grove_accelerometer
Embed:
(wiki syntax)
Show/hide line numbers
grove_accelerometer.cpp
00001 00002 00003 #include "suli2.h" 00004 #include "grove_accelerometer.h" 00005 00006 00007 00008 //local functions 00009 static void grove_accelerometer_setmode(I2C_T *i2c, uint8_t mode); 00010 static void grove_accelerometer_setsamplerate(I2C_T *i2c, uint8_t rate); 00011 static void grove_accelerometer_getxyz(I2C_T *i2c, int8_t *x, int8_t *y, int8_t *z); 00012 00013 //local variables 00014 static unsigned char cmdbuf[2]; 00015 00016 00017 void grove_accelerometer_init(I2C_T *i2c, int pinsda, int pinscl) 00018 { 00019 suli_i2c_init(i2c, pinsda, pinscl); 00020 } 00021 00022 bool grove_accelerometer_write_setup(I2C_T *i2c) 00023 { 00024 grove_accelerometer_setmode(i2c, MMA7660_STAND_BY); 00025 grove_accelerometer_setsamplerate(i2c, AUTO_SLEEP_32); 00026 grove_accelerometer_setmode(i2c, MMA7660_ACTIVE); 00027 00028 return true; 00029 } 00030 00031 static void grove_accelerometer_setmode(I2C_T *i2c, uint8_t mode) 00032 { 00033 //write(MMA7660_MODE,mode); 00034 cmdbuf[0] = MMA7660_MODE; 00035 cmdbuf[1] = mode; 00036 suli_i2c_write(i2c, MMA7660_ADDR, cmdbuf, 2); 00037 00038 } 00039 static void grove_accelerometer_setsamplerate(I2C_T *i2c, uint8_t rate) 00040 { 00041 //write(MMA7660_SR,rate); 00042 cmdbuf[0] = MMA7660_SR; 00043 cmdbuf[1] = rate; 00044 suli_i2c_write(i2c, MMA7660_ADDR, cmdbuf, 2); 00045 } 00046 00047 /*Function: Get the contents of the registers in the MMA7660*/ 00048 /* so as to calculate the acceleration. */ 00049 static void grove_accelerometer_getxyz(I2C_T *i2c, int8_t *x, int8_t *y, int8_t *z) 00050 { 00051 unsigned char val[3]; 00052 val[0] = val[1] = val[2] = 64; 00053 00054 suli_i2c_read(i2c, MMA7660_ADDR, val, 3); 00055 00056 *x = ((char)(val[0]<<2))/4; 00057 *y = ((char)(val[1]<<2))/4; 00058 *z = ((char)(val[2]<<2))/4; 00059 } 00060 00061 bool grove_accelerometer_getacceleration(I2C_T *i2c, float *ax, float *ay, float *az) 00062 { 00063 int8_t x,y,z; 00064 grove_accelerometer_getxyz(i2c, &x,&y,&z); 00065 *ax = x/21.00; 00066 *ay = y/21.00; 00067 *az = z/21.00; 00068 00069 return true; 00070 } 00071 00072
Generated on Thu Aug 4 2022 04:31:52 by
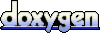