Library for supporting the Nucleo Sensor Shield.
Dependents: Nucleo_Sensors_Demo m2x-temp_ethernet_demo m2x-MEMS_ACKme_Wifi_demo m2x_MEMS_Ublox_Cellular_demo ... more
Fork of Nucleo_Sensor_Shield by
lis3mdl.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file x_cube_mems_lis3mdl.h 00004 * @author AST / EST 00005 * @version V0.0.1 00006 * @date 9-December-2014 00007 * @brief Implementation file for component LIS3MDL 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "mbed.h" 00040 #include "lis3mdl.h" 00041 #include "lis3mdl_platform.h" 00042 #include <math.h> 00043 00044 /* Methods -------------------------------------------------------------------*/ 00045 00046 /** 00047 * @brief Read data from LIS3MDL Magnetic sensor and calculate Magnetic in mgauss. 00048 * @param float *pfData 00049 * @retval None. 00050 */ 00051 void LIS3MDL::GetAxes(AxesRaw_TypeDef *pData) 00052 { 00053 uint8_t tempReg = 0x00; 00054 int16_t pDataRaw[3]; 00055 float sensitivity = 0; 00056 int ret; 00057 00058 GetAxesRaw(pDataRaw); 00059 00060 ret = dev_i2c.i2c_read(&tempReg, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG2_M, 1); 00061 00062 if (ret == 0) 00063 { 00064 tempReg &= LIS3MDL_M_FS_MASK; 00065 00066 switch(tempReg) 00067 { 00068 case LIS3MDL_M_FS_4: 00069 sensitivity = 0.14; 00070 break; 00071 case LIS3MDL_M_FS_8: 00072 sensitivity = 0.29; 00073 break; 00074 case LIS3MDL_M_FS_12: 00075 sensitivity = 0.43; 00076 break; 00077 case LIS3MDL_M_FS_16: 00078 sensitivity = 0.58; 00079 break; 00080 } 00081 } 00082 00083 pData->AXIS_X = (int32_t)(pDataRaw[0] * sensitivity); 00084 pData->AXIS_Y = (int32_t)(pDataRaw[1] * sensitivity); 00085 pData->AXIS_Z = (int32_t)(pDataRaw[2] * sensitivity); 00086 00087 } 00088 00089 /** 00090 * @brief Read raw data from LIS3MDL Magnetic sensor output register. 00091 * @param float *pfData 00092 * @retval None. 00093 */ 00094 void LIS3MDL::GetAxesRaw(int16_t *pData) 00095 { 00096 uint8_t tempReg[2] = {0,0}; 00097 int ret; 00098 00099 pData[0] = pData[1] = pData[2] = 0; 00100 00101 ret = dev_i2c.i2c_read(&tempReg[0], LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_OUT_X_L_M + 0x80, 2); 00102 00103 if (ret == 0) 00104 { 00105 pData[0] = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00106 ret = dev_i2c.i2c_read(&tempReg[0], LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_OUT_Y_L_M + 0x80, 2); 00107 } 00108 00109 if (ret == 0) 00110 { 00111 pData[1] = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00112 ret = dev_i2c.i2c_read(&tempReg[0], LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_OUT_Z_L_M + 0x80, 2); 00113 } 00114 00115 if (ret == 0) 00116 { 00117 pData[2] = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00118 } 00119 } 00120 00121 /** 00122 * @brief Read ID address of HTS221 00123 * @param Device ID address 00124 * @retval ID name 00125 */ 00126 uint8_t LIS3MDL::ReadID(void) 00127 { 00128 uint8_t tmp=0x00; 00129 int ret; 00130 00131 /* Read WHO I AM register */ 00132 ret = dev_i2c.i2c_read(&tmp, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_WHO_AM_I_ADDR, 1); 00133 00134 /* Return the ID */ 00135 return ((ret == 0) ? (uint8_t)tmp : 0); 00136 } 00137 00138 /** 00139 * @brief Set HTS221 Initialization. 00140 * @param InitStruct: it contains the configuration setting for the HTS221. 00141 * @retval None 00142 */ 00143 void LIS3MDL::Init() { 00144 00145 uint8_t tmp1 = 0x00; 00146 int ret; 00147 00148 /****** Magnetic sensor *******/ 00149 00150 ret = dev_i2c.i2c_read(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG3_M, 1); 00151 00152 /* Conversion mode selection */ 00153 if (ret == 0) 00154 { 00155 tmp1 &= ~(LIS3MDL_M_MD_MASK); 00156 tmp1 |= LIS3MDL_M_MD_CONTINUOUS; 00157 ret = dev_i2c.i2c_write(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG3_M, 1); 00158 } 00159 00160 if (ret == 0) 00161 { 00162 ret = dev_i2c.i2c_read(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG1_M, 1); 00163 } 00164 00165 if (ret == 0) 00166 { 00167 /* Output data rate selection */ 00168 tmp1 &= ~(LIS3MDL_M_DO_MASK); 00169 tmp1 |= LIS3MDL_M_DO_80; 00170 00171 /* X and Y axes Operative mode selection */ 00172 tmp1 &= ~(LIS3MDL_M_OM_MASK); 00173 tmp1 |= LIS3MDL_M_OM_HP; 00174 00175 ret = dev_i2c.i2c_write(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG1_M, 1); 00176 } 00177 00178 if (ret == 0) 00179 { 00180 ret = dev_i2c.i2c_read(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG2_M, 1); 00181 } 00182 00183 if (ret == 0) 00184 { 00185 /* Full scale selection */ 00186 tmp1 &= ~(LIS3MDL_M_FS_MASK); 00187 tmp1 |= LIS3MDL_M_FS_4; 00188 00189 ret = dev_i2c.i2c_write(&tmp1, LIS3MDL_M_MEMS_ADDRESS, LIS3MDL_M_CTRL_REG2_M, 1); 00190 } 00191 00192 /******************************/ 00193 00194 if (ret == 0) 00195 { 00196 if(ReadID() == I_AM_LIS3MDL_M) 00197 { 00198 LIS3MDLInitialized = 1; 00199 } 00200 } 00201 00202 return; 00203 }
Generated on Tue Jul 12 2022 14:47:53 by
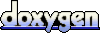