NFC NDEF library
Dependents: Nucleo_NFC_Example I2C_NFC_Master Print_Entire_Nucleo_NFC01A1_Memory
Fork of lib_NDEF by
lib_NDEF_Vcard.c
00001 /** 00002 ****************************************************************************** 00003 * @file lib_NDEF_Vcard.c 00004 * @author MMY Application Team 00005 * @version V1.0.0 00006 * @date 20-November-2013 00007 * @brief This file help to manage NDEF file that represent Vcard. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT 2014 STMicroelectronics</center></h2> 00012 * 00013 * Licensed under MMY-ST Liberty SW License Agreement V2, (the "License"); 00014 * You may not use this file except in compliance with the License. 00015 * You may obtain a copy of the License at: 00016 * 00017 * http://www.st.com/software_license_agreement_liberty_v2 00018 * 00019 * Unless required by applicable law or agreed to in writing, software 00020 * distributed under the License is distributed on an "AS IS" BASIS, 00021 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00022 * See the License for the specific language governing permissions and 00023 * limitations under the License. 00024 * 00025 ****************************************************************************** 00026 */ 00027 00028 /* Includes ------------------------------------------------------------------*/ 00029 #include "lib_NDEF_Vcard.h" 00030 00031 /** @addtogroup NFC_libraries 00032 * @{ 00033 * @brief <b>This is the library used to manage the content of the TAG (data) 00034 * But also the specific feature of the tag, for instance 00035 * password, gpo... </b> 00036 */ 00037 00038 00039 /** @addtogroup libNFC_FORUM 00040 * @{ 00041 * @brief This part of the library manage data which follow NFC forum organisation. 00042 */ 00043 00044 /** 00045 * @brief This buffer contains the data send/received by TAG 00046 */ 00047 extern uint8_t NDEF_Buffer [NDEF_MAX_SIZE]; 00048 00049 /** @defgroup libVcard_Private_Functions 00050 * @{ 00051 */ 00052 00053 00054 static void NDEF_FillVcardStruct( uint8_t* pPayload, uint32_t PayloadSize, char* pKeyWord, uint32_t SizeOfKeyWord, uint8_t* pString); 00055 static void NDEF_ExtractVcard ( sRecordInfo *pRecordStruct, sVcardInfo *pVcardStruct ); 00056 00057 /** 00058 * @brief This fonction fill Vcard structure with information of NDEF message 00059 * @param pPayload : pointer on the payload data of the NDEF message 00060 * @param PayloadSize : number of data in the payload 00061 * @param pKeyWord : pointer on the keyword to look for. 00062 * @param SizeOfKeyWord : number of byte of the keyword we are looking for 00063 * @param pString : Pointer on the data string to fill 00064 * @retval NONE 00065 */ 00066 static void NDEF_FillVcardStruct( uint8_t* pPayload, uint32_t PayloadSize, char* pKeyWord, uint32_t SizeOfKeyWord, uint8_t* pString) 00067 { 00068 uint8_t* pLastByteAdd, *pLook4Word, *pEndString ; 00069 00070 /* First charactere force to NULL in case not matching found */ 00071 *pString = 0; 00072 00073 /* Interresting information are stored before picture if any */ 00074 /* Moreover picture is not used in this demonstration SW */ 00075 pLastByteAdd = pPayload; 00076 while( memcmp( pLastByteAdd, JPEG, JPEG_STRING_SIZE) && pLastByteAdd<(pPayload+PayloadSize) ) 