
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
GapAdvertisingData.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GAP_ADVERTISING_DATA_H__ 00018 #define __GAP_ADVERTISING_DATA_H__ 00019 00020 #include "blecommon.h" 00021 00022 #define GAP_ADVERTISING_DATA_MAX_PAYLOAD (31) 00023 00024 /**************************************************************************/ 00025 /*! 00026 \brief 00027 This class provides several helper functions to generate properly 00028 formatted GAP Advertising and Scan Response data payloads 00029 00030 \note 00031 See Bluetooth Specification 4.0 (Vol. 3), Part C, Section 11 and 18 00032 for further information on Advertising and Scan Response data. 00033 00034 \par Advertising and Scan Response Payloads 00035 Advertising data and Scan Response data are organized around a set of 00036 data types called 'AD types' in Bluetooth 4.0 (see the Bluetooth Core 00037 Specification v4.0, Vol. 3, Part C, Sections 11 and 18). 00038 00039 \par 00040 Each AD type has it's own standardized 'assigned number', as defined 00041 by the Bluetooth SIG: 00042 https://www.bluetooth.org/en-us/specification/assigned-numbers/generic-access-profile 00043 00044 \par 00045 For convenience sake, all appropriate AD types have been encapsulated 00046 into GapAdvertisingData::DataType. 00047 00048 \par 00049 Before the AD Types and their payload (if any) can be inserted into 00050 the Advertising or Scan Response frames, they need to be formatted as 00051 follows: 00052 00053 \li \c Record length (1 byte) 00054 \li \c AD Type (1 byte) 00055 \li \c AD payload (optional, only present if record length > 1) 00056 00057 \par 00058 This class takes care of properly formatting the payload, performs 00059 some basic checks on the payload length, and tries to avoid common 00060 errors like adding an exclusive AD field twice in the Advertising 00061 or Scan Response payload. 00062 00063 \par EXAMPLE 00064 00065 \code 00066 00067 // ToDo 00068 00069 \endcode 00070 */ 00071 /**************************************************************************/ 00072 class GapAdvertisingData 00073 { 00074 public: 00075 /**********************************************************************/ 00076 /*! 00077 \brief 00078 A list of Advertising Data types commonly used by peripherals. 00079 These AD types are used to describe the capabilities of the 00080 peripheral, and get inserted inside the advertising or scan 00081 response payloads. 00082 00083 \par Source 00084 \li \c Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 11, 18 00085 \li \c https://www.bluetooth.org/en-us/specification/assigned-numbers/generic-access-profile 00086 */ 00087 /**********************************************************************/ 00088 enum DataType { 00089 FLAGS = 0x01, /**< \ref *Flags */ 00090 INCOMPLETE_LIST_16BIT_SERVICE_IDS = 0x02, /**< Incomplete list of 16-bit Service IDs */ 00091 COMPLETE_LIST_16BIT_SERVICE_IDS = 0x03, /**< Complete list of 16-bit Service IDs */ 00092 INCOMPLETE_LIST_32BIT_SERVICE_IDS = 0x04, /**< Incomplete list of 32-bit Service IDs (not relevant for Bluetooth 