LoRaWAN MAC layer implementation
Dependents: LoRaWAN-demo-72_tjm LoRaWAN-demo-72_jlc LoRaWAN-demo-elmo frdm_LoRa_Connect_Woodstream_Demo_tjm ... more
LoRaMac-api-v3.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2013 Semtech 00008 ___ _____ _ ___ _ _____ ___ ___ ___ ___ 00009 / __|_ _/_\ / __| |/ / __/ _ \| _ \/ __| __| 00010 \__ \ | |/ _ \ (__| ' <| _| (_) | / (__| _| 00011 |___/ |_/_/ \_\___|_|\_\_| \___/|_|_\\___|___| 00012 embedded.connectivity.solutions=============== 00013 00014 Description: LoRa MAC layer implementation 00015 00016 License: Revised BSD License, see LICENSE.TXT file include in the project 00017 00018 Maintainer: Miguel Luis ( Semtech ), Gregory Cristian ( Semtech ) and Daniel Jäckle ( STACKFORCE ) 00019 */ 00020 #include "board.h" 00021 00022 #include "LoRaMac-api-v3.h" 00023 #include "LoRaMacTest.h" 00024 00025 /*! 00026 * Extern function declarations. 00027 */ 00028 extern LoRaMacStatus_t Send( LoRaMacHeader_t *macHdr, uint8_t fPort, 00029 void *fBuffer, uint16_t fBufferSize ); 00030 extern LoRaMacStatus_t PrepareFrame( LoRaMacHeader_t *macHdr, LoRaMacFrameCtrl_t *fCtrl, 00031 uint8_t fPort, void *fBuffer, uint16_t fBufferSize ); 00032 extern LoRaMacStatus_t SendFrameOnChannel( ChannelParams_t channel ); 00033 extern uint32_t LoRaMacState; 00034 extern LoRaMacFlags_t LoRaMacFlags; 00035 00036 /*! 00037 * Static variables 00038 */ 00039 static LoRaMacEventFlags_t LoRaMacEventFlags; 00040 static LoRaMacEventInfo_t LoRaMacEventInfo; 00041 static LoRaMacPrimitives_t LoRaMacPrimitives; 00042 static LoRaMacCallback_t LoRaMacCallback; 00043 static LoRaMacCallbacks_t LoRaMacCallbacks; 00044 00045 /*! 00046 * \brief MCPS-Confirm event function 00047 * 00048 * \param [IN] mcpsConfirm - Pointer to the confirm structure, 00049 * containing confirm attributes. 00050 */ 00051 static void McpsConfirm( McpsConfirm_t *mcpsConfirm ) 00052 { 00053 LoRaMacEventInfo.Status = mcpsConfirm->Status ; 00054 LoRaMacEventFlags.Bits.Tx = 1; 00055 00056 LoRaMacEventInfo.TxDatarate = mcpsConfirm->Datarate ; 00057 LoRaMacEventInfo.TxNbRetries = mcpsConfirm->NbRetries ; 00058 LoRaMacEventInfo.TxAckReceived = mcpsConfirm->AckReceived ; 00059 00060 if( ( LoRaMacFlags.Bits.McpsInd != 1 ) && ( LoRaMacFlags.Bits.MlmeReq != 1 ) ) 00061 { 00062 LoRaMacCallbacks.MacEvent ( &LoRaMacEventFlags, &LoRaMacEventInfo ); 00063 LoRaMacEventFlags.Value = 0; 00064 } 00065 } 00066 00067 /*! 00068 * \brief MCPS-Indication event function 00069 * 00070 * \param [IN] mcpsIndication - Pointer to the indication structure, 00071 * containing indication attributes. 