LoRaWAN MAC layer implementation
Dependents: LoRaWAN-demo-72_tjm LoRaWAN-demo-72_jlc LoRaWAN-demo-elmo frdm_LoRa_Connect_Woodstream_Demo_tjm ... more
LoRaMac.h
00001 /*! 00002 * \file LoRaMac.h 00003 * 00004 * \brief LoRa MAC layer implementation 00005 * 00006 * \copyright Revised BSD License, see section \ref LICENSE. 00007 * 00008 * \code 00009 * ______ _ 00010 * / _____) _ | | 00011 * ( (____ _____ ____ _| |_ _____ ____| |__ 00012 * \____ \| ___ | (_ _) ___ |/ ___) _ \ 00013 * _____) ) ____| | | || |_| ____( (___| | | | 00014 * (______/|_____)_|_|_| \__)_____)\____)_| |_| 00015 * (C)2013 Semtech 00016 * 00017 * ___ _____ _ ___ _ _____ ___ ___ ___ ___ 00018 * / __|_ _/_\ / __| |/ / __/ _ \| _ \/ __| __| 00019 * \__ \ | |/ _ \ (__| ' <| _| (_) | / (__| _| 00020 * |___/ |_/_/ \_\___|_|\_\_| \___/|_|_\\___|___| 00021 * embedded.connectivity.solutions=============== 00022 * 00023 * \endcode 00024 * 00025 * \author Miguel Luis ( Semtech ) 00026 * 00027 * \author Gregory Cristian ( Semtech ) 00028 * 00029 * \author Daniel Jäckle ( STACKFORCE ) 00030 * 00031 * \defgroup LORAMAC LoRa MAC layer implementation 00032 * This module specifies the API implementation of the LoRaMAC layer. 00033 * This is a placeholder for a detailed description of the LoRaMac 00034 * layer and the supported features. 00035 * \{ 00036 * 00037 * \example classA/LoRaMote/main.c 00038 * LoRaWAN class A application example for the LoRaMote. 00039 * 00040 * \example classB/LoRaMote/main.c 00041 * LoRaWAN class B application example for the LoRaMote. 00042 * 00043 * \example classC/LoRaMote/main.c 00044 * LoRaWAN class C application example for the LoRaMote. 00045 */ 00046 #ifndef __LORAMAC_H__ 00047 #define __LORAMAC_H__ 00048 00049 // Includes board dependent definitions such as channels frequencies 00050 #include "LoRaMac-board.h" 00051 00052 /*! 00053 * Beacon interval in us 00054 */ 00055 #define BEACON_INTERVAL 128000000 00056 00057 /*! 00058 * Class A&B receive delay 1 in us 00059 */ 00060 #define RECEIVE_DELAY1 1000000 00061 00062 /*! 00063 * Class A&B receive delay 2 in us 00064 */ 00065 #define RECEIVE_DELAY2 2000000 00066 00067 /*! 00068 * Join accept receive delay 1 in us 00069 */ 00070 #define JOIN_ACCEPT_DELAY1 5000000 00071 00072 /*! 00073 * Join accept receive delay 2 in us 00074 */ 00075 #define JOIN_ACCEPT_DELAY2 6000000 00076 00077 /*! 00078 * Class A&B maximum receive window delay in us 00079 */ 00080 #define MAX_RX_WINDOW 3000000 00081 00082 /*! 00083 * Maximum allowed gap for the FCNT field 00084 */ 00085 #define MAX_FCNT_GAP 16384 00086 00087 /*! 00088 * ADR acknowledgement counter limit 00089 */ 00090 #define ADR_ACK_LIMIT 64 00091 00092 /*! 00093 * Number of ADR acknowledgement requests before returning to default datarate 00094 */ 00095 #define ADR_ACK_DELAY 32 00096 00097 /*! 00098 * Number of seconds after the start of the second reception window without 00099 * receiving an acknowledge. 00100 * AckTimeout = \ref ACK_TIMEOUT + Random( -\ref ACK_TIMEOUT_RND, \ref ACK_TIMEOUT_RND ) 00101 */ 00102 #define ACK_TIMEOUT 2000000 00103 00104 /*! 00105 * Random number of seconds after the start of the second reception window without 00106 * receiving an acknowledge 00107 * AckTimeout = \ref ACK_TIMEOUT + Random( -\ref ACK_TIMEOUT_RND, \ref ACK_TIMEOUT_RND ) 00108 */ 00109 #define ACK_TIMEOUT_RND 1000000 00110 00111 /*! 00112 * Check the Mac layer state every MAC_STATE_CHECK_TIMEOUT in us 00113 */ 00114 #define MAC_STATE_CHECK_TIMEOUT 1000000 00115 00116 /*! 00117 * Maximum number of times the MAC layer tries to get an acknowledge. 00118 */ 00119 #define MAX_ACK_RETRIES 8 00120 00121 /*! 00122 * RSSI free threshold [dBm] 00123 */ 00124 #define RSSI_FREE_TH ( int8_t )( -90 ) 00125 00126 /*! 00127 * Frame direction definition for up-link communications 00128 */ 00129 #define UP_LINK 0 00130 00131 /*! 00132 * Frame direction definition for down-link communications 00133 */ 00134 #define DOWN_LINK 1 00135 00136 /*! 00137 * Sets the length of the LoRaMAC footer field. 00138 * Mainly indicates the MIC field length 00139 */ 00140 #define LORAMAC_MFR_LEN 4 00141 00142 /*! 00143 * Syncword for Private LoRa networks 00144 */ 00145 #define LORA_MAC_PRIVATE_SYNCWORD 0x12 00146 00147 /*! 00148 * Syncword for Public LoRa networks 00149 */ 00150 #define LORA_MAC_PUBLIC_SYNCWORD 0x34 00151 00152 /*! 00153 * LoRaMac internal state 00154 */ 00155 //uint32_t LoRaMacState; 00156 00157 /*! 00158 * LoRaWAN devices classes definition 00159 */ 00160 typedef enum eDeviceClass 00161 { 00162 /*! 00163 * LoRaWAN device class A 00164 * 00165 * LoRaWAN Specification V1.0, chapter 3ff 00166 */ 00167 CLASS_A , 00168 /*! 00169 * LoRaWAN device class B 00170 * 00171 * LoRaWAN Specification V1.0, chapter 8ff 00172 */ 00173 CLASS_B , 00174 /*! 00175 * LoRaWAN device class C 00176 * 00177 * LoRaWAN Specification V1.0, chapter 17ff 00178 */ 00179 CLASS_C , 00180 }DeviceClass_t ; 00181 00182 /*! 00183 * LoRaMAC channels parameters definition 00184 */ 00185 typedef union uDrRange 00186 { 00187 /*! 00188 * Byte-access to the bits 00189 */ 00190 int8_t Value ; 00191 /*! 00192 * Structure to store the minimum and the maximum datarate 00193 */ 00194 struct sFields 00195 { 00196 /*! 00197 * Minimum data rate 00198 * 00199 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00200 * 00201 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4] 00202 */ 00203 int8_t Min : 4; 00204 /*! 00205 * Maximum data rate 00206 * 00207 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00208 * 00209 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4] 00210 */ 00211 int8_t Max : 4; 00212 }Fields; 00213 }DrRange_t ; 00214 00215 /*! 