4.0) */ 00093 COMPLETE_LIST_32BIT_SERVICE_IDS = 0x05, /**< Complete list of 32-bit Service IDs (not relevant for Bluetooth 4.0) */ 00094 INCOMPLETE_LIST_128BIT_SERVICE_IDS = 0x06, /**< Incomplete list of 128-bit Service IDs */ 00095 COMPLETE_LIST_128BIT_SERVICE_IDS = 0x07, /**< Complete list of 128-bit Service IDs */ 00096 SHORTENED_LOCAL_NAME = 0x08, /**< Shortened Local Name */ 00097 COMPLETE_LOCAL_NAME = 0x09, /**< Complete Local Name */ 00098 TX_POWER_LEVEL = 0x0A, /**< TX Power Level (in dBm) */ 00099 DEVICE_ID = 0x10, /**< Device ID */ 00100 SLAVE_CONNECTION_INTERVAL_RANGE = 0x12, /**< Slave Connection Interval Range */ 00101 SERVICE_DATA = 0x16, /**< Service Data */ 00102 APPEARANCE = 0x19, /**< \ref Appearance */ 00103 ADVERTISING_INTERVAL = 0x1A, /**< Advertising Interval */ 00104 MANUFACTURER_SPECIFIC_DATA = 0xFF /**< Manufacturer Specific Data */ 00105 }; 00106 00107 /**********************************************************************/ 00108 /*! 00109 \brief 00110 A list of values for the FLAGS AD Type 00111 00112 \note 00113 You can use more than one value in the FLAGS AD Type (ex. 00114 LE_GENERAL_DISCOVERABLE and BREDR_NOT_SUPPORTED). 00115 00116 \par Source 00117 \li \c Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 18.1 00118 */ 00119 /**********************************************************************/ 00120 enum Flags { 00121 LE_LIMITED_DISCOVERABLE = 0x01, /**< *Peripheral device is discoverable for a limited period of time */ 00122 LE_GENERAL_DISCOVERABLE = 0x02, /**< Peripheral device is discoverable at any moment */ 00123 BREDR_NOT_SUPPORTED = 0x04, /**< Peripheral device is LE only */ 00124 SIMULTANEOUS_LE_BREDR_C = 0x08, /**< Not relevant - central mode only */ 00125 SIMULTANEOUS_LE_BREDR_H = 0x10 /**< Not relevant - central mode only */ 00126 }; 00127 00128 /**********************************************************************/ 00129 /*! 00130 \brief 00131 A list of values for the APPEARANCE AD Type, which describes the 00132 physical shape or appearance of the device 00133 00134 \par Source 00135 \li \c Bluetooth Core Specification Supplement, Part A, Section 1.12 00136 \li \c Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 12.2 00137 \li \c https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.gap.appearance.xml 00138 */ 00139 /**********************************************************************/ 00140 enum Appearance { 00141 UNKNOWN = 0, /**< Unknown of unspecified appearance type */ 00142 GENERIC_PHONE = 64, /**< Generic Phone */ 00143 GENERIC_COMPUTER = 128, /**< Generic Computer */ 00144 GENERIC_WATCH = 192, /**< Generic Watch */ 00145 WATCH_SPORTS_WATCH = 193, /**< Sports Watch */ 00146 GENERIC_CLOCK = 256, /**< Generic Clock */ 00147 GENERIC_DISPLAY = 320, /**< Generic Display */ 00148 GENERIC_REMOTE_CONTROL = 384, /**< Generic Remote Control */ 00149 GENERIC_EYE_GLASSES = 448, /**< Generic Eye Glasses */ 00150 GENERIC_TAG = 512, /**< Generic Tag */ 00151 GENERIC_KEYRING = 576, /**< Generic Keyring */ 00152 GENERIC_MEDIA_PLAYER = 640, /**< Generic Media Player */ 00153 GENERIC_BARCODE_SCANNER = 