
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
x_nucleo_iks01a1.c
00001 /** 00002 ****************************************************************************** 00003 * @file x_nucleo_iks01a1.c 00004 * @author MEMS Application Team 00005 * @version V1.0.0 00006 * @date 30-July-2014 00007 * @brief This file provides X_NUCLEO_IKS01A1 MEMS shield board specific functions 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "x_nucleo_iks01a1.h" 00039 00040 /** @addtogroup BSP 00041 * @{ 00042 */ 00043 00044 /** @addtogroup X_NUCLEO_IKS01A1 00045 * @{ 00046 */ 00047 00048 /** @defgroup X_NUCLEO_IKS01A1_Private_Types_Definitions 00049 * @{ 00050 */ 00051 00052 /** 00053 * @} 00054 */ 00055 00056 00057 /** @defgroup X_NUCLEO_IKS01A1_Private_Defines 00058 * @{ 00059 */ 00060 #ifndef NULL 00061 #define NULL (void *) 0 00062 #endif 00063 /** 00064 * @} 00065 */ 00066 00067 /** @defgroup X_NUCLEO_IKS01A1_Private_Macros 00068 * @{ 00069 */ 00070 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup X_NUCLEO_IKS01A1_Private_Variables 00076 * @{ 00077 */ 00078 00079 uint32_t I2C_SHIELDS_Timeout = NUCLEO_I2C_SHIELDS_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00080 00081 static I2C_HandleTypeDef I2C_SHIELDS_Handle; 00082 00083 00084 #ifdef USE_FREE_RTOS 00085 osMutexId I2C1_Mutex_id = 0; 00086 #endif 00087 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup X_NUCLEO_IKS01A1_Private_Function_Prototypes 00093 * @{ 00094 */ 00095 00096 /* Link function for 6 Axes IMU peripheral */ 00097 void IMU_6AXES_IO_Init(void); 00098 void IMU_6AXES_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t WriteAddr, uint16_t NumByteToWrite); 00099 void IMU_6AXES_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t ReadAddr, uint16_t NumByteToRead); 00100 00101 /* Link function for MAGNETO peripheral */ 00102 void MAGNETO_IO_Init(void); 00103 void MAGNETO_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t WriteAddr, uint16_t NumByteToWrite); 00104 void MAGNETO_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t ReadAddr, uint16_t NumByteToRead); 00105 00106 /* Link function for PRESSURE peripheral */ 00107 void PRESSURE_IO_Init(void); 00108 void PRESSURE_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t WriteAddr, uint16_t NumByteToWrite); 00109 void PRESSURE_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead); 00110 00111 /* Link function for HUM_TEMP peripheral */ 00112 void HUM_TEMP_IO_Init(void); 00113 void HUM_TEMP_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t WriteAddr, uint16_t NumByteToWrite); 00114 void HUM_TEMP_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead); 00115 00116 00117 static void I2C_SHIELDS_MspInit(void); 00118 static void I2C_SHIELDS_Error(uint8_t Addr); 00119 static void I2C_SHIELDS_ReadData(uint8_t* pBuffer, uint8_t Addr, uint8_t Reg, uint16_t Size); 00120 static void I2C_SHIELDS_WriteData(uint8_t* pBuffer, uint8_t Addr, uint8_t Reg, uint16_t Size); 00121 static void I2C_SHIELDS_Init(void); 00122 00123 /** 00124 * @} 00125 */ 00126 00127 /** @defgroup X_NUCLEO_IKS01A1_Private_Functions 00128 * @{ 00129 */ 00130 00131 00132 00133 /********************************* LINK IMU 6 AXES *****************************/ 00134 /** 00135 * @brief Configures Imu 6 axes I2C interface. 00136 * @param None 00137 * @retval None 00138 */ 00139 void IMU_6AXES_IO_Init(void) 00140 { 00141 I2C_SHIELDS_Init(); 00142 } 00143 00144 00145 /** 00146 * @brief Writes a buffer to the IMU 6 axes sensor. 00147 * @param pBuffer: pointer to data to be written. 00148 * @param DeviceAddr: specifies the slave address to be programmed. 00149 * @param RegisterAddr: specifies the IMU 6 axes register to be written. 00150 * @param NumByteToWrite: number of bytes to be written. 