LoRaWAN MAC layer implementation
Dependents: LoRaWAN-demo-72_tjm LoRaWAN-demo-72_jlc LoRaWAN-demo-elmo frdm_LoRa_Connect_Woodstream_Demo_tjm ... more
LoRaMac-api-v3.h
00001 /*! 00002 * \file LoRaMac-api-v3.h 00003 * 00004 * \brief LoRa MAC wrapper layer implementation 00005 * 00006 * \copyright Revised BSD License, see section \ref LICENSE. 00007 * 00008 * \code 00009 * ______ _ 00010 * / _____) _ | | 00011 * ( (____ _____ ____ _| |_ _____ ____| |__ 00012 * \____ \| ___ | (_ _) ___ |/ ___) _ \ 00013 * _____) ) ____| | | || |_| ____( (___| | | | 00014 * (______/|_____)_|_|_| \__)_____)\____)_| |_| 00015 * (C)2013 Semtech 00016 * 00017 * ___ _____ _ ___ _ _____ ___ ___ ___ ___ 00018 * / __|_ _/_\ / __| |/ / __/ _ \| _ \/ __| __| 00019 * \__ \ | |/ _ \ (__| ' <| _| (_) | / (__| _| 00020 * |___/ |_/_/ \_\___|_|\_\_| \___/|_|_\\___|___| 00021 * embedded.connectivity.solutions=============== 00022 * 00023 * \endcode 00024 * 00025 * \author Miguel Luis ( Semtech ) 00026 * 00027 * \author Gregory Cristian ( Semtech ) 00028 * 00029 * \author Daniel Jäckle ( STACKFORCE ) 00030 */ 00031 #ifndef __LORAMAC_API_V3_H__ 00032 #define __LORAMAC_API_V3_H__ 00033 00034 // Includes board dependent definitions such as channels frequencies 00035 #include "LoRaMac.h" 00036 #include "LoRaMac-board.h" 00037 00038 /*! 00039 * Beacon interval in us 00040 */ 00041 #define BEACON_INTERVAL 128000000 00042 00043 /*! 00044 * Class A&B receive delay 1 in us 00045 */ 00046 #define RECEIVE_DELAY1 1000000 00047 00048 /*! 00049 * Class A&B receive delay 2 in us 00050 */ 00051 #define RECEIVE_DELAY2 2000000 00052 00053 /*! 00054 * Join accept receive delay 1 in us 00055 */ 00056 #define JOIN_ACCEPT_DELAY1 5000000 00057 00058 /*! 00059 * Join accept receive delay 2 in us 00060 */ 00061 #define JOIN_ACCEPT_DELAY2 6000000 00062 00063 /*! 00064 * Class A&B maximum receive window delay in us 00065 */ 00066 #define MAX_RX_WINDOW 3000000 00067 00068 /*! 00069 * Maximum allowed gap for the FCNT field 00070 */ 00071 #define MAX_FCNT_GAP 16384 00072 00073 /*! 00074 * ADR acknowledgement counter limit 00075 */ 00076 #define ADR_ACK_LIMIT 64 00077 00078 /*! 00079 * Number of ADR acknowledgement requests before returning to default datarate 00080 */ 00081 #define ADR_ACK_DELAY 32 00082 00083 /*! 00084 * Number of seconds after the start of the second reception window without 00085 * receiving an acknowledge. 00086 * AckTimeout = \ref ACK_TIMEOUT + Random( -\ref ACK_TIMEOUT_RND, \ref ACK_TIMEOUT_RND ) 00087 */ 00088 #define ACK_TIMEOUT 2000000 00089 00090 /*! 00091 * Random number of seconds after the start of the second reception window without 00092 * receiving an acknowledge 00093 * AckTimeout = \ref ACK_TIMEOUT + Random( -\ref ACK_TIMEOUT_RND, \ref ACK_TIMEOUT_RND ) 00094 */ 00095 #define ACK_TIMEOUT_RND 1000000 00096 00097 /*! 00098 * Check the Mac layer state every MAC_STATE_CHECK_TIMEOUT in us 00099 */ 00100 #define MAC_STATE_CHECK_TIMEOUT 1000000 00101 00102 /*! 00103 * Maximum number of times the MAC layer tries to get an acknowledge. 00104 */ 00105 #define MAX_ACK_RETRIES 8 00106 00107 /*! 00108 * RSSI free threshold [dBm] 00109 */ 00110 #define RSSI_FREE_TH ( int8_t )( -90 ) 00111 00112 /*! 00113 * Frame direction definition for up-link communications 00114 */ 00115 #define UP_LINK 0 00116 00117 /*! 00118 * Frame direction definition for down-link communications 00119 */ 00120 #define DOWN_LINK 1 00121 00122 /*! 00123 * Sets the length of the LoRaMAC footer field. 00124 * Mainly indicates the MIC field length 00125 */ 00126 #define LORAMAC_MFR_LEN 4 00127 00128 /*! 00129 * Syncword for Private LoRa networks 00130 */ 00131 #define LORA_MAC_PRIVATE_SYNCWORD 0x12 00132 00133 /*! 00134 * Syncword for Public LoRa networks 00135 */ 00136 #define LORA_MAC_PUBLIC_SYNCWORD 0x34 00137 00138 /*! 