LoRaWAN MAC layer implementation
Dependents: LoRaWAN-demo-72_tjm LoRaWAN-demo-72_jlc LoRaWAN-demo-elmo frdm_LoRa_Connect_Woodstream_Demo_tjm ... more
LoRaMac-board.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2013 Semtech 00008 00009 Description: LoRa MAC layer board dependent definitions 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #ifndef __LORAMAC_BOARD_H__ 00016 #define __LORAMAC_BOARD_H__ 00017 00018 /*! 00019 * Returns individual channel mask 00020 * 00021 * \param[IN] channelIndex Channel index 1 based 00022 * \retval channelMask 00023 */ 00024 #define LC( channelIndex ) ( uint16_t )( 1 << ( channelIndex - 1 ) ) 00025 00026 #if defined( USE_BAND_433 ) 00027 00028 /*! 00029 * LoRaMac maximum number of channels 00030 */ 00031 #define LORA_MAX_NB_CHANNELS 16 00032 00033 /*! 00034 * Minimal datarate that can be used by the node 00035 */ 00036 #define LORAMAC_TX_MIN_DATARATE DR_0 00037 00038 /*! 00039 * Minimal datarate that can be used by the node 00040 */ 00041 #define LORAMAC_TX_MAX_DATARATE DR_7 00042 00043 /*! 00044 * Minimal datarate that can be used by the node 00045 */ 00046 #define LORAMAC_RX_MIN_DATARATE DR_0 00047 00048 /*! 00049 * Minimal datarate that can be used by the node 00050 */ 00051 #define LORAMAC_RX_MAX_DATARATE DR_7 00052 00053 /*! 00054 * Default datarate used by the node 00055 */ 00056 #define LORAMAC_DEFAULT_DATARATE DR_0 00057 00058 /*! 00059 * Minimal Rx1 receive datarate offset 00060 */ 00061 #define LORAMAC_MIN_RX1_DR_OFFSET 0 00062 00063 /*! 00064 * Maximal Rx1 receive datarate offset 00065 */ 00066 #define LORAMAC_MAX_RX1_DR_OFFSET 5 00067 00068 /*! 00069 * Minimal Tx output power that can be used by the node 00070 */ 00071 #define LORAMAC_MIN_TX_POWER TX_POWER_M5_DBM 00072 00073 /*! 00074 * Minimal Tx output power that can be used by the node 00075 */ 00076 #define LORAMAC_MAX_TX_POWER TX_POWER_10_DBM 00077 00078 /*! 00079 * Default Tx output power used by the node 00080 */ 00081 #define LORAMAC_DEFAULT_TX_POWER TX_POWER_10_DBM 00082 00083 /*! 00084 * LoRaMac TxPower definition 00085 */ 00086 #define TX_POWER_10_DBM 0 00087 #define TX_POWER_07_DBM 1 00088 #define TX_POWER_04_DBM 2 00089 #define TX_POWER_01_DBM 3 00090 #define TX_POWER_M2_DBM 4 00091 #define TX_POWER_M5_DBM 5 00092 00093 /*! 00094 * LoRaMac datarates definition 00095 */ 00096 #define DR_0 0 // SF12 - BW125 00097 #define DR_1 1 // SF11 - BW125 00098 #define DR_2 2 // SF10 - BW125 00099 #define DR_3 3 // SF9 - BW125 00100 #define DR_4 4 // SF8 - BW125 00101 #define DR_5 5 // SF7 - BW125 00102 #define DR_6 6 // SF7 - BW250 00103 #define DR_7 7 // FSK 00104 00105 /*! 00106 * Second reception window channel definition. 00107 */ 00108 // Channel = { Frequency [Hz], Datarate } 00109 #define RX_WND_2_CHANNEL { 434665000, DR_0 } 00110 00111 /*! 