
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
hci.h
00001 /******************** (C) COPYRIGHT 2012 STMicroelectronics ******************** 00002 * File Name : hci.h 00003 * Author : AMS - HEA&RF BU 00004 * Version : V1.0.0 00005 * Date : 19-July-2012 00006 * Description : Constants and functions for HCI layer. See Bluetooth Core 00007 * v 4.0, Vol. 2, Part E. 00008 ******************************************************************************** 00009 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00010 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME. 00011 * AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT, 00012 * INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE 00013 * CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING 00014 * INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00015 *******************************************************************************/ 00016 00017 #ifndef __HCI_H_ 00018 #define __HCI_H_ 00019 00020 #include "hal_types.h" 00021 #include "link_layer.h" 00022 #include <list.h> 00023 00024 00025 #define HCI_PACKET_SIZE 255 00026 00027 00028 /*** Data types ***/ 00029 00030 /* structure used to read received data */ 00031 typedef struct _tHciDataPacket 00032 { 00033 tListNode currentNode; 00034 uint8_t dataBuff[HCI_PACKET_SIZE]; 00035 }tHciDataPacket; 00036 00037 00038 /* HCI Error codes */ 00039 #define HCI_UNKNOWN_COMMAND 0x01 00040 #define HCI_NO_CONNECTION 0x02 00041 #define HCI_HARDWARE_FAILURE 0x03 00042 #define HCI_PAGE_TIMEOUT 0x04 00043 #define HCI_AUTHENTICATION_FAILURE 0x05 00044 #define HCI_PIN_OR_KEY_MISSING 0x06 00045 #define HCI_MEMORY_FULL 0x07 00046 #define HCI_CONNECTION_TIMEOUT 0x08 00047 #define HCI_MAX_NUMBER_OF_CONNECTIONS 0x09 00048 #define HCI_MAX_NUMBER_OF_SCO_CONNECTIONS 0x0a 00049 #define HCI_ACL_CONNECTION_EXISTS 0x0b 00050 #define HCI_COMMAND_DISALLOWED 0x0c 00051 #define HCI_REJECTED_LIMITED_RESOURCES 0x0d 00052 #define HCI_REJECTED_SECURITY 0x0e 00053 #define HCI_REJECTED_PERSONAL 0x0f 00054 #define HCI_HOST_TIMEOUT 0x10 00055 #define HCI_UNSUPPORTED_FEATURE 0x11 00056 #define HCI_INVALID_PARAMETERS 0x12 00057 #define HCI_OE_USER_ENDED_CONNECTION 0x13 00058 #define HCI_OE_LOW_RESOURCES 0x14 00059 #define HCI_OE_POWER_OFF 0x15 00060 #define HCI_CONNECTION_TERMINATED 0x16 00061 #define HCI_REPEATED_ATTEMPTS 0x17 00062 #define HCI_PAIRING_NOT_ALLOWED 0x18 00063 #define HCI_UNKNOWN_LMP_PDU 0x19 00064 #define HCI_UNSUPPORTED_REMOTE_FEATURE 0x1a 00065 #define HCI_SCO_OFFSET_REJECTED 0x1b 00066 #define HCI_SCO_INTERVAL_REJECTED 0x1c 00067 #define HCI_AIR_MODE_REJECTED 0x1d 00068 #define HCI_INVALID_LMP_PARAMETERS 0x1e 00069 #define HCI_UNSPECIFIED_ERROR 0x1f 00070 #define HCI_UNSUPPORTED_LMP_PARAMETER_VALUE 0x20 00071 #define HCI_ROLE_CHANGE_NOT_ALLOWED 0x21 00072 #define HCI_LMP_RESPONSE_TIMEOUT 0x22 00073 #define HCI_LMP_ERROR_TRANSACTION_COLLISION 0x23 00074 #define HCI_LMP_PDU_NOT_ALLOWED 0x24 00075 #define HCI_ENCRYPTION_MODE_NOT_ACCEPTED 0x25 00076 #define HCI_UNIT_LINK_KEY_USED 0x26 00077 #define HCI_QOS_NOT_SUPPORTED 0x27 00078 #define HCI_INSTANT_PASSED 0x28 00079 #define HCI_PAIRING_NOT_SUPPORTED 0x29 00080 #define HCI_TRANSACTION_COLLISION 0x2a 00081 #define HCI_QOS_UNACCEPTABLE_PARAMETER 0x2c 00082 #define HCI_QOS_REJECTED 0x2d 00083 #define HCI_CLASSIFICATION_NOT_SUPPORTED 0x2e 00084 #define HCI_INSUFFICIENT_SECURITY 0x2f 00085 #define HCI_PARAMETER_OUT_OF_RANGE 0x30 00086 #define HCI_ROLE_SWITCH_PENDING 0x32 00087 #define HCI_SLOT_VIOLATION 0x34 00088 #define HCI_ROLE_SWITCH_FAILED 0x35 00089 #define HCI_EIR_TOO_LARGE 0x36 00090 #define HCI_SIMPLE_PAIRING_NOT_SUPPORTED 0x37 00091 #define HCI_HOST_BUSY_PAIRING 0x38 00092 #define HCI_CONN_REJ_NO_CH_FOUND 0x39 00093 #define HCI_CONTROLLER_BUSY 0x3A 00094 #define HCI_UNACCEPTABLE_CONN_INTERV 0x3B 00095 #define HCI_DIRECTED_ADV_TIMEOUT 0x3C 00096 #define HCI_CONN_TERM_MIC_FAIL 0x3D 00097 #define HCI_CONN_FAIL_TO_BE_ESTABL 0x3E 00098 #define HCI_MAC_CONN_FAILED 0x3F 00099 00100 00101 /* 00102 * HCI library functions. 