00139 * LoRaMAC event flags 00140 */ 00141 typedef union 00142 { 00143 uint8_t Value; 00144 struct 00145 { 00146 uint8_t Tx : 1; 00147 uint8_t Rx : 1; 00148 uint8_t RxData : 1; 00149 uint8_t Multicast : 1; 00150 uint8_t RxSlot : 2; 00151 uint8_t LinkCheck : 1; 00152 uint8_t JoinAccept : 1; 00153 }Bits; 00154 }LoRaMacEventFlags_t ; 00155 00156 /*! 00157 * LoRaMAC event information 00158 */ 00159 typedef struct 00160 { 00161 LoRaMacEventInfoStatus_t Status; 00162 bool TxAckReceived; 00163 uint8_t TxNbRetries; 00164 uint8_t TxDatarate; 00165 uint8_t RxPort; 00166 uint8_t *RxBuffer; 00167 uint8_t RxBufferSize; 00168 int16_t RxRssi; 00169 uint8_t RxSnr; 00170 uint16_t Energy; 00171 uint8_t DemodMargin; 00172 uint8_t NbGateways; 00173 }LoRaMacEventInfo_t ; 00174 00175 /*! 00176 * LoRaMAC events structure 00177 * Used to notify upper layers of MAC events 00178 */ 00179 typedef struct sLoRaMacCallbacks 00180 { 00181 /*! 00182 * MAC layer event callback prototype. 00183 * 00184 * \param [IN] flags Bit field indicating the MAC events occurred 00185 * \param [IN] info Details about MAC events occurred 00186 */ 00187 void ( *MacEvent )( LoRaMacEventFlags_t *flags, LoRaMacEventInfo_t *info ); 00188 /*! 00189 * Function callback to get the current battery level 00190 * 00191 * \retval batteryLevel Current battery level 00192 */ 00193 uint8_t ( *GetBatteryLevel )( void ); 00194 }LoRaMacCallbacks_t ; 00195 00196 /*! 00197 * LoRaMAC layer initialization 00198 * 00199 * \param [IN] callbacks Pointer to a structure defining the LoRaMAC 00200 * callback functions. 00201 */ 00202 void LoRaMacInit( LoRaMacCallbacks_t *callbacks ); 00203 00204 /*! 00205 * Enables/Disables the ADR (Adaptive Data Rate) 00206 * 00207 * \param [IN] enable [true: ADR ON, false: ADR OFF] 00208 */ 00209 void LoRaMacSetAdrOn( bool enable ); 00210 00211 /*! 00212 * Initializes the network IDs. Device address, 00213 * network session AES128 key and application session AES128 key. 00214 * 00215 * \remark To be only used when Over-the-Air activation isn't used. 00216 * 00217 * \param [IN] netID 24 bits network identifier 00218 * ( provided by network operator ) 00219 * \param [IN] devAddr 32 bits device address on the network 00220 * (must be unique to the network) 00221 * \param [IN] nwkSKey Pointer to the network session AES128 key array 00222 * ( 16 bytes ) 00223 * \param [IN] appSKey Pointer to the application session AES128 key array 00224 * ( 16 bytes ) 00225 */ 00226 void LoRaMacInitNwkIds( uint32_t netID, uint32_t devAddr, uint8_t *nwkSKey, uint8_t *appSKey ); 00227 00228 /* 00229 * Wrapper function which calls \ref LoRaMacMulticastChannelLink. 00230 */ 00231 void LoRaMacMulticastChannelAdd( MulticastParams_t *channelParam ); 00232 00233 /* 00234 * Wrapper function which calls \ref LoRaMacMulticastChannelUnlink. 00235 */ 00236 void LoRaMacMulticastChannelRemove( MulticastParams_t *channelParam ); 00237 00238 /*! 00239 * Initiates the Over-the-Air activation 00240 * 00241 * \param [IN] devEui Pointer to the device EUI array ( 8 bytes ) 00242 * \param [IN] appEui Pointer to the application EUI array ( 8 bytes ) 00243 * \param [IN] appKey Pointer to the application AES128 key array ( 16 bytes ) 00244 * 00245 * \retval status [0: OK, 1: Tx error, 2: Already joined a network] 00246 */ 00247 uint8_t LoRaMacJoinReq( uint8_t *devEui, uint8_t *appEui, uint8_t *appKey ); 00248 00249 /*! 00250 * Sends a LinkCheckReq MAC command on the next uplink frame 00251 * 00252 * \retval status Function status [0: OK, 1: Busy] 00253 */ 00254 uint8_t LoRaMacLinkCheckReq( void ); 00255 00256 /*! 00257 * LoRaMAC layer send frame 00258 * 00259 * \param [IN] fPort MAC payload port (must be > 0) 00260 * \param [IN] fBuffer MAC data buffer to be sent 00261 * \param [IN] fBufferSize MAC data buffer size 00262 * 00263 * \retval status [0: OK, 1: Busy, 2: No network joined, 00264 * 3: Length or port error, 4: Unknown MAC command 00265 * 5: Unable to find a free channel 00266 * 6: Device switched off] 00267 */ 00268 uint8_t LoRaMacSendFrame( uint8_t fPort, void *fBuffer, uint16_t fBufferSize ); 00269 00270 /*! 