offer some APIs to control the movement of motor. Suitable with Seeed motor shield
Fork of MotorDriver by
MotorDriver.h
00001 /* 00002 MotorDriver.h 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet.zou@gmail.com 00006 2014-02-11 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 #ifndef __MOTORDRIVER_H__ 00023 #define __MOTORDRIVER_H__ 00024 00025 #include "mbed.h" 00026 #include "SoftwarePWM.h" 00027 00028 /**************Motor ID**********************/ 00029 #define MOTORA 0 00030 #define MOTORB 1 00031 00032 #define LOW 0 00033 #define HIGH 1 00034 00035 #define MOTOR_POSITION_LEFT 0 00036 #define MOTOR_POSITION_RIGHT 1 00037 #define MOTOR_CLOCKWISE 0 00038 #define MOTOR_ANTICLOCKWISE 1 00039 00040 #define USE_DC_MOTOR 0 00041 00042 #define MOTOR_PERIOD 10000 //10ms 00043 00044 struct MotorStruct { 00045 uint8_t speed; 00046 uint8_t direction; 00047 uint8_t position; 00048 }; 00049 00050 /** Motor Driver class. 00051 * offer API to control the movement of motor 00052 */ 00053 class MotorDriver 00054 { 00055 00056 public: 00057 00058 /** Create Motor Driver instance 00059 * @param int1 pin 1 of motor movement control 00060 * @param int2 pin 2 of motor movement control 00061 * @param int3 pin 3 of motor movement control 00062 * @param int4 pin 4 of motor movement control 00063 @param speedA speed control of motorA 00064 @param speedB speed control of motorB 00065 */ 00066 MotorDriver(PinName int1, PinName int2, PinName int3, PinName int4, PinName speedA, PinName speedB):_int1(int1),_int2(int2),_int3(int3),_int4(int4),_speedA(speedA),_speedB(speedB) { 00067 _int1 = 0; 00068 _int2 = 0; 00069 _int3 = 0; 00070 _int4 = 0; 00071 }; 00072 00073 /** set motor to initialized state 00074 */ 00075 void init(); 00076 00077 /** config position of motor 00078 * @param position the position set to motor,MOTOR_POSITION_LEFT or MOTOR_POSITION_RIGHT will be allowed 00079 * @param motorID the ID define which motor will be set, you can choose MOTORA or MOTORB 00080 */ 00081 void configure(uint8_t position, uint8_t motorID); 00082 00083 /** set speed of motor 00084 * @param speed speed value set to motor, [0,100] will be allowed 00085 * @param motorID the ID define which motor will be set, you can choose MOTORA or MOTORB 00086 */ 00087 void setSpeed(uint8_t speed, uint8_t motorID); 00088 00089 /** set direction of motor, 00090 * @param direction the direction of motor, MOTOR_CLOCKWISE or MOTOR_ANTICLOCKWISE will be allowed 00091 * @param motorID the ID define which motor will be set, you can choose MOTORA or MOTORB 00092 */ 00093 void setDirection(uint8_t direction, uint8_t motorID); 00094 00095 /** rotate motor 00096 * @param direction the direction set to motor, MOTOR_CLOCKWISE or MOTOR_ANTICLOCKWISE will be allowed 00097 * @param motor_position the position set to motor,MOTOR_POSITION_LEFT or MOTOR_POSITION_RIGHT will be allowed 00098 */ 00099 void rotate(uint8_t direction, uint8_t motor_position); 00100 00101 /** rotate motorA or motorB 00102 * @param direction the direction set to motor, MOTOR_CLOCKWISE or MOTOR_ANTICLOCKWISE will be allowed 00103 * @param motorID the ID define which motor will be set, you can choose MOTORA or MOTORB 00104 */ 00105 void rotateWithID(uint8_t direction, uint8_t motorID); 00106 00107 /** make motor go forward 00108 */ 00109 void goForward(); 00110 00111 /** make motor go backward 00112 */ 00113 void goBackward(); 00114 00115 /** make motor go left 00116 */ 00117 void goLeft(); 00118 00119 /** make motor go right 00120 */ 00121 void goRight(); 00122 00123 /** make motor stop 00124 */ 00125 void stop(); 00126 00127 /** make motorA or motorB stop 00128 * @param motorID the ID define which motor will be set, you can choose MOTORA or MOTORB 00129 */ 00130 void stop(uint8_t motorID); 00131 00132 /** motor A 00133 */ 00134 MotorStruct motorA; 00135 00136 /** motor B 00137 */ 00138 MotorStruct motorB; 00139 00140 private: 00141 00142 DigitalOut _int1; 00143 DigitalOut _int2; 00144 DigitalOut _int3; 00145 DigitalOut _int4; 00146 SoftwarePWM _speedA; 00147 SoftwarePWM _speedB; 00148 }; 00149 #endif
Generated on Fri Jul 15 2022 20:45:17 by
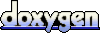