00077 { 00078 pLastByteAdd++; 00079 } 00080 00081 pLook4Word = pPayload; 00082 while( memcmp( pLook4Word, pKeyWord, SizeOfKeyWord) && pLook4Word<pLastByteAdd ) 00083 { 00084 pLook4Word++; 00085 } 00086 00087 /* Word found */ 00088 if( pLook4Word != pLastByteAdd) 00089 { 00090 pLook4Word += SizeOfKeyWord; 00091 pEndString = pLook4Word; 00092 while( memcmp( pEndString, LIMIT, LIMIT_STRING_SIZE) && pEndString<pLastByteAdd ) 00093 { 00094 pEndString++; 00095 } 00096 if( pEndString != pLastByteAdd) 00097 { 00098 memcpy( pString, pLook4Word, pEndString-pLook4Word); 00099 /* add end of string charactere */ 00100 pString += pEndString-pLook4Word; 00101 *pString = '\0'; 00102 } 00103 } 00104 } 00105 00106 /** 00107 * @brief This fonction read the Vcard and store data in a structure 00108 * @param pRecordStruct : Pointer on the record structure 00109 * @param pSMSStruct : pointer on the structure to fill 00110 * @retval NONE 00111 */ 00112 static void NDEF_ExtractVcard ( sRecordInfo *pRecordStruct, sVcardInfo *pVcardStruct ) 00113 { 00114 uint32_t PayloadSize; 00115 uint8_t* pPayload; 00116 00117 00118 PayloadSize = ((uint32_t)(pRecordStruct->PayloadLength3)<<24) | ((uint32_t)(pRecordStruct->PayloadLength2)<<16) | 00119 ((uint32_t)(pRecordStruct->PayloadLength1)<<8) | pRecordStruct->PayloadLength0; 00120 00121 /* Read record header */ 00122 pPayload = (uint8_t*)(pRecordStruct->PayloadBufferAdd); 00123 00124 NDEF_FillVcardStruct(pPayload , PayloadSize,VERSION, VERSION_STRING_SIZE, (uint8_t*)(pVcardStruct->Version)); 00125 if( !memcmp(pVcardStruct->Version, VCARD_VERSION_2_1, VCARD_VERSION_2_1_SIZE)) 00126 { 00127 NDEF_FillVcardStruct(pPayload , PayloadSize,FIRSTNAME, FIRSTNAME_STRING_SIZE, (uint8_t*)(pVcardStruct->FirstName)); 00128 NDEF_FillVcardStruct(pPayload , PayloadSize,TITLE, TITLE_STRING_SIZE, (uint8_t*)(pVcardStruct->Title)); 00129 NDEF_FillVcardStruct(pPayload , PayloadSize,ORG, ORG_STRING_SIZE, (uint8_t*)(pVcardStruct->Org)); 00130 NDEF_FillVcardStruct(pPayload , PayloadSize,HOME_ADDRESS, HOME_ADDRESS_STRING_SIZE, (uint8_t*)(pVcardStruct->HomeAddress)); 00131 NDEF_FillVcardStruct(pPayload , PayloadSize,WORK_ADDRESS, WORK_ADDRESS_STRING_SIZE, (uint8_t*)(pVcardStruct->WorkAddress)); 00132 NDEF_FillVcardStruct(pPayload , PayloadSize,HOME_TEL, HOME_TEL_STRING_SIZE, (uint8_t*)(pVcardStruct->HomeTel)); 00133 NDEF_FillVcardStruct(pPayload , PayloadSize,WORK_TEL, WORK_TEL_STRING_SIZE, (uint8_t*)(pVcardStruct->WorkTel)); 00134 NDEF_FillVcardStruct(pPayload , PayloadSize,CELL_TEL, CELL_TEL_STRING_SIZE, (uint8_t*)(pVcardStruct->CellTel)); 00135 NDEF_FillVcardStruct(pPayload , PayloadSize,HOME_EMAIL, HOME_EMAIL_STRING_SIZE, (uint8_t*)(pVcardStruct->HomeEmail)); 00136 NDEF_FillVcardStruct(pPayload , PayloadSize,WORK_EMAIL, WORK_EMAIL_STRING_SIZE, (uint8_t*)(pVcardStruct->WorkEmail)); 00137 } 00138 else if( !memcmp(pVcardStruct->Version, VCARD_VERSION_3_0, VCARD_VERSION_3_0_SIZE)) 00139 { 00140 /* need to be implemented */ 00141 } 00142 else 00143 { 00144 /* maybe new version but not supported in this sw */ 00145 } 00146 00147 } 00148 00149 /** 00150 * @} 00151 */ 00152 00153 /** @defgroup libVcard_Public_Functions 00154 * @{ 00155 * @brief This file is used to manage