00216 * LoRaMAC band parameters definition 00217 */ 00218 typedef struct sBand 00219 { 00220 /*! 00221 * Duty cycle 00222 */ 00223 uint16_t DCycle ; 00224 /*! 00225 * Maximum Tx power 00226 */ 00227 int8_t TxMaxPower ; 00228 /*! 00229 * Time stamp of the last Tx frame 00230 */ 00231 TimerTime_t LastTxDoneTime ; 00232 /*! 00233 * Holds the time where the device is off 00234 */ 00235 TimerTime_t TimeOff ; 00236 }Band_t ; 00237 00238 /*! 00239 * LoRaMAC channel definition 00240 */ 00241 typedef struct sChannelParams 00242 { 00243 /*! 00244 * Frequency in Hz 00245 */ 00246 uint32_t Frequency ; 00247 /*! 00248 * Data rate definition 00249 */ 00250 DrRange_t DrRange ; 00251 /*! 00252 * Band index 00253 */ 00254 uint8_t Band ; 00255 }ChannelParams_t ; 00256 00257 /*! 00258 * LoRaMAC receive window 2 channel parameters 00259 */ 00260 typedef struct sRx2ChannelParams 00261 { 00262 /*! 00263 * Frequency in Hz 00264 */ 00265 uint32_t Frequency ; 00266 /*! 00267 * Data rate 00268 * 00269 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00270 * 00271 * US915 - [DR_8, DR_9, DR_10, DR_11, DR_12, DR_13] 00272 */ 00273 uint8_t Datarate ; 00274 }Rx2ChannelParams_t ; 00275 00276 /*! 00277 * LoRaMAC multicast channel parameter 00278 */ 00279 typedef struct sMulticastParams 00280 { 00281 /*! 00282 * Address 00283 */ 00284 uint32_t Address ; 00285 /*! 00286 * Network session key 00287 */ 00288 uint8_t NwkSKey [16]; 00289 /*! 00290 * Application session key 00291 */ 00292 uint8_t AppSKey [16]; 00293 /*! 00294 * Downlink counter 00295 */ 00296 uint32_t DownLinkCounter ; 00297 /*! 00298 * Reference pointer to the next multicast channel parameters in the list 00299 */ 00300 struct sMulticastParams *Next ; 00301 }MulticastParams_t ; 00302 00303 /*! 00304 * LoRaMAC frame types 00305 * 00306 * LoRaWAN Specification V1.0, chapter 4.2.1, table 1 00307 */ 00308 typedef enum eLoRaMacFrameType 00309 { 00310 /*! 00311 * LoRaMAC join request frame 00312 */ 00313 FRAME_TYPE_JOIN_REQ = 0x00, 00314 /*! 00315 * LoRaMAC join accept frame 00316 */ 00317 FRAME_TYPE_JOIN_ACCEPT = 0x01, 00318 /*! 00319 * LoRaMAC unconfirmed up-link frame 00320 */ 00321 FRAME_TYPE_DATA_UNCONFIRMED_UP = 0x02, 00322 /*! 00323 * LoRaMAC unconfirmed down-link frame 00324 */ 00325 FRAME_TYPE_DATA_UNCONFIRMED_DOWN = 0x03, 00326 /*! 00327 * LoRaMAC confirmed up-link frame 00328 */ 00329 FRAME_TYPE_DATA_CONFIRMED_UP = 0x04, 00330 /*! 00331 * LoRaMAC confirmed down-link frame 00332 */ 00333 FRAME_TYPE_DATA_CONFIRMED_DOWN = 0x05, 00334 /*! 00335 * LoRaMAC RFU frame 00336 */ 00337 FRAME_TYPE_RFU = 0x06, 00338 /*! 00339 * LoRaMAC proprietary frame 00340 */ 00341 FRAME_TYPE_PROPRIETARY = 0x07, 00342 }LoRaMacFrameType_t ; 00343 00344 /*! 00345 * LoRaMAC mote MAC commands 00346 * 00347 * LoRaWAN Specification V1.0, chapter 5, table 4 00348 */ 00349 typedef enum eLoRaMacMoteCmd 00350 { 00351 /*! 00352 * LinkCheckReq 00353 */ 00354 MOTE_MAC_LINK_CHECK_REQ = 0x02, 00355 /*! 00356 * LinkADRAns 00357 */ 00358 MOTE_MAC_LINK_ADR_ANS = 0x03, 00359 /*! 00360 * DutyCycleAns 00361 */ 00362 MOTE_MAC_DUTY_CYCLE_ANS = 0x04, 00363 /*! 00364 * RXParamSetupAns 00365 */ 00366 MOTE_MAC_RX_PARAM_SETUP_ANS = 0x05, 00367 /*! 00368 * DevStatusAns 00369 */ 00370 MOTE_MAC_DEV_STATUS_ANS = 0x06, 00371 /*! 00372 * NewChannelAns 00373 */ 00374 MOTE_MAC_NEW_CHANNEL_ANS = 0x07, 00375 /*! 00376 * RXTimingSetupAns 00377 */ 00378 MOTE_MAC_RX_TIMING_SETUP_ANS = 0x08, 00379 }LoRaMacMoteCmd_t ; 00380 00381 /*! 00382 * LoRaMAC server MAC commands 00383 * 00384 * LoRaWAN Specification V1.0, chapter 5, table 4 00385 */ 00386 typedef enum eLoRaMacSrvCmd 00387 { 00388 /*! 00389 * LinkCheckAns 00390 */ 00391 SRV_MAC_LINK_CHECK_ANS = 0x02, 00392 /*! 00393 * LinkADRReq 00394 */ 00395 SRV_MAC_LINK_ADR_REQ = 0x03, 00396 /*! 00397 * DutyCycleReq 00398 */ 00399 SRV_MAC_DUTY_CYCLE_REQ = 0x04, 00400 /*! 00401 * RXParamSetupReq 00402 */ 00403 SRV_MAC_RX_PARAM_SETUP_REQ = 0x05, 00404 /*! 00405 * DevStatusReq 00406 */ 00407 SRV_MAC_DEV_STATUS_REQ = 0x06, 00408 /*! 00409 * NewChannelReq 00410 */ 00411 SRV_MAC_NEW_CHANNEL_REQ = 0x07, 00412 /*! 00413 * RXTimingSetupReq 00414 */ 00415 SRV_MAC_RX_TIMING_SETUP_REQ = 0x08, 00416 }LoRaMacSrvCmd_t ; 00417 00418 /*! 00419 * LoRaMAC Battery level indicator 00420 */ 00421 typedef enum eLoRaMacBatteryLevel 00422 { 00423 /*! 00424 * External power source 00425 */ 00426 BAT_LEVEL_EXT_SRC = 0x00, 00427 /*! 00428 * Battery level empty 00429 */ 00430 BAT_LEVEL_EMPTY = 0x01, 00431 /*! 00432 * Battery level full 00433 */ 00434 BAT_LEVEL_FULL = 0xFE, 00435 /*! 00436 * Battery level - no measurement available 00437 */ 00438 BAT_LEVEL_NO_MEASURE = 0xFF, 00439 }LoRaMacBatteryLevel_t ; 00440 00441 /*! 00442 * LoRaMAC header field definition (MHDR field) 00443 * 00444 * LoRaWAN Specification V1.0, chapter 4.2 00445 */ 00446 typedef union uLoRaMacHeader 00447 { 00448 /*! 00449 * Byte-access to the bits 00450 */ 00451 uint8_t Value ; 00452 /*! 00453 * Structure containing single access to header bits 00454 */ 00455 struct sHdrBits 00456 { 00457 /*! 00458 * Major version 00459 */ 00460 uint8_t Major : 2; 00461 /*! 00462 * RFU 00463 */ 00464 uint8_t RFU : 3; 00465 /*! 00466 * Message type 00467 */ 00468 uint8_t MType : 3; 00469 }Bits; 00470 }LoRaMacHeader_t ; 00471 00472 /*! 00473 * LoRaMAC frame control field definition (FCtrl) 00474 * 00475 * LoRaWAN Specification V1.0, chapter 4.3.1 00476 */ 00477 typedef union uLoRaMacFrameCtrl 00478 { 00479 /*! 00480 * Byte-access to the bits 00481 */ 00482 uint8_t Value ; 00483 /*! 00484 * Structure containing single access to bits 00485 */ 00486 struct sCtrlBits 00487 { 00488 /*! 