704, /**< Generic Barcode Scanner */ 00154 GENERIC_THERMOMETER = 768, /**< Generic Thermometer */ 00155 THERMOMETER_EAR = 769, /**< Ear Thermometer */ 00156 GENERIC_HEART_RATE_SENSOR = 832, /**< Generic Heart Rate Sensor */ 00157 HEART_RATE_SENSOR_HEART_RATE_BELT = 833, /**< Belt Heart Rate Sensor */ 00158 GENERIC_BLOOD_PRESSURE = 896, /**< Generic Blood Pressure */ 00159 BLOOD_PRESSURE_ARM = 897, /**< Arm Blood Pressure */ 00160 BLOOD_PRESSURE_WRIST = 898, /**< Wrist Blood Pressure */ 00161 HUMAN_INTERFACE_DEVICE_HID = 960, /**< Human Interface Device (HID) */ 00162 KEYBOARD = 961, /**< Keyboard */ 00163 MOUSE = 962, /**< Mouse */ 00164 JOYSTICK = 963, /**< Joystick */ 00165 GAMEPAD = 964, /**< Gamepad */ 00166 DIGITIZER_TABLET = 965, /**< Digitizer Tablet */ 00167 CARD_READER = 966, /**< Card Read */ 00168 DIGITAL_PEN = 967, /**< Digital Pen */ 00169 BARCODE_SCANNER = 968, /**< Barcode Scanner */ 00170 GENERIC_GLUCOSE_METER = 1024, /**< Generic Glucose Meter */ 00171 GENERIC_RUNNING_WALKING_SENSOR = 1088, /**< Generic Running/Walking Sensor */ 00172 RUNNING_WALKING_SENSOR_IN_SHOE = 1089, /**< In Shoe Running/Walking Sensor */ 00173 RUNNING_WALKING_SENSOR_ON_SHOE = 1090, /**< On Shoe Running/Walking Sensor */ 00174 RUNNING_WALKING_SENSOR_ON_HIP = 1091, /**< On Hip Running/Walking Sensor */ 00175 GENERIC_CYCLING = 1152, /**< Generic Cycling */ 00176 CYCLING_CYCLING_COMPUTER = 1153, /**< Cycling Computer */ 00177 CYCLING_SPEED_SENSOR = 1154, /**< Cycling Speed Senspr */ 00178 CYCLING_CADENCE_SENSOR = 1155, /**< Cycling Cadence Sensor */ 00179 CYCLING_POWER_SENSOR = 1156, /**< Cycling Power Sensor */ 00180 CYCLING_SPEED_AND_CADENCE_SENSOR = 1157, /**< Cycling Speed and Cadence Sensor */ 00181 PULSE_OXIMETER_GENERIC = 3136, /**< Generic Pulse Oximeter */ 00182 PULSE_OXIMETER_FINGERTIP = 3137, /**< Fingertip Pulse Oximeter */ 00183 PULSE_OXIMETER_WRIST_WORN = 3138, /**< Wrist Worn Pulse Oximeter */ 00184 OUTDOOR_GENERIC = 5184, /**< Generic Outdoor */ 00185 OUTDOOR_LOCATION_DISPLAY_DEVICE = 5185, /**< Outdoor Location Display Device */ 00186 OUTDOOR_LOCATION_AND_NAVIGATION_DISPLAY_DEVICE = 5186, /**< Outdoor Location and Navigation Display Device */ 00187 OUTDOOR_LOCATION_POD = 5187, /**< Outdoor Location Pod */ 00188 OUTDOOR_LOCATION_AND_NAVIGATION_POD = 5188 /**< Outdoor Location and Navigation Pod */ 00189 }; 00190 00191 GapAdvertisingData(void); 00192 virtual ~GapAdvertisingData (void); 00193 00194 ble_error_t addData(DataType, const uint8_t *, uint8_t); 00195 ble_error_t addAppearance(Appearance appearance = GENERIC_TAG); 00196 ble_error_t addFlags(uint8_t flags = LE_GENERAL_DISCOVERABLE); 00197 ble_error_t addTxPower(int8_t txPower); 00198 void clear(void); 00199 const uint8_t *getPayload(void) const; 00200 uint8_t getPayloadLen(void) const; 00201 uint16_t getAppearance(void) const; 00202 00203 private: 00204 uint8_t _payload[GAP_ADVERTISING_DATA_MAX_PAYLOAD]; 00205 uint8_t _payloadLen; 00206 uint16_t _appearance; 00207 }; 00208 00209 #endif // ifndef __GAP_ADVERTISING_DATA_H__
Generated on Tue Jul 12 2022 21:41:59 by
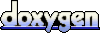