Vcard (stored or loaded in tag) 00156 */ 00157 00158 /** 00159 * @brief This fonction read NDEF and retrieve Vcard information if any 00160 * @param pRecordStruct : Pointer on the record structure 00161 * @param pVcardStruct : pointer on the structure to fill 00162 * @retval SUCCESS : Vcard information from NDEF have been retrieved 00163 * @retval ERROR : Not able to retrieve Vcard information 00164 */ 00165 uint16_t NDEF_ReadVcard ( sRecordInfo *pRecordStruct, sVcardInfo *pVcardStruct ) 00166 { 00167 uint16_t status = ERROR; 00168 00169 if( pRecordStruct->NDEF_Type == VCARD_TYPE) 00170 { 00171 NDEF_ExtractVcard(pRecordStruct, pVcardStruct ); 00172 status = SUCCESS; 00173 } 00174 00175 return status; 00176 } 00177 00178 /** 00179 * @brief This fonction write the NDEF file with the Vcard data given in the structure 00180 * @param pVcardStruct : pointer on structure that contain the Vcard information 00181 * @retval SUCCESS : the function is succesful 00182 * @retval ERROR : Not able to store NDEF file inside tag. 00183 */ 00184 uint16_t NDEF_WriteVcard ( sVcardInfo *pVcardStruct ) 00185 { 00186 uint16_t status = ERROR; 00187 uint16_t DataSize; 00188 uint32_t PayloadSize = 0; 00189 00190 /* Vcard Record Header */ 00191 /************************************/ 00192 /* 7 | 6 | 5 | 4 | 3 | 2 1 0 */ 00193 /*----------------------------------*/ 00194 /* MB ME CF SR IL TNF */ /* <---- CF=0, IL=0 and SR=0 TNF=2 NFC Forum Media type*/ 00195 /*----------------------------------*/ 00196 /* TYPE LENGTH */ 00197 /*----------------------------------*/ 00198 /* PAYLOAD LENGTH 3 */ 00199 /*----------------------------------*/ 00200 /* PAYLOAD LENGTH 2 */ 00201 /*----------------------------------*/ 00202 /* PAYLOAD LENGTH 1 */ 00203 /*----------------------------------*/ 00204 /* PAYLOAD LENGTH 0 */ 00205 /*----------------------------------*/ 00206 /* ID LENGTH */ /* <---- Not Used */ 00207 /*----------------------------------*/ 00208 /* TYPE */ 00209 /*----------------------------------*/ 00210 /* ID */ /* <---- Not Used */ 00211 /************************************/ 00212 00213 /* As we don't have embedded a jpeg encoder/decoder in this firmware */ 00214 /* We have made the choice to manage only string content of the vCard */ 00215 /* For demonstration purpose in order to fill the 8kB of the M24SR */ 00216 /* We have embedded a NDEF vCard in the STM32 to be able to fill M24SR */ 00217 00218 00219 /* NDEF file must be written in 2 phases, first phase NDEF size is Null */ 00220 NDEF_Buffer[NDEF_SIZE_OFFSET] = 0x00; 00221 NDEF_Buffer[NDEF_SIZE_OFFSET+1] = 0x00; 00222 00223 /* fill record header */ 00224 NDEF_Buffer[FIRST_RECORD_OFFSET] = 0xC2; /* Record Flag */ 00225 NDEF_Buffer[FIRST_RECORD_OFFSET+1] = VCARD_TYPE_STRING_LENGTH; 00226 NDEF_Buffer[FIRST_RECORD_OFFSET+2] = 0x00; /* Will be filled at the end when payload size is known */ 00227 NDEF_Buffer[FIRST_RECORD_OFFSET+3] = 0x00; 00228 NDEF_Buffer[FIRST_RECORD_OFFSET+4] = 0x00; 00229 NDEF_Buffer[FIRST_RECORD_OFFSET+5] = 0x00; 00230 memcpy(&NDEF_Buffer[FIRST_RECORD_OFFSET+6], VCARD_TYPE_STRING, VCARD_TYPE_STRING_LENGTH); 00231 00232 /* Payload is positionned in the NDEF after record header */ 00233 PayloadSize = FIRST_RECORD_OFFSET+6+VCARD_TYPE_STRING_LENGTH; 