
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
hts221.h
00001 /** 00002 ****************************************************************************** 00003 * @file hts221.h 00004 * @author MEMS Application Team 00005 * @version V1.0.0 00006 * @date 30-July-2014 00007 * @brief This file contains definitions for the hts221.c 00008 * firmware driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 #ifndef __HTS221_H 00042 #define __HTS221_H 00043 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "hum_temp.h" 00050 00051 /** @addtogroup BSP 00052 * @{ 00053 */ 00054 00055 /** @addtogroup HTS221 00056 * @{ 00057 */ 00058 00059 /** @defgroup HTS221_Exported_Constants 00060 * @{ 00061 */ 00062 00063 /** 00064 * @brief Device Address 00065 */ 00066 #define HTS221_ADDRESS 0xBE 00067 00068 /******************************************************************************/ 00069 /*************************** START REGISTER MAPPING **************************/ 00070 /******************************************************************************/ 00071 00072 00073 /** 00074 * @brief Device identification register. 00075 * \code 00076 * Read 00077 * Default value: 0xBC 00078 * 7:0 This read-only register contains the device identifier that, for HTS221, is set to BCh. 00079 * \endcode 00080 */ 00081 #define HTS221_WHO_AM_I_ADDR 0x0F 00082 00083 00084 /** 00085 * @brief Humidity resolution Register 00086 * \code 00087 * Read/write 00088 * Default value: 0x1B 00089 * 7:6 RFU 00090 * 5:3 AVGT2-AVGT0: Temperature internal average. 00091 * AVGT2 | AVGT1 | AVGT0 | Nr. Internal Average 00092 * ------------------------------------------------------ 00093 * 0 | 0 | 0 | 2 00094 * 0 | 0 | 1 | 4 00095 * 0 | 1 | 0 | 8 00096 * 0 | 1 | 1 | 16 00097 * 1 | 0 | 0 | 32 00098 * 1 | 0 | 1 | 64 00099 * 1 | 1 | 0 | 128 00100 * 1 | 1 | 1 | 256 00101 * 00102 * 2:0 AVGH2-AVGH0: Humidity internal average. 00103 * AVGH2 | AVGH1 | AVGH0 | Nr. Internal Average 00104 * ------------------------------------------------------ 00105 * 0 | 0 | 0 | 4 00106 * 0 | 0 | 1 | 8 00107 * 0 | 1 | 0 | 16 00108 * 0 | 1 | 1 | 32 00109 * 1 | 0 | 0 | 64 00110 * 1 | 0 | 1 | 128 00111 * 1 | 1 | 0 | 256 00112 * 1 | 1 | 1 | 512 00113 * 00114 * \endcode 00115 */ 00116 #define HTS221_RES_CONF_ADDR 0x10 00117 00118 00119 /** 00120 * @brief INFO Register (LSB data) 00121 * \code 00122 * Read/write 00123 * Default value: 0x00 00124 * 7:0 INFO7-INFO0: Lower part of the INFO reference 00125 * used for traceability of the sample. 00126 * \endcode 00127 */ 00128 #define HTS221_INFO_L_ADDR 0x1E 00129 00130 00131 /** 00132 * @brief INFO & Calibration Version Register (LSB data) 00133 * \code 00134 * Read/write 00135 * Default value: 0x00 00136 * 7:6 CALVER1:CALVER0 00137 * 5:0 INFO13-INFO8: Higher part of the INFO reference 00138 * used for traceability of the sample. 00139 * \endcode 00140 */ 00141 #define HTS221_INFO_H_ADDR 0x1F 00142 00143 00144 /** 00145 * @brief Humidity sensor control register 1 00146 * \code 00147 * Read/write 00148 * Default value: 0x00 00149 * 7 PD: power down control. 