
how to control a servo with temp & humidity
Dependencies: DHT22 Servo mbed
main.cpp
00001 #include "mbed.h" // this tells us to load mbed related functions 00002 #include "Servo.h" // library for the Servo 00003 #include "DHT22.h" // library for the Temp&Humidity sensor 00004 00005 DigitalOut myled(LED1); // used as an output for the sensor 00006 Servo myservo(p10); // p10 works, using other pins is possible too 00007 DHT22 sensor(p6); // the pin of the connected grove port 00008 Ticker fan; 00009 00010 void fanFunction() 00011 { 00012 // YOUR CODE HERE: to reverse myservo between 0 and 1 00013 00014 } 00015 00016 // this code runs when the microcontroller starts up 00017 int main() 00018 { 00019 bool status; 00020 float temp = 0; 00021 float hum = 0; 00022 00023 // spin a main loop all the time 00024 while (1) { 00025 // led hearbeat 00026 myled = !myled; 00027 status = sensor.sample(); 00028 00029 // sensor checksum successful 00030 if (status) { 00031 // printf the temperature and humidity from the sensor 00032 temp = sensor.getTemperature()/10.0f; 00033 hum = sensor.getHumidity()/10.0f; 00034 printf("Temperature is %f C \r\n", temp); 00035 printf("Humidity is %f \r\n", hum); 00036 00037 // start fanning if temperature > 25C or humidity > 90% 00038 if(temp > 25 && hum > 80) { 00039 printf("Starting Fan! \r\n"); 00040 fan.attach(fanFunction,0.5); 00041 } else { 00042 printf("Stopping Fan! \r\n"); 00043 fan.detach(); 00044 } 00045 } else { // sensor checksum failed 00046 printf("Error reading sample \r\n"); 00047 } 00048 wait(2.0f); 00049 } 00050 }
Generated on Mon Jul 18 2022 07:42:50 by
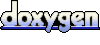