3-Axis Digital Accelerometer is the key part in projects like orientation detection, gesture detection and Motion detection. This 3-Asix Digital Accelerometer(±1.5g) is based on Freescale's low power consumption module, MMA7660FC. It features up to 10,000g high shock surviability and configurable Samples per Second rate. For generous applications that don't require too large measurement range, this is a great choice because it's durable, energy saving and cost-efficient.
Fork of Grove_3-Axis_Digital_Accelerometer_MMA7660FC_Library by
MMA7660.h
00001 /* 00002 * MMA7760.h 00003 * Library for accelerometer_MMA7760 00004 * 00005 * Copyright (c) 2013 seeed technology inc. 00006 * Author : FrankieChu 00007 * Create Time : Jan 2013 00008 * Change Log : 00009 * 00010 * The MIT License (MIT) 00011 * 00012 * Permission is hereby granted, free of charge, to any person obtaining a copy 00013 * of this software and associated documentation files (the "Software"), to deal 00014 * in the Software without restriction, including without limitation the rights 00015 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00016 * copies of the Software, and to permit persons to whom the Software is 00017 * furnished to do so, subject to the following conditions: 00018 * 00019 * The above copyright notice and this permission notice shall be included in 00020 * all copies or substantial portions of the Software. 00021 * 00022 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00023 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00024 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00025 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00026 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00027 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00028 * THE SOFTWARE. 00029 */ 00030 #include "mbed.h" 00031 00032 #ifndef __MMC7660_H__ 00033 #define __MMC7660_H__ 00034 00035 #define MMA7660_ADDR 0x4c 00036 00037 #define MMA7660_X 0x00 00038 #define MMA7660_Y 0x01 00039 #define MMA7660_Z 0x02 00040 #define MMA7660_TILT 0x03 00041 #define MMA7660_SRST 0x04 00042 #define MMA7660_SPCNT 0x05 00043 #define MMA7660_INTSU 0x06 00044 #define MMA7660_MODE 0x07 00045 #define MMA7660_STAND_BY 0x00 00046 #define MMA7660_ACTIVE 0x01 00047 #define MMA7660_SR 0x08 //sample rate register 00048 #define AUTO_SLEEP_120 0X00//120 sample per second 00049 #define AUTO_SLEEP_64 0X01 00050 #define AUTO_SLEEP_32 0X02 00051 #define AUTO_SLEEP_16 0X03 00052 #define AUTO_SLEEP_8 0X04 00053 #define AUTO_SLEEP_4 0X05 00054 #define AUTO_SLEEP_2 0X06 00055 #define AUTO_SLEEP_1 0X07 00056 #define MMA7660_PDET 0x09 00057 #define MMA7660_PD 0x0A 00058 class MMA7660 00059 { 00060 private: 00061 void write(uint8_t _register, uint8_t _data); 00062 uint8_t read(uint8_t _register); 00063 public: 00064 void init(); 00065 void setMode(uint8_t mode); 00066 void setSampleRate(uint8_t rate); 00067 void getXYZ(int8_t *x,int8_t *y,int8_t *z); 00068 void getSignedXYZ(int8_t *x,int8_t *y,int8_t *z); 00069 void getAcceleration(float *ax,float *ay,float *az); 00070 }; 00071 00072 #endif //__MMC7660_H__
Generated on Sat Jul 16 2022 10:18:49 by
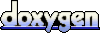