0 - disable; 1 - enable 00150 * 6:3 RFU 00151 * 2 BDU: block data update. 0 - disable; 1 - enable 00152 * 1:0 RFU 00153 * \endcode 00154 */ 00155 00156 #define HTS221_CTRL_REG1_ADDR 0x20 00157 00158 00159 /** 00160 * @brief Humidity sensor control register 2 00161 * \code 00162 * Read/write 00163 * Default value: 0x00 00164 * 7 BOOT: Reboot memory content. 0: normal mode; 1: reboot memory content 00165 * 6:3 Reserved. 00166 * 2 Reserved. 00167 * 1 Reserved. 00168 * 0 ONE_SHOT: One shot enable. 0: waiting for start of conversion; 1: start for a new dataset 00169 * \endcode 00170 */ 00171 #define HTS221_CTRL_REG2_ADDR 0x21 00172 00173 00174 /** 00175 * @brief Humidity sensor control register 3 00176 * \code 00177 * Read/write 00178 * Default value: 0x00 00179 * [7] DRDY_H_L: Data Ready output signal active high, low (0: active high -default;1: active low) 00180 * [6] PP_OD: Push-pull / Open Drain selection on pin 3 (DRDY) (0: push-pull - default; 1: open drain) 00181 * [5:3] Reserved 00182 * [2] DRDY_EN: Data Ready enable (0: Data Ready disabled - default;1: Data Ready signal available on pin 3) 00183 * [1:0] Reserved 00184 * \endcode 00185 */ 00186 #define HTS221_CTRL_REG3_ADDR 0x22 00187 00188 00189 /** 00190 * @brief Status Register 00191 * \code 00192 * Read 00193 * Default value: 0x00 00194 * 7:2 RFU 00195 * 1 H_DA: Humidity data available. 0: new data for Humidity is not yet available; 1: new data for Humidity is available. 00196 * 0 T_DA: Temperature data available. 0: new data for temperature is not yet available; 1: new data for temperature is available. 00197 * \endcode 00198 */ 00199 #define HTS221_STATUS_REG_ADDR 0x27 00200 00201 00202 /** 00203 * @brief Humidity data (LSB). 00204 * \code 00205 * Read 00206 * Default value: 0x00. 00207 * POUT7 - POUT0: Humidity data LSB (2's complement) => signed 16 bits 00208 * RAW Humidity output data: Hout(%)=(HUMIDITY_OUT_H & HUMIDITY_OUT_L). 00209 * \endcode 00210 */ 00211 #define HTS221_HUMIDITY_OUT_L_ADDR 0x28 00212 00213 00214 /** 00215 * @brief Humidity data (MSB). 00216 * \code 00217 * Read 00218 * Default value: 0x00. 00219 * POUT7 - POUT0: Humidity data LSB (2's complement) => signed 16 bits 00220 * RAW Humidity output data: Hout(%)=(HUMIDITY_OUT_H & HUMIDITY_OUT_L). 00221 * \endcode 00222 */ 00223 #define HTS221_HUMIDITY_OUT_H_ADDR 0x29 00224 00225 00226 /** 00227 * @brief Temperature data (LSB). 00228 * \code 00229 * Read 00230 * Default value: 0x00. 00231 * TOUT7 - TOUT0: temperature data LSB (2's complement) => signed 16 bits 00232 * RAW Temperature output data: Tout (LSB)=(TEMP_OUT_H & TEMP_OUT_L). 00233 * \endcode 00234 */ 00235 #define HTS221_TEMP_OUT_L_ADDR 0x2A 00236 00237 00238 /** 00239 * @brief Temperature data (MSB). 00240 * \code 00241 * Read 00242 * Default value: 0x00. 00243 * TOUT15 - TOUT8: temperature data MSB (2's complement) => signed 16 bits 00244 * RAW Temperature output data: Tout (LSB)=(TEMP_OUT_H & TEMP_OUT_L). 