00151 * @retval None. 00152 */ 00153 void IMU_6AXES_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToWrite) 00154 { 00155 #ifdef USE_FREE_RTOS 00156 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00157 #endif 00158 00159 /* call I2C_SHIELDS Read data bus function */ 00160 I2C_SHIELDS_WriteData(pBuffer, DeviceAddr, RegisterAddr, NumByteToWrite); 00161 00162 #ifdef USE_FREE_RTOS 00163 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00164 #endif 00165 } 00166 00167 /** 00168 * @brief Reads a buffer from the IMU 6 axes sensor. 00169 * @param pBuffer: pointer to data to be read. 00170 * @param DeviceAddr: specifies the address of the device. 00171 * @param RegisterAddr: specifies the IMU 6 axes internal address register to read from. 00172 * @param NumByteToRead: number of bytes to be read. 00173 * @retval None. 00174 */ 00175 void IMU_6AXES_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead) 00176 { 00177 #ifdef USE_FREE_RTOS 00178 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00179 #endif 00180 00181 /* call I2C_SHIELDS Read data bus function */ 00182 I2C_SHIELDS_ReadData(pBuffer, DeviceAddr, RegisterAddr, NumByteToRead); 00183 00184 #ifdef USE_FREE_RTOS 00185 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00186 #endif 00187 } 00188 00189 00190 00191 /********************************* LINK MAGNETO *****************************/ 00192 /** 00193 * @brief Configures MAGNETO I2C interface. 00194 * @param None 00195 * @retval None 00196 */ 00197 void MAGNETO_IO_Init(void) 00198 { 00199 I2C_SHIELDS_Init(); 00200 } 00201 00202 00203 /** 00204 * @brief Writes a buffer to the MAGNETO sensor. 00205 * @param pBuffer: pointer to data to be written. 00206 * @param DeviceAddr: specifies the slave address to be programmed. 00207 * @param RegisterAddr: specifies the MAGNETO register to be written. 00208 * @param NumByteToWrite: number of bytes to be written. 00209 * @retval None. 00210 */ 00211 void MAGNETO_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToWrite) 00212 { 00213 #ifdef USE_FREE_RTOS 00214 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00215 #endif 00216 00217 /* call I2C_SHIELDS Read data bus function */ 00218 I2C_SHIELDS_WriteData(pBuffer, DeviceAddr, RegisterAddr, NumByteToWrite); 00219 00220 #ifdef USE_FREE_RTOS 00221 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00222 #endif 00223 } 00224 00225 /** 00226 * @brief Reads a buffer from the MAGNETO sensor. 00227 * @param pBuffer: pointer to data to be read. 00228 * @param DeviceAddr: specifies the address of the device. 00229 * @param RegisterAddr: specifies the MAGNETO internal address register to read from. 00230 * @param NumByteToRead: number of bytes to be read. 00231 * @retval None. 00232 */ 00233 void MAGNETO_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead) 00234 { 00235 #ifdef USE_FREE_RTOS 00236 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00237 #endif 00238 00239 /* call I2C_SHIELDS Read data bus function */ 00240 I2C_SHIELDS_ReadData(pBuffer, DeviceAddr, RegisterAddr, NumByteToRead); 00241 00242 #ifdef USE_FREE_RTOS 00243 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00244 #endif 00245 } 00246 00247 00248 00249 /********************************* LINK PRESSURE *****************************/ 00250 /** 00251 * @brief Configures Pressure I2C interface. 00252 * @param None 00253 * @retval None 00254 */ 00255 void PRESSURE_IO_Init(void) 00256 { 00257 I2C_SHIELDS_Init(); 00258 } 00259 00260 00261 /** 00262 * @brief Writes a buffer to the Pressure sensor. 00263 * @param pBuffer: pointer to data to be written. 00264 * @param DeviceAddr: specifies the slave address to be programmed. 00265 * @param RegisterAddr: specifies the Pressure register to be written. 00266 * @param NumByteToWrite: number of bytes to be written. 