
SX1276 Tx Continuous Wave Demo Application
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 ( C )2014 Semtech 00008 00009 Description: Tx Continuous Wave implementation 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainers: Miguel Luis, Gregory Cristian and Nicolas Huguenin 00014 */ 00015 #include "mbed.h" 00016 #include "sx1276-hal.h" 00017 #include "debug.h" 00018 00019 /* Set this flag to '1' to test the HF max output power or '0' to the the LF max output power */ 00020 #define TEST_HF_OUTPUT 1 00021 #define TEST_LF_OUTPUT = !TEST_HF_OUTPUT 00022 00023 #define LORA_BANDWIDTH 0 // [0: 125 kHz, 00024 // 1: 250 kHz, 00025 // 2: 500 kHz, 00026 // 3: Reserved] 00027 #define LORA_SPREADING_FACTOR 9 // [SF7..SF12] 00028 #define LORA_CODINGRATE 1 // [1: 4/5, 00029 // 2: 4/6, 00030 // 3: 4/7, 00031 // 4: 4/8] 00032 #define LORA_PREAMBLE_LENGTH 8 // Same for Tx and Rx 00033 #define LORA_SYMBOL_TIMEOUT 5 // Symbols 00034 #define LORA_FIX_LENGTH_PAYLOAD_ON false 00035 #define LORA_FHSS_ENABLED false 00036 #define LORA_NB_SYMB_HOP 4 // Symbols 00037 #define LORA_IQ_INVERSION_ON false 00038 #define LORA_CRC_ENABLED true 00039 00040 /*! 00041 * Radio events function pointer 00042 */ 00043 static RadioEvents_t RadioEvents; 00044 00045 /*! 00046 * Tx continuous wave parameters 00047 */ 00048 struct 00049 { 00050 uint32_t RfFrequency; 00051 uint32_t TxOutputPower; 00052 }TxCwParams; 00053 00054 /*! 00055 * Radio object 00056 */ 00057 SX1276MB1xAS Radio( NULL ); 00058 00059 /*! 00060 * \brief Function executed on Radio Tx Timeout event 00061 */ 00062 void OnRadioTxTimeout( void ) 00063 { 00064 // Restarts continuous wave transmission when timeout expires after 65535 seconds 00065 Radio.SetTxContinuousWave( TxCwParams.RfFrequency, TxCwParams.TxOutputPower, 65535 ); 00066 } 00067 00068 /** 00069 * Main application entry point. 00070 */ 00071 int main( void ) 00072 { 00073 debug( "\n\r\n\r SX1276 Continuous Wave at full power Demo Application \n\r" ); 00074 00075 #if defined TARGET_NUCLEO_L152RE 00076 debug( " > Nucleo-L152RE Platform <\r\n" ); 00077 #elif defined TARGET_KL25Z 00078 debug( " > KL25Z Platform <\r\n" ); 00079 #elif defined TARGET_LPC11U6X 00080 debug( " > LPC11U6X Platform <\r\n" ); 00081 #else 00082 debug( " > Untested Platform <\r\n" ); 00083 #endif 00084 00085 #if( TEST_HF_OUTPUT == 1 ) 00086 00087 if( Radio.DetectBoardType( ) == SX1276MB1LAS ) // 00088 { 00089 debug("\r\n TEST_HF_OUTPUT on SX1276MB1LAS: 20 dBm at 915 MHz \r\n" ); 00090 00091 TxCwParams.RfFrequency = 915e6; 00092 TxCwParams.TxOutputPower = 20; 00093 } 00094 else 00095 { // SX1276MB1MAS 00096 debug("\r\n TEST_HF_OUTPUT on SX1276MB1MAS: 14 dBm at 868 MHz \r\n" ); 00097 00098 TxCwParams.RfFrequency = 868e6; 00099 TxCwParams.TxOutputPower = 14; 00100 } 00101 00102 #else //if( TEST_LF_OUTPUT == 1 ) 00103 00104 debug("\r\n TEST_LF_OUTPUT on SX1276MB1xAS: 14 dBm at 434 MHz \r\n" ); 00105 TxCwParams.RfFrequency = 433e6; 00106 TxCwParams.TxOutputPower = 14; 00107 00108 #endif 00109 00110 // Radio initialization 00111 RadioEvents.TxTimeout = OnRadioTxTimeout; 00112 Radio.Init( &RadioEvents ); 00113 00114 // Start continuous wave transmission fucntion expires after 65535 seconds 00115 Radio.SetTxContinuousWave( TxCwParams.RfFrequency, TxCwParams.TxOutputPower, 65535 ); 00116 00117 debug( "Start main loop: \r\n" ); 00118 // Blink LEDs just to show some activity 00119 while( 1 ) 00120 { 00121 debug( "Continuous Wave activated... \r\n" ); 00122 wait_ms( 200 ); 00123 } 00124 }
Generated on Fri Jul 15 2022 15:22:11 by
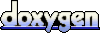