00072 */ 00073 static void McpsIndication( McpsIndication_t *mcpsIndication ) 00074 { 00075 LoRaMacEventInfo.Status = mcpsIndication->Status ; 00076 LoRaMacEventFlags.Bits.Rx = 1; 00077 LoRaMacEventFlags.Bits.RxSlot = mcpsIndication->RxSlot ; 00078 LoRaMacEventFlags.Bits.Multicast = mcpsIndication->Multicast ; 00079 if( mcpsIndication->RxData == true ) 00080 { 00081 LoRaMacEventFlags.Bits.RxData = 1; 00082 } 00083 00084 LoRaMacEventInfo.RxPort = mcpsIndication->Port ; 00085 LoRaMacEventInfo.RxBuffer = mcpsIndication->Buffer ; 00086 LoRaMacEventInfo.RxBufferSize = mcpsIndication->BufferSize ; 00087 LoRaMacEventInfo.RxRssi = mcpsIndication->Rssi ; 00088 LoRaMacEventInfo.RxSnr = mcpsIndication->Snr ; 00089 00090 LoRaMacCallbacks.MacEvent ( &LoRaMacEventFlags, &LoRaMacEventInfo ); 00091 LoRaMacEventFlags.Value = 0; 00092 } 00093 00094 /*! 00095 * \brief MLME-Confirm event function 00096 * 00097 * \param [IN] mlmeConfirm - Pointer to the confirm structure, 00098 * containing confirm attributes. 00099 */ 00100 static void MlmeConfirm( MlmeConfirm_t *mlmeConfirm ) 00101 { 00102 if( mlmeConfirm->Status == LORAMAC_EVENT_INFO_STATUS_OK ) 00103 { 00104 switch( mlmeConfirm->MlmeRequest ) 00105 { 00106 case MLME_JOIN : 00107 { 00108 // Status is OK, node has joined the network 00109 LoRaMacEventFlags.Bits.Tx = 1; 00110 LoRaMacEventFlags.Bits.Rx = 1; 00111 LoRaMacEventFlags.Bits.JoinAccept = 1; 00112 break; 00113 } 00114 case MLME_LINK_CHECK : 00115 { 00116 LoRaMacEventFlags.Bits.Tx = 1; 00117 LoRaMacEventFlags.Bits.Rx = 1; 00118 LoRaMacEventFlags.Bits.LinkCheck = 1; 00119 00120 LoRaMacEventInfo.DemodMargin = mlmeConfirm->DemodMargin ; 00121 LoRaMacEventInfo.NbGateways = mlmeConfirm->NbGateways ; 00122 break; 00123 } 00124 default: 00125 break; 00126 } 00127 } 00128 LoRaMacEventInfo.Status = mlmeConfirm->Status ; 00129 00130 if( LoRaMacFlags.Bits.McpsInd != 1 ) 00131 { 00132 LoRaMacCallbacks.MacEvent ( &LoRaMacEventFlags, &LoRaMacEventInfo ); 00133 LoRaMacEventFlags.Value = 0; 00134 } 00135 } 00136 00137 void LoRaMacInit( LoRaMacCallbacks_t *callbacks ) 00138 { 00139 LoRaMacPrimitives.MacMcpsConfirm = McpsConfirm; 00140 LoRaMacPrimitives.MacMcpsIndication = McpsIndication; 00141 LoRaMacPrimitives.MacMlmeConfirm = MlmeConfirm; 00142 00143 LoRaMacCallbacks.MacEvent = callbacks->MacEvent ; 00144 LoRaMacCallbacks.GetBatteryLevel = callbacks->GetBatteryLevel ; 00145 LoRaMacCallback.GetBatteryLevel = callbacks->GetBatteryLevel ; 00146 00147 LoRaMacInitialization( &LoRaMacPrimitives, &LoRaMacCallback ); 00148 } 00149 00150 void LoRaMacSetAdrOn( bool enable ) 00151 { 00152 MibRequestConfirm_t mibSet; 00153 00154 mibSet.Type = MIB_ADR ; 00155 mibSet.Param .AdrEnable = enable; 00156 00157 LoRaMacMibSetRequestConfirm( &mibSet ); 00158 } 00159 00160 void LoRaMacInitNwkIds( uint32_t netID, uint32_t devAddr, uint8_t *nwkSKey, uint8_t *appSKey ) 00161 { 00162 MibRequestConfirm_t mibSet; 00163 00164 mibSet.Type = MIB_NET_ID ; 00165 mibSet.Param .NetID = netID; 00166 00167 LoRaMacMibSetRequestConfirm( &mibSet ); 00168 00169 mibSet.Type = MIB_DEV_ADDR ; 00170 mibSet.Param .DevAddr = devAddr; 00171 00172 LoRaMacMibSetRequestConfirm( &mibSet ); 00173 00174 mibSet.Type = MIB_NWK_SKEY ; 00175 mibSet.Param .NwkSKey = nwkSKey; 00176 00177 