NUCLEO-NFC01A1 board drivers.
Dependents: Nucleo_NFC_Example I2C_NFC_Master Print_Entire_Nucleo_NFC01A1_Memory
Fork of X-NUCLEO-NFC01A1 by
drv_I2C_M24SR.h
00001 /** 00002 ****************************************************************************** 00003 * @file drv_I2C_M24SR.h 00004 * @author MMY Application Team 00005 * @version V1.1.0 00006 * @date 20-October-2014 00007 * @brief This file provides a set of functions needed to manage the I2C of 00008 the M24SR device. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT 2014 STMicroelectronics</center></h2> 00013 * 00014 * Licensed under MMY-ST Liberty SW License Agreement V2, (the "License"); 00015 * You may not use this file except in compliance with the License. 00016 * You may obtain a copy of the License at: 00017 * 00018 * http://www.st.com/software_license_agreement_liberty_v2 00019 * 00020 * Unless required by applicable law or agreed to in writing, software 00021 * distributed under the License is distributed on an "AS IS" BASIS, 00022 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00023 * See the License for the specific language governing permissions and 00024 * limitations under the License. 00025 * 00026 ****************************************************************************** 00027 */ 00028 00029 /* Define to prevent recursive inclusion -------------------------------------*/ 00030 #ifndef __DRV_I2CM24SR_H 00031 #define __DRV_I2CM24SR_H 00032 00033 00034 #ifdef __MBED__ /* UNDEFINED types with mbed */ 00035 #ifdef TARGET_NUCLEO_F411RE 00036 #include "stm32f4xx.h" 00037 #elif TARGET_NUCLEO_F401RE 00038 #include "stm32f4xx.h" 00039 #elif defined TARGET_NUCLEO_L053R8 00040 #include "stm32l0xx.h" 00041 #elif defined TARGET_NUCLEO_F030R8 00042 #include "stm32F0xx.h" 00043 #elif defined TARGET_NUCLEO_F302R8 00044 #include "stm32F3xx.h" 00045 #elif defined TARGET_NUCLEO_L152RE 00046 #include "stm32l1xx.h" 00047 #else 00048 #error "You need to update your code to this new microcontroller" 00049 #endif 00050 #else 00051 #ifdef STM32F401xE 00052 #include "stm32f4xx_hal.h" 00053 #elif defined STM32F030x8 00054 #include "stm32f0xx_hal.h" 00055 #elif defined STM32F302x8 00056 #include "stm32f3xx_hal.h" 00057 #elif defined STM32L053xx 00058 #include "stm32l0xx_hal.h" 00059 #elif defined STM32L152xE 00060 #include "stm32l1xx_hal.h" 00061 #else 00062 #error "You need to update your code to this new microcontroller" 00063 #endif 00064 #endif 00065 00066 00067 #ifdef __cplusplus 00068 extern "C" { 00069 #endif 00070 00071 /* Includes ------------------------------------------------------------------*/ 00072 #include "string.h" 00073 #include "stdio.h" 00074 #include "stdbool.h" 00075 00076 /* Flags ---------------------------------------------------------------------*/ 00077 /* If both of this two flags are disabled, then the I2C polling will be used */ 00078 //#define I2C_GPO_SYNCHRO_ALLOWED /* allow tu use GPO polling as I2C synchronization */ 00079 //#define I2C_GPO_INTERRUPT_ALLOWED /* allow tu use GPO interrupt as I2C synchronization ! NOT SUPPORTED BY MBED ! */ 00080 00081 #define EXTERNAL_PULLUP /* If SCL is already pulled up using an external resistor */ 00082 00083 #define M24SR_POLL_DELAY 50 /* Delay (in ms) before starting to poll the device for command completion */ 00084 00085 /* macro function ------------------------------------------------------------*/ 00086 00087 #ifndef errchk 00088 #define errchk(fCall) if (status = (fCall), status != M24SR_STATUS_SUCCESS) \ 00089 {goto Error;} else 00090 #endif 00091 00092 /*!