
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
hts221.c
00001 /** 00002 ****************************************************************************** 00003 * @file hts221.c 00004 * @author MEMS Application Team 00005 * @version V1.0.0 00006 * @date 30-July-2014 00007 * @brief This file provides a set of functions needed to manage the hts221. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "hts221.h" 00039 #include <math.h> 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32F439_SENSITRON 00046 * @{ 00047 */ 00048 00049 /** @addtogroup HTS221 00050 * @{ 00051 */ 00052 00053 00054 /** @defgroup HTS221_Private_TypesDefinitions 00055 * @{ 00056 */ 00057 00058 /** 00059 * @} 00060 */ 00061 00062 /** @defgroup HTS221_Private_Defines 00063 * @{ 00064 */ 00065 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup HTS221_Private_Macros 00071 * @{ 00072 */ 00073 00074 /** 00075 * @} 00076 */ 00077 00078 /** @defgroup HTS221_Private_Variables 00079 * @{ 00080 */ 00081 00082 HUM_TEMP_DrvTypeDef Hts221Drv = 00083 { 00084 HTS221_Init, 00085 HTS221_Power_OFF, 00086 HTS221_ReadID, 00087 HTS221_RebootCmd, 00088 0, 00089 0, 00090 0, 00091 0, 00092 0, 00093 HTS221_GetHumidity, 00094 HTS221_GetTemperature 00095 }; 00096 00097 00098 /* Temperature in degree for calibration */ 00099 float T0_degC, T1_degC; 00100 00101 /* Output temperature value for calibration */ 00102 int16_t T0_out, T1_out; 00103 00104 00105 /* Humidity for calibration */ 00106 float H0_rh, H1_rh; 00107 00108 /* Output Humidity value for calibration */ 00109 int16_t H0_T0_out, H1_T0_out; 00110 00111 00112 /** 00113 * @} 00114 */ 00115 00116 /** @defgroup HTS221_Private_FunctionPrototypes 00117 * @{ 00118 */ 00119 static void HTS221_Power_On(void); 00120 00121 static void HTS221_Calibration(void); 00122 /** 00123 * @} 00124 */ 00125 00126 /** @defgroup HTS221_Private_Functions 00127 * @{ 00128 */ 00129 00130 00131 00132 /** 00133 * @brief HTS221 Calibration procedure. 00134 * @param None 00135 * @retval None 00136 */ 00137 static void HTS221_Calibration(void) 00138 { 00139 /* Temperature Calibration */ 00140 /* Temperature in degree for calibration ( "/8" to obtain float) */ 00141 uint16_t T0_degC_x8_L, T0_degC_x8_H, T1_degC_x8_L, T1_degC_x8_H; 00142 uint8_t H0_rh_x2, H1_rh_x2; 00143 uint8_t tempReg[2] = {0,0}; 00144 00145 HUM_TEMP_IO_Read(tempReg, HTS221_ADDRESS, HTS221_T0_degC_X8_ADDR, 1); 00146 T0_degC_x8_L = (uint16_t)tempReg[0]; 00147 00148 HUM_TEMP_IO_Read(tempReg, HTS221_ADDRESS, HTS221_T1_T0_MSB_X8_ADDR, 1); 00149 T0_degC_x8_H = (uint16_t) (tempReg[0] & 0x03); 00150 00151 T0_degC = ((float)((T0_degC_x8_H<<8) | (T0_degC_x8_L)))/8; 00152 00153 HUM_TEMP_IO_Read(tempReg, HTS221_ADDRESS, HTS221_T1_degC_X8_ADDR, 1); 00154 T1_degC_x8_L = (uint16_t)tempReg[0]; 00155 00156 HUM_TEMP_IO_Read(tempReg, HTS221_ADDRESS, HTS221_T1_T0_MSB_X8_ADDR, 1); 00157 T1_degC_x8_H = (uint16_t) (tempReg[0] & 0x0C); 00158 T1_degC_x8_H = T1_degC_x8_H >> 2; 00159 00160 T1_degC = ((float)((T1_degC_x8_H<<8) | (T1_degC_x8_L)))/8; 00161 00162 HUM_TEMP_IO_Read(tempReg, HTS221_ADDRESS, HTS221_T0_OUT_L_ADDR + 0x80, 2); 00163 T0_out = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00164 00165 