00271 * LoRaMAC layer send frame 00272 * 00273 * \param [IN] fPort MAC payload port (must be > 0) 00274 * \param [IN] fBuffer MAC data buffer to be sent 00275 * \param [IN] fBufferSize MAC data buffer size 00276 * \param [IN] fBufferSize MAC data buffer size 00277 * \param [IN] nbRetries Number of retries to receive the acknowledgement 00278 * 00279 * \retval status [0: OK, 1: Busy, 2: No network joined, 00280 * 3: Length or port error, 4: Unknown MAC command 00281 * 5: Unable to find a free channel 00282 * 6: Device switched off] 00283 */ 00284 uint8_t LoRaMacSendConfirmedFrame( uint8_t fPort, void *fBuffer, uint16_t fBufferSize, uint8_t nbRetries ); 00285 00286 /*! 00287 * ============================================================================ 00288 * = LoRaMac test functions = 00289 * ============================================================================ 00290 */ 00291 00292 /*! 00293 * LoRaMAC layer generic send frame 00294 * 00295 * \param [IN] macHdr MAC header field 00296 * \param [IN] fOpts MAC commands buffer 00297 * \param [IN] fPort MAC payload port 00298 * \param [IN] fBuffer MAC data buffer to be sent 00299 * \param [IN] fBufferSize MAC data buffer size 00300 * \retval status [0: OK, 1: Busy, 2: No network joined, 00301 * 3: Length or port error, 4: Unknown MAC command 00302 * 5: Unable to find a free channel 00303 * 6: Device switched off] 00304 */ 00305 uint8_t LoRaMacSend( LoRaMacHeader_t *macHdr, uint8_t *fOpts, uint8_t fPort, void *fBuffer, uint16_t fBufferSize ); 00306 00307 /*! 00308 * LoRaMAC layer frame buffer initialization. 00309 * 00310 * \param [IN] channel Channel parameters 00311 * \param [IN] macHdr MAC header field 00312 * \param [IN] fCtrl MAC frame control field 00313 * \param [IN] fOpts MAC commands buffer 00314 * \param [IN] fPort MAC payload port 00315 * \param [IN] fBuffer MAC data buffer to be sent 00316 * \param [IN] fBufferSize MAC data buffer size 00317 * \retval status [0: OK, 1: N/A, 2: No network joined, 00318 * 3: Length or port error, 4: Unknown MAC command] 00319 */ 00320 uint8_t LoRaMacPrepareFrame( ChannelParams_t channel,LoRaMacHeader_t *macHdr, LoRaMacFrameCtrl_t *fCtrl, uint8_t *fOpts, uint8_t fPort, void *fBuffer, uint16_t fBufferSize ); 00321 00322 /*! 00323 * LoRaMAC layer prepared frame buffer transmission with channel specification 00324 * 00325 * \remark LoRaMacPrepareFrame must be called at least once before calling this 00326 * function. 00327 * 00328 * \param [IN] channel Channel parameters 00329 * \retval status [0: OK, 1: Busy] 00330 */ 00331 uint8_t LoRaMacSendFrameOnChannel( ChannelParams_t channel ); 00332 00333 /*! 00334 * LoRaMAC layer generic send frame with channel specification 00335 * 00336 * \param [IN] channel Channel parameters 00337 * \param [IN] macHdr MAC header field 00338 * \param [IN] fCtrl MAC frame control field 00339 * \param [IN] fOpts MAC commands buffer 00340 * \param [IN] fPort MAC payload port 00341 * \param [IN] fBuffer MAC data buffer to be sent 00342 * \param [IN] fBufferSize MAC data buffer size 00343 * \retval status [0: OK, 1: Busy, 2: No network joined, 00344 * 3: Length or port error, 4: Unknown MAC command] 00345 */ 00346 uint8_t LoRaMacSendOnChannel( ChannelParams_t channel, LoRaMacHeader_t *macHdr, LoRaMacFrameCtrl_t *fCtrl, uint8_t *fOpts, uint8_t fPort, void *fBuffer, uint16_t fBufferSize ); 00347 00348 /*! 00349 * ============================================================================ 00350 * = LoRaMac setup functions = 00351 * ============================================================================ 00352 */ 00353 00354 /* 00355 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00356 * set the LoRaWan device class. 