LoRaMacMibSetRequestConfirm( &mibSet ); 00178 00179 mibSet.Type = MIB_APP_SKEY ; 00180 mibSet.Param .AppSKey = appSKey; 00181 00182 LoRaMacMibSetRequestConfirm( &mibSet ); 00183 00184 mibSet.Type = MIB_NETWORK_JOINED ; 00185 mibSet.Param .IsNetworkJoined = true; 00186 00187 LoRaMacMibSetRequestConfirm( &mibSet ); 00188 } 00189 00190 void LoRaMacMulticastChannelAdd( MulticastParams_t *channelParam ) 00191 { 00192 LoRaMacMulticastChannelLink( channelParam ); 00193 } 00194 00195 void LoRaMacMulticastChannelRemove( MulticastParams_t *channelParam ) 00196 { 00197 LoRaMacMulticastChannelUnlink( channelParam ); 00198 } 00199 00200 uint8_t LoRaMacJoinReq( uint8_t *devEui, uint8_t *appEui, uint8_t *appKey ) 00201 { 00202 MlmeReq_t mlmeRequest; 00203 uint8_t status; 00204 00205 mlmeRequest.Type = MLME_JOIN ; 00206 mlmeRequest.Req.Join .AppEui = appEui; 00207 mlmeRequest.Req.Join .AppKey = appKey; 00208 mlmeRequest.Req.Join .DevEui = devEui; 00209 00210 switch( LoRaMacMlmeRequest( &mlmeRequest ) ) 00211 { 00212 case LORAMAC_STATUS_OK : 00213 { 00214 status = 0; 00215 break; 00216 } 00217 case LORAMAC_STATUS_BUSY : 00218 { 00219 status = 1; 00220 break; 00221 } 00222 case LORAMAC_STATUS_NO_NETWORK_JOINED : 00223 { 00224 status = 2; 00225 break; 00226 } 00227 case LORAMAC_STATUS_LENGTH_ERROR : 00228 case LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR : 00229 { 00230 status = 3; 00231 break; 00232 } 00233 case LORAMAC_STATUS_SERVICE_UNKNOWN : 00234 { 00235 status = 4; 00236 break; 00237 } 00238 case LORAMAC_STATUS_DEVICE_OFF : 00239 { 00240 status = 6; 00241 break; 00242 } 00243 default: 00244 { 00245 status = 1; 00246 break; 00247 } 00248 } 00249 00250 return status; 00251 } 00252 00253 uint8_t LoRaMacLinkCheckReq( void ) 00254 { 00255 MlmeReq_t mlmeRequest; 00256 uint8_t status; 00257 00258 mlmeRequest.Type = MLME_LINK_CHECK ; 00259 00260 switch( LoRaMacMlmeRequest( &mlmeRequest ) ) 00261 { 00262 case LORAMAC_STATUS_OK : 00263 { 00264 status = 0; 00265 break; 00266 } 00267 case LORAMAC_STATUS_SERVICE_UNKNOWN : 00268 { 00269 status = 1; 00270 break; 00271 } 00272 default: 00273 { 00274 status = 1; 00275 break; 00276 } 00277 } 00278 00279 return status; 00280 } 00281 00282 uint8_t LoRaMacSendFrame( uint8_t fPort, void *fBuffer, uint16_t fBufferSize ) 00283 { 00284 MibRequestConfirm_t mibGet; 00285 McpsReq_t mcpsRequest; 00286 uint8_t retStatus; 00287 00288 memset1( ( uint8_t* )&LoRaMacEventInfo, 0, sizeof( LoRaMacEventInfo ) ); 00289 00290 mibGet.Type = MIB_CHANNELS_DATARATE ; 00291 LoRaMacMibGetRequestConfirm( &mibGet ); 00292 00293 mcpsRequest.Type = MCPS_UNCONFIRMED ; 00294 mcpsRequest.Req.Unconfirmed .fBuffer = fBuffer; 00295 mcpsRequest.Req.Unconfirmed .fBufferSize = fBufferSize; 00296 mcpsRequest.Req.Unconfirmed .fPort = fPort; 00297 mcpsRequest.Req.Unconfirmed .Datarate = mibGet.Param .ChannelsDatarate ; 00298 00299 switch( LoRaMacMcpsRequest( &mcpsRequest ) ) 00300 { 00301 case LORAMAC_STATUS_OK : 00302 retStatus = 0U; 00303 break; 00304 case LORAMAC_STATUS_BUSY : 00305 retStatus = 1U; 00306 break; 00307 case LORAMAC_STATUS_NO_NETWORK_JOINED : 00308 retStatus = 2U; 00309 break; 00310 case LORAMAC_STATUS_LENGTH_ERROR : 00311 case LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR : 00312 retStatus = 3U; 00313 break; 00314 case LORAMAC_STATUS_SERVICE_UNKNOWN : 00315 retStatus = 4U; 00316 break; 00317 case LORAMAC_STATUS_DEVICE_OFF : 00318 retStatus = 6U; 00319 break; 00320 default: 00321 retStatus = 1U; 00322 break; 00323 } 00324 00325 return retStatus; 00326 } 00327 00328 uint8_t LoRaMacSendConfirmedFrame( uint8_t fPort, void *fBuffer, uint16_t fBufferSize, uint8_t nbRetries ) 00329 { 00330 MibRequestConfirm_t mibGet; 00331 McpsReq_t mcpsRequest; 00332 uint8_t retStatus; 00333 00334 memset1( ( uint8_t* )&LoRaMacEventInfo, 0, sizeof( LoRaMacEventInfo ) ); 00335 00336 mibGet.Type = MIB_CHANNELS_DATARATE ; 00337 LoRaMacMibGetRequestConfirm( &mibGet ); 00338 00339 mcpsRequest.Type = MCPS_CONFIRMED ; 00340 mcpsRequest.Req.Confirmed .fBuffer = fBuffer; 00341 mcpsRequest.Req.Confirmed .fBufferSize = fBufferSize; 00342 mcpsRequest.Req.Confirmed .fPort = fPort; 00343 mcpsRequest.Req.Confirmed .NbTrials = nbRetries; 00344 mcpsRequest.Req.Confirmed .Datarate = mibGet.Param .ChannelsDatarate ; 00345 00346 switch( LoRaMacMcpsRequest( &mcpsRequest ) ) 00347 { 00348 case LORAMAC_STATUS_OK : 00349 retStatus = 0U; 00350 break; 00351 case LORAMAC_STATUS_BUSY : 00352 retStatus = 1U; 00353 break; 00354 case LORAMAC_STATUS_NO_NETWORK_JOINED : 00355 retStatus = 2U; 00356 break; 00357 case LORAMAC_STATUS_LENGTH_ERROR : 00358 case LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR : 00359 retStatus = 3U; 00360 break; 00361 case LORAMAC_STATUS_SERVICE_UNKNOWN : 00362 retStatus = 4U; 00363 break; 00364 case LORAMAC_STATUS_DEVICE_OFF : 00365 retStatus = 6U; 00366 break; 00367 default: 00368 retStatus = 1U; 00369 break; 00370 } 00371 00372 return retStatus; 00373 } 00374 00375 uint8_t LoRaMacSend( LoRaMacHeader_t *macHdr, uint8_t *fOpts, uint8_t fPort, void *fBuffer, uint16_t fBufferSize ) 00376 { 00377 uint8_t retStatus; 00378 00379 memset1( ( uint8_t* ) &LoRaMacEventInfo, 0, sizeof( LoRaMacEventInfo ) ); 00380 00381 switch( Send( macHdr, fPort, fBuffer, fBufferSize ) ) 00382 { 00383 case LORAMAC_STATUS_OK : 00384 retStatus = 0U; 00385 break; 00386 case LORAMAC_STATUS_BUSY : 00387 retStatus = 1U; 00388 break; 00389 case LORAMAC_STATUS_NO_NETWORK_JOINED : 00390 retStatus = 2U; 00391 break; 00392 case LORAMAC_STATUS_LENGTH_ERROR : 00393 case LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR : 00394 retStatus = 3U; 00395 break; 00396 case LORAMAC_STATUS_SERVICE_UNKNOWN : 00397 retStatus = 4U; 00398 break; 00399 case LORAMAC_STATUS_DEVICE_OFF : 00400 retStatus = 6U; 00401 break; 00402 default: 00403 retStatus = 1U; 00404 break; 00405 } 00406 00407 