
M2X with Seeed Ethernet using Seeed Accelerometer demo
Dependencies: LM75B M2XStreamClient jsonlite mbed-rtos mbed
Fork of m2x-seeed_ethernet_demo by
main.cpp
00001 #include <jsonlite.h> 00002 #include "M2XStreamClient.h" 00003 00004 #include "mbed.h" 00005 #include "WIZnetInterface.h" 00006 #include "LM75B.h" //I2C Temperature Sensor 00007 00008 #define ST_NUCLEO 00009 char feedId[] = "fe08906d21a70b05241234077386e041"; // Feed you want to post to 00010 char m2xKey[] = "ca9c1e4db697886906de09c701879b19"; // Your M2X access key 00011 char streamName[] = "temperature"; // Stream you want to post to 00012 00013 char name[] = "Dallas"; // Name of current location of datasource 00014 double latitude = 33.007872; 00015 double longitude = -96.751614; // You can also read those values from a GPS 00016 double elevation = 697.00; 00017 00018 PwmOut mypwm(PWM_OUT); 00019 AnalogIn temp_sensor(A0); 00020 00021 /** 00022 * Configure the SPI interfac to the ethernet module 00023 * D11 - MOSI pin 00024 * D12 - MISO pin 00025 * D13 - SCK pin 00026 * D10 - SEL pin 00027 * NC - Reset pin; use D5 otherwise the shield might get into reset loop 00028 */ 00029 00030 SPI spi(PA_7, PA_6, PA_5); // mosi, miso, sclk 00031 WIZnetInterface eth(&spi, PB_6, PA_10); // spi, cs, reset 00032 00033 /* Instantiate the M2X Stream Client */ 00034 Client client; 00035 M2XStreamClient m2xClient(&client, m2xKey); 00036 00037 00038 /* Call back function for reading back data point data from M2X */ 00039 void on_data_point_found(const char* at, const char* value, int index, void* context, int type) 00040 { 00041 printf("Found a data point, index: %d\r\n", index); 00042 printf("At: %s Value: %s\r\n", at, value); 00043 } 00044 00045 /* Call back function for reading back location data from M2X */ 00046 void on_location_found(const char* name, 00047 double latitude, 00048 double longitude, 00049 double elevation, 00050 const char* timestamp, 00051 int index, 00052 void* context) 00053 { 00054 printf("Found a location, index: %d\r\n", index); 00055 printf("Name: %s Latitude: %lf Longitude: %lf\r\n", name, latitude, longitude); 00056 printf("Elevation: %lf Timestamp: %s\r\n", elevation, timestamp); 00057 } 00058 00059 int main() 00060 { 00061 uint8_t mac[6]; 00062 int adc_scale = 65536; //4096; 00063 int B = 3975; 00064 float resistance; 00065 float temperature; 00066 float temperature_f; 00067 char amb_temp[6]; 00068 00069 mypwm.period_ms(500); 00070 mypwm.pulsewidth_ms(250); 00071 /* Have mbed assign the mac address */ 00072 mbed_mac_address((char *)mac); 00073 00074 printf("Start...\n"); 00075 wait_ms(3000); 00076 00077 /* Initialize ethernet interface */ 00078 int ret = eth.init(mac); //Use DHCP 00079 00080 00081 if (!ret) { 00082 printf("Initialized, MAC: %s\n", eth.getMACAddress()); 00083 } else { 00084 printf("Error eth.init() - ret = %d\n", ret); 00085 return -1; 00086 } 00087 00088 00089 /* Get IP address */ 00090 while (1) { 00091 printf(">>> Get IP address...\n"); 00092 ret = eth.connect(); // Connect to network 00093 00094 printf("ret: %d\n",ret); 00095 if (ret < 0) { 00096 printf(">>> Could not connect to network! Retrying ...\n"); 00097 wait_ms(3000); 00098 printf("past this point...\n"); 00099 } else { 00100 break; 00101 } 00102 } 00103 00104 if (!ret) { 00105 printf("IP: %s, MASK: %s, GW: %s\n", 00106 eth.getIPAddress(), eth.getNetworkMask(), eth.getGateway()); 00107 } else { 00108 printf("Error eth.connect() - ret = %d\n", ret); 00109 return -1; 00110 } 00111 00112 00113 00114 mypwm.pulsewidth_ms(500); 00115 00116 /* Main loop */ 00117 while (true) { 00118 00119 00120 00121 /* Wait 5 secs and then loop */ 00122 delay(2500); 00123 mypwm.pulsewidth_ms(0); 00124 00125 /* Read ADC value from analog sensor */ 00126 uint16_t a = temp_sensor.read_u16(); 00127 00128 /* Calculate the temperature in Fareheight and Celsius */ 00129 resistance = (float)(adc_scale-a)*10000/a; //get the resistance of the sensor; 00130 temperature = 1/(log(resistance/10000)/B+1/298.15)-273.15; //convert to temperature via datasheet ; 00131 temperature_f =(1.8 * temperature) + 32.0; 00132 sprintf(amb_temp, "%0.2f", temperature_f); 00133 00134 printf("Temp Sensor Analog Reading is 0x%X = %d \r\n", a, a); 00135 printf("Current Temperature: %f C %f F \r\n", temperature, temperature_f); 00136 00137 delay(2500); 00138 mypwm.pulsewidth_ms(500); 00139 00140 /* Post temperature to M2X site */ 00141 int response = m2xClient.put(feedId, streamName, amb_temp); 00142 printf("Post response code: %d\r\n", response); 00143 if (response == -1) 00144 { 00145 mypwm.pulsewidth_ms(250); 00146 printf("Temperature data transmit post error\n"); 00147 } 00148 /* Update location data */ 00149 response = m2xClient.updateLocation(feedId, name, latitude, longitude, elevation); 00150 printf("updateLocation response code: %d\r\n", response); 00151 if (response == -1) 00152 { 00153 mypwm.pulsewidth_ms(250); 00154 printf("Location data transmit post error\n"); 00155 } 00156 00157 printf("\r\n"); 00158 00159 00160 } 00161 }
Generated on Sun Jul 17 2022 01:08:28 by
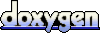