new Xadow GPS module
Dependents: xadow_smartstrap_for_pebble Avnet_ATT_Cellular_IOT Xadow-M0_Xadow-OLED_Accelerometer
XadowGPS.h
00001 /** 00002 * \file xadow_gps.h 00003 * \brief Module to handle GPS message interface of Xadow kit 00004 */ 00005 00006 #ifndef _XADOW_GPS_H_ 00007 #define _XADOW_GPS_H_ 00008 00009 #include "mbed.h" 00010 00011 /*! 00012 \def GPS_DEVICE_ADDR 00013 The I2C address GPS 00014 00015 \def GPS_SCAN_ID 00016 The id of scan data, the format is 0,0,0,Device address 00017 \def GPS_SCAN_SIZE 00018 The length of scan data 00019 00020 \def GPS_UTC_DATE_TIME_ID 00021 The id of utc date and time, the format is YMDHMS 00022 \def GPS_UTC_DATE_TIME_SIZE 00023 The length of utc date and time data 00024 00025 \def GPS_STATUS_ID 00026 The id of GPS status, the format is A/V 00027 \def GPS_STATUS_SIZE 00028 The length of GPS status data 00029 00030 \def GPS_LATITUDE_ID 00031 The id of latitude, the format is dd.dddddd 00032 \def GPS_LATITUDE_SIZE 00033 The length of latitude data 00034 00035 \def GPS_NS_ID 00036 The id of latitude direction, the format is N/S 00037 \def GPS_NS_SIZE 00038 The length of latitude direction data 00039 00040 \def GPS_LONGITUDE_ID 00041 The id of longitude, the format is ddd.dddddd 00042 \def GPS_LONGITUDE_SIZE 00043 The length of longitude data 00044 00045 \def GPS_EW_ID 00046 The id of longitude direction, the format is E/W 00047 \def GPS_EW_SIZE 00048 The length of longitude direction data 00049 00050 \def GPS_SPEED_ID 00051 The id of speed, the format is 000.0~999.9 Knots 00052 \def GPS_SPEED_SIZE 00053 The length of speed data 00054 00055 \def GPS_COURSE_ID 00056 The id of course, the format is 000.0~359.9 00057 \def GPS_COURSE_SIZE 00058 The length of course data 00059 00060 \def GPS_POSITION_FIX_ID 00061 The id of position fix status, the format is 0,1,2,6 00062 \def GPS_POSITION_FIX_SIZE 00063 The length of position fix status data 00064 00065 \def GPS_SATE_USED_ID 00066 The id of state used, the format is 00~12 00067 \def GPS_SATE_USED_SIZE 00068 The length of sate used data 00069 00070 \def GPS_ALTITUDE_ID 00071 The id of altitude, the format is -9999.9~99999.9 00072 \def GPS_ALTITUDE_SIZE 00073 The length of altitude data 00074 00075 \def GPS_MODE_ID 00076 The id of locate mode, the format is A/M 00077 \def GPS_MODE_SIZE 00078 The length of locate mode data 00079 00080 \def GPS_MODE2_ID 00081 The id of current status, the format is 1,2,3 00082 \def GPS_MODE2_SIZE 00083 The length of current status data 00084 */ 00085 00086 /* Data format: 00087 * Data_id(1 byte), Data_length(1 byte), Data 1, Data 2, ... Data n (n bytes, n = data length) 00088 * For example, get the scan data. 00089 * First, Send GPS_SCAN_ID(1 byte) to device. 00090 * Second, Receive scan data(Data_id + Data_length + GPS_SCAN_SIZE = 6 bytes). 00091 * Third, The scan data begin from the third data of received. 00092 * End 00093 */ 00094 00095 #define GPS_DEVICE_ADDR (0x05<<1) 00096 00097 #define GPS_SCAN_ID 0 // 4 bytes 00098 #define GPS_SCAN_SIZE 4 // 0,0,0,Device address 00099 00100 #define GPS_UTC_DATE_TIME_ID 1 // YMDHMS 00101 #define GPS_UTC_DATE_TIME_SIZE 6 // 6 bytes 00102 00103 #define GPS_STATUS_ID 2 // A/V 00104 #define GPS_STATUS_SIZE 1 // 1 byte 00105 00106 #define GPS_LATITUDE_ID 3 // dd.dddddd 00107 #define GPS_LATITUDE_SIZE 9 // 9 bytes 00108 00109 #define GPS_NS_ID 4 // N/S 00110 #define GPS_NS_SIZE 1 // 1 byte 00111 00112 #define GPS_LONGITUDE_ID 5 // ddd.dddddd 00113 #define GPS_LONGITUDE_SIZE 10 // 10 bytes 00114 00115 #define GPS_EW_ID 6 // E/W 00116 #define GPS_EW_SIZE 1 // 1 byte 00117 00118 #define GPS_SPEED_ID 7 // 000.0~999.9 Knots 00119 #define GPS_SPEED_SIZE 5 // 5 bytes 00120 00121 #define GPS_COURSE_ID 8 // 