return retStatus; 00408 } 00409 00410 uint8_t LoRaMacPrepareFrame( ChannelParams_t channel,LoRaMacHeader_t *macHdr, LoRaMacFrameCtrl_t *fCtrl, uint8_t *fOpts, uint8_t fPort, void *fBuffer, uint16_t fBufferSize ) 00411 { 00412 uint8_t retStatus; 00413 00414 switch( PrepareFrame( macHdr, fCtrl, fPort, fBuffer, fBufferSize ) ) 00415 { 00416 case LORAMAC_STATUS_OK : 00417 retStatus = 0U; 00418 break; 00419 case LORAMAC_STATUS_BUSY : 00420 retStatus = 1U; 00421 break; 00422 case LORAMAC_STATUS_NO_NETWORK_JOINED : 00423 retStatus = 2U; 00424 break; 00425 case LORAMAC_STATUS_LENGTH_ERROR : 00426 case LORAMAC_STATUS_MAC_CMD_LENGTH_ERROR : 00427 retStatus = 3U; 00428 break; 00429 case LORAMAC_STATUS_SERVICE_UNKNOWN : 00430 retStatus = 4U; 00431 break; 00432 default: 00433 retStatus = 1U; 00434 break; 00435 } 00436 00437 return retStatus; 00438 } 00439 00440 uint8_t LoRaMacSendFrameOnChannel( ChannelParams_t channel ) 00441 { 00442 memset1( ( uint8_t* ) &LoRaMacEventInfo, 0, sizeof( LoRaMacEventInfo ) ); 00443 00444 SendFrameOnChannel( channel ); 00445 00446 /* SendFrameOnChannel has always status "OK" */ 00447 return 0; 00448 } 00449 00450 uint8_t LoRaMacSendOnChannel( ChannelParams_t channel, LoRaMacHeader_t *macHdr, LoRaMacFrameCtrl_t *fCtrl, uint8_t *fOpts, uint8_t fPort, void *fBuffer, uint16_t fBufferSize ) 00451 { 00452 uint8_t status = 0; 00453 00454 if( ( LoRaMacState & 0x00000001 ) == 0x00000001 ) 00455 { 00456 return 1; // MAC is busy transmitting a previous frame 00457 } 00458 00459 status = LoRaMacPrepareFrame( channel, macHdr, fCtrl, fOpts, fPort, fBuffer, fBufferSize ); 00460 if( status != 0 ) 00461 { 00462 return status; 00463 } 00464 00465 LoRaMacEventInfo.TxNbRetries = 0; 00466 LoRaMacEventInfo.TxAckReceived = false; 00467 00468 return LoRaMacSendFrameOnChannel( channel ); 00469 } 00470 00471 void LoRaMacSetDeviceClass( DeviceClass_t deviceClass ) 00472 { 00473 MibRequestConfirm_t mibSet; 00474 00475 mibSet.Type = MIB_DEVICE_CLASS ; 00476 mibSet.Param .Class = deviceClass; 00477 00478 LoRaMacMibSetRequestConfirm( &mibSet ); 00479 } 00480 00481 void LoRaMacSetPublicNetwork( bool enable ) 00482 { 00483 MibRequestConfirm_t mibSet; 00484 00485 mibSet.Type = MIB_PUBLIC_NETWORK ; 00486 mibSet.Param .EnablePublicNetwork = enable; 00487 00488 LoRaMacMibSetRequestConfirm( &mibSet ); 00489 } 00490 00491 void LoRaMacSetDutyCycleOn( bool enable ) 00492 { 00493 LoRaMacTestSetDutyCycleOn ( enable ); 00494 } 00495 00496 void LoRaMacSetChannel( uint8_t id, ChannelParams_t params ) 00497 { 00498 LoRaMacChannelAdd( id, params ); 00499 } 00500 00501 void LoRaMacSetRx2Channel( Rx2ChannelParams_t param ) 00502 { 00503 MibRequestConfirm_t mibSet; 00504 00505 mibSet.Type = MIB_RX2_CHANNEL ; 00506 mibSet.Param .Rx2Channel = param; 00507 00508 LoRaMacMibSetRequestConfirm( &mibSet ); 00509 } 00510 00511 void LoRaMacSetChannelsMask( uint16_t *mask ) 00512 { 00513 MibRequestConfirm_t