MAX31855 library
Embed:
(wiki syntax)
Show/hide line numbers
MAX31855.cpp
00001 /* 00002 * A library for MAX31855 00003 * 00004 * Copyright (c) 2015 Seeed Technology Limited. 00005 * Author : Yihui Xiong 00006 * 00007 * The MIT License (MIT) 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining a copy 00010 * of this software and associated documentation files (the "Software"), to deal 00011 * in the Software without restriction, including without limitation the rights 00012 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 * copies of the Software, and to permit persons to whom the Software is 00014 * furnished to do so, subject to the following conditions: 00015 * 00016 * The above copyright notice and this permission notice shall be included in 00017 * all copies or substantial portions of the Software. 00018 * 00019 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 * THE SOFTWARE. 00026 */ 00027 00028 00029 #include "max31855.h" 00030 00031 #define LOG(args...) printf(args) 00032 00033 MAX31855::MAX31855(SPI& _spi, PinName _ncs) : spi(_spi), ncs(_ncs) 00034 { 00035 thermocoupleT = 0.0 / 0.0; 00036 internalT = 0.0 / 0.0; 00037 lastReadTime = 0; 00038 } 00039 00040 float MAX31855::read(temperature_type_t type) { 00041 uint8_t buf[4]; 00042 00043 uint32_t time = us_ticker_read(); 00044 uint32_t duration = time - lastReadTime; 00045 if (duration > 250000) { // more than 250ms 00046 //Set CS low to start transmission (interrupts conversion) 00047 ncs = 0; 00048 00049 //Read in Probe tempeature 00050 for (int i = 0; i < 4; i++) { 00051 buf[i] = spi.write(0); 00052 } 00053 00054 //Set CS high to stop transmission (restarts conversion) 00055 ncs = 1; 00056 00057 if (buf[1] & 1) { 00058 uint8_t fault = buf[3] & 0x7; 00059 if (fault & 0x1) { 00060 LOG("The thermocouple is open (no connections).\r\n"); 00061 } 00062 if (fault & 0x2) { 00063 LOG("The thermocouple is short-circuited to GND.\r\n"); 00064 } 00065 if (fault & 0x4) { 00066 LOG("The thermocouple is short-circuited to VCC.\r\n"); 00067 } 00068 return 0.0 / 0.0; 00069 } 00070 00071 int t1 = ((buf[0] & 0x7F) << 6) + (buf[1] >> 2); 00072 if (buf[0] & 0x80) { // sign bit 00073 t1 = -t1; 00074 } 00075 thermocoupleT = t1 * 0.25; 00076 00077 int t2 = ((buf[2] & 0x7F) << 4) + (buf[3] >> 4); 00078 if (buf[2] & 0x80) { 00079 t2 = -t2; 00080 } 00081 internalT = t2 * 0.0625; 00082 } 00083 00084 if (THERMOCOUPLE_T == type) { 00085 return thermocoupleT; 00086 } else { 00087 return internalT; 00088 } 00089 } 00090 00091
Generated on Fri Jul 22 2022 09:22:24 by
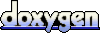