00489 * Frame options length 00490 */ 00491 uint8_t FOptsLen : 4; 00492 /*! 00493 * Frame pending bit 00494 */ 00495 uint8_t FPending : 1; 00496 /*! 00497 * Message acknowledge bit 00498 */ 00499 uint8_t Ack : 1; 00500 /*! 00501 * ADR acknowledgment request bit 00502 */ 00503 uint8_t AdrAckReq : 1; 00504 /*! 00505 * ADR control in frame header 00506 */ 00507 uint8_t Adr : 1; 00508 }Bits; 00509 }LoRaMacFrameCtrl_t ; 00510 00511 /*! 00512 * Enumeration containing the status of the operation of a MAC service 00513 */ 00514 typedef enum eLoRaMacEventInfoStatus 00515 { 00516 /*! 00517 * Service performed successfully 00518 */ 00519 LORAMAC_EVENT_INFO_STATUS_OK = 0, 00520 /*! 00521 * An error occured during the execution of the service 00522 */ 00523 LORAMAC_EVENT_INFO_STATUS_ERROR , 00524 /*! 00525 * A Tx timeouit occured 00526 */ 00527 LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT , 00528 /*! 00529 * An Rx timeout occured on receive window 2 00530 */ 00531 LORAMAC_EVENT_INFO_STATUS_RX2_TIMEOUT , 00532 /*! 00533 * An Rx error occured on receive window 2 00534 */ 00535 LORAMAC_EVENT_INFO_STATUS_RX2_ERROR , 00536 /*! 00537 * An error occured in the join procedure 00538 */ 00539 LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL , 00540 /*! 00541 * A frame with an invalid downlink counter was received. The 00542 * downlink counter of the frame was equal to the local copy 00543 * of the downlink counter of the node. 00544 */ 00545 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_REPEATED , 00546 /*! 00547 * The node has lost MAX_FCNT_GAP or more frames. 00548 */ 00549 LORAMAC_EVENT_INFO_STATUS_DOWNLINK_TOO_MANY_FRAMES_LOSS , 00550 /*! 00551 * An address error occured 00552 */ 00553 LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL , 00554 /*! 00555 * message integrity check failure 00556 */ 00557 LORAMAC_EVENT_INFO_STATUS_MIC_FAIL , 00558 }LoRaMacEventInfoStatus_t ; 00559 00560 /*! 00561 * LoRaMac tx/rx operation state 00562 */ 00563 typedef union eLoRaMacFlags_t 00564 { 00565 /*! 00566 * Byte-access to the bits 00567 */ 00568 uint8_t Value ; 00569 /*! 00570 * Structure containing single access to bits 00571 */ 00572 struct sMacFlagBits 00573 { 00574 /*! 00575 * MCPS-Req pending 00576 */ 00577 uint8_t McpsReq : 1; 00578 /*! 00579 * MCPS-Ind pending 00580 */ 00581 uint8_t McpsInd : 1; 00582 /*! 00583 * MLME-Req pending 00584 */ 00585 uint8_t MlmeReq : 1; 00586 /*! 00587 * MAC cycle done 00588 */ 00589 uint8_t MacDone : 1; 00590 }Bits; 00591 }LoRaMacFlags_t ; 00592 00593 /*! 00594 * 00595 * \brief LoRaMAC data services 00596 * 00597 * \details The following table list the primitives which are supported by the 00598 * specific MAC data service: 00599 * 00600 * Name | Request | Indication | Response | Confirm 00601 * --------------------- | :-----: | :--------: | :------: | :-----: 00602 * \ref MCPS_UNCONFIRMED | YES | YES | NO | YES 00603 * \ref MCPS_CONFIRMED | YES | YES | NO | YES 00604 * \ref MCPS_MULTICAST | NO | YES | NO | NO 00605 * \ref MCPS_PROPRIETARY | YES | YES | NO | YES 00606 * 00607 * The following table provides links to the function implementations of the 00608 * related MCPS primitives: 00609 * 00610 * Primitive | Function 00611 * ---------------- | :---------------------: 00612 * MCPS-Request | \ref LoRaMacMlmeRequest 00613 * MCPS-Confirm | MacMcpsConfirm in \ref LoRaMacPrimitives_t 00614 * MCPS-Indication | MacMcpsIndication in \ref LoRaMacPrimitives_t 00615 */ 00616 typedef enum eMcps 00617 { 00618 /*! 00619 * Unconfirmed LoRaMAC frame 00620 */ 00621 MCPS_UNCONFIRMED , 00622 /*! 00623 * Confirmed LoRaMAC frame 00624 */ 00625 MCPS_CONFIRMED , 00626 /*! 00627 * Multicast LoRaMAC frame 00628 */ 00629 MCPS_MULTICAST , 00630 /*! 00631 * Proprietary frame 00632 */ 00633 MCPS_PROPRIETARY , 00634 }Mcps_t; 00635 00636 /*! 00637 * LoRaMAC MCPS-Request for an unconfirmed frame 00638 */ 00639 typedef struct sMcpsReqUnconfirmed 00640 { 00641 /*! 00642 * Frame port field. Must be set if the payload is not empty. Use the 00643 * application specific frame port values: [1...223] 00644 * 00645 * LoRaWAN Specification V1.0, chapter 4.3.2 00646 */ 00647 uint8_t fPort ; 00648 /*! 00649 * Pointer to the buffer of the frame payload 00650 */ 00651 void *fBuffer ; 00652 /*! 00653 * Size of the frame payload 00654 */ 00655 uint16_t fBufferSize ; 00656 /*! 00657 * Uplink datarate, if ADR is off 00658 */ 00659 int8_t Datarate ; 00660 }McpsReqUnconfirmed_t ; 00661 00662 /*! 00663 * LoRaMAC MCPS-Request for a confirmed frame 00664 */ 00665 typedef struct sMcpsReqConfirmed 00666 { 00667 /*! 00668 * Frame port field. Must be set if the payload is not empty. Use the 00669 * application specific frame port values: [1...223] 00670 * 00671 * LoRaWAN Specification V1.0, chapter 4.3.2 00672 */ 00673 uint8_t fPort ; 00674 /*! 00675 * Pointer to the buffer of the frame payload 00676 */ 00677 void *fBuffer ; 00678 /*! 00679 * Size of the frame payload 00680 */ 00681 uint16_t fBufferSize ; 00682 /*! 00683 * Uplink datarate, if ADR is off 00684 */ 00685 int8_t Datarate ; 00686 /*! 00687 * Number of trials to transmit the frame, if the LoRaMAC layer did not 00688 * receive an acknowledgment. The MAC performs a datarate adaptation, 00689 * according to the LoRaWAN Specification V1.0, chapter 18.4, according 00690 * to the following table: 00691 * 00692 * Transmission nb | Data Rate 00693 * ----------------|----------- 00694 * 1 (first) | DR 00695 * 2 | DR 00696 * 3 | max(DR-1,0) 00697 * 4 | max(DR-1,0) 00698 * 5 | max(DR-2,0) 00699 * 6 | max(DR-2,0) 00700 * 7 | max(DR-3,0) 00701 * 8 | max(DR-3,0) 00702 * 00703 * Note, that if NbTrials is set to 1 or 2, the MAC will not decrease 00704 * the datarate, in case the LoRaMAC layer did not receive an acknowledgment 00705 */ 00706 uint8_t NbTrials ; 00707 }McpsReqConfirmed_t ; 00708 00709 /*! 