mibSet; 00514 00515 mibSet.Type = MIB_CHANNELS_MASK ; 00516 mibSet.Param .ChannelsMask = mask; 00517 00518 LoRaMacMibSetRequestConfirm( &mibSet ); 00519 } 00520 00521 void LoRaMacSetChannelsNbRep( uint8_t nbRep ) 00522 { 00523 MibRequestConfirm_t mibSet; 00524 00525 mibSet.Type = MIB_CHANNELS_NB_REP ; 00526 mibSet.Param .ChannelNbRep = nbRep; 00527 00528 LoRaMacMibSetRequestConfirm( &mibSet ); 00529 } 00530 00531 void LoRaMacSetMaxRxWindow( uint32_t delay ) 00532 { 00533 MibRequestConfirm_t mibSet; 00534 00535 mibSet.Type = MIB_MAX_RX_WINDOW_DURATION ; 00536 mibSet.Param .MaxRxWindow = delay; 00537 00538 LoRaMacMibSetRequestConfirm( &mibSet ); 00539 } 00540 00541 void LoRaMacSetReceiveDelay1( uint32_t delay ) 00542 { 00543 MibRequestConfirm_t mibSet; 00544 00545 mibSet.Type = MIB_RECEIVE_DELAY_1 ; 00546 mibSet.Param .ReceiveDelay1 = delay; 00547 00548 LoRaMacMibSetRequestConfirm( &mibSet ); 00549 } 00550 00551 void LoRaMacSetReceiveDelay2( uint32_t delay ) 00552 { 00553 MibRequestConfirm_t mibSet; 00554 00555 mibSet.Type = MIB_RECEIVE_DELAY_2 ; 00556 mibSet.Param .ReceiveDelay2 = delay; 00557 00558 LoRaMacMibSetRequestConfirm( &mibSet ); 00559 } 00560 00561 void LoRaMacSetJoinAcceptDelay1( uint32_t delay ) 00562 { 00563 MibRequestConfirm_t mibSet; 00564 00565 mibSet.Type = MIB_JOIN_ACCEPT_DELAY_1 ; 00566 mibSet.Param .JoinAcceptDelay1 = delay; 00567 00568 LoRaMacMibSetRequestConfirm( &mibSet ); 00569 } 00570 00571 void LoRaMacSetJoinAcceptDelay2( uint32_t delay ) 00572 { 00573 MibRequestConfirm_t mibSet; 00574 00575 mibSet.Type = MIB_JOIN_ACCEPT_DELAY_2 ; 00576 mibSet.Param .JoinAcceptDelay2 = delay; 00577 00578 LoRaMacMibSetRequestConfirm( &mibSet ); 00579 } 00580 00581 void LoRaMacSetChannelsDatarate( int8_t datarate ) 00582 { 00583 MibRequestConfirm_t mibSet; 00584 00585 mibSet.Type = MIB_CHANNELS_DATARATE ; 00586 mibSet.Param .ChannelsDatarate = datarate; 00587 00588 LoRaMacMibSetRequestConfirm( &mibSet ); 00589 } 00590 00591 void LoRaMacSetChannelsTxPower( int8_t txPower ) 00592 { 00593 MibRequestConfirm_t mibSet; 00594 00595 mibSet.Type = MIB_CHANNELS_TX_POWER ; 00596 mibSet.Param .ChannelsTxPower = txPower; 00597 00598 LoRaMacMibSetRequestConfirm( &mibSet ); 00599 } 00600 00601 uint32_t LoRaMacGetUpLinkCounter( void ) 00602 { 00603 MibRequestConfirm_t mibGet; 00604 00605 mibGet.Type = MIB_UPLINK_COUNTER ; 00606 00607 LoRaMacMibGetRequestConfirm( &mibGet ); 00608 00609 return mibGet.Param .UpLinkCounter ; 00610 } 00611 00612 uint32_t LoRaMacGetDownLinkCounter( void ) 00613 { 00614 MibRequestConfirm_t mibGet; 00615 00616 mibGet.Type = MIB_DOWNLINK_COUNTER ; 00617 00618 LoRaMacMibGetRequestConfirm( &mibGet ); 00619 00620 return mibGet.Param .DownLinkCounter ; 00621 } 00622 00623 void LoRaMacSetMicTest( uint16_t txPacketCounter ) 00624 { 00625 LoRaMacTestSetMic ( txPacketCounter ); 00626 }
Generated on Tue Jul 12 2022 14:27:10 by
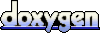