HUM_TEMP_IO_Read(tempReg, HTS221_ADDRESS, HTS221_T1_OUT_L_ADDR + 0x80, 2); 00166 T1_out = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00167 00168 /* Humidity Calibration */ 00169 /* Humidity in degree for calibration ( "/2" to obtain float) */ 00170 00171 HUM_TEMP_IO_Read(&H0_rh_x2, HTS221_ADDRESS, HTS221_H0_RH_X2_ADDR, 1); 00172 00173 HUM_TEMP_IO_Read(&H1_rh_x2, HTS221_ADDRESS, HTS221_H1_RH_X2_ADDR, 1); 00174 00175 HUM_TEMP_IO_Read(&tempReg[0], HTS221_ADDRESS, HTS221_H0_T0_OUT_L_ADDR + 0x80, 2); 00176 H0_T0_out = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00177 00178 HUM_TEMP_IO_Read(&tempReg[0], HTS221_ADDRESS, HTS221_H1_T0_OUT_L_ADDR + 0x80, 2); 00179 H1_T0_out = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00180 00181 H0_rh = ((float)H0_rh_x2)/2; 00182 H1_rh = ((float)H1_rh_x2)/2; 00183 } 00184 00185 00186 /** 00187 * @brief Set HTS221 Initialization. 00188 * @param InitStruct: it contains the configuration setting for the HTS221. 00189 * @retval None 00190 */ 00191 void HTS221_Init(HUM_TEMP_InitTypeDef *HTS221_Init) 00192 { 00193 uint8_t tmp = 0x00; 00194 00195 /* Configure the low level interface ---------------------------------------*/ 00196 HUM_TEMP_IO_Init(); 00197 00198 HTS221_Power_On(); 00199 00200 HTS221_Calibration(); 00201 00202 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00203 00204 /* Output Data Rate selection */ 00205 tmp &= ~(HTS221_ODR_MASK); 00206 tmp |= HTS221_Init->OutputDataRate; 00207 00208 HUM_TEMP_IO_Write(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00209 } 00210 00211 /** 00212 * @brief Read ID address of HTS221 00213 * @param Device ID address 00214 * @retval ID name 00215 */ 00216 uint8_t HTS221_ReadID(void) 00217 { 00218 uint8_t tmp; 00219 00220 /* Read WHO I AM register */ 00221 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_WHO_AM_I_ADDR, 1); 00222 00223 /* Return the ID */ 00224 return (uint8_t)tmp; 00225 } 00226 00227 /** 00228 * @brief Reboot memory content of HTS221 00229 * @param None 00230 * @retval None 00231 */ 00232 void HTS221_RebootCmd(void) 00233 { 00234 uint8_t tmpreg; 00235 00236 /* Read CTRL_REG2 register */ 00237 HUM_TEMP_IO_Read(&tmpreg, HTS221_ADDRESS, HTS221_CTRL_REG2_ADDR, 1); 00238 00239 /* Enable or Disable the reboot memory */ 00240 tmpreg |= HTS221_BOOT_REBOOTMEMORY; 00241 00242 /* Write value to MEMS CTRL_REG2 regsister */ 00243 HUM_TEMP_IO_Write(&tmpreg, HTS221_ADDRESS, HTS221_CTRL_REG2_ADDR, 1); 00244 } 00245 00246 00247 /** 00248 * @brief Read HTS221 output register, and calculate the humidity. 00249 * @param pfData : Data out pointer 00250 * @retval None 00251 */ 00252 void HTS221_GetHumidity(float* pfData) 00253 { 00254 int16_t H_T_out, humidity_t; 00255 uint8_t tempReg[2] = {0,0}; 00256 uint8_t tmp = 0x00; 00257 float H_rh; 00258 00259 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00260 00261 /* Output Data Rate selection */ 00262 tmp &= (HTS221_ODR_MASK); 00263 00264 if(tmp == 0x00){ 00265 00266 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG2_ADDR, 1); 00267 00268 /* Serial Interface Mode selection */ 00269 tmp &= ~(HTS221_ONE_SHOT_MASK); 00270 tmp |= HTS221_ONE_SHOT_START; 00271 00272 HUM_TEMP_IO_Write(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG2_ADDR, 1); 00273 00274 do{ 00275 00276 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_STATUS_REG_ADDR, 1); 00277 00278 }while(!