00245 * \endcode 00246 */ 00247 #define HTS221_TEMP_OUT_H_ADDR 0x2B 00248 00249 00250 /* 00251 *@brief Humidity 0 Register in %RH with sensitivity=2 00252 *\code 00253 * Read 00254 * Value: (Unsigned 8 Bit)/2 00255 *\endcode 00256 */ 00257 #define HTS221_H0_RH_X2_ADDR 0x30 00258 00259 00260 /* 00261 *@brief Humidity 1 Register in %RH with sensitivity=2 00262 *\code 00263 * Read 00264 * Value: (Unsigned 8 Bit)/2 00265 *\endcode 00266 */ 00267 #define HTS221_H1_RH_X2_ADDR 0x31 00268 00269 00270 /* 00271 *@brief Temperature 0 Register in deg with sensitivity=8 00272 *\code 00273 * Read 00274 * Value: (Unsigned 16 Bit)/2 00275 *\endcode 00276 */ 00277 #define HTS221_T0_degC_X8_ADDR 0x32 00278 00279 00280 /* 00281 *@brief Temperature 1 Register in deg with sensitivity=8 00282 *\code 00283 * Read 00284 * Value: (Unsigned 16 Bit)/2 00285 *\endcode 00286 */ 00287 #define HTS221_T1_degC_X8_ADDR 0x33 00288 00289 00290 /* 00291 *@brief Temperature 1/0 MSB Register in deg with sensitivity=8 00292 *\code 00293 * Read 00294 * Value: (Unsigned 16 Bit)/2 00295 * 3:2 T1(9):T1(8) MSB T1_degC_X8 bits 00296 * 1:0 T0(9):T0(8) MSB T0_degC_X8 bits 00297 *\endcode 00298 */ 00299 #define HTS221_T1_T0_MSB_X8_ADDR 0x35 00300 00301 00302 /* 00303 *@brief Humidity LOW CALIBRATION Register 00304 *\code 00305 * Read 00306 * Default value: 0x00. 00307 * H0_T0_TOUT7 - H0_T0_TOUT0: HUMIDITY data lSB (2's complement) => signed 16 bits 00308 *\endcode 00309 */ 00310 #define HTS221_H0_T0_OUT_L_ADDR 0x36 00311 00312 00313 /* 00314 *@brief Humidity LOW CALIBRATION Register 00315 *\code 00316 * Read 00317 * Default value: 0x00. 00318 * H0_T0_TOUT15 - H0_T0_TOUT8: HUMIDITY data mSB (2's complement) => signed 16 bits 00319 *\endcode 00320 */ 00321 #define HTS221_H0_T0_OUT_H_ADDR 0x37 00322 00323 00324 /* 00325 *@brief Humidity HIGH CALIBRATION Register 00326 *\code 00327 * Read 00328 * Default value: 0x00. 00329 * H1_T0_TOUT7 - H1_T0_TOUT0: HUMIDITY data lSB (2's complement) => signed 16 bits 00330 *\endcode 00331 */ 00332 #define HTS221_H1_T0_OUT_L_ADDR 0x3A 00333 00334 00335 /* 00336 *@brief Humidity HIGH CALIBRATION Register 00337 *\code 00338 * Read 00339 * Default value: 0x00. 00340 * H1_T0_TOUT15 - H1_T0_TOUT8: HUMIDITY data mSB (2's complement) => signed 16 bits 00341 *\endcode 00342 */ 00343 #define HTS221_H1_T0_OUT_H_ADDR 0x3B 00344 00345 00346 /** 00347 * @brief Low Calibration Temperature Register (LSB). 00348 * \code 00349 * Read 00350 * Default value: 0x00. 00351 * T0_OUT7 - T0_OUT0: temperature data LSB (2's complement) => signed 16 bits 00352 * RAW LOW Calibration data: T0_OUT (LSB)=(T0_OUT_H & T0_OUT_L). 00353 * \endcode 00354 */ 00355 #define HTS221_T0_OUT_L_ADDR 0x3C 00356 00357 00358 /** 00359 * @brief Low Calibration Temperature Register (MSB) 00360 * \code 00361 * Read 00362 * Default value: 0x00. 00363 * T0_OUT15 - T0_OUT8: temperature data MSB (2's complement) => signed 16 bits 00364 * RAW LOW Calibration data: T0_OUT (LSB)=(T0_OUT_H & T0_OUT_L). 00365 * \endcode 00366 */ 00367 #define HTS221_T0_OUT_H_ADDR 0x3D 00368 00369 00370 /** 00371 * @brief Low Calibration Temperature Register (LSB). 