got mp3
Fork of VS1053 by
Embed:
(wiki syntax)
Show/hide line numbers
player.cpp
00001 #include "player.h" 00002 #include"SDFileSystem.h" 00003 00004 SDFileSystem sd(D11, D12, D13, D9, "sd"); // the pinout on the mbed Cool 00005 vs10xx vs1053(D11, D12, D13, D6, D7, D2, D8);//mosi,miso,sclk,xcs,xdcs,dreq,xreset 3spi+chip select 00006 Serial aa(USBTX, USBRX); 00007 Serial pc(D1, D0); 00008 playerStatetype playerState; 00009 static unsigned char fileBuf[48000]; 00010 unsigned char *bufptr; 00011 char green; 00012 bool startplaysong; 00013 bool playsong; 00014 char datainput; 00015 00016 char list[20][50]; //song list 00017 00018 char data ; 00019 DigitalIn sw_in(USER_BUTTON); 00020 00021 void Player::begin(void) 00022 { 00023 DirHandle *dir; 00024 struct dirent *ptr; 00025 FileHandle *fp; 00026 00027 vs1053.reset(); 00028 dir = opendir("/sd"); 00029 //printf("\r\n**********playing list**********\r\n"); 00030 unsigned char i = 0,j=0; 00031 while(((ptr = dir->readdir()) != NULL)&&(i <20)) 00032 { 00033 if(strstr(ptr->d_name,".mp3")||strstr(ptr->d_name,".MP3")) 00034 { 00035 fp =sd.open(ptr->d_name, O_RDONLY); 00036 if(fp != NULL) 00037 { 00038 char *byte = ptr->d_name; 00039 j=0; 00040 while(*byte){ 00041 list[i][j++] = *byte++; 00042 green=i; 00043 } 00044 aa.printf("%2d . %s\r\n", i,list[i++]); 00045 //fp->close(); 00046 } 00047 } 00048 } 00049 dir->closedir(); 00050 } 00051 00052 00053 00054 00055 00056 /* select file by num. */ 00057 void Player::selectfile(char n){ 00058 00059 startplaysong=false; 00060 playerState =PS_STOP; 00061 playsong=false; 00062 green = n; 00063 } 00064 00065 00066 00067 /* This function stop an audio file. */ 00068 void Player::stop(void){ 00069 startplaysong=true; 00070 playerState =PS_STOP; 00071 } 00072 00073 00074 void Player::run(void){ 00075 if(datainput=='0'){ 00076 selectfile(0); 00077 playFile(list[green]); 00078 //stop(); 00079 } 00080 if(datainput=='1'){ 00081 selectfile(1); 00082 playFile(list[green]); 00083 //stop(); 00084 00085 } 00086 if(datainput=='2'){ 00087 selectfile(2); 00088 playFile(list[green]); 00089 //stop(); 00090 } 00091 if(datainput=='3'){ 00092 selectfile(3); 00093 playFile(list[green]); 00094 //stop(); 00095 } 00096 if(datainput=='4'){ 00097 selectfile(4); 00098 playFile(list[green]); 00099 //stop(); 00100 } 00101 if(datainput=='5'){ 00102 selectfile(5); 00103 playFile(list[green]); 00104 //stop(); 00105 } 00106 else{ 00107 stop(); 00108 } 00109 } 00110 00111 00112 00113 void Player::read(void){ 00114 pc.readable(); 00115 datainput = pc.getc(); 00116 00117 } 00118 00119 00120 00121 /* This function plays audio file. */ 00122 void Player::playFile(char *file) { 00123 int bytes; // How many bytes in buffer left 00124 char n; 00125 00126 playerState = PS_PLAY; 00127 00128 vs1053.setFreq(24000000); //hight speed 00129 00130 FileHandle *fp =sd.open(file, O_RDONLY); 00131 00132 if(fp == NULL) { 00133 printf("Could not open %s\r\n",file); 00134 00135 } 00136 else 00137 { 00138 printf("Playing %s ...\r\n",file); 00139 00140 /* Main playback loop */ 00141 while((bytes = fp->read(fileBuf,48000)) > 0) { 00142 { 00143 pc.printf("green\n"); 00144 if(pc.readable()){ 00145 break; 00146 //stop(); 00147 } 00148 vs1053.setFreq(24000000); 00149 bufptr = fileBuf; 00150 // actual audio data gets sent to VS10xx. 00151 while(bytes > 0) 00152 { 00153 n = (bytes < 32)?bytes:32; //defalt 32 00154 vs1053.writeData(bufptr,n); 00155 bytes -= n; 00156 bufptr += n; 00157 if(pc.readable()){ 00158 break; 00159 //stop(); 00160 } 00161 00162 } 00163 uint8_t vol = 0x00;//set vlume 00164 vs1053.setVolume(vol); //set vlume 00165 00166 if(playerState != PS_PLAY) //stop 00167 { 00168 fp->close(); 00169 vs1053.softReset(); 00170 } 00171 00172 } 00173 } 00174 } 00175 00176 } 00177
Generated on Thu Jul 14 2022 16:30:39 by
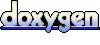