00357 */ 00358 void LoRaMacSetDeviceClass( DeviceClass_t deviceClass ); 00359 00360 /* 00361 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00362 * set the network type to public or private. 00363 */ 00364 void LoRaMacSetPublicNetwork( bool enable ); 00365 00366 /* 00367 * Wrapper function which calls \ref LoRaMacChannelAdd. 00368 */ 00369 void LoRaMacSetChannel( uint8_t id, ChannelParams_t params ); 00370 00371 /* 00372 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00373 * set the receive window 2 channel. 00374 */ 00375 void LoRaMacSetRx2Channel( Rx2ChannelParams_t param ); 00376 00377 /*! 00378 * Sets channels tx output power 00379 * 00380 * \param [IN] txPower [TX_POWER_20_DBM, TX_POWER_14_DBM, 00381 TX_POWER_11_DBM, TX_POWER_08_DBM, 00382 TX_POWER_05_DBM, TX_POWER_02_DBM] 00383 */ 00384 void LoRaMacSetChannelsTxPower( int8_t txPower ); 00385 00386 /*! 00387 * Sets channels datarate 00388 * 00389 * \param [IN] datarate eu868 - [DR_0, DR_1, DR_2, DR_3, DR_4, DR_5, DR_6, DR_7] 00390 * us915 - [DR_0, DR_1, DR_2, DR_3, DR_4] 00391 */ 00392 void LoRaMacSetChannelsDatarate( int8_t datarate ); 00393 00394 /* 00395 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00396 * set the channels mask. 00397 */ 00398 void LoRaMacSetChannelsMask( uint16_t *mask ); 00399 00400 /* 00401 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00402 * set the number of repetitions on a channel. 00403 */ 00404 void LoRaMacSetChannelsNbRep( uint8_t nbRep ); 00405 00406 /* 00407 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00408 * set the maximum receive window duration in [us]. 00409 */ 00410 void LoRaMacSetMaxRxWindow( uint32_t delay ); 00411 00412 /* 00413 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00414 * set the receive delay 1 in [us]. 00415 */ 00416 void LoRaMacSetReceiveDelay1( uint32_t delay ); 00417 00418 /* 00419 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00420 * set the receive delay 2 in [us]. 00421 */ 00422 void LoRaMacSetReceiveDelay2( uint32_t delay ); 00423 00424 /* 00425 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00426 * set the join accept delay 1 in [us]. 00427 */ 00428 void LoRaMacSetJoinAcceptDelay1( uint32_t delay ); 00429 00430 /* 00431 * Wrapper function which calls \ref LoRaMacMibSetRequestConfirm to 00432 * set the join accept delay 2 in [us]. 00433 */ 00434 void LoRaMacSetJoinAcceptDelay2( uint32_t delay ); 00435 00436 /* 00437 * Wrapper function which calls \ref LoRaMacMibGetRequestConfirm to 00438 * get the up-link counter. 00439 */ 00440 uint32_t LoRaMacGetUpLinkCounter( void ); 00441 00442 /* 00443 * Wrapper function which calls \ref LoRaMacMibGetRequestConfirm to 00444 * get the down-link counter. 00445 */ 00446 uint32_t LoRaMacGetDownLinkCounter( void ); 00447 00448 /* 00449 * ============================================================================ 00450 * = LoRaMac test functions = 00451 * ============================================================================ 00452 */ 00453 00454 /*! 00455 * Disables/Enables the duty cycle enforcement (EU868) 00456 * 00457 * \param [IN] enable - Enabled or disables the duty cycle 00458 */ 00459 void LoRaMacTestSetDutyCycleOn ( bool enable ); 00460 00461 /*! 00462 * Disables/Enables the reception windows opening 00463 * 00464 * \param [IN] enable [true: enable, false: disable] 00465 */ 00466 void LoRaMacTestRxWindowsOn ( bool enable ); 00467 00468 /*! 00469 * Enables the MIC field test 00470 * 00471 * \param [IN] upLinkCounter Fixed Tx packet counter value 00472 */ 00473 void LoRaMacTestSetMic ( uint16_t upLinkCounter ); 00474 00475 #endif /* __LORAMAC_API_V3_H__ */
Generated on Tue Jul 12 2022 14:27:10 by
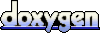