
ble
Dependencies: HC_SR04_Ultrasonic_Library Servo mbed
Fork of FIP_REV1 by
BlueNRGGap.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLUENRG_GAP_H__ 00018 #define __BLUENRG_GAP_H__ 00019 00020 #include "mbed.h" 00021 #include "blecommon.h" 00022 #include "btle.h" 00023 #include "GapAdvertisingParams.h" 00024 #include "GapAdvertisingData.h" 00025 #include "public/Gap.h" 00026 00027 #define BLE_CONN_HANDLE_INVALID 0x0 00028 #define BDADDR_SIZE 6 00029 00030 /**************************************************************************/ 00031 /*! 00032 \brief 00033 00034 */ 00035 /**************************************************************************/ 00036 class BlueNRGGap : public Gap 00037 { 00038 public: 00039 static BlueNRGGap &getInstance() { 00040 static BlueNRGGap m_instance; 00041 return m_instance; 00042 } 00043 00044 /* Functions that must be implemented from Gap */ 00045 virtual ble_error_t setAddress(addr_type_t type, 00046 const uint8_t address[6]); 00047 virtual ble_error_t setAdvertisingData(const GapAdvertisingData &, 00048 const GapAdvertisingData &); 00049 virtual ble_error_t startAdvertising(const GapAdvertisingParams &); 00050 virtual ble_error_t stopAdvertising(void); 00051 virtual ble_error_t disconnect(void); 00052 virtual ble_error_t getPreferredConnectionParams(ConnectionParams_t *params); 00053 virtual ble_error_t setPreferredConnectionParams(const ConnectionParams_t *params); 00054 virtual ble_error_t updateConnectionParams(Handle_t handle, const ConnectionParams_t *params); 00055 00056 void setConnectionHandle(uint16_t con_handle); 00057 uint16_t getConnectionHandle(void); 00058 00059 tHalUint8* getAddress(); 00060 bool getIsSetAddress(); 00061 00062 private: 00063 uint16_t m_connectionHandle; 00064 tHalUint8 bdaddr[BDADDR_SIZE]; 00065 bool isSetAddress; 00066 tBleStatus ret; 00067 00068 //const char local_name[];// = {AD_TYPE_COMPLETE_LOCAL_NAME,'B','l','u','e','N','R','G'}; 00069 //Local Variables 00070 //uint8_t *device_name; 00071 BlueNRGGap() { 00072 m_connectionHandle = BLE_CONN_HANDLE_INVALID; 00073 isSetAddress = false; 00074 //local_name[] = {AD_TYPE_COMPLETE_LOCAL_NAME,'B','l','u','e','N','R','G'}; 00075 00076 } 00077 00078 BlueNRGGap(BlueNRGGap const &); 00079 void operator=(BlueNRGGap const &); 00080 }; 00081 00082 #endif // ifndef __BLUENRG_GAP_H__
Generated on Tue Jul 12 2022 21:41:59 by
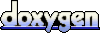