(tmp&&0x02)); 00279 00280 } 00281 00282 00283 HUM_TEMP_IO_Read(&tempReg[0], HTS221_ADDRESS, HTS221_HUMIDITY_OUT_L_ADDR + 0x80, 2); 00284 H_T_out = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00285 00286 H_rh = ((float)(H_T_out - H0_T0_out))/(H1_T0_out - H0_T0_out) * (H1_rh - H0_rh) + H0_rh; 00287 00288 humidity_t = (uint16_t)(H_rh * pow(10,HUM_DECIMAL_DIGITS)); 00289 00290 *pfData = ((float)humidity_t)/pow(10,HUM_DECIMAL_DIGITS); 00291 } 00292 00293 /** 00294 * @brief Read HTS221 output register, and calculate the temperature. 00295 * @param pfData : Data out pointer 00296 * @retval None 00297 */ 00298 void HTS221_GetTemperature(float* pfData) 00299 { 00300 int16_t T_out, temperature_t; 00301 uint8_t tempReg[2] = {0,0}; 00302 uint8_t tmp = 0x00; 00303 float T_degC; 00304 00305 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00306 00307 /* Output Data Rate selection */ 00308 tmp &= (HTS221_ODR_MASK); 00309 00310 if(tmp == 0x00){ 00311 00312 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG2_ADDR, 1); 00313 00314 /* Serial Interface Mode selection */ 00315 tmp &= ~(HTS221_ONE_SHOT_MASK); 00316 tmp |= HTS221_ONE_SHOT_START; 00317 00318 HUM_TEMP_IO_Write(&tmp, HTS221_ADDRESS, HTS221_CTRL_REG2_ADDR, 1); 00319 00320 do{ 00321 00322 HUM_TEMP_IO_Read(&tmp, HTS221_ADDRESS, HTS221_STATUS_REG_ADDR, 1); 00323 00324 }while(!(tmp&&0x01)); 00325 00326 } 00327 00328 HUM_TEMP_IO_Read(&tempReg[0], HTS221_ADDRESS, HTS221_TEMP_OUT_L_ADDR + 0x80, 2); 00329 T_out = ((((int16_t)tempReg[1]) << 8)+(int16_t)tempReg[0]); 00330 00331 T_degC = ((float)(T_out - T0_out))/(T1_out - T0_out) * (T1_degC - T0_degC) + T0_degC; 00332 00333 temperature_t = (int16_t)(T_degC * pow(10,TEMP_DECIMAL_DIGITS)); 00334 00335 *pfData = ((float)temperature_t)/pow(10,TEMP_DECIMAL_DIGITS); 00336 } 00337 00338 00339 /** 00340 * @brief Exit the shutdown mode for HTS221. 00341 * @retval None 00342 */ 00343 static void HTS221_Power_On() 00344 { 00345 uint8_t tmpReg; 00346 00347 /* Read the register content */ 00348 HUM_TEMP_IO_Read(&tmpReg, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00349 00350 /* Set the power down bit */ 00351 tmpReg |= HTS221_MODE_ACTIVE; 00352 00353 /* Write register */ 00354 HUM_TEMP_IO_Write(&tmpReg, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00355 } 00356 00357 /** 00358 * @brief Enter the shutdown mode for HTS221. 00359 * @retval None 00360 */ 00361 void HTS221_Power_OFF() 00362 { 00363 uint8_t tmpReg; 00364 00365 /* Read the register content */ 00366 HUM_TEMP_IO_Read(&tmpReg, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00367 00368 /* Reset the power down bit */ 00369 tmpReg &= ~(HTS221_MODE_ACTIVE); 00370 00371 /* Write register */ 00372 HUM_TEMP_IO_Write(&tmpReg, HTS221_ADDRESS, HTS221_CTRL_REG1_ADDR, 1); 00373 } 00374 00375 /** 00376 * @} 00377 */ 00378 00379 /** 00380 * @} 00381 */ 00382 00383 /** 00384 * @} 00385 */ 00386 00387 /** 00388 * @} 00389 */ 00390 00391 00392 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00393
Generated on Tue Jul 12 2022 21:42:00 by
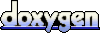