< constant Unsigned integer types */ 00093 typedef const unsigned char uc8; 00094 typedef const unsigned short uc16; 00095 //typedef const unsigned long uc32; 00096 00097 #define M24SR_I2C_TIMEOUT 200 /* I2C Time out (ms), this is the maximum time needed by M24SR to complete any command */ 00098 #define M24SR_I2C_POLLING 1 /* In case M24SR will reply ACK failed allow to perform retry before returning error (HAL option not used) */ 00099 #define M24SR_ADDR 0xAC /*!< M24SR address */ 00100 00101 00102 /* error code ---------------------------------------------------------------------------------*/ 00103 #define M24SR_ERRORCODE_FILEOVERFLOW 0x6280 00104 #define M24SR_ERRORCODE_ENDOFFILE 0x6282 00105 #define M24SR_ERRORCODE_PASSWORDREQUIRED 0x63C0 00106 #define M24SR_ERRORCODE_PASSWORDINCORRECT2RETRY 0x63C2 00107 #define M24SR_ERRORCODE_PASSWORDINCORRECT1RETRY 0x63C1 00108 #define M24SR_ERRORCODE_RFSESSIONKILLED 0x6500 00109 #define M24SR_ERRORCODE_UNSUCCESSFULUPDATING 0x6581 00110 #define M24SR_ERRORCODE_WRONGHLENGTH 0x6700 00111 #define M24SR_ERRORCODE_COMMANDINCORRECT 0x6981 00112 #define M24SR_ERRORCODE_SECURITYSTATUS 0x6982 00113 #define M24SR_ERRORCODE_REFERENCEDATANOTUSABLE 0x6984 00114 #define M24SR_ERRORCODE_INCORRECTPARAMETER 0x6A80 00115 #define M24SR_ERRORCODE_FILENOTFOUND 0x6A82 00116 #define M24SR_ERRORCODE_FILEOVERFLOWLC 0x6A84 00117 #define M24SR_ERRORCODE_INCORRECTP1P2 0x6A86 00118 #define M24SR_ERRORCODE_INSNOTSUPPORTED 0x6D00 00119 #define M24SR_ERRORCODE_CLASSNOTSUPPORTED 0x6E00 00120 #define M24SR_ERRORCODE_DAFAULT 0x6F00 00121 00122 /* Status and error code -----------------------------------------------------*/ 00123 #define M24SR_STATUS_SUCCESS 0x0000 00124 #define M24SR_ERROR_DEFAULT 0x0010 00125 #define M24SR_ERROR_I2CTIMEOUT 0x0011 00126 #define M24SR_ERROR_CRC 0x0012 00127 #define M24SR_ERROR_NACK 0x0013 00128 #define M24SR_ERROR_PARAMETER 0x0014 00129 #define M24SR_ERROR_NBATEMPT 0x0015 00130 #define M24SR_ERROR_NOACKNOWLEDGE 0x0016 00131 00132 /* mask ------------------------------------------------------------------------------------*/ 00133 typedef enum{ 00134 M24SR_WAITINGTIME_UNKNOWN= 0, 00135 M24SR_WAITINGTIME_POLLING, 00136 M24SR_WAITINGTIME_TIMEOUT, 00137 M24SR_WAITINGTIME_GPO, 00138 M24SR_INTERRUPT_GPO 00139 }M24SR_WAITINGTIME_MGMT; 00140 00141 /* public function --------------------------------------------------------------------------*/ 00142 00143 void M24SR_I2CInit ( void ); 00144 void M24SR_GPOInit ( void ); 00145 void M24SR_WaitMs ( uint32_t time_ms ); 00146 void M24SR_GetTick ( uint32_t *ptickstart ); 00147 void M24SR_GPO_ReadPin ( GPIO_PinState *pPinState); 00148 void M24SR_RFDIS_WritePin ( GPIO_PinState PinState); 00149 void M24SR_SetI2CSynchroMode ( uint8_t mode ); 00150 int8_t M24SR_SendI2Ccommand ( uint8_t NbByte , uint8_t *pBuffer ); 00151 int8_t M24SR_IsAnswerReady ( void ); 00152 int8_t M24SR_PollI2C ( void ); 00153 int8_t M24SR_ReceiveI2Cresponse ( uint8_t NbByte , uint8_t *pBuffer ); 00154 void M24SR_RFConfig_Hard ( uint8_t OnOffChoice); 00155 00156 #endif 00157 00158 #ifdef __cplusplus 00159 } 00160 #endif 00161 00162 /******************* (C) COPYRIGHT 2013 STMicroelectronics *****END OF FILE****/
Generated on Sat Jul 16 2022 11:22:31 by
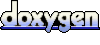