00710 * LoRaMAC MCPS-Request for a proprietary frame 00711 */ 00712 typedef struct sMcpsReqProprietary 00713 { 00714 /*! 00715 * Pointer to the buffer of the frame payload 00716 */ 00717 void *fBuffer ; 00718 /*! 00719 * Size of the frame payload 00720 */ 00721 uint16_t fBufferSize ; 00722 /*! 00723 * Uplink datarate, if ADR is off 00724 */ 00725 int8_t Datarate ; 00726 }McpsReqProprietary_t ; 00727 00728 /*! 00729 * LoRaMAC MCPS-Request structure 00730 */ 00731 typedef struct sMcpsReq 00732 { 00733 /*! 00734 * MCPS-Request type 00735 */ 00736 Mcps_t Type ; 00737 00738 /*! 00739 * MCPS-Request parameters 00740 */ 00741 union uMcpsParam 00742 { 00743 /*! 00744 * MCPS-Request parameters for an unconfirmed frame 00745 */ 00746 McpsReqUnconfirmed_t Unconfirmed ; 00747 /*! 00748 * MCPS-Request parameters for a confirmed frame 00749 */ 00750 McpsReqConfirmed_t Confirmed ; 00751 /*! 00752 * MCPS-Request parameters for a proprietary frame 00753 */ 00754 McpsReqProprietary_t Proprietary ; 00755 }Req; 00756 }McpsReq_t ; 00757 00758 /*! 00759 * LoRaMAC MCPS-Confirm 00760 */ 00761 typedef struct sMcpsConfirm 00762 { 00763 /*! 00764 * Holds the previously performed MCPS-Request 00765 */ 00766 Mcps_t McpsRequest ; 00767 /*! 00768 * Status of the operation 00769 */ 00770 LoRaMacEventInfoStatus_t Status ; 00771 /*! 00772 * Uplink datarate 00773 */ 00774 uint8_t Datarate ; 00775 /*! 00776 * Transmission power 00777 */ 00778 int8_t TxPower ; 00779 /*! 00780 * Set if an acknowledgement was received 00781 */ 00782 bool AckReceived ; 00783 /*! 00784 * Provides the number of retransmissions 00785 */ 00786 uint8_t NbRetries ; 00787 /*! 00788 * The transmission time on air of the frame 00789 */ 00790 TimerTime_t TxTimeOnAir ; 00791 /*! 00792 * The uplink counter value related to the frame 00793 */ 00794 uint32_t UpLinkCounter ; 00795 }McpsConfirm_t ; 00796 00797 /*! 00798 * LoRaMAC MCPS-Indication primitive 00799 */ 00800 typedef struct sMcpsIndication 00801 { 00802 /*! 00803 * MCPS-Indication type 00804 */ 00805 Mcps_t McpsIndication ; 00806 /*! 00807 * Status of the operation 00808 */ 00809 LoRaMacEventInfoStatus_t Status ; 00810 /*! 00811 * Multicast 00812 */ 00813 uint8_t Multicast ; 00814 /*! 00815 * Application port 00816 */ 00817 uint8_t Port ; 00818 /*! 00819 * Downlink datarate 00820 */ 00821 uint8_t RxDatarate ; 00822 /*! 00823 * Frame pending status 00824 */ 00825 uint8_t FramePending ; 00826 /*! 00827 * Pointer to the received data stream 00828 */ 00829 uint8_t *Buffer ; 00830 /*! 00831 * Size of the received data stream 00832 */ 00833 uint8_t BufferSize ; 00834 /*! 00835 * Indicates, if data is available 00836 */ 00837 bool RxData ; 00838 /*! 00839 * Rssi of the received packet 00840 */ 00841 int16_t Rssi ; 00842 /*! 00843 * Snr of the received packet 00844 */ 00845 uint8_t Snr ; 00846 /*! 00847 * Receive window 00848 * 00849 * [0: Rx window 1, 1: Rx window 2] 00850 */ 00851 uint8_t RxSlot ; 00852 /*! 00853 * Set if an acknowledgement was received 00854 */ 00855 bool AckReceived ; 00856 /*! 00857 * The downlink counter value for the received frame 00858 */ 00859 uint32_t DownLinkCounter ; 00860 }McpsIndication_t ; 00861 00862 /*! 00863 * \brief LoRaMAC management services 00864 * 00865 * \details The following table list the primitives which are supported by the 00866 * specific MAC management service: 00867 * 00868 * Name | Request | Indication | Response | Confirm 00869 * --------------------- | :-----: | :--------: | :------: | :-----: 00870 * \ref MLME_JOIN | YES | NO | NO | YES 00871 * \ref MLME_LINK_CHECK | YES | NO | NO | YES 00872 * 00873 * The following table provides links to the function implementations of the 00874 * related MLME primitives. 00875 * 00876 * Primitive | Function 00877 * ---------------- | :---------------------: 00878 * MLME-Request | \ref LoRaMacMlmeRequest 00879 * MLME-Confirm | MacMlmeConfirm in \ref LoRaMacPrimitives_t 00880 */ 00881 typedef enum eMlme 00882 { 00883 /*! 00884 * Initiates the Over-the-Air activation 00885 * 00886 * LoRaWAN Specification V1.0, chapter 6.2 00887 */ 00888 MLME_JOIN , 00889 /*! 00890 * LinkCheckReq - Connectivity validation 00891 * 00892 * LoRaWAN Specification V1.0, chapter 5, table 4 00893 */ 00894 MLME_LINK_CHECK , 00895 }Mlme_t; 00896 00897 /*! 00898 * LoRaMAC MLME-Request for the join service 00899 */ 00900 typedef struct sMlmeReqJoin 00901 { 00902 /*! 00903 * Globally unique end-device identifier 00904 * 00905 * LoRaWAN Specification V1.0, chapter 6.2.1 00906 */ 00907 uint8_t *DevEui ; 00908 /*! 00909 * Application identifier 00910 * 00911 * LoRaWAN Specification V1.0, chapter 6.1.2 00912 */ 00913 uint8_t *AppEui ; 00914 /*! 00915 * AES-128 application key 00916 * 00917 * LoRaWAN Specification V1.0, chapter 6.2.2 00918 */ 00919 uint8_t *AppKey ; 00920 }MlmeReqJoin_t ; 00921 00922 /*! 00923 * LoRaMAC MLME-Request structure 00924 */ 00925 typedef struct sMlmeReq 00926 { 00927 /*! 00928 * MLME-Request type 00929 */ 00930 Mlme_t Type ; 00931 00932 /*! 00933 * MLME-Request parameters 00934 */ 00935 union uMlmeParam 00936 { 00937 /*! 00938 * MLME-Request parameters for a join request 00939 */ 00940 MlmeReqJoin_t Join ; 00941 }Req; 00942 }MlmeReq_t ; 00943 00944 /*! 00945 * LoRaMAC MLME-Confirm primitive 00946 */ 00947 typedef struct sMlmeConfirm 00948 { 00949 /*! 00950 * Holds the previously performed MLME-Request 00951 */ 00952 Mlme_t MlmeRequest ; 00953 /*! 00954 * Status of the operation 00955 */ 00956 LoRaMacEventInfoStatus_t Status ; 00957 /*! 