00103 * Each function returns 0 in case of success, -1 otherwise. 00104 */ 00105 00106 int hci_reset(void); 00107 00108 int hci_disconnect(uint16_t handle, uint8_t reason); 00109 00110 int hci_le_set_advertise_enable(tHalUint8 enable); 00111 00112 int hci_le_set_advertising_parameters(uint16_t min_interval, uint16_t max_interval, uint8_t advtype, 00113 uint8_t own_bdaddr_type, uint8_t direct_bdaddr_type, tBDAddr direct_bdaddr, uint8_t chan_map, 00114 uint8_t filter); 00115 00116 int hci_le_set_advertising_data(uint8_t length, const uint8_t data[]); 00117 00118 int hci_le_set_scan_resp_data(uint8_t length, const uint8_t data[]); 00119 00120 int hci_le_rand(uint8_t random_number[8]); 00121 00122 int hci_le_read_advertising_channel_tx_power(int8_t *tx_power_level); 00123 00124 int hci_acl_data(const uint8_t * data, uint16_t len); 00125 00126 int hci_le_set_random_address(tBDAddr bdaddr); 00127 00128 int hci_read_bd_addr(tBDAddr bdaddr); 00129 00130 int hci_le_read_white_list_size(uint8_t *size); 00131 00132 int hci_le_clear_white_list(); 00133 00134 int hci_le_add_device_to_white_list(uint8_t bdaddr_type, tBDAddr bdaddr); 00135 00136 int hci_le_remove_device_from_white_list(uint8_t bdaddr_type, tBDAddr bdaddr); 00137 00138 int hci_le_encrypt(uint8_t key[16], uint8_t plaintextData[16], uint8_t encryptedData[16]); 00139 00140 int hci_le_ltk_request_reply(uint8_t key[16]); 00141 00142 int hci_le_ltk_request_neg_reply(); 00143 00144 int hci_le_read_buffer_size(uint16_t *pkt_len, uint8_t *max_pkt); 00145 00146 int hci_le_create_connection(uint16_t interval, uint16_t window, uint8_t initiator_filter, uint8_t peer_bdaddr_type, 00147 const tBDAddr peer_bdaddr, uint8_t own_bdaddr_type, uint16_t min_interval, uint16_t max_interval, 00148 uint16_t latency, uint16_t supervision_timeout, uint16_t min_ce_length, uint16_t max_ce_length); 00149 00150 int hci_read_transmit_power_level(uint16_t *conn_handle, uint8_t type, int8_t * tx_level); 00151 00152 int hci_read_rssi(uint16_t *conn_handle, int8_t * rssi); 00153 00154 int hci_le_read_local_supported_features(uint8_t *features); 00155 00156 int hci_le_read_channel_map(uint16_t conn_handle, uint8_t ch_map[5]); 00157 00158 int hci_le_read_supported_states(uint8_t states[8]); 00159 00160 int hci_le_receiver_test(uint8_t frequency); 00161 00162 int hci_le_transmitter_test(uint8_t frequency, uint8_t length, uint8_t payload); 00163 00164 int hci_le_test_end(uint16_t *num_pkts); 00165 00166 /** 00167 * This function must be used to pass the packet received from the HCI 00168 * interface to the BLE Stack HCI state machine. 00169 * 00170 * @param[in] hciReadPacket The packet that is received from HCI interface. 00171 * 00172 */ 00173 void HCI_Input(tHciDataPacket * hciReadPacket); 00174 00175 /** 00176 * Initialization function. Must be done before any data can be received from 00177 * BLE controller. 00178 */ 00179 void HCI_Init(void); 00180 00181 /** 00182 * Callback used to pass events to application. 00183 * 00184 * @param[in] pckt The event. 00185 * 00186 */ 00187 extern void HCI_Event_CB(void *pckt); 00188 00189 /** 00190 * Processing function that must be called after an event is received from 00191 * HCI interface. Must be called outside ISR. It will call HCI_Event_CB if 00192 * necessary. 00193 */ 00194 void HCI_Process(void); 00195 00196 00197 extern tListNode hciReadPktPool; 00198 extern tListNode hciReadPktRxQueue; 00199 00200 #endif /* __HCI_H_ */
Generated on Tue Jul 12 2022 21:42:00 by
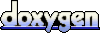