00112 * LoRaMac maximum number of bands 00113 */ 00114 #define LORA_MAX_NB_BANDS 1 00115 00116 // Band = { DutyCycle, TxMaxPower, LastTxDoneTime, TimeOff } 00117 #define BAND0 { 100, TX_POWER_10_DBM, 0, 0 } // 1.0 % 00118 00119 /*! 00120 * LoRaMac default channels 00121 */ 00122 // Channel = { Frequency [Hz], { ( ( DrMax << 4 ) | DrMin ) }, Band } 00123 #define LC1 { 433175000, { ( ( DR_5 << 4 ) | DR_0 ) }, 0 } 00124 #define LC2 { 433375000, { ( ( DR_5 << 4 ) | DR_0 ) }, 0 } 00125 #define LC3 { 433575000, { ( ( DR_5 << 4 ) | DR_0 ) }, 0 } 00126 00127 /*! 00128 * LoRaMac duty cycle for the join procedure 00129 */ 00130 #define JOIN_DC 1000 00131 00132 /*! 00133 * LoRaMac channels which are allowed for the join procedure 00134 */ 00135 #define JOIN_CHANNELS ( uint16_t )( LC( 1 ) | LC( 2 ) | LC( 3 ) ) 00136 00137 #elif defined( USE_BAND_780 ) 00138 00139 /*! 00140 * LoRaMac maximum number of channels 00141 */ 00142 #define LORA_MAX_NB_CHANNELS 16 00143 00144 /*! 00145 * Minimal datarate that can be used by the node 00146 */ 00147 #define LORAMAC_TX_MIN_DATARATE DR_0 00148 00149 /*! 00150 * Minimal datarate that can be used by the node 00151 */ 00152 #define LORAMAC_TX_MAX_DATARATE DR_7 00153 00154 /*! 00155 * Minimal datarate that can be used by the node 00156 */ 00157 #define LORAMAC_RX_MIN_DATARATE DR_0 00158 00159 /*! 00160 * Minimal datarate that can be used by the node 00161 */ 00162 #define LORAMAC_RX_MAX_DATARATE DR_7 00163 00164 /*! 00165 * Default datarate used by the node 00166 */ 00167 #define LORAMAC_DEFAULT_DATARATE DR_0 00168 00169 /*! 00170 * Minimal Rx1 receive datarate offset 00171 */ 00172 #define LORAMAC_MIN_RX1_DR_OFFSET 0 00173 00174 /*! 00175 * Maximal Rx1 receive datarate offset 00176 */ 00177 #define LORAMAC_MAX_RX1_DR_OFFSET 5 00178 00179 /*! 00180 * Minimal Tx output power that can be used by the node 00181 */ 00182 #define LORAMAC_MIN_TX_POWER TX_POWER_M5_DBM 00183 00184 /*! 00185 * Minimal Tx output power that can be used by the node 00186 */ 00187 #define LORAMAC_MAX_TX_POWER TX_POWER_10_DBM 00188 00189 /*! 00190 * Default Tx output power used by the node 00191 */ 00192 #define LORAMAC_DEFAULT_TX_POWER TX_POWER_10_DBM 00193 00194 /*! 00195 * LoRaMac TxPower definition 00196 */ 00197 #define TX_POWER_10_DBM 0 00198 #define TX_POWER_07_DBM 1 00199 #define TX_POWER_04_DBM 2 00200 #define TX_POWER_01_DBM 3 00201 #define TX_POWER_M2_DBM 4 00202 #define TX_POWER_M5_DBM 5 00203 00204 /*! 00205 * LoRaMac datarates definition 00206 */ 00207 #define DR_0 0 // SF12 - BW125 00208 #define DR_1 1 // SF11 - BW125 00209 #define DR_2 2 // SF10 - BW125 00210 #define DR_3 3 // SF9 - BW125 00211 #define DR_4 4 // SF8 - BW125 00212 #define DR_5 5 // SF7 - BW125 00213 #define DR_6 6 // SF7 - BW250 00214 #define DR_7 7 // FSK 00215 00216 /*! 