Rohm BH1749NUC color sensor library
Dependents: rohm-SensorShield-example
BH1749NUC.h
00001 /***************************************************************************** 00002 BH1749NUC.h 00003 00004 Copyright (c) 2018 ROHM Co.,Ltd. 00005 00006 Permission is hereby granted, free of charge, to any person obtaining a copy 00007 of this software and associated documentation files (the "Software"), to deal 00008 in the Software without restriction, including without limitation the rights 00009 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 copies of the Software, and to permit persons to whom the Software is 00011 furnished to do so, subject to the following conditions: 00012 00013 The above copyright notice and this permission notice shall be included in 00014 all copies or substantial portions of the Software. 00015 00016 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 THE SOFTWARE. 00023 ******************************************************************************/ 00024 #ifndef _BH1749NUC_H_ 00025 #define _BH1749NUC_H_ 00026 00027 #include "mbed.h" 00028 00029 #ifdef _DEBUG 00030 #undef DEBUG_PRINT 00031 #define DEBUG_PRINT(...) printf(__VA_ARGS__) 00032 #else 00033 #define DEBUG_PRINT(...) 00034 #endif 00035 00036 #define BH1749NUC_DEVICE_ADDRESS_38 (0x38 << 1) // 7bit Addrss 00037 #define BH1749NUC_DEVICE_ADDRESS_39 (0x39 << 1) // 7bit Addrss 00038 #define BH1749NUC_PART_ID_VAL (0x0D) 00039 #define BH1749NUC_MANUFACT_ID_VAL (0xE0) 00040 00041 #define BH1749NUC_SYSTEM_CONTROL (0x40) 00042 #define BH1749NUC_MODE_CONTROL1 (0x41) 00043 #define BH1749NUC_MODE_CONTROL2 (0x42) 00044 #define BH1749NUC_RED_DATA_LSB (0x50) 00045 #define BH1749NUC_MANUFACTURER_ID (0x92) 00046 00047 #define BH1749NUC_MODE_CONTROL1_MEAS_MODE_120MS (2) 00048 #define BH1749NUC_MODE_CONTROL1_MEAS_MODE_240MS (3) 00049 #define BH1749NUC_MODE_CONTROL1_RGB_GAIN_X1 (1 << 3) 00050 #define BH1749NUC_MODE_CONTROL1_RGB_GAIN_X32 (3 << 3) 00051 #define BH1749NUC_MODE_CONTROL1_IR_GAIN_X1 (1 << 5) 00052 #define BH1749NUC_MODE_CONTROL1_IR_GAIN_X32 (3 << 5) 00053 #define BH1749NUC_MODE_CONTROL2_RGB_EN (1 << 4) 00054 00055 #define BH1749NUC_MODE_CONTROL1_VAL (BH1749NUC_MODE_CONTROL1_MEAS_MODE_120MS | BH1749NUC_MODE_CONTROL1_RGB_GAIN_X1 | BH1749NUC_MODE_CONTROL1_IR_GAIN_X1) 00056 #define BH1749NUC_MODE_CONTROL2_VAL (BH1749NUC_MODE_CONTROL2_RGB_EN) 00057 00058 #define GET_BYTE_RED_TO_GREEN2 (12) 00059 #define WAIT_TMT2_MAX (340) 00060 00061 /** Interface for controlling BME280 Combined humidity and pressure sensor 00062 * 00063 * @code 00064 * #include "mbed.h" 00065 * #include "BH1749NUC.h" 00066 * 00067 * BH1749NUC color(I2C_SDA, I2C_SCL); 00068 * 00069 * int main(void) { 00070 * uint16_t buf[5]; 00071 * color.init(); 00072 * 00073 * while (1) { 00074 * color.get_val(buf); 00075 * printf("BH1749 color : [R] %04x, [G] %04x, [B] %04x, [IR] %04x, [G2] %04x\n", buf[0], buf[1], buf[2], buf[3], buf[4]); 00076 * wait(1); 00077 * } 00078 * } 00079 * @endcode 00080 */ 00081 00082 /** BH1749NUC class 00083 * 00084 * BH1749NUC: A library to correct color data using Rohm BH1749NUC color sensor device 00085 * 00086 */ 00087 class BH1749NUC 00088 { 00089 public: 00090 /** Create a BH1749NUC instance 00091 * which is connected to specified I2C pins with specified address 00092 * 00093 * @param sda I2C-bus SDA pin 00094 * @param sdl I2C-bus SCL pin 00095 * @param addr slave address of the I2C peripheral (default: 0x72) 00096 */ 00097 BH1749NUC(PinName sda, PinName scl, int addr = BH1749NUC_DEVICE_ADDRESS_39); 00098 /** 00099 * BH1749NUC destructor 00100 */ 00101 ~BH1749NUC(); 00102 /** 00103 * Initialize BH1749NUC device 00104 */ 00105 int init(void) ; 00106 /** 00107 * Get color sensor raw value 00108 * @param data buffer to store raw data 00109 */ 00110 int get_rawval(char *data); 00111 /** 00112 * Get color sensor value 00113 * @param data buffer to store sensor data (R, B, G, IR and G2) 00114 */ 00115 int get_val(unsigned short *data); 00116 private: 00117 int write(char memory_address, char *data, int size); 00118 int read(char memory_address, char *data, int size); 00119 I2C m_i2c; 00120 int m_addr; 00121 }; 00122 00123 #endif // _BH1749NUC_H_
Generated on Wed Jul 13 2022 22:24:55 by
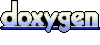