Specific Pelion ready example using features of Seeed Wio boards including cellular and SD Card
Dependencies: WS2812 PixelArray
Fork of simple-mbed-cloud-example-wio_3g by
main.cpp
00001 // ---------------------------------------------------------------------------- 00002 // Copyright 2016-2018 ARM Ltd. 00003 // 00004 // SPDX-License-Identifier: Apache-2.0 00005 // 00006 // Licensed under the Apache License, Version 2.0 (the "License"); 00007 // you may not use this file except in compliance with the License. 00008 // You may obtain a copy of the License at 00009 // 00010 // http://www.apache.org/licenses/LICENSE-2.0 00011 // 00012 // Unless required by applicable law or agreed to in writing, software 00013 // distributed under the License is distributed on an "AS IS" BASIS, 00014 // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 // See the License for the specific language governing permissions and 00016 // limitations under the License. 00017 // ---------------------------------------------------------------------------- 00018 #ifndef MBED_TEST_MODE 00019 00020 #include "mbed.h" 00021 #include "simple-mbed-cloud-client.h" 00022 #include "FATFileSystem.h" 00023 #include "LittleFileSystem.h" 00024 00025 #include "string.h" 00026 00027 // Default network interface object. Don't forget to change the WiFi SSID/password in mbed_app.json if you're using WiFi. 00028 NetworkInterface *net = NetworkInterface::get_default_instance(); 00029 00030 // Default block device available on the target board 00031 BlockDevice *bd = BlockDevice::get_default_instance(); 00032 00033 #if COMPONENT_SD || COMPONENT_NUSD 00034 // Use FATFileSystem for SD card type blockdevices 00035 FATFileSystem fs("fs"); 00036 #else 00037 // Use LittleFileSystem for non-SD block devices to enable wear leveling and other functions 00038 LittleFileSystem fs("fs"); 00039 #endif 00040 00041 #if USE_BUTTON == 1 00042 InterruptIn button(BUTTON1); 00043 #endif /* USE_BUTTON */ 00044 00045 DigitalOut SD_POWER(PA_15, 1); 00046 #include "Wio_LED.h" 00047 00048 // Declaring pointers for access to Pelion Device Management Client resources outside of main() 00049 MbedCloudClientResource *button_res; 00050 MbedCloudClientResource *led_res; 00051 MbedCloudClientResource *post_res; 00052 00053 // An event queue is a very useful structure to debounce information between contexts (e.g. ISR and normal threads) 00054 // This is great because things such as network operations are illegal in ISR, so updating a resource in a button's fall() function is not allowed 00055 EventQueue eventQueue; 00056 00057 /** 00058 * PUT handler - sets the value of the built-in LED 00059 * @param resource The resource that triggered the callback 00060 * @param newValue Updated value for the resource 00061 */ 00062 void put_callback(MbedCloudClientResource *resource, m2m::String newValue) { 00063 printf("PUT received. New value: %s\n", newValue.c_str()); 00064 if(atoi(newValue.c_str())) { 00065 setColor(WS2812_RED); 00066 } else { 00067 setColor(WS2812_BLACK); 00068 } 00069 } 00070 00071 /** 00072 * POST handler - prints the content of the payload 00073 * @param resource The resource that triggered the callback 00074 * @param buffer If a body was passed to the POST function, this contains the data. 00075 * Note that the buffer is deallocated after leaving this function, so copy it if you need it longer. 00076 * @param size Size of the body 00077 */ 00078 void post_callback(MbedCloudClientResource *resource, const uint8_t *buffer, uint16_t size) { 00079 printf("POST received (length %u). Payload: ", size); 00080 for (size_t ix = 0; ix < size; ix++) { 00081 printf("%02x ", buffer[ix]); 00082 } 00083 printf("\n"); 00084 } 00085 00086 /** 00087 * Button handler 00088 * This function will be triggered either by a physical button press or by a ticker every 5 seconds (see below) 00089 */ 00090 void button_press() { 00091 int v = button_res->get_value_int() + 1; 00092 button_res->set_value(v); 00093 printf("Button clicked %d times\n", v); 00094 } 00095 00096 /** 00097 * Notification callback handler 00098 * @param resource The resource that triggered the callback 00099 * @param status The delivery status of the notification 00100 */ 00101 void button_callback(MbedCloudClientResource *resource, const NoticationDeliveryStatus status) { 00102 printf("Button notification, status %s (%d)\n", MbedCloudClientResource::delivery_status_to_string(status), status); 00103 } 00104 00105 /** 00106 * Registration callback handler 00107 * @param endpoint Information about the registered endpoint such as the name (so you can find it back in portal) 00108 */ 00109 void registered(const ConnectorClientEndpointInfo *endpoint) { 00110 printf("Registered to Pelion Device Management. Endpoint Name: %s\n", endpoint->internal_endpoint_name.c_str()); 00111 } 00112 00113 int main(void) { 00114 printf("\nStarting Simple Pelion Device Management Client example\n"); 00115 00116 int storage_status = fs.mount(bd); 00117 if (storage_status != 0) { 00118 printf("Storage mounting failed.\n"); 00119 } 00120 00121 #if USE_BUTTON == 1 00122 // If the User button is pressed ons start, then format storage. 00123 bool btn_pressed = (button.read() == MBED_CONF_APP_BUTTON_PRESSED_STATE); 00124 if (btn_pressed) { 00125 printf("User button is pushed on start...\n"); 00126 } 00127 #else 00128 bool btn_pressed = false; 00129 #endif /* USE_BUTTON */ 00130 00131 if (storage_status || btn_pressed) { 00132 printf("Formatting the storage...\n"); 00133 int storage_status = StorageHelper::format(&fs, bd); 00134 if (storage_status != 0) { 00135 printf("ERROR: Failed to reformat the storage (%d).\n", storage_status); 00136 } 00137 } else { 00138 printf("You can hold the user button during boot to format the storage and change the device identity.\n"); 00139 } 00140 00141 // Connect to the Internet (DHCP is expected to be on) 00142 printf("Connecting to the network using the default network interface...\n"); 00143 net = NetworkInterface::get_default_instance(); 00144 00145 nsapi_error_t net_status = NSAPI_ERROR_NO_CONNECTION; 00146 while ((net_status = net->connect()) != NSAPI_ERROR_OK) { 00147 printf("Unable to connect to network (%d). Retrying...\n", net_status); 00148 } 00149 00150 printf("Connected to the network successfully. IP address: %s\n", net->get_ip_address()); 00151 00152 printf("Initializing Pelion Device Management Client...\n"); 00153 00154 // SimpleMbedCloudClient handles registering over LwM2M to Pelion Device Management 00155 SimpleMbedCloudClient client(net, bd, &fs); 00156 int client_status = client.init(); 00157 if (client_status != 0) { 00158 printf("Pelion Client initialization failed (%d)\n", client_status); 00159 return -1; 00160 } 00161 00162 // Creating resources, which can be written or read from the cloud 00163 button_res = client.create_resource("3200/0/5501", "button_count"); 00164 button_res->set_value(0); 00165 button_res->methods(M2MMethod::GET); 00166 button_res->observable(true); 00167 button_res->attach_notification_callback(button_callback); 00168 00169 led_res = client.create_resource("3201/0/5853", "led_state"); 00170 led_res->set_value(0); 00171 led_res->methods(M2MMethod::GET | M2MMethod::PUT); 00172 led_res->attach_put_callback(put_callback); 00173 00174 post_res = client.create_resource("3300/0/5605", "execute_function"); 00175 post_res->methods(M2MMethod::POST); 00176 post_res->attach_post_callback(post_callback); 00177 00178 printf("Initialized Pelion Device Management Client. Registering...\n"); 00179 00180 // Callback that fires when registering is complete 00181 client.on_registered(®istered); 00182 00183 // Register with Pelion DM 00184 client.register_and_connect(); 00185 00186 #if USE_BUTTON == 1 00187 // The button fires on an interrupt context, but debounces it to the eventqueue, so it's safe to do network operations 00188 button.fall(eventQueue.event(&button_press)); 00189 printf("Press the user button to increment the LwM2M resource value...\n"); 00190 #else 00191 // The timer fires on an interrupt context, but debounces it to the eventqueue, so it's safe to do network operations 00192 Ticker timer; 00193 timer.attach(eventQueue.event(&button_press), 5.0); 00194 printf("Simulating button press every 5 seconds...\n"); 00195 #endif /* USE_BUTTON */ 00196 00197 // You can easily run the eventQueue in a separate thread if required 00198 eventQueue.dispatch_forever(); 00199 } 00200 00201 #endif /* MBED_TEST_MODE */
Generated on Tue Jul 12 2022 19:09:28 by
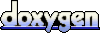