
this is a demo code to show how to use the GPRSInterface library
Dependencies: GPRSInterface mbed
Fork of Seeed_GPRS_Library_HelloWorld by
main.cpp
00001 /* 00002 main.cpp 00003 2013 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-02-18 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "GPRSInterface.h" 00024 #include "mbed.h" 00025 00026 #if defined(TARGET_LPC11U24)//SEEEDUINO_ARCH 00027 #define PIN_TX P1_22 00028 #define PIN_RX P1_21 00029 #elif defined(TARGET_LPC1768)//SEEEDUINO_ARCH_PRO 00030 #define PIN_TX P0_0 00031 #define PIN_RX P0_1 00032 #else //please redefine the following pins 00033 #define PIN_TX 00034 #define PIN_RX 00035 #endif 00036 00037 GPRSInterface gprsInterface(PIN_TX,PIN_RX,19200,"cmnet",NULL,NULL); 00038 00039 int main(void) 00040 { 00041 // use DHCP 00042 gprsInterface.init(); 00043 00044 // attempt DHCP 00045 while(false == gprsInterface.connect()) { 00046 wait(2); 00047 } 00048 00049 // successful DHCP 00050 printf("IP Address is %s\n", gprsInterface.getIPAddress()); 00051 00052 TCPSocketConnection sock; 00053 if(false == sock.connect("mbed.org", 80)) { 00054 return -1; 00055 } 00056 00057 char http_cmd[] = "GET /media/uploads/mbed_official/hello.txt HTTP/1.0\r\n\r\n"; 00058 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00059 00060 char buffer[512]; 00061 int ret; 00062 while (true) { 00063 ret = sock.receive(buffer, sizeof(buffer)-1); 00064 if (ret <= 0) 00065 break; 00066 buffer[ret] = '\0'; 00067 printf("Recv %d bytes:\n%s\n",ret,buffer); 00068 } 00069 sock.close(); 00070 gprsInterface.disconnect(); 00071 00072 return 0; 00073 }
Generated on Thu Jul 21 2022 03:26:57 by
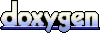