00958 * The transmission time on air of the frame 00959 */ 00960 TimerTime_t TxTimeOnAir ; 00961 /*! 00962 * Demodulation margin. Contains the link margin [dB] of the last 00963 * successfully received LinkCheckReq 00964 */ 00965 uint8_t DemodMargin ; 00966 /*! 00967 * Number of gateways which received the last LinkCheckReq 00968 */ 00969 uint8_t NbGateways ; 00970 }MlmeConfirm_t ; 00971 00972 /*! 00973 * LoRa Mac Information Base (MIB) 00974 * 00975 * The following table lists the MIB parameters and the related attributes: 00976 * 00977 * Attribute | Get | Set 00978 * --------------------------------- | :-: | :-: 00979 * \ref MIB_DEVICE_CLASS | YES | YES 00980 * \ref MIB_NETWORK_JOINED | YES | YES 00981 * \ref MIB_ADR | YES | YES 00982 * \ref MIB_NET_ID | YES | YES 00983 * \ref MIB_DEV_ADDR | YES | YES 00984 * \ref MIB_NWK_SKEY | YES | YES 00985 * \ref MIB_APP_SKEY | YES | YES 00986 * \ref MIB_PUBLIC_NETWORK | YES | YES 00987 * \ref MIB_REPEATER_SUPPORT | YES | YES 00988 * \ref MIB_CHANNELS | YES | NO 00989 * \ref MIB_RX2_CHANNEL | YES | YES 00990 * \ref MIB_CHANNELS_MASK | YES | YES 00991 * \ref MIB_CHANNELS_NB_REP | YES | YES 00992 * \ref MIB_MAX_RX_WINDOW_DURATION | YES | YES 00993 * \ref MIB_RECEIVE_DELAY_1 | YES | YES 00994 * \ref MIB_RECEIVE_DELAY_2 | YES | YES 00995 * \ref MIB_JOIN_ACCEPT_DELAY_1 | YES | YES 00996 * \ref MIB_JOIN_ACCEPT_DELAY_2 | YES | YES 00997 * \ref MIB_CHANNELS_DATARATE | YES | YES 00998 * \ref MIB_CHANNELS_DEFAULT_DATARATE| YES | YES 00999 * \ref MIB_CHANNELS_TX_POWER | YES | YES 01000 * \ref MIB_UPLINK_COUNTER | YES | YES 01001 * \ref MIB_DOWNLINK_COUNTER | YES | YES 01002 * \ref MIB_MULTICAST_CHANNEL | YES | NO 01003 * 01004 * The following table provides links to the function implementations of the 01005 * related MIB primitives: 01006 * 01007 * Primitive | Function 01008 * ---------------- | :---------------------: 01009 * MIB-Set | \ref LoRaMacMibSetRequestConfirm 01010 * MIB-Get | \ref LoRaMacMibGetRequestConfirm 01011 */ 01012 typedef enum eMib 01013 { 01014 /*! 01015 * LoRaWAN device class 01016 * 01017 * LoRaWAN Specification V1.0 01018 */ 01019 MIB_DEVICE_CLASS , 01020 /*! 01021 * LoRaWAN Network joined attribute 01022 * 01023 * LoRaWAN Specification V1.0 01024 */ 01025 MIB_NETWORK_JOINED , 01026 /*! 01027 * Adaptive data rate 01028 * 01029 * LoRaWAN Specification V1.0, chapter 4.3.1.1 01030 * 01031 * [true: ADR enabled, false: ADR disabled] 01032 */ 01033 MIB_ADR , 01034 /*! 01035 * Network identifier 01036 * 01037 * LoRaWAN Specification V1.0, chapter 6.2.5 01038 */ 01039 MIB_NET_ID , 01040 /*! 01041 * End-device address 01042 * 01043 * LoRaWAN Specification V1.0, chapter 6.1.2 01044 */ 01045 MIB_DEV_ADDR , 01046 /*! 01047 * Network session key 01048 * 01049 * LoRaWAN Specification V1.0, chapter 6.1.3 01050 */ 01051 MIB_NWK_SKEY , 01052 /*! 01053 * Application session key 01054 * 01055 * LoRaWAN Specification V1.0, chapter 6.1.4 01056 */ 01057 MIB_APP_SKEY , 01058 /*! 01059 * Set the network type to public or private 01060 * 01061 * LoRaWAN Specification V1.0, chapter 7 01062 * 01063 * [true: public network, false: private network] 01064 */ 01065 MIB_PUBLIC_NETWORK , 01066 /*! 01067 * Support the operation with repeaters 01068 * 01069 * LoRaWAN Specification V1.0, chapter 7 01070 * 01071 * [true: repeater support enabled, false: repeater support disabled] 01072 */ 01073 MIB_REPEATER_SUPPORT , 01074 /*! 01075 * Communication channels. A get request will return a 01076 * pointer which references the first entry of the channel list. The 01077 * list is of size LORA_MAX_NB_CHANNELS 01078 * 01079 * LoRaWAN Specification V1.0, chapter 7 01080 */ 01081 MIB_CHANNELS , 01082 /*! 01083 * Set receive window 2 channel 01084 * 01085 * LoRaWAN Specification V1.0, chapter 3.3.2 01086 */ 01087 MIB_RX2_CHANNEL , 01088 /*! 01089 * LoRaWAN channels mask 01090 * 01091 * LoRaWAN Specification V1.0, chapter 5.2 01092 */ 01093 MIB_CHANNELS_MASK , 01094 /*! 01095 * Set the number of repetitions on a channel 01096 * 01097 * LoRaWAN Specification V1.0, chapter 5.2 01098 */ 01099 MIB_CHANNELS_NB_REP , 01100 /*! 01101 * Maximum receive window duration in [us] 01102 * 01103 * LoRaWAN Specification V1.0, chapter 3.3.3 01104 */ 01105 MIB_MAX_RX_WINDOW_DURATION , 01106 /*! 01107 * Receive delay 1 in [us] 01108 * 01109 * LoRaWAN Specification V1.0, chapter 6 01110 */ 01111 MIB_RECEIVE_DELAY_1 , 01112 /*! 01113 * Receive delay 2 in [us] 01114 * 01115 * LoRaWAN Specification V1.0, chapter 6 01116 */ 01117 MIB_RECEIVE_DELAY_2 , 01118 /*! 01119 * Join accept delay 1 in [us] 01120 * 01121 * LoRaWAN Specification V1.0, chapter 6 01122 */ 01123 MIB_JOIN_ACCEPT_DELAY_1 , 01124 /*! 01125 * Join accept delay 2 in [us] 01126 * 01127 * LoRaWAN Specification V1.0, chapter 6 01128 */ 01129 MIB_JOIN_ACCEPT_DELAY_2 , 01130 /*! 01131 * Default Data rate of a channel 01132 * 01133 * LoRaWAN Specification V1.0, chapter 7 01134 * 01135 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 01136 * 01137 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_8, DR_9, DR_10, DR_11, DR_12, DR_13] 01138 */ 01139 MIB_CHANNELS_DEFAULT_DATARATE , 01140 /*! 01141 * Data rate of a channel 01142 * 01143 * LoRaWAN Specification V1.0, chapter 7 01144 * 01145 * EU868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 01146 * 01147 * US915 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_8, DR_9, DR_10, DR_11, DR_12, DR_13] 01148 */ 01149 MIB_CHANNELS_DATARATE , 01150 /*! 