00267 * @retval None. 00268 */ 00269 void PRESSURE_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToWrite) 00270 { 00271 #ifdef USE_FREE_RTOS 00272 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00273 #endif 00274 /* call I2C_SHIELDS Read data bus function */ 00275 I2C_SHIELDS_WriteData(pBuffer, DeviceAddr, RegisterAddr, NumByteToWrite); 00276 00277 #ifdef USE_FREE_RTOS 00278 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00279 #endif 00280 } 00281 00282 /** 00283 * @brief Reads a buffer from the Pressure sensor. 00284 * @param pBuffer: pointer to data to be read. 00285 * @param DeviceAddr: specifies the address of the device. 00286 * @param RegisterAddr: specifies the Pressure internal address register to read from. 00287 * @param NumByteToRead: number of bytes to be read. 00288 * @retval None. 00289 */ 00290 void PRESSURE_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead) 00291 { 00292 #ifdef USE_FREE_RTOS 00293 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00294 #endif 00295 /* call I2C_SHIELDS Read data bus function */ 00296 I2C_SHIELDS_ReadData(pBuffer, DeviceAddr, RegisterAddr, NumByteToRead); 00297 00298 #ifdef USE_FREE_RTOS 00299 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00300 #endif 00301 } 00302 00303 00304 /********************************* LINK HUM_TEMP *****************************/ 00305 /** 00306 * @brief Configures Humidity and Temperature I2C interface. 00307 * @param None 00308 * @retval None 00309 */ 00310 void HUM_TEMP_IO_Init(void) 00311 { 00312 I2C_SHIELDS_Init(); 00313 } 00314 00315 00316 /** 00317 * @brief Writes a buffer to the HUM_TEMP sensor. 00318 * @param pBuffer: pointer to data to be written. 00319 * @param DeviceAddr: specifies the slave address to be programmed. 00320 * @param RegisterAddr: specifies the Pressure register to be written. 00321 * @param NumByteToWrite: number of bytes to be written. 00322 * @retval None. 00323 */ 00324 void HUM_TEMP_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToWrite) 00325 { 00326 #ifdef USE_FREE_RTOS 00327 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00328 #endif 00329 /* call I2C_SHIELDS Read data bus function */ 00330 I2C_SHIELDS_WriteData(pBuffer, DeviceAddr, RegisterAddr, NumByteToWrite); 00331 #ifdef USE_FREE_RTOS 00332 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00333 #endif 00334 } 00335 00336 /** 00337 * @brief Reads a buffer from the Uvi sensor. 00338 * @param pBuffer: pointer to data to be read. 00339 * @param DeviceAddr: specifies the address of the device. 00340 * @param RegisterAddr: specifies the Pressure internal address register to read from. 00341 * @param NumByteToRead: number of bytes to be read. 00342 * @retval None. 00343 */ 00344 void HUM_TEMP_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead) 00345 { 00346 #ifdef USE_FREE_RTOS 00347 NUCLEO_I2C_SHIELDS_MUTEX_TAKE(); 00348 #endif 00349 /* call I2C_SHIELDS Read data bus function */ 00350 I2C_SHIELDS_ReadData(pBuffer, DeviceAddr, RegisterAddr, NumByteToRead); 00351 #ifdef USE_FREE_RTOS 00352 NUCLEO_I2C_SHIELDS_MUTEX_RELEASE(); 00353 #endif 00354 } 00355 00356 00357 00358 /******************************* I2C Routines**********************************/ 00359 /** 00360 * @brief Configures I2C interface. 00361 * @param None 00362 * @retval None 00363 */ 00364 static void I2C_SHIELDS_Init(void) 00365 { 00366 if(HAL_I2C_GetState(&I2C_SHIELDS_Handle) == HAL_I2C_STATE_RESET) 00367 { 00368 /* I2C_SHIELDS peripheral configuration */ 00369 // I2C_SHIELDS_Handle.Init.ClockSpeed = NUCLEO_I2C_SHIELDS_SPEED; 00370 // I2C_SHIELDS_Handle.Init.DutyCycle = I2C_DUTYCYCLE_2; 00371 #ifdef STM32F401xE 00372 I2C_SHIELDS_Handle.Init.ClockSpeed = NUCLEO_I2C_SHIELDS_SPEED; 00373 I2C_SHIELDS_Handle.Init.DutyCycle = I2C_DUTYCYCLE_2; 00374 #endif 00375 #ifdef STM32L053xx 00376 I2C_SHIELDS_Handle.Init.Timing = 0x0070D8FF; /*Refer AN4235-Application note Document*/ 00377 #endif 00378 I2C_SHIELDS_Handle.Init.OwnAddress1 = 0x33; 00379 I2C_SHIELDS_Handle.