
Example application for the STMicroelectronics X-NUCLEO-NFC05A1
Dependencies: RFAL ST25R3911 BSP05
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * File Name : main.c 00004 * Description : Main program body 00005 ****************************************************************************** 00006 * 00007 * COPYRIGHT(c) 2017 STMicroelectronics 00008 * 00009 * Redistribution and use in source and binary forms, with or without modification, 00010 * are permitted provided that the following conditions are met: 00011 * 1. Redistributions of source code must retain the above copyright notice, 00012 * this list of conditions and the following disclaimer. 00013 * 2. Redistributions in binary form must reproduce the above copyright notice, 00014 * this list of conditions and the following disclaimer in the documentation 00015 * and/or other materials provided with the distribution. 00016 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00017 * may be used to endorse or promote products derived from this software 00018 * without specific prior written permission. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00021 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00022 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00023 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00024 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00025 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00026 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00027 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00028 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00029 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00030 * 00031 ****************************************************************************** 00032 */ 00033 00034 /*! \file 00035 * 00036 * \author 00037 * 00038 * \brief Main program body. 00039 * 00040 */ 00041 00042 00043 00044 /* USER CODE BEGIN Includes */ 00045 #include "mbed.h" 00046 #include "logger.h" 00047 #include "st_errno.h" 00048 #include "rfal_rf.h" 00049 #include "rfal_AnalogConfig.h" 00050 #include "ST25R3911.h" 00051 #include "x_nucleo_nfc05.h" 00052 #include "x_nucleo_nfc05a1.h" 00053 #include "st25r3911_interrupt.h" 00054 00055 #include <stdint.h> 00056 00057 00058 /* USER CODE END Includes */ 00059 00060 00061 00062 #ifndef MYBOOLEAN_H 00063 #define MYBOOLEAN_H 00064 00065 #define false 0 00066 #define true 1 00067 #define bool int 00068 00069 #endif 00070 00071 00072 00073 00074 SPI_HandleTypeDef hspi1; 00075 SPI_InitTypeDef Init; 00076 ST25R3911 mST25; 00077 00078 SPI mspiChannel(SPI_MOSI, SPI_MISO, SPI_SCK); 00079 DigitalOut gpio_cs(SPI_CS); 00080 00081 DigitalIn uButton(BUTTON1); 00082 DigitalOut fieldLED_01(A1); //LED_F_PIN 00083 DigitalOut fieldLED_02(A2); //LED_B_PIN 00084 DigitalOut fieldLED_03(A3); //LED_A_PIN 00085 DigitalOut fieldLED_04(D5); //LED_V_PIN 00086 DigitalOut fieldLED_05(D4); //LED_AP2P_PIN 00087 DigitalOut fieldLED_06(D7); //LED_FIELD_PIN 00088 00089 X_Nucleo_NFC05 mNFC05(&mspiChannel, &fieldLED_01); 00090 00091 00092 InterruptIn IRQ(SYS_WKUP); 00093 00094 volatile bool wakeupFlag = 0; 00095 00096 void st25Handler( void ) 00097 { 00098 00099 //st25r3911Isr( &mspiChannel, &mST25, &gpio_cs, &IRQ ); 00100 } 00101 00102 00103 00104 int main(void) 00105 { 00106 00107 /* Setting format and frequency for SPI communication */ 00108 gpio_cs = 1; 00109 mspiChannel.format(8, 1); 00110 mspiChannel.frequency(4000000); 00111 00112 /* Set IRQ handler */ 00113 IRQ.rise(&st25Handler); 00114 00115 /* Set default Config for rfal */ 00116 rfalAnalogConfigInitialize(); 00117 00118 if( rfalInitialize(&mspiChannel, &mST25, &gpio_cs, &IRQ, &fieldLED_01, &fieldLED_02, &fieldLED_03, &fieldLED_04, &fieldLED_05, &fieldLED_06 ) != ERR_NONE ) 00119 { 00120 00121 /* If here, there is an error in rfal Initialization */ 00122 while(1) 00123 { 00124 /* Blink the LED to communicate an error */ 00125 fieldLED_01.write(1); 00126 fieldLED_02.write(1); 00127 fieldLED_03.write(1); 00128 fieldLED_04.write(1); 00129 fieldLED_05.write(1); 00130 fieldLED_06.write(1); 00131 wait_ms(200); 00132 fieldLED_01.write(0); 00133 fieldLED_02.write(0); 00134 fieldLED_03.write(0); 00135 fieldLED_04.write(0); 00136 fieldLED_05.write(0); 00137 fieldLED_06.write(0); 00138 wait_ms(300); 00139 } 00140 } 00141 else 00142 { 00143 /* Initialization succeeded */ 00144 for(int i = 0; i < 6; i++) 00145 { 00146 fieldLED_01.write(1); 00147 fieldLED_02.write(1); 00148 fieldLED_03.write(1); 00149 fieldLED_04.write(1); 00150 fieldLED_05.write(1); 00151 fieldLED_06.write(1); 00152 wait_ms(200); 00153 fieldLED_01.write(0); 00154 fieldLED_02.write(0); 00155 fieldLED_03.write(0); 00156 fieldLED_04.write(0); 00157 fieldLED_05.write(0); 00158 fieldLED_06.write(0); 00159 wait_ms(300); 00160 00161 } 00162 00163 } 00164 00165 00166 while(1) 00167 { 00168 00169 00170 /* Run RFAL Worker */ 00171 rfalWorker(&mspiChannel, &mST25, &gpio_cs, &IRQ, &fieldLED_01, &fieldLED_02, &fieldLED_03, &fieldLED_04, &fieldLED_05, &fieldLED_06 ); 00172 00173 /* Run Demo Application */ 00174 demoCycle(&mspiChannel, &mST25, &uButton, &gpio_cs, &IRQ, &fieldLED_01, &fieldLED_02, &fieldLED_03, &fieldLED_04, &fieldLED_05, &fieldLED_06 ); 00175 } 00176 00177 } 00178 00179 /** 00180 * @} 00181 */ 00182 00183 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jul 21 2022 02:04:36 by
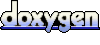