01151 * Transmission power of a channel 01152 * 01153 * LoRaWAN Specification V1.0, chapter 7 01154 * 01155 * EU868 - [TX_POWER_20_DBM, TX_POWER_14_DBM, TX_POWER_11_DBM, 01156 * TX_POWER_08_DBM, TX_POWER_05_DBM, TX_POWER_02_DBM] 01157 * 01158 * US915 - [TX_POWER_30_DBM, TX_POWER_28_DBM, TX_POWER_26_DBM, 01159 * TX_POWER_24_DBM, TX_POWER_22_DBM, TX_POWER_20_DBM, 01160 * TX_POWER_18_DBM, TX_POWER_14_DBM, TX_POWER_12_DBM, 01161 * TX_POWER_10_DBM] 01162 */ 01163 MIB_CHANNELS_TX_POWER , 01164 /*! 01165 * LoRaWAN Up-link counter 01166 * 01167 * LoRaWAN Specification V1.0, chapter 4.3.1.5 01168 */ 01169 MIB_UPLINK_COUNTER , 01170 /*! 01171 * LoRaWAN Down-link counter 01172 * 01173 * LoRaWAN Specification V1.0, chapter 4.3.1.5 01174 */ 01175 MIB_DOWNLINK_COUNTER , 01176 /*! 01177 * Multicast channels. A get request will return a pointer to the first 01178 * entry of the multicast channel linked list. If the pointer is equal to 01179 * NULL, the list is empty. 01180 */ 01181 MIB_MULTICAST_CHANNEL , 01182 }Mib_t ; 01183 01184 /*! 01185 * LoRaMAC MIB parameters 01186 */ 01187 typedef union uMibParam 01188 { 01189 /*! 01190 * LoRaWAN device class 01191 * 01192 * Related MIB type: \ref MIB_DEVICE_CLASS 01193 */ 01194 DeviceClass_t Class ; 01195 /*! 01196 * LoRaWAN network joined attribute 01197 * 01198 * Related MIB type: \ref MIB_NETWORK_JOINED 01199 */ 01200 bool IsNetworkJoined ; 01201 /*! 01202 * Activation state of ADR 01203 * 01204 * Related MIB type: \ref MIB_ADR 01205 */ 01206 bool AdrEnable ; 01207 /*! 01208 * Network identifier 01209 * 01210 * Related MIB type: \ref MIB_NET_ID 01211 */ 01212 uint32_t NetID ; 01213 /*! 01214 * End-device address 01215 * 01216 * Related MIB type: \ref MIB_DEV_ADDR 01217 */ 01218 uint32_t DevAddr ; 01219 /*! 01220 * Network session key 01221 * 01222 * Related MIB type: \ref MIB_NWK_SKEY 01223 */ 01224 uint8_t *NwkSKey ; 01225 /*! 01226 * Application session key 01227 * 01228 * Related MIB type: \ref MIB_APP_SKEY 01229 */ 01230 uint8_t *AppSKey ; 01231 /*! 01232 * Enable or disable a public network 01233 * 01234 * Related MIB type: \ref MIB_PUBLIC_NETWORK 01235 */ 01236 bool EnablePublicNetwork ; 01237 /*! 01238 * Enable or disable repeater support 01239 * 01240 * Related MIB type: \ref MIB_REPEATER_SUPPORT 01241 */ 01242 bool EnableRepeaterSupport ; 01243 /*! 01244 * LoRaWAN Channel 01245 * 01246 * Related MIB type: \ref MIB_CHANNELS 01247 */ 01248 ChannelParams_t * ChannelList ; 01249 /*! 01250 * Channel for the receive window 2 01251 * 01252 * Related MIB type: \ref MIB_RX2_CHANNEL 01253 */ 01254 Rx2ChannelParams_t Rx2Channel ; 01255 /*! 01256 * Channel mask 01257 * 01258 * Related MIB type: \ref MIB_CHANNELS_MASK 01259 */ 01260 uint16_t* ChannelsMask ; 01261 /*! 01262 * Number of frame repetitions 01263 * 01264 * Related MIB type: \ref MIB_CHANNELS_NB_REP 01265 */ 01266 uint8_t ChannelNbRep ; 01267 /*! 01268 * Maximum receive window duration 01269 * 01270 * Related MIB type: \ref MIB_MAX_RX_WINDOW_DURATION 01271 */ 01272 uint32_t MaxRxWindow ; 01273 /*! 01274 * Receive delay 1 01275 * 01276 * Related MIB type: \ref MIB_RECEIVE_DELAY_1 01277 */ 01278 uint32_t ReceiveDelay1 ; 01279 /*! 01280 * Receive delay 2 01281 * 01282 * Related MIB type: \ref MIB_RECEIVE_DELAY_2 01283 */ 01284 uint32_t ReceiveDelay2 ; 01285 /*! 01286 * Join accept delay 1 01287 * 01288 * Related MIB type: \ref MIB_JOIN_ACCEPT_DELAY_1 01289 */ 01290 uint32_t JoinAcceptDelay1 ; 01291 /*! 01292 * Join accept delay 2 01293 * 01294 * Related MIB type: \ref MIB_JOIN_ACCEPT_DELAY_2 01295 */ 01296 uint32_t JoinAcceptDelay2 ; 01297 /*! 01298 * Channels data rate 01299 * 01300 * Related MIB type: \ref MIB_CHANNELS_DEFAULT_DATARATE 01301 */ 01302 int8_t ChannelsDefaultDatarate ; 01303 /*! 01304 * Channels data rate 01305 * 01306 * Related MIB type: \ref MIB_CHANNELS_DATARATE 01307 */ 01308 int8_t ChannelsDatarate ; 01309 /*! 01310 * Channels TX power 01311 * 01312 * Related MIB type: \ref MIB_CHANNELS_TX_POWER 01313 */ 01314 int8_t ChannelsTxPower ; 01315 /*! 01316 * LoRaWAN Up-link counter 01317 * 01318 * Related MIB type: \ref MIB_UPLINK_COUNTER 01319 */ 01320 uint32_t UpLinkCounter ; 01321 /*! 01322 * LoRaWAN Down-link counter 01323 * 01324 * Related MIB type: \ref MIB_DOWNLINK_COUNTER 01325 */ 01326 uint32_t DownLinkCounter ; 01327 /*! 01328 * Multicast channel 01329 * 01330 * Related MIB type: \ref MIB_MULTICAST_CHANNEL 01331 */ 01332 MulticastParams_t * MulticastList ; 01333 }MibParam_t ; 01334 01335 /*! 01336 * LoRaMAC MIB-RequestConfirm structure 01337 */ 01338 typedef struct eMibRequestConfirm 01339 { 01340 /*! 01341 * MIB-Request type 01342 */ 01343 Mib_t Type ; 01344 01345 /*! 01346 * MLME-RequestConfirm parameters 01347 */ 01348 MibParam_t Param ; 01349 }MibRequestConfirm_t ; 01350 01351 /*! 01352 * LoRaMAC tx information 01353 */ 01354 typedef struct sLoRaMacTxInfo 01355 { 01356 /*! 01357 * Defines the size of the applicative payload which can be processed 01358 */ 01359 uint8_t MaxPossiblePayload ; 01360 /*! 01361 * The current payload size, dependent on the current datarate 01362 */ 01363 uint8_t CurrentPayloadSize ; 01364 }LoRaMacTxInfo_t ; 01365 01366 /*! 01367 * LoRaMAC Status 01368 */ 01369 typedef enum eLoRaMacStatus 01370 { 01371 /*! 01372 * Service started successfully 01373 */ 01374 LORAMAC_STATUS_OK , 01375 /*! 01376 * Service not started - LoRaMAC is busy 01377 */ 01378 LORAMAC_STATUS_BUSY , 01379 /*! 01380 * Service unknown 01381 */ 01382 LORAMAC_STATUS_SERVICE_UNKNOWN , 01383 /*! 01384 * Service not started - invalid parameter 01385 */ 01386 LORAMAC_STATUS_PARAMETER_INVALID , 01387 /*! 01388 * Service not started - invalid frequency 01389 */ 01390 LORAMAC_STATUS_FREQUENCY_INVALID , 01391 /*! 01392 * Service not started - invalid datarate 01393 */ 01394 LORAMAC_STATUS_DATARATE_INVALID , 01395 /*! 