
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
hal.h
00001 /******************** (C) COPYRIGHT 2012 STMicroelectronics ******************** 00002 * File Name : hal.h 00003 * Author : AMS - HEA&RF BU 00004 * Version : V1.0.0 00005 * Date : 19-July-2012 00006 * Description : Header file which defines Hardware abstraction layer APIs 00007 * used by the BLE stack. It defines the set of functions 00008 * which needs to be ported to the target platform. 00009 ******************************************************************************** 00010 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00011 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME. 00012 * AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT, 00013 * INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE 00014 * CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING 00015 * INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00016 *******************************************************************************/ 00017 #ifndef __HAL_H__ 00018 #define __HAL_H__ 00019 00020 /****************************************************************************** 00021 * Includes 00022 *****************************************************************************/ 00023 #include <hal_types.h> 00024 #include <ble_status.h> 00025 00026 /****************************************************************************** 00027 * Macros 00028 *****************************************************************************/ 00029 /* Little Endian buffer to Controller Byte order conversion */ 00030 #define LE_TO_NRG_16(ptr) (uint16) ( ((uint16) \ 00031 *((tHalUint8 *)ptr)) | \ 00032 ((tHalUint16) \ 00033 *((tHalUint8 *)ptr + 1) << 8 ) ) 00034 00035 #define LE_TO_NRG_32(ptr) (tHalUint32) ( ((tHalUint32) \ 00036 *((tHalUint8 *)ptr)) | \ 00037 ((tHalUint32) \ 00038 *((tHalUint8 *)ptr + 1) << 8) | \ 00039 ((tHalUint32) \ 00040 *((tHalUint8 *)ptr + 2) << 16) | \ 00041 ((tHalUint32) \ 00042 *((tHalUint8 *)ptr + 3) << 24) ) 00043 00044 /* Store Value into a buffer in Little Endian Format */ 00045 #define STORE_LE_16(buf, val) ( ((buf)[0] = (tHalUint8) (val) ) , \ 00046 ((buf)[1] = (tHalUint8) (val>>8) ) ) 00047 00048 #define STORE_LE_32(buf, val) ( ((buf)[0] = (tHalUint8) (val) ) , \ 00049 ((buf)[1] = (tHalUint8) (val>>8) ) , \ 00050 ((buf)[2] = (tHalUint8) (val>>16) ) , \ 00051 ((buf)[3] = (tHalUint8) (val>>24) ) ) 00052 00053 /****************************************************************************** 00054 * Types 00055 *****************************************************************************/ 00056 00057 /****************************************************************************** 00058 * Function Prototypes 00059 *****************************************************************************/ 00060 00061 /** 00062 * Writes data to a serial interface. 00063 * 00064 * @param[in] data1 1st buffer 00065 * @param[in] data2 2nd buffer 00066 * @param[in] n_bytes1 number of bytes in 1st buffer 00067 * @param[in] n_bytes2 number of bytes in 2nd buffer 00068 */ 00069 void Hal_Write_Serial(const void* data1, const void* data2, tHalInt32 n_bytes1, tHalInt32 n_bytes2); 00070 00071 /** 00072 * Enable interrupts from HCI controller. 00073 */ 00074 void Enable_SPI_IRQ(void); 00075 00076 /** 00077 * Disable interrupts from BLE controller. 00078 */ 00079 void Disable_SPI_IRQ(void); 00080 00081 00082 00083 00084 #endif /* __HAL_H__ */
Generated on Tue Jul 12 2022 21:41:59 by
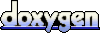