
This code holds the complete demo set for the sx1280: PingPong, PER and Ranging Outdoor demo application. >>>>> This code MUST run on the mbed library release 127 or everything will be painfully slow.
Dependencies: mbed SX1280Lib DmTftLibrary
Timers.h
00001 /* 00002 ______ _ 00003 / _____) _ | | 00004 ( (____ _____ ____ _| |_ _____ ____| |__ 00005 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00006 _____) ) ____| | | || |_| ____( (___| | | | 00007 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00008 (C)2016 Semtech 00009 00010 Description: Timers header 00011 00012 Maintainer: Gregory Cristian & Gilbert Menth 00013 */ 00014 00015 #ifndef TIMERS_H 00016 #define TIMERS_H 00017 00018 00019 #define TIM_MSEC ( uint32_t )1 00020 #define TIM_SEC ( uint32_t )1000 00021 #define TIM_MIN ( uint32_t )60000 00022 #define TIM_HOUR ( uint32_t )3600000 00023 #define MAX_TIMER_VALUE ( TIM_MIN * 150 ) // maximum time for timer 00024 00025 00026 /*! 00027 * \brief Initialses the hardware and variables associated with the timers. 00028 */ 00029 void TimersInit( void ); 00030 00031 /*! 00032 * \brief Sets a timer to a specific value 00033 * 00034 * \param [in] *STimer Pointer to the timer value to be set. 00035 * \param [in] TimeLength Value to set the timer to in milliseconds. 00036 */ 00037 void TimersSetTimer( uint32_t *sTimer, uint32_t timeLength ); 00038 00039 /*! 00040 * \brief Checks if a timer has expired. 00041 * 00042 * \param [in] *STimer Pointer to the timer value to be read. 00043 * 00044 * \retval Status Non zero if the timer has not expired and is still 00045 * running. 00046 */ 00047 uint32_t TimersTimerHasExpired ( const uint32_t * sTimer ); 00048 00049 /*! 00050 * \brief Returns the value of the current time in milliseconds 00051 * 00052 * \param [in] refresh Flag indicates refresh display required (touch) 00053 * 00054 * \retval Value value of current time in milliseconds 00055 */ 00056 uint32_t TimersTimerValue ( void ); 00057 00058 #endif //TIMERS_H 00059
Generated on Wed Jul 13 2022 01:03:22 by
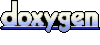