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00380 I2C_SHIELDS_Handle.Instance = NUCLEO_I2C_SHIELDS; 00381 00382 /* Init the I2C */ 00383 I2C_SHIELDS_MspInit(); 00384 HAL_I2C_Init(&I2C_SHIELDS_Handle); 00385 } 00386 } 00387 00388 /** 00389 * @brief Write a value in a register of the device through BUS. 00390 * @param Addr: Device address on BUS Bus. 00391 * @param Reg: The target register address to write 00392 * @param Value: The target register value to be written 00393 * @retval HAL status 00394 */ 00395 00396 static void I2C_SHIELDS_WriteData(uint8_t* pBuffer, uint8_t Addr, uint8_t Reg, uint16_t Size) 00397 { 00398 HAL_StatusTypeDef status = HAL_OK; 00399 00400 status = HAL_I2C_Mem_Write(&I2C_SHIELDS_Handle, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, pBuffer, Size, I2C_SHIELDS_Timeout); 00401 00402 /* Check the communication status */ 00403 if(status != HAL_OK) 00404 { 00405 /* Execute user timeout callback */ 00406 I2C_SHIELDS_Error(Addr); 00407 } 00408 } 00409 00410 00411 /** 00412 * @brief Read a register of the device through BUS 00413 * @param Addr: Device address on BUS . 00414 * @param Reg: The target register address to read 00415 * @retval HAL status 00416 */ 00417 static void I2C_SHIELDS_ReadData(uint8_t* pBuffer, uint8_t Addr, uint8_t Reg, uint16_t Size) 00418 { 00419 HAL_StatusTypeDef status = HAL_OK; 00420 00421 status = HAL_I2C_Mem_Read(&I2C_SHIELDS_Handle, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, pBuffer, Size, I2C_SHIELDS_Timeout); 00422 00423 /* Check the communication status */ 00424 if(status != HAL_OK) 00425 { 00426 /* Execute user timeout callback */ 00427 I2C_SHIELDS_Error(Addr); 00428 } 00429 00430 } 00431 00432 /** 00433 * @brief Manages error callback by re-initializing I2C. 00434 * @param Addr: I2C Address 00435 * @retval None 00436 */ 00437 static void I2C_SHIELDS_Error(uint8_t Addr) 00438 { 00439 /* De-initialize the I2C comunication bus */ 00440 HAL_I2C_DeInit(&I2C_SHIELDS_Handle); 00441 00442 /* Re-Initiaize the I2C comunication bus */ 00443 I2C_SHIELDS_Init(); 00444 } 00445 00446 /** 00447 * @brief I2C MSP Initialization 00448 * @param None 00449 * @retval None 00450 */ 00451 00452 static void I2C_SHIELDS_MspInit(void) 00453 { 00454 GPIO_InitTypeDef GPIO_InitStruct; 00455 00456 #ifdef USE_FREE_RTOS 00457 if(!NUCLEO_I2C_SHIELDS_MUTEX) { 00458 NUCLEO_I2C_SHIELDS_MUTEX = osMutexCreate(0); 00459 } 00460 #endif 00461 00462 /* Enable I2C GPIO clocks */ 00463 NUCLEO_I2C_SHIELDS_SCL_SDA_GPIO_CLK_ENABLE(); 00464 00465 /* I2C_SHIELDS SCL and SDA pins configuration -------------------------------------*/ 00466 GPIO_InitStruct.Pin = NUCLEO_I2C_SHIELDS_SCL_PIN | NUCLEO_I2C_SHIELDS_SDA_PIN; 00467 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00468 GPIO_InitStruct.Speed = GPIO_SPEED_FAST; 00469 GPIO_InitStruct.Pull = GPIO_NOPULL; 00470 GPIO_InitStruct.Alternate = NUCLEO_I2C_SHIELDS_SCL_SDA_AF; 00471 HAL_GPIO_Init(NUCLEO_I2C_SHIELDS_SCL_SDA_GPIO_PORT, &GPIO_InitStruct); 00472 00473 /* Enable the I2C_SHIELDS peripheral clock */ 00474 NUCLEO_I2C_SHIELDS_CLK_ENABLE(); 00475 00476 /* Force the I2C peripheral clock reset */ 00477 NUCLEO_I2C_SHIELDS_FORCE_RESET(); 00478 00479 /* Release the I2C peripheral clock reset */ 00480 NUCLEO_I2C_SHIELDS_RELEASE_RESET(); 00481 00482 /* Enable and set I2C_SHIELDS Interrupt to the highest priority */ 00483 HAL_NVIC_SetPriority(NUCLEO_I2C_SHIELDS_EV_IRQn, 0, 0); 00484 HAL_NVIC_EnableIRQ(NUCLEO_I2C_SHIELDS_EV_IRQn); 00485 00486 #ifdef STM32F401xE 00487 /* Enable and set I2C_SHIELDS Interrupt to the highest priority */ 00488 HAL_NVIC_SetPriority(NUCLEO_I2C_SHIELDS_ER_IRQn, 0, 0); 00489 HAL_NVIC_EnableIRQ(NUCLEO_I2C_SHIELDS_ER_IRQn); 00490 #endif 00491 } 00492 00493 00494 /** 00495 * @} 00496 */ 00497 00498 /** 00499 * @} 00500 */ 00501 00502 /** 00503 * @} 00504 */ 00505 00506 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00507
Generated on Tue Jul 12 2022 21:42:00 by
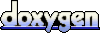