
This is a NetworkSocketAPI example for LWIPBP3595Interface_STA library. LWIPBP3595Interface_STA library only works with GR-PEACH. The base example is HelloLWIPInterface.
Dependencies: LWIPBP3595Interface_STA LWIPInterface NetworkSocketAPI mbed-rtos mbed
main.cpp
00001 /* main.cpp in HelloLWIPBP3595Interface */ 00002 /* Copyright (C) 2016 Grape Systems, Inc. */ 00003 /* The base file is main.cpp in HelloLWIPInterface. */ 00004 00005 /* main.cpp in HelloLWIPInterface */ 00006 /* NetworkSocketAPI Example Program 00007 * Copyright (c) 2015 ARM Limited 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); 00010 * you may not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, 00017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 */ 00021 00022 /* This is a NetworkSocketAPI example for LWIPBP3595Interface_STA library. */ 00023 /* LWIPBP3595Interface_STA library only works with GR-PEACH. */ 00024 /* The base example is HelloLWIPInterface. */ 00025 00026 /* 00027 Warning! 00028 When exporting and using it, increase the following stack size. 00029 00030 [LWIPInterface/lwip/lwipopts.h]------------- 00031 #define TCPIP_THREAD_STACKSIZE 1024 00032 -> 00033 #define TCPIP_THREAD_STACKSIZE 2048 00034 -------------------------------------------- 00035 */ 00036 00037 /* 00038 This program works with the following library. 00039 mbed-rtos : revision 115 00040 */ 00041 00042 #include "mbed.h" 00043 #include "LWIPBP3595Interface.h" 00044 #include "TCPSocket.h" 00045 00046 LWIPBP3595Interface wifi; 00047 00048 DigitalOut led(LED_GREEN); 00049 void blink() 00050 { 00051 led = !led; 00052 } 00053 00054 int main() 00055 { 00056 Ticker blinky; 00057 blinky.attach(blink, 0.4f); 00058 00059 printf("NetworkSocketAPI Example\r\n"); 00060 00061 wifi.connect("ssid", "password"); 00062 const char *ip = wifi.get_ip_address(); 00063 const char *mac = wifi.get_mac_address(); 00064 printf("IP address is: %s\r\n", ip ? ip : "No IP"); 00065 printf("MAC address is: %s\r\n", mac ? mac : "No MAC"); 00066 00067 SocketAddress addr(&wifi, "mbed.org"); 00068 printf("mbed.org resolved to: %s\r\n", addr.get_ip_address()); 00069 00070 TCPSocket socket(&wifi); 00071 socket.connect("4.ifcfg.me", 23); 00072 00073 char buffer[64]; 00074 int count = socket.recv(buffer, sizeof buffer); 00075 printf("public IP address is: %.15s\r\n", &buffer[15]); 00076 00077 socket.close(); 00078 wifi.disconnect(); 00079 00080 printf("Done\r\n"); 00081 }
Generated on Wed Jul 13 2022 00:27:18 by
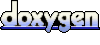