01396 * Service not started - invalid frequency and datarate 01397 */ 01398 LORAMAC_STATUS_FREQ_AND_DR_INVALID , 01399 /*! 01400 * Service not started - the device is not in a LoRaWAN 01401 */ 01402 LORAMAC_STATUS_NO_NETWORK_JOINED , 01403 /*! 01404 * Service not started - playload lenght error 01405 */ 01406 LORAMAC_STATUS_LENGTH_ERROR , 01407 /*! 01408 * Service not started - playload lenght error 01409 */ 01410 LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR , 01411 /*! 01412 * Service not started - the device is switched off 01413 */ 01414 LORAMAC_STATUS_DEVICE_OFF , 01415 }LoRaMacStatus_t ; 01416 01417 /*! 01418 * LoRaMAC events structure 01419 * Used to notify upper layers of MAC events 01420 */ 01421 typedef struct sLoRaMacPrimitives 01422 { 01423 /*! 01424 * \brief MCPS-Confirm primitive 01425 * 01426 * \param [OUT] MCPS-Confirm parameters 01427 */ 01428 void ( *MacMcpsConfirm )( McpsConfirm_t *McpsConfirm ); 01429 /*! 01430 * \brief MCPS-Indication primitive 01431 * 01432 * \param [OUT] MCPS-Indication parameters 01433 */ 01434 void ( *MacMcpsIndication )( McpsIndication_t *McpsIndication ); 01435 /*! 01436 * \brief MLME-Confirm primitive 01437 * 01438 * \param [OUT] MLME-Confirm parameters 01439 */ 01440 void ( *MacMlmeConfirm )( MlmeConfirm_t *MlmeConfirm ); 01441 }LoRaMacPrimitives_t ; 01442 01443 typedef struct sLoRaMacCallback 01444 { 01445 /*! 01446 * \brief Measures the battery level 01447 * 01448 * \retval Battery level [0: node is connected to an external 01449 * power source, 1..254: battery level, where 1 is the minimum 01450 * and 254 is the maximum value, 255: the node was not able 01451 * to measure the battery level] 01452 */ 01453 uint8_t ( *GetBatteryLevel )( void ); 01454 }LoRaMacCallback_t; 01455 01456 /*! 01457 * \brief LoRaMAC layer initialization 01458 * 01459 * \details In addition to the initialization of the LoRaMAC layer, this 01460 * function initializes the callback primitives of the MCPS and 01461 * MLME services. Every data field of \ref LoRaMacPrimitives_t must be 01462 * set to a valid callback function. 01463 * 01464 * \param [IN] events - Pointer to a structure defining the LoRaMAC 01465 * event functions. Refer to \ref LoRaMacPrimitives_t. 01466 * 01467 * \param [IN] events - Pointer to a structure defining the LoRaMAC 01468 * callback functions. Refer to \ref LoRaMacCallback_t. 01469 * 01470 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01471 * returns are: 01472 * \ref LORAMAC_STATUS_OK, 01473 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01474 */ 01475 LoRaMacStatus_t LoRaMacInitialization( LoRaMacPrimitives_t *primitives, LoRaMacCallback_t *callbacks ); 01476 01477 /*! 01478 * \brief Queries the LoRaMAC if it is possible to send the next frame with 01479 * a given payload size. The LoRaMAC takes scheduled MAC commands into 01480 * account and reports, when the frame can be send or not. 01481 * 01482 * \param [IN] size - Size of applicative payload to be send next 01483 * 01484 * \param [OUT] txInfo - The structure \ref LoRaMacTxInfo_t contains 01485 * information about the actual maximum payload possible 01486 * ( according to the configured datarate or the next 01487 * datarate according to ADR ), and the maximum frame 01488 * size, taking the scheduled MAC commands into account. 01489 * 01490 * \retval LoRaMacStatus_t Status of the operation. When the parameters are 01491 * not valid, the function returns \ref LORAMAC_STATUS_PARAMETER_INVALID. 01492 * In case of a length error caused by the applicative payload size, the 01493 * function returns LORAMAC_STATUS_LENGTH_ERROR. In case of a length error 01494 * due to additional MAC commands in the queue, the function returns 01495 * LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR. In case the query is valid, and 01496 * the LoRaMAC is able to send the frame, the function returns LORAMAC_STATUS_OK. * 01497 */ 01498 LoRaMacStatus_t LoRaMacQueryTxPossible( uint8_t size, LoRaMacTxInfo_t * txInfo ); 01499 01500 /*! 01501 * \brief LoRaMAC channel add service 01502 * 01503 * \details Adds a new channel to the channel list and activates the id in 01504 * the channel mask. For the US915 band, all channels are enabled 01505 * by default. It is not possible to activate less than 6 125 kHz 01506 * channels. 01507 * 01508 * \param [IN] id - Id of the channel. Possible values are: 01509 * 01510 * 0-15 for EU868 01511 * 0-72 for US915 01512 * 01513 * \param [IN] params - Channel parameters to set. 01514 * 01515 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01516 * \ref LORAMAC_STATUS_OK, 01517 * \ref LORAMAC_STATUS_BUSY, 01518 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01519 */ 01520 LoRaMacStatus_t LoRaMacChannelAdd( uint8_t id, ChannelParams_t params ); 01521 01522 /*! 01523 * \brief LoRaMAC channel remove service 01524 * 01525 * \details Deactivates the id in the channel mask. 01526 * 01527 * \param [IN] id - Id of the channel. 01528 * 01529 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01530 * \ref LORAMAC_STATUS_OK, 01531 * \ref LORAMAC_STATUS_BUSY, 01532 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01533 */ 01534 LoRaMacStatus_t LoRaMacChannelRemove( uint8_t id ); 01535 01536 /*! 01537 * \brief LoRaMAC multicast channel link service 01538 * 01539 * \details Links a multicast channel into the linked list. 01540 * 01541 * \param [IN] channelParam - Multicast channel parameters to link. 01542 * 01543 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01544 * \ref LORAMAC_STATUS_OK, 01545 * \ref LORAMAC_STATUS_BUSY, 01546 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01547 */ 01548 LoRaMacStatus_t LoRaMacMulticastChannelLink( MulticastParams_t *channelParam ); 01549 01550 /*! 