
Uses 2 HC-SR04 ultrasonic modules to steer the RenBuggy away from obstacles. Renishaw Team page fork.
Dependencies: mbed
Fork of RenBuggy_Ultrasonic by
main.cpp
00001 /* 00002 *RenBuggy_Ultrasonic 00003 * 00004 *A Program that allows the RenBuggy to avoid obstacles using two HC-SR04 00005 *ultrasonic modules. 00006 * 00007 *Copyright (c) 2016 Elijah Orr 00008 00009 *Permission is hereby granted, free of charge, to any person obtaining a copy 00010 *of this software and associated documentation files (the "Software"), to deal 00011 *in the Software without restriction, including without limitation the rights 00012 *to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 *copies of the Software, and to permit persons to whom the Software is 00014 *furnished to do so, subject to the following conditions: 00015 00016 *The above copyright notice and this permission notice shall be included in 00017 *all copies or substantial portions of the Software. 00018 00019 *THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 *IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 *FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 *AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 *LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 *OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 *THE SOFTWARE. 00026 */ 00027 00028 //include files - mbed library and our ultrasonic library 00029 #include "mbed.h" 00030 #include "ultrasonic_buggy.h" 00031 00032 //The main function is where the program will begin to execute 00033 int main(){ 00034 00035 //create two floating point vairables to store distance readings in 00036 float left_distance; 00037 float right_distance; 00038 //call the stop function to make sure buggy is stationary 00039 stop(); 00040 //wait for some setup time 00041 wait(5); 00042 //the code in this while loop will always execute and will repeat forever 00043 while(1){ 00044 00045 //take a distance reading from each of the sensors 00046 left_distance = getDistance_l(); 00047 right_distance = getDistance_r(); 00048 00049 //if there is an object on the left, turn right 00050 if(left_distance < 0.4){ 00051 right(0.1); 00052 } 00053 //else if there is an object on the right, turn left 00054 else if(right_distance < 0.4){ 00055 left(0.1); 00056 } 00057 //else move forwards 00058 else{ 00059 forward(0.1); 00060 } 00061 //code loops back to start of while loop 00062 } 00063 } 00064
Generated on Wed Jul 20 2022 03:45:32 by
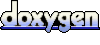