00234 00235 /* "BEGIN:VCARD\r\n" */ 00236 memcpy(&NDEF_Buffer[PayloadSize], BEGIN, BEGIN_STRING_SIZE); 00237 PayloadSize += BEGIN_STRING_SIZE; 00238 memcpy( &NDEF_Buffer[PayloadSize], VCARD,VCARD_STRING_SIZE); 00239 PayloadSize += VCARD_STRING_SIZE; 00240 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00241 PayloadSize += LIMIT_STRING_SIZE; 00242 00243 /* "VERSION:2.1\r\n" */ 00244 memcpy(&NDEF_Buffer[PayloadSize], VERSION, VERSION_STRING_SIZE); 00245 PayloadSize += VERSION_STRING_SIZE; 00246 memcpy( &NDEF_Buffer[PayloadSize], VCARD_VERSION_2_1,VCARD_VERSION_2_1_SIZE); 00247 PayloadSize += VCARD_VERSION_2_1_SIZE; 00248 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00249 PayloadSize += LIMIT_STRING_SIZE; 00250 00251 /* "FN:\r\n" */ 00252 memcpy(&NDEF_Buffer[PayloadSize], FIRSTNAME, FIRSTNAME_STRING_SIZE); 00253 PayloadSize += FIRSTNAME_STRING_SIZE; 00254 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->FirstName,strlen(pVcardStruct->FirstName)); 00255 PayloadSize += strlen(pVcardStruct->FirstName); 00256 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00257 PayloadSize += LIMIT_STRING_SIZE; 00258 00259 /* "TITLE:\r\n" */ 00260 memcpy(&NDEF_Buffer[PayloadSize], TITLE,TITLE_STRING_SIZE); 00261 PayloadSize += TITLE_STRING_SIZE; 00262 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->Title,strlen(pVcardStruct->Title)); 00263 PayloadSize += strlen(pVcardStruct->Title); 00264 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00265 PayloadSize += LIMIT_STRING_SIZE; 00266 00267 /* "ORG:\r\n" */ 00268 memcpy(&NDEF_Buffer[PayloadSize], ORG,ORG_STRING_SIZE); 00269 PayloadSize += ORG_STRING_SIZE; 00270 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->Org,strlen(pVcardStruct->Org)); 00271 PayloadSize += strlen(pVcardStruct->Org); 00272 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00273 PayloadSize += LIMIT_STRING_SIZE; 00274 00275 /* "ADR;HOME:\r\n" */ 00276 memcpy(&NDEF_Buffer[PayloadSize], HOME_ADDRESS,HOME_ADDRESS_STRING_SIZE); 00277 PayloadSize += HOME_ADDRESS_STRING_SIZE; 00278 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->HomeAddress,strlen(pVcardStruct->HomeAddress)); 00279 PayloadSize += strlen(pVcardStruct->HomeAddress); 00280 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00281 PayloadSize += LIMIT_STRING_SIZE; 00282 00283 /* "ADR;WORK:\r\n" */ 00284 memcpy(&NDEF_Buffer[PayloadSize], WORK_ADDRESS,WORK_ADDRESS_STRING_SIZE); 00285 PayloadSize += WORK_ADDRESS_STRING_SIZE; 00286 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->WorkAddress,strlen(pVcardStruct->WorkAddress)); 00287 PayloadSize += strlen(pVcardStruct->WorkAddress); 00288 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00289 PayloadSize += LIMIT_STRING_SIZE; 00290 00291 /* "TEL;HOME:\r\n" */ 00292 memcpy(&NDEF_Buffer[PayloadSize], HOME_TEL, HOME_TEL_STRING_SIZE); 00293 PayloadSize += HOME_TEL_STRING_SIZE; 00294 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->HomeTel,strlen(pVcardStruct->HomeTel)); 00295 PayloadSize += strlen(pVcardStruct->HomeTel); 00296 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00297 PayloadSize += LIMIT_STRING_SIZE; 00298 00299 /* "TEL;WORK:\r\n" */ 00300 memcpy(&NDEF_Buffer[PayloadSize], WORK_TEL, WORK_TEL_STRING_SIZE); 