00217 * Second reception window channel definition. 00218 */ 00219 // Channel = { Frequency [Hz], Datarate } 00220 #define RX_WND_2_CHANNEL { 786000000, DR_0 } 00221 00222 /*! 00223 * LoRaMac maximum number of bands 00224 */ 00225 #define LORA_MAX_NB_BANDS 1 00226 00227 // Band = { DutyCycle, TxMaxPower, LastTxDoneTime, TimeOff } 00228 #define BAND0 { 100, TX_POWER_10_DBM, 0, 0 } // 1.0 % 00229 00230 /*! 00231 * LoRaMac default channels 00232 */ 00233 // Channel = { Frequency [Hz], { ( ( DrMax << 4 ) | DrMin ) }, Band } 00234 #define LC1 { 779500000, { ( ( DR_5 << 4 ) | DR_0 ) }, 0 } 00235 #define LC2 { 779700000, { ( ( DR_5 << 4 ) | DR_0 ) }, 0 } 00236 #define LC3 { 779900000, { ( ( DR_5 << 4 ) | DR_0 ) }, 0 } 00237 00238 /*! 00239 * LoRaMac duty cycle for the join procedure 00240 */ 00241 #define JOIN_DC 1000 00242 00243 /*! 00244 * LoRaMac channels which are allowed for the join procedure 00245 */ 00246 #define JOIN_CHANNELS ( uint16_t )( LC( 1 ) | LC( 2 ) | LC( 3 ) ) 00247 00248 #elif defined( USE_BAND_868 ) 00249 00250 /*! 00251 * LoRaMac maximum number of channels 00252 */ 00253 #define LORA_MAX_NB_CHANNELS 16 00254 00255 /*! 00256 * Minimal datarate that can be used by the node 00257 */ 00258 #define LORAMAC_TX_MIN_DATARATE DR_0 00259 00260 /*! 00261 * Minimal datarate that can be used by the node 00262 */ 00263 #define LORAMAC_TX_MAX_DATARATE DR_7 00264 00265 /*! 00266 * Minimal datarate that can be used by the node 00267 */ 00268 #define LORAMAC_RX_MIN_DATARATE DR_0 00269 00270 /*! 00271 * Minimal datarate that can be used by the node 00272 */ 00273 #define LORAMAC_RX_MAX_DATARATE DR_7 00274 00275 /*! 00276 * Default datarate used by the node 00277 */ 00278 #define LORAMAC_DEFAULT_DATARATE DR_0 00279 00280 /*! 00281 * Minimal Rx1 receive datarate offset 00282 */ 00283 #define LORAMAC_MIN_RX1_DR_OFFSET 0 00284 00285 /*! 00286 * Maximal Rx1 receive datarate offset 00287 */ 00288 #define LORAMAC_MAX_RX1_DR_OFFSET 5 00289 00290 /*! 00291 * Minimal Tx output power that can be used by the node 00292 */ 00293 #define LORAMAC_MIN_TX_POWER TX_POWER_02_DBM 00294 00295 /*! 00296 * Minimal Tx output power that can be used by the node 00297 */ 00298 #define LORAMAC_MAX_TX_POWER TX_POWER_20_DBM 00299 00300 /*! 00301 * Default Tx output power used by the node 00302 */ 00303 #define LORAMAC_DEFAULT_TX_POWER TX_POWER_14_DBM 00304 00305 /*! 00306 * LoRaMac TxPower definition 00307 */ 00308 #define TX_POWER_20_DBM 0 00309 #define TX_POWER_14_DBM 1 00310 #define TX_POWER_11_DBM 2 00311 #define TX_POWER_08_DBM 3 00312 #define TX_POWER_05_DBM 4 00313 #define TX_POWER_02_DBM 5 00314 00315 /*! 