 00087 case START_PAGE: 00088 break; 00089 00090 case PAGE_PING_PONG: 00091 demoStatusUpdate = RunDemoApplicationPingPong( ); 00092 break; 00093 00094 case PAGE_PER: 00095 demoStatusUpdate = RunDemoApplicationPer( ); 00096 break; 00097 00098 case PAGE_RANGING_MASTER: 00099 case PAGE_RANGING_SLAVE: 00100 demoStatusUpdate = RunDemoApplicationRanging( ); 00101 break; 00102 00103 case PAGE_SLEEP_MODE: 00104 demoStatusUpdate = RunDemoSleepMode( ); 00105 break; 00106 00107 case PAGE_STBY_RC_MODE: 00108 demoStatusUpdate = RunDemoStandbyRcMode( ); 00109 break; 00110 00111 case PAGE_STBY_XOSC_MODE: 00112 demoStatusUpdate = RunDemoStandbyXoscMode( ); 00113 break; 00114 00115 case PAGE_TX_CW: 00116 demoStatusUpdate = RunDemoTxCw( ); 00117 break; 00118 00119 case PAGE_CONT_MODULATION: 00120 demoStatusUpdate = RunDemoTxContinuousModulation( ); 00121 break; 00122 00123 case PAGE_UTILITIES: 00124 // Extracts time and position information from the GPS module 00125 #if defined(HAS_GPS_SENSOR) 00126 Max7GpsHandle( ); 00127 #endif 00128 #if defined(HAS_PROXIMITY_SENSOR) 00129 SX9306ProximityHandle( ); //Deals with the proximity IC readings 00130 #endif 00131 break; 00132 00133 default: // Any page not running a demo 00134 break; 00135 } 00136 } 00137 } 00138 00139 void FactoryReset( void ) 00140 { 00141 EepromFactoryReset( ); 00142 HAL_NVIC_SystemReset( ); 00143 } 00144 00145 void PrintCompileSupportMessage( void ) 00146 { 00147 printf( "\nCompile time configuration:\n" ); 00148 #if defined(HAS_GPS_SENSOR) 00149 printf( " + gps\n" ); 00150 #else 00151 printf( " - gps\n" ); 00152 #endif 00153 #if defined(HAS_PROXIMITY_SENSOR) 00154 printf( " + proximity\n" ); 00155 #else 00156 printf( " - proximity\n" ); 00157 #endif 00158 printf("( '+ <FEAT>' means <FEAT> is enabled, '- <FEAT>' means <FEAT> is disabled)\n"); 00159 }
Generated on Wed Jul 13 2022 01:03:22 by
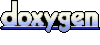