
MaKey MaKey mbed version, http://makeymakey.com/
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBKeyboard.h" 00003 00004 #define LOG(args...) // printf(args) 00005 00006 #define THRESHOLD 2 00007 #define TOUCH_N 6 00008 00009 BusOut leds(LED1, LED2, LED3, LED4); 00010 Ticker tick; 00011 USBKeyboard keyboard; 00012 00013 uint8_t key_map[TOUCH_N] = {RIGHT_ARROW, LEFT_ARROW, DOWN_ARROW, UP_ARROW, ' ', '\n'}; 00014 PinName touch_pin[TOUCH_N] = {A0, A1, A2, A3, A4, A5}; 00015 DigitalInOut *p_touch_io[TOUCH_N]; 00016 00017 uint8_t touch_data[TOUCH_N] = {0, }; 00018 00019 void detect(void) 00020 { 00021 for (int i = 0; i < TOUCH_N; i++) { 00022 uint8_t count = 0; 00023 DigitalInOut *touch_io = p_touch_io[i]; 00024 00025 touch_io->input(); 00026 touch_data[i] <<= 1; 00027 while (touch_io->read()) { 00028 count++; 00029 if (count > THRESHOLD) { 00030 touch_data[i] |= 0x01; 00031 break; 00032 } 00033 } 00034 touch_io->output(); 00035 touch_io->write(1); 00036 00037 if (0x01 == touch_data[i]) { // a measurement is about the threshold, get a touch 00038 leds = 1 << i; 00039 keyboard.putc(key_map[i]); 00040 LOG("No %d key is touched\r\n", i); 00041 } else if (0x80 == touch_data[i]) { // last 7 measurement is under the threshold, touch is released 00042 leds = 0x00; 00043 LOG("No %d key is released\r\n", i); 00044 } 00045 } 00046 } 00047 00048 int main() 00049 { 00050 // setup 00051 for (int i = 0; i < TOUCH_N; i++) { 00052 p_touch_io[i] = new DigitalInOut(touch_pin[i]); 00053 p_touch_io[i]->mode(PullDown); 00054 p_touch_io[i]->output(); 00055 p_touch_io[i]->write(1); 00056 } 00057 00058 tick.attach(detect, 1.0 / 64.0); 00059 00060 while(1) { 00061 // do something 00062 wait(1); 00063 } 00064 }
Generated on Sun Jul 24 2022 07:05:13 by
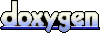