00316 * LoRaMac datarates definition 00317 */ 00318 #define DR_0 0 // SF12 - BW125 00319 #define DR_1 1 // SF11 - BW125 00320 #define DR_2 2 // SF10 - BW125 00321 #define DR_3 3 // SF9 - BW125 00322 #define DR_4 4 // SF8 - BW125 00323 #define DR_5 5 // SF7 - BW125 00324 #define DR_6 6 // SF7 - BW250 00325 #define DR_7 7 // FSK 00326 00327 /*! 00328 * Second reception window channel definition. 00329 */ 00330 // Channel = { Frequency [Hz], Datarate } 00331 #define RX_WND_2_CHANNEL { 869525000, DR_0 } 00332 00333 /*! 00334 * LoRaMac maximum number of bands 00335 */ 00336 #define LORA_MAX_NB_BANDS 5 00337 00338 /*! 00339 * LoRaMac EU868 default bands 00340 */ 00341 typedef enum 00342 { 00343 BAND_G1_0, 00344 BAND_G1_1, 00345 BAND_G1_2, 00346 BAND_G1_3, 00347 BAND_G1_4, 00348 }BandId_t; 00349 00350 // Band = { DutyCycle, TxMaxPower, LastTxDoneTime, TimeOff } 00351 #define BAND0 { 100 , TX_POWER_14_DBM, 0, 0 } // 1.0 % 00352 #define BAND1 { 100 , TX_POWER_14_DBM, 0, 0 } // 1.0 % 00353 #define BAND2 { 1000, TX_POWER_14_DBM, 0, 0 } // 0.1 % 00354 #define BAND3 { 10 , TX_POWER_14_DBM, 0, 0 } // 10.0 % 00355 #define BAND4 { 100 , TX_POWER_14_DBM, 0, 0 } // 1.0 % 00356 00357 /*! 00358 * LoRaMac default channels 00359 */ 00360 // Channel = { Frequency [Hz], { ( ( DrMax << 4 ) | DrMin ) }, Band } 00361 #define LC1 { 868100000, { ( ( DR_5 << 4 ) | DR_0 ) }, 1 } 00362 #define LC2 { 868300000, { ( ( DR_5 << 4 ) | DR_0 ) }, 1 } 00363 #define LC3 { 868500000, { ( ( DR_5 << 4 ) | DR_0 ) }, 1 } 00364 00365 /*! 00366 * LoRaMac duty cycle for the join procedure 00367 */ 00368 #define JOIN_DC 1000 00369 00370 /*! 00371 * LoRaMac channels which are allowed for the join procedure 00372 */ 00373 #define JOIN_CHANNELS ( uint16_t )( LC( 1 ) | LC( 2 ) | LC( 3 ) ) 00374 00375 #elif defined( USE_BAND_915 ) || defined( USE_BAND_915_HYBRID ) 00376 00377 /*! 00378 * LoRaMac maximum number of channels 00379 */ 00380 #define LORA_MAX_NB_CHANNELS 72 00381 00382 /*! 00383 * Minimal datarate that can be used by the node 00384 */ 00385 #define LORAMAC_TX_MIN_DATARATE DR_0 00386 00387 /*! 00388 * Minimal datarate that can be used by the node 00389 */ 00390 #define LORAMAC_TX_MAX_DATARATE DR_4 00391 00392 /*! 00393 * Minimal datarate that can be used by the node 00394 */ 00395 #define LORAMAC_RX_MIN_DATARATE DR_8 00396 00397 /*! 00398 * Minimal datarate that can be used by the node 00399 */ 00400 #define LORAMAC_RX_MAX_DATARATE DR_13 00401 00402 /*! 00403 * Default datarate used by the node 00404 */ 00405 #define LORAMAC_DEFAULT_DATARATE DR_0 00406 00407 /*! 00408 * Minimal Rx1 receive datarate offset 00409 */ 00410 #define LORAMAC_MIN_RX1_DR_OFFSET 0 00411 00412 /*! 00413 * Maximal Rx1 receive datarate offset 00414 */ 00415 #define LORAMAC_MAX_RX1_DR_OFFSET 3 00416 00417 /*! 00418 * Minimal Tx output power that can be used by the node 00419 */ 00420 #define LORAMAC_MIN_TX_POWER TX_POWER_10_DBM 00421 00422 /*! 