000.0~359.9 00122 #define GPS_COURSE_SIZE 5 // 5 bytes 00123 00124 #define GPS_POSITION_FIX_ID 9 // 0,1,2,6 00125 #define GPS_POSITION_FIX_SIZE 1 // 1 byte 00126 00127 #define GPS_SATE_USED_ID 10 // 00~12 00128 #define GPS_SATE_USED_SIZE 2 // 2 bytes 00129 00130 #define GPS_ALTITUDE_ID 11 // -9999.9~99999.9 00131 #define GPS_ALTITUDE_SIZE 7 // 7 bytes 00132 00133 #define GPS_MODE_ID 12 // A/M 00134 #define GPS_MODE_SIZE 1 // 1 byte 00135 00136 #define GPS_MODE2_ID 13 // 1,2,3 00137 #define GPS_MODE2_SIZE 1 // 1 byte 00138 00139 #define GPS_SATE_IN_VIEW_ID 14 // 0~12 00140 #define GPS_SATE_IN_VIEW_SIZE 1 // 1 byte 00141 00142 00143 /** 00144 * \brief Get the status of the device. 00145 * 00146 * \return Return TRUE or FALSE. TRUE is on line, FALSE is off line. 00147 * 00148 */ 00149 unsigned char gps_check_online(void); 00150 00151 /** 00152 * \brief Get the utc date and time. 00153 * 00154 * \return Return the pointer of utc date sand time, the format is YMDHMS. 00155 * 00156 */ 00157 unsigned char* gps_get_utc_date_time(void); 00158 00159 /** 00160 * \brief Get the status of GPS. 00161 * 00162 * \return Return A or V. A is orientation, V is navigation. 00163 * 00164 */ 00165 unsigned char gps_get_status(void); 00166 00167 /** 00168 * \brief Get the latitude. 00169 * 00170 * \return Return latitude data. The format is dd.dddddd. 00171 * 00172 */ 00173 float gps_get_latitude(void); 00174 00175 /** 00176 * \brief Get the latitude direction. 00177 * 00178 * \return Return latitude direction data. The format is N/S. N is north, S is south. 00179 * 00180 */ 00181 unsigned char gps_get_ns(void); 00182 00183 /** 00184 * \brief Get the longitude. 00185 * 00186 * \return Return longitude data. The format is ddd.dddddd. 00187 * 00188 */ 00189 float gps_get_longitude(void); 00190 00191 /** 00192 * \brief Get the longitude direction. 00193 * 00194 * \return Return longitude direction data. The format is E/W. E is east, W is west. 00195 * 00196 */ 00197 unsigned char gps_get_ew(void); 00198 00199 /** 00200 * \brief Get the speed. 00201 * 00202 * \return Return speed data. The format is 000.0~999.9 Knots. 00203 * 00204 */ 00205 float gps_get_speed(void); 00206 00207 /** 00208 * \brief Get the course. 00209 * 00210 * \return Return course data. The format is 000.0~359.9. 00211 * 00212 */ 00213 float gps_get_course(void); 00214 00215 /** 00216 * \brief Get the status of position fix. 00217 * 00218 * \return Return the status of position fix. The format is 0,1,2,6. 00219 * 0 - Invalid, 1 - GPS fix, 2 - DGPS fix, 6 - evaluation 00220 * 00221 */ 00222 unsigned char gps_get_position_fix(void); 00223 00224 /** 00225 * \brief Get the number of state used. 00226 * 00227 * \return Return number of state used. The format is 0-12. 00228 * 00229 */ 00230 unsigned char gps_get_sate_used(void); 00231 00232 /** 00233 * \brief Get the altitude. the format is -9999.9~99999.9 00234 * 00235 * \return Return altitude data. The format is -9999.9~99999.9. 00236 * 00237 */ 00238 float gps_get_altitude(void); 00239 00240 /** 00241 * \brief Get the mode of location. 00242 * 00243 * \return Return mode of location. The format is A/M. A:automatic, M:manual. 00244 * 00245 */ 00246 char gps_get_mode(void); 00247 00248 /** 00249 * \brief Get the current status of GPS. 00250 * 00251 * \return Return current status. The format is 1,2,3. 1:null, 2:2D, 3:3D. 00252 * 00253 */ 00254 unsigned char gps_get_mode2(void); 00255 00256 /** 00257 * \brief Get the number of sate in view. 00258 * 00259 * \return Return the number of sate in view. 00260 * 00261 */ 00262 unsigned char gps_get_sate_in_veiw(void); 00263 00264 00265 #endif
Generated on Tue Jul 19 2022 03:13:40 by
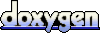