01551 * \brief LoRaMAC multicast channel unlink service 01552 * 01553 * \details Unlinks a multicast channel from the linked list. 01554 * 01555 * \param [IN] channelParam - Multicast channel parameters to unlink. 01556 * 01557 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01558 * \ref LORAMAC_STATUS_OK, 01559 * \ref LORAMAC_STATUS_BUSY, 01560 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01561 */ 01562 LoRaMacStatus_t LoRaMacMulticastChannelUnlink( MulticastParams_t *channelParam ); 01563 01564 /*! 01565 * \brief LoRaMAC MIB-Get 01566 * 01567 * \details The mac information base service to get attributes of the LoRaMac 01568 * layer. 01569 * 01570 * The following code-snippet shows how to use the API to get the 01571 * parameter AdrEnable, defined by the enumeration type 01572 * \ref MIB_ADR. 01573 * \code 01574 * MibRequestConfirm_t mibReq; 01575 * mibReq.Type = MIB_ADR; 01576 * 01577 * if( LoRaMacMibGetRequestConfirm( &mibReq ) == LORAMAC_STATUS_OK ) 01578 * { 01579 * // LoRaMAC updated the parameter mibParam.AdrEnable 01580 * } 01581 * \endcode 01582 * 01583 * \param [IN] mibRequest - MIB-GET-Request to perform. Refer to \ref MibRequestConfirm_t. 01584 * 01585 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01586 * \ref LORAMAC_STATUS_OK, 01587 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01588 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01589 */ 01590 LoRaMacStatus_t LoRaMacMibGetRequestConfirm( MibRequestConfirm_t *mibGet ); 01591 01592 /*! 01593 * \brief LoRaMAC MIB-Set 01594 * 01595 * \details The mac information base service to set attributes of the LoRaMac 01596 * layer. 01597 * 01598 * The following code-snippet shows how to use the API to set the 01599 * parameter AdrEnable, defined by the enumeration type 01600 * \ref MIB_ADR. 01601 * 01602 * \code 01603 * MibRequestConfirm_t mibReq; 01604 * mibReq.Type = MIB_ADR; 01605 * mibReq.Param.AdrEnable = true; 01606 * 01607 * if( LoRaMacMibGetRequestConfirm( &mibReq ) == LORAMAC_STATUS_OK ) 01608 * { 01609 * // LoRaMAC updated the parameter 01610 * } 01611 * \endcode 01612 * 01613 * \param [IN] mibRequest - MIB-SET-Request to perform. Refer to \ref MibRequestConfirm_t. 01614 * 01615 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01616 * \ref LORAMAC_STATUS_OK, 01617 * \ref LORAMAC_STATUS_BUSY, 01618 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01619 * \ref LORAMAC_STATUS_PARAMETER_INVALID. 01620 */ 01621 LoRaMacStatus_t LoRaMacMibSetRequestConfirm( MibRequestConfirm_t *mibSet ); 01622 01623 /*! 01624 * \brief LoRaMAC MLME-Request 01625 * 01626 * \details The Mac layer management entity handles management services. The 01627 * following code-snippet shows how to use the API to perform a 01628 * network join request. 01629 * 01630 * \code 01631 * static uint8_t DevEui[] = 01632 * { 01633 * 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 01634 * }; 01635 * static uint8_t AppEui[] = 01636 * { 01637 * 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 01638 * }; 01639 * static uint8_t AppKey[] = 01640 * { 01641 * 0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6, 01642 * 0xAB, 0xF7, 0x15, 0x88, 0x09, 0xCF, 0x4F, 0x3C 01643 * }; 01644 * 01645 * MlmeReq_t mlmeReq; 01646 * mlmeReq.Type = MLME_JOIN; 01647 * mlmeReq.Req.Join.DevEui = DevEui; 01648 * mlmeReq.Req.Join.AppEui = AppEui; 01649 * mlmeReq.Req.Join.AppKey = AppKey; 01650 * 01651 * if( LoRaMacMlmeRequest( &mlmeReq ) == LORAMAC_STATUS_OK ) 01652 * { 01653 * // Service started successfully. Waiting for the Mlme-Confirm event 01654 * } 01655 * \endcode 01656 * 01657 * \param [IN] mlmeRequest - MLME-Request to perform. Refer to \ref MlmeReq_t. 01658 * 01659 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01660 * \ref LORAMAC_STATUS_OK, 01661 * \ref LORAMAC_STATUS_BUSY, 01662 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01663 * \ref LORAMAC_STATUS_PARAMETER_INVALID, 01664 * \ref LORAMAC_STATUS_NO_NETWORK_JOINED, 01665 * \ref LORAMAC_STATUS_LENGTH_ERROR, 01666 * \ref LORAMAC_STATUS_DEVICE_OFF. 01667 */ 01668 LoRaMacStatus_t LoRaMacMlmeRequest( MlmeReq_t *mlmeRequest ); 01669 01670 /*! 01671 * \brief LoRaMAC MCPS-Request 01672 * 01673 * \details The Mac Common Part Sublayer handles data services. The following 01674 * code-snippet shows how to use the API to send an unconfirmed 01675 * LoRaMAC frame. 01676 * 01677 * \code 01678 * uint8_t myBuffer[] = { 1, 2, 3 }; 01679 * 01680 * McpsReq_t mcpsReq; 01681 * mcpsReq.Type = MCPS_UNCONFIRMED; 01682 * mcpsReq.Req.Unconfirmed.fPort = 1; 01683 * mcpsReq.Req.Unconfirmed.fBuffer = myBuffer; 01684 * mcpsReq.Req.Unconfirmed.fBufferSize = sizeof( myBuffer ); 01685 * 01686 * if( LoRaMacMcpsRequest( &mcpsReq ) == LORAMAC_STATUS_OK ) 01687 * { 01688 * // Service started successfully. Waiting for the MCPS-Confirm event 01689 * } 01690 * \endcode 01691 * 01692 * \param [IN] mcpsRequest - MCPS-Request to perform. Refer to \ref McpsReq_t. 01693 * 01694 * \retval LoRaMacStatus_t Status of the operation. Possible returns are: 01695 * \ref LORAMAC_STATUS_OK, 01696 * \ref LORAMAC_STATUS_BUSY, 01697 * \ref LORAMAC_STATUS_SERVICE_UNKNOWN, 01698 * \ref LORAMAC_STATUS_PARAMETER_INVALID, 01699 * \ref LORAMAC_STATUS_NO_NETWORK_JOINED, 01700 * \ref LORAMAC_STATUS_LENGTH_ERROR, 01701 * \ref LORAMAC_STATUS_DEVICE_OFF. 01702 */ 01703 LoRaMacStatus_t LoRaMacMcpsRequest( McpsReq_t *mcpsRequest ); 01704 01705 /*! \} defgroup LORAMAC */ 01706 01707 #endif // __LORAMAC_H__
Generated on Tue Jul 12 2022 14:27:11 by
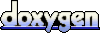