
An example program that drives an RGB LED using PWM outputs
Dependencies: mbed
Fork of Renbed_RGB_PWM by
main.cpp
00001 /********************************************************* 00002 *Renbed_RGB_PWM * 00003 *Author: Dan Argust * 00004 * * 00005 *A program that controls an RGB LED * 00006 *********************************************************/ 00007 00008 /* include the mbed library made by mbed.org that contains 00009 classes/functions designed to make programming mbed 00010 microcontrollers easier */ 00011 #include "mbed.h" 00012 00013 /* Set up 3 pins as pwm out to control the colour 00014 cathodes of the RGB LED */ 00015 PwmOut Red(P1_24); 00016 PwmOut Green(P1_26); 00017 PwmOut Blue(P0_19); 00018 00019 int main() 00020 { 00021 Red = Blue = Green = 0; 00022 float increment = 0.1; 00023 00024 for(;;) 00025 { 00026 for(int i=0;i<10;i++) 00027 { 00028 Green = Green + increment; 00029 wait(increment); 00030 } 00031 for(int i=0;i<10;i++) 00032 { 00033 Red = Red + increment; 00034 wait(increment); 00035 } 00036 for(int i=0;i<10;i++) 00037 { 00038 Green = Green - increment; 00039 wait(increment); 00040 } 00041 for(int i=0;i<10;i++) 00042 { 00043 Blue = Blue + increment; 00044 wait(increment); 00045 } 00046 for(int i=0;i<10;i++) 00047 { 00048 Red = Red - increment; 00049 wait(increment); 00050 } 00051 for(int i=0;i<10;i++) 00052 { 00053 Green = Green + increment; 00054 wait(increment); 00055 } 00056 for(int i=0;i<10;i++) 00057 { 00058 Blue = Blue - increment; 00059 Green = Green - increment; 00060 wait(increment); 00061 } 00062 } 00063 }
Generated on Mon Jul 25 2022 11:25:26 by
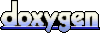