00301 PayloadSize += WORK_TEL_STRING_SIZE; 00302 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->WorkTel,strlen(pVcardStruct->WorkTel)); 00303 PayloadSize += strlen(pVcardStruct->WorkTel); 00304 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00305 PayloadSize += LIMIT_STRING_SIZE; 00306 00307 /* "TEL;CELL:\r\n" */ 00308 memcpy(&NDEF_Buffer[PayloadSize], CELL_TEL, CELL_TEL_STRING_SIZE); 00309 PayloadSize += CELL_TEL_STRING_SIZE; 00310 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->CellTel,strlen(pVcardStruct->CellTel)); 00311 PayloadSize += strlen(pVcardStruct->CellTel); 00312 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00313 PayloadSize += LIMIT_STRING_SIZE; 00314 00315 /* "EMAIL;HOME:\r\n" */ 00316 memcpy(&NDEF_Buffer[PayloadSize], HOME_EMAIL, HOME_EMAIL_STRING_SIZE); 00317 PayloadSize += HOME_EMAIL_STRING_SIZE; 00318 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->HomeEmail,strlen(pVcardStruct->HomeEmail)); 00319 PayloadSize += strlen(pVcardStruct->HomeEmail); 00320 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00321 PayloadSize += LIMIT_STRING_SIZE; 00322 00323 /* "EMAIL;WORK:\r\n" */ 00324 memcpy(&NDEF_Buffer[PayloadSize], WORK_EMAIL, WORK_EMAIL_STRING_SIZE); 00325 PayloadSize += WORK_EMAIL_STRING_SIZE; 00326 memcpy( &NDEF_Buffer[PayloadSize], pVcardStruct->WorkEmail,strlen(pVcardStruct->WorkEmail)); 00327 PayloadSize += strlen(pVcardStruct->WorkEmail); 00328 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00329 PayloadSize += LIMIT_STRING_SIZE; 00330 00331 /* "END:VCARD\r\n" */ 00332 memcpy(&NDEF_Buffer[PayloadSize], END,END_STRING_SIZE); 00333 PayloadSize += END_STRING_SIZE; 00334 memcpy( &NDEF_Buffer[PayloadSize], VCARD,VCARD_STRING_SIZE); 00335 PayloadSize += VCARD_STRING_SIZE; 00336 memcpy( &NDEF_Buffer[PayloadSize], LIMIT, LIMIT_STRING_SIZE); 00337 PayloadSize += LIMIT_STRING_SIZE; 00338 00339 DataSize = (uint16_t)(PayloadSize); /* Must not count the 2 byte that represent the NDEF size */ 00340 PayloadSize -= FIRST_RECORD_OFFSET+6+VCARD_TYPE_STRING_LENGTH; 00341 00342 NDEF_Buffer[FIRST_RECORD_OFFSET+2] = (PayloadSize & 0xFF000000)>>24; 00343 NDEF_Buffer[FIRST_RECORD_OFFSET+3] = (PayloadSize & 0x00FF0000)>>16; 00344 NDEF_Buffer[FIRST_RECORD_OFFSET+4] = (PayloadSize & 0x0000FF00)>>8; 00345 NDEF_Buffer[FIRST_RECORD_OFFSET+5] = (PayloadSize & 0x000000FF); 00346 00347 00348 /* Write NDEF */ 00349 status = WriteData ( 0x00 , DataSize , NDEF_Buffer); 00350 00351 /* Write NDEF size to complete*/ 00352 if( status == NDEF_ACTION_COMPLETED) 00353 { 00354 DataSize -= 2; /* Must not count the 2 byte that represent the NDEF size */ 00355 NDEF_Buffer[0] = (DataSize & 0xFF00)>>8; 00356 NDEF_Buffer[1] = (DataSize & 0x00FF); 00357 00358 status = WriteData ( 0x00 , 2 , NDEF_Buffer); 00359 } 00360 00361 00362 if( status == NDEF_ACTION_COMPLETED) 00363 return SUCCESS; 00364 else 00365 return ERROR; 00366 } 00367 00368 00369 /** 00370 * @} 00371 */ 00372 00373 /** 00374 * @} 00375 */ 00376 00377 /** 00378 * @} 00379 */ 00380 00381 /******************* (C) COPYRIGHT 2013 STMicroelectronics *****END OF FILE****/ 00382 00383 00384
Generated on Tue Jul 12 2022 14:05:02 by
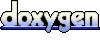