00423 * Minimal Tx output power that can be used by the node 00424 */ 00425 #define LORAMAC_MAX_TX_POWER TX_POWER_30_DBM 00426 00427 /*! 00428 * Default Tx output power used by the node 00429 */ 00430 #define LORAMAC_DEFAULT_TX_POWER TX_POWER_20_DBM 00431 00432 /*! 00433 * LoRaMac TxPower definition 00434 */ 00435 #define TX_POWER_30_DBM 0 00436 #define TX_POWER_28_DBM 1 00437 #define TX_POWER_26_DBM 2 00438 #define TX_POWER_24_DBM 3 00439 #define TX_POWER_22_DBM 4 00440 #define TX_POWER_20_DBM 5 00441 #define TX_POWER_18_DBM 6 00442 #define TX_POWER_16_DBM 7 00443 #define TX_POWER_14_DBM 8 00444 #define TX_POWER_12_DBM 9 00445 #define TX_POWER_10_DBM 10 00446 00447 /*! 00448 * LoRaMac datarates definition 00449 */ 00450 #define DR_0 0 // SF10 - BW125 | 00451 #define DR_1 1 // SF9 - BW125 | 00452 #define DR_2 2 // SF8 - BW125 +-> Up link 00453 #define DR_3 3 // SF7 - BW125 | 00454 #define DR_4 4 // SF8 - BW500 | 00455 #define DR_5 5 // RFU 00456 #define DR_6 6 // RFU 00457 #define DR_7 7 // RFU 00458 #define DR_8 8 // SF12 - BW500 | 00459 #define DR_9 9 // SF11 - BW500 | 00460 #define DR_10 10 // SF10 - BW500 | 00461 #define DR_11 11 // SF9 - BW500 | 00462 #define DR_12 12 // SF8 - BW500 +-> Down link 00463 #define DR_13 13 // SF7 - BW500 | 00464 #define DR_14 14 // RFU | 00465 #define DR_15 15 // RFU | 00466 00467 /*! 00468 * Second reception window channel definition. 00469 */ 00470 // Channel = { Frequency [Hz], Datarate } 00471 #define RX_WND_2_CHANNEL { 923300000, DR_8 } 00472 00473 /*! 00474 * LoRaMac maximum number of bands 00475 */ 00476 #define LORA_MAX_NB_BANDS 1 00477 00478 // Band = { DutyCycle, TxMaxPower, LastTxDoneTime, TimeOff } 00479 #define BAND0 { 1, TX_POWER_20_DBM, 0, 0 } // 100.0 % 00480 00481 /*! 00482 * LoRaMac default channels 00483 */ 00484 // Channel = { Frequency [Hz], { ( ( DrMax << 4 ) | DrMin ) }, Band } 00485 /* 00486 * US band channels are initialized using a loop in LoRaMacInit function 00487 * \code 00488 * // 125 kHz channels 00489 * for( uint8_t i = 0; i < LORA_MAX_NB_CHANNELS - 8; i++ ) 00490 * { 00491 * Channels[i].Frequency = 902.3e6 + i * 200e3; 00492 * Channels[i].DrRange.Value = ( DR_3 << 4 ) | DR_0; 00493 * Channels[i].Band = 0; 00494 * } 00495 * // 500 kHz channels 00496 * for( uint8_t i = LORA_MAX_NB_CHANNELS - 8; i < LORA_MAX_NB_CHANNELS; i++ ) 00497 * { 00498 * Channels[i].Frequency = 903.0e6 + ( i - ( LORA_MAX_NB_CHANNELS - 8 ) ) * 1.6e6; 00499 * Channels[i].DrRange.Value = ( DR_4 << 4 ) | DR_4; 00500 * Channels[i].Band = 0; 00501 * } 00502 * \endcode 00503 */ 00504 #else 00505 #error "Please define a frequency band in the compiler options." 00506 #endif 00507 00508 #endif // __LORAMAC_BOARD_H__
Generated on Tue Jul 12 2022 14:27:10 by
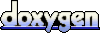