00372 * \code 00373 * Read 00374 * Default value: 0x00. 00375 * T1_OUT7 - T1_OUT0: temperature data LSB (2's complement) => signed 16 bits 00376 * RAW LOW Calibration data: T1_OUT (LSB)=(T1_OUT_H & T1_OUT_L). 00377 * \endcode 00378 */ 00379 #define HTS221_T1_OUT_L_ADDR 0x3E 00380 00381 00382 /** 00383 * @brief Low Calibration Temperature Register (MSB) 00384 * \code 00385 * Read 00386 * Default value: 0x00. 00387 * T1_OUT15 - T1_OUT8: temperature data MSB (2's complement) => signed 16 bits 00388 * RAW LOW Calibration data: T1_OUT (LSB)=(T1_OUT_H & T1_OUT_L). 00389 * \endcode 00390 */ 00391 #define HTS221_T1_OUT_H_ADDR 0x3F 00392 00393 00394 /******************************************************************************/ 00395 /**************************** END REGISTER MAPPING ***************************/ 00396 /******************************************************************************/ 00397 00398 00399 /** 00400 * @brief Device Identifier. Default value of the WHO_AM_I register. 00401 */ 00402 #define I_AM_HTS221 ((uint8_t)0xBC) 00403 00404 00405 /** @defgroup HTS221 Power Mode selection - CTRL_REG1 00406 * @{ 00407 */ 00408 #define HTS221_MODE_POWERDOWN ((uint8_t)0x00) 00409 #define HTS221_MODE_ACTIVE ((uint8_t)0x80) 00410 00411 #define HTS221_MODE_MASK ((uint8_t)0x80) 00412 /** 00413 * @} 00414 */ 00415 00416 00417 /** @defgroup HTS221 Block Data Update Mode selection - CTRL_REG1 00418 * @{ 00419 */ 00420 #define HTS221_BDU_CONTINUOUS ((uint8_t)0x00) 00421 #define HTS221_BDU_NOT_UNTIL_READING ((uint8_t)0x04) 00422 00423 #define HTS221_BDU_MASK ((uint8_t)0x04) 00424 /** 00425 * @} 00426 */ 00427 00428 /** @defgroup HTS221 Output Data Rate selection - CTRL_REG1 00429 * @{ 00430 */ 00431 #define HTS221_ODR_ONE_SHOT ((uint8_t)0x00) /*!< Output Data Rate: H - one shot, T - one shot */ 00432 #define HTS221_ODR_1Hz ((uint8_t)0x01) /*!< Output Data Rate: H - 1Hz, T - 1Hz */ 00433 #define HTS221_ODR_7Hz ((uint8_t)0x02) /*!< Output Data Rate: H - 7Hz, T - 7Hz */ 00434 #define HTS221_ODR_12_5Hz ((uint8_t)0x03) /*!< Output Data Rate: H - 12.5Hz, T - 12.5Hz */ 00435 00436 #define HTS221_ODR_MASK ((uint8_t)0x03) 00437 /** 00438 * @} 00439 */ 00440 00441 00442 /** @defgroup HTS221 Boot Mode selection - CTRL_REG2 00443 * @{ 00444 */ 00445 #define HTS221_BOOT_NORMALMODE ((uint8_t)0x00) 00446 #define HTS221_BOOT_REBOOTMEMORY ((uint8_t)0x80) 00447 00448 #define HTS221_BOOT_MASK ((uint8_t)0x80) 00449 /** 00450 * @} 00451 */ 00452 00453 00454 /** @defgroup HTS221 One Shot selection - CTRL_REG2 00455 * @{ 00456 */ 00457 #define HTS221_ONE_SHOT_START ((uint8_t)0x01) 00458 00459 #define HTS221_ONE_SHOT_MASK ((uint8_t)0x01) 00460 /** 00461 * @} 00462 */ 00463 00464 00465 /** @defgroup HTS221 Boot Mode selection - CTRL_REG2 00466 * @{ 00467 */ 00468 #define HTS221_BOOT_NORMALMODE ((uint8_t)0x00) 00469 #define HTS221_BOOT_REBOOTMEMORY ((uint8_t)0x80) 00470 00471 #define HTS221_BOOT_MASK ((uint8_t)0x80) 00472 /** 00473 * @} 00474 */ 00475 00476 00477 /** @defgroup HTS221 PushPull_OpenDrain