
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
link_layer.h
00001 /******************** (C) COPYRIGHT 2012 STMicroelectronics ******************** 00002 * File Name : link_layer.h 00003 * Author : AMS - HEA&RF BU 00004 * Version : V1.0.0 00005 * Date : 19-July-2012 00006 * Description : Header file for BlueNRG's link layer. It contains 00007 * definition of functions for link layer, most of which are 00008 * mapped to HCI commands. 00009 ******************************************************************************** 00010 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00011 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME. 00012 * AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT, 00013 * INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE 00014 * CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING 00015 * INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00016 *******************************************************************************/ 00017 00018 #ifndef _LINK_LAYER_H 00019 #define _LINK_LAYER_H 00020 00021 #include <ble_status.h> 00022 00023 /****************************************************************************** 00024 * Types 00025 *****************************************************************************/ 00026 00027 /** 00028 * advertising policy for filtering (white list related) 00029 */ 00030 typedef tHalUint8 tAdvPolicy; 00031 #define NO_WHITE_LIST_USE (0X00) 00032 #define WHITE_LIST_FOR_ONLY_SCAN (0X01) 00033 #define WHITE_LIST_FOR_ONLY_CONN (0X02) 00034 #define WHITE_LIST_FOR_ALL (0X03) 00035 00036 /** 00037 * Bluetooth 48 bit address (in little-endian order). 00038 */ 00039 typedef tHalUint8 tBDAddr[6]; 00040 00041 00042 /** 00043 * Bluetooth address type 00044 */ 00045 typedef tHalUint8 tAddrType; 00046 #define RANDOM_ADDR (1) 00047 #define PUBLIC_ADDR (0) 00048 00049 /** 00050 * Advertising type 00051 */ 00052 typedef tHalUint8 tAdvType; 00053 /** 00054 * undirected scannable and connectable 00055 */ 00056 #define ADV_IND (0x00) 00057 00058 /** 00059 * directed non scannable 00060 */ 00061 #define ADV_DIRECT_IND (0x01) 00062 00063 /** 00064 * scannable non connectable 00065 */ 00066 #define ADV_SCAN_IND (0x02) 00067 00068 /** 00069 * non-connectable and no scan response (used for passive scan) 00070 */ 00071 #define ADV_NONCONN_IND (0x03) 00072 00073 00074 /* 0X04-0XFF RESERVED */ 00075 00076 00077 /** 00078 * lowest allowed interval value for connectable types(20ms)..multiple of 625us 00079 */ 00080 #define ADV_INTERVAL_LOWEST_CONN (0X0020) 00081 00082 /** 00083 * highest allowed interval value (10.24s)..multiple of 625us. 00084 */ 00085 #define ADV_INTERVAL_HIGHEST (0X4000) 00086 00087 /** 00088 * lowest allowed interval value for non connectable types 00089 * (100ms)..multiple of 625us. 00090 */ 00091 #define ADV_INTERVAL_LOWEST_NONCONN (0X00a0) 00092 00093 /** 00094 * Default value of advertising interval for both min/max values. 00095 * This will be used if host does not specify any advertising parameters 00096 * including advIntervalMax and advIntervalMin 00097 * value = 1.28 sec (in units of 625 us) 00098 */ 00099 #define ADV_INTERVAL_DEFAULT (0x0800) 00100 00101 #define ADV_CH_37 0x01 00102 #define ADV_CH_38 0x02 00103 #define ADV_CH_39 0x04 00104 00105 00106 00107 #endif /* _LINK_LAYER_H */
Generated on Tue Jul 12 2022 21:42:00 by
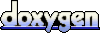