SAKURA Internet IoT Communication Module Library for mbed
Dependents: patlite_sakuraio sakuraio_lte_firmwareupdater shownet2017-iinebutton patlite_sakuraio_stack ... more
SakuraIO_SPI.cpp
00001 /* SAKURA Internet IoT Communication Module Library for mbed 00002 * 00003 * The MIT License (MIT) 00004 * 00005 * Copyright (c) SAKURA Internet Inc. 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), 00009 * to deal in the Software without restriction, including without limitation 00010 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 * and/or sell copies of the Software, and to permit persons to whom the Software 00012 * is furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included 00015 * in all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00018 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00020 * IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00021 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00022 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00023 */ 00024 00025 #include "mbed.h" 00026 #include "SakuraIO.h" 00027 #include "SakuraIO/debug.h" 00028 00029 00030 void SakuraIO_SPI::begin(){ 00031 dbgln("CS=0"); 00032 cs = 0; 00033 } 00034 00035 void SakuraIO_SPI::end(){ 00036 dbgln("CS=1"); 00037 cs = 1; 00038 wait_us(20); 00039 } 00040 00041 void SakuraIO_SPI::sendByte(uint8_t data){ 00042 wait_us(20); 00043 dbg("Send="); 00044 dbgln(data); 00045 wait_us(10); 00046 spi.write(data); 00047 } 00048 00049 00050 uint8_t SakuraIO_SPI::receiveByte(bool stop){ 00051 return receiveByte(); 00052 } 00053 00054 uint8_t SakuraIO_SPI::receiveByte(){ 00055 uint8_t ret; 00056 wait_us(10); 00057 ret = spi.write(0x00); 00058 dbg("Recv="); 00059 dbgln(ret); 00060 return ret; 00061 } 00062 00063 SakuraIO_SPI::SakuraIO_SPI(SPI &_spi, DigitalOut &_cs): spi(_spi), cs(_cs){ 00064 cs = 1; 00065 }
Generated on Tue Jul 12 2022 17:05:53 by
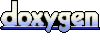