selection - CTRL_REG3 00478 * @{ 00479 */ 00480 #define HTS221_PP_OD_PUSH_PULL ((uint8_t)0x00) 00481 #define HTS221_PP_OD_OPEN_DRAIN ((uint8_t)0x40) 00482 00483 #define HTS221_PP_OD_MASK ((uint8_t)0x40) 00484 /** 00485 * @} 00486 */ 00487 00488 00489 /** @defgroup HTS221 Data ready selection - CTRL_REG3 00490 * @{ 00491 */ 00492 #define HTS221_DRDY_DISABLE ((uint8_t)0x00) 00493 #define HTS221_DRDY_AVAILABLE ((uint8_t)0x40) 00494 00495 #define HTS221_DRDY_MASK ((uint8_t)0x40) 00496 /** 00497 * @} 00498 */ 00499 00500 00501 /** @defgroup HTS221 Humidity resolution selection - RES_CONF 00502 * @{ 00503 */ 00504 #define HTS221_H_RES_AVG_4 ((uint8_t)0x00) 00505 #define HTS221_H_RES_AVG_8 ((uint8_t)0x01) 00506 #define HTS221_H_RES_AVG_16 ((uint8_t)0x02) 00507 #define HTS221_H_RES_AVG_32 ((uint8_t)0x03) 00508 #define HTS221_H_RES_AVG_64 ((uint8_t)0x04) 00509 #define HTS221_H_RES_AVG_128 ((uint8_t)0x05) 00510 00511 #define HTS221_H_RES_MASK ((uint8_t)0x07) 00512 /** 00513 * @} 00514 */ 00515 00516 00517 /** @defgroup HTS221 Temperature resolution - RES_CONF 00518 * @{ 00519 */ 00520 #define HTS221_T_RES_AVG_2 ((uint8_t)0x00) 00521 #define HTS221_T_RES_AVG_4 ((uint8_t)0x08) 00522 #define HTS221_T_RES_AVG_8 ((uint8_t)0x10) 00523 #define HTS221_T_RES_AVG_16 ((uint8_t)0x18) 00524 #define HTS221_T_RES_AVG_32 ((uint8_t)0x20) 00525 #define HTS221_T_RES_AVG_64 ((uint8_t)0x28) 00526 00527 #define HTS221_T_RES_MASK ((uint8_t)0x38) 00528 /** 00529 * @} 00530 */ 00531 00532 00533 /** @defgroup HTS221 Temperature Humidity data available - STATUS_REG 00534 * @{ 00535 */ 00536 #define HTS221_H_DATA_AVAILABLE_MASK ((uint8_t)0x02) 00537 #define HTS221_T_DATA_AVAILABLE_MASK ((uint8_t)0x01) 00538 /** 00539 * @} 00540 */ 00541 00542 00543 00544 /* Data resolution */ 00545 #define HUM_DECIMAL_DIGITS (2) 00546 #define TEMP_DECIMAL_DIGITS (2) 00547 00548 00549 00550 /** 00551 * @} 00552 */ 00553 00554 00555 00556 /** @defgroup UVIS3_Exported_Functions 00557 * @{ 00558 */ 00559 /* Sensor Configuration Functions */ 00560 void HTS221_Init(HUM_TEMP_InitTypeDef *HTS221_Init); 00561 uint8_t HTS221_ReadID(void); 00562 void HTS221_RebootCmd(void); 00563 void HTS221_Power_OFF(void); 00564 void HTS221_GetHumidity(float* pfData); 00565 void HTS221_GetTemperature(float* pfData); 00566 00567 /* HUM_TEMP sensor driver structure */ 00568 extern HUM_TEMP_DrvTypeDef Hts221Drv; 00569 00570 /* HUM_TEMP sensor IO functions */ 00571 void HUM_TEMP_IO_Init(void); 00572 void HUM_TEMP_IO_Write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t WriteAddr, uint16_t NumByteToWrite); 00573 void HUM_TEMP_IO_Read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, uint16_t NumByteToRead); 00574 00575 /** 00576 * @} 00577 */ 00578 00579 /** 00580 * @} 00581 */ 00582 00583 /** 00584 * @} 00585 */ 00586 00587 #ifdef __cplusplus 00588 } 00589 #endif 00590 00591 #endif /* __HTS221_H */ 00592 00593 00594 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00595
Generated on Tue Jul 12 2022 21:42:00 by
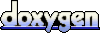