The driver for the ESP32 WiFi module
Embed:
(wiki syntax)
Show/hide line numbers
ESP32InterfaceAP.cpp
00001 /* ESP32 implementation of NetworkInterfaceAPI 00002 * Copyright (c) 2017 Renesas Electronics Corporation 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <string.h> 00018 #include "ESP32InterfaceAP.h" 00019 00020 // ESP32InterfaceAP implementation 00021 ESP32InterfaceAP::ESP32InterfaceAP(PinName en, PinName io0, PinName tx, PinName rx, bool debug, 00022 PinName rts, PinName cts, int baudrate) : 00023 ESP32Stack(en, io0, tx, rx, debug, rts, cts, baudrate), 00024 _dhcp(true), 00025 _own_ch(1), 00026 _own_ssid(), 00027 _own_pass(), 00028 _own_sec(NSAPI_SECURITY_NONE), 00029 _ip_address(), 00030 _netmask(), 00031 _gateway(), 00032 _connection_status(NSAPI_STATUS_DISCONNECTED), 00033 _connection_status_cb(NULL) 00034 { 00035 } 00036 00037 ESP32InterfaceAP::ESP32InterfaceAP(PinName tx, PinName rx, bool debug) : 00038 ESP32Stack(NC, NC, tx, rx, debug, NC, NC, 230400), 00039 _dhcp(true), 00040 _own_ch(1), 00041 _own_ssid(), 00042 _own_pass(), 00043 _own_sec(NSAPI_SECURITY_NONE), 00044 _ip_address(), 00045 _netmask(), 00046 _gateway(), 00047 _connection_status(NSAPI_STATUS_DISCONNECTED), 00048 _connection_status_cb(NULL) 00049 { 00050 } 00051 00052 nsapi_error_t ESP32InterfaceAP::set_network(const char *ip_address, const char *netmask, const char *gateway) 00053 { 00054 _dhcp = false; 00055 00056 strncpy(_ip_address, ip_address ? ip_address : "", sizeof(_ip_address)); 00057 _ip_address[sizeof(_ip_address) - 1] = '\0'; 00058 strncpy(_netmask, netmask ? netmask : "", sizeof(_netmask)); 00059 _netmask[sizeof(_netmask) - 1] = '\0'; 00060 strncpy(_gateway, gateway ? gateway : "", sizeof(_gateway)); 00061 _gateway[sizeof(_gateway) - 1] = '\0'; 00062 00063 return NSAPI_ERROR_OK; 00064 } 00065 00066 nsapi_error_t ESP32InterfaceAP::set_dhcp(bool dhcp) 00067 { 00068 _dhcp = dhcp; 00069 00070 return NSAPI_ERROR_OK; 00071 } 00072 00073 int ESP32InterfaceAP::connect(const char *ssid, const char *pass, nsapi_security_t security, 00074 uint8_t channel) 00075 { 00076 int ret; 00077 00078 ret = set_credentials(ssid, pass, security); 00079 if (ret != 0) { 00080 return ret; 00081 } 00082 00083 ret = set_channel(channel); 00084 if (ret != 0) { 00085 return ret; 00086 } 00087 00088 return connect(); 00089 } 00090 00091 int ESP32InterfaceAP::connect() 00092 { 00093 if (!_esp->set_mode(ESP32::WIFIMODE_STATION_SOFTAP)) { 00094 return NSAPI_ERROR_DEVICE_ERROR; 00095 } 00096 00097 if (!_esp->dhcp(_dhcp, 1)) { 00098 return NSAPI_ERROR_DHCP_FAILURE; 00099 } 00100 00101 if (!_dhcp) { 00102 if (!_esp->set_network_ap(_ip_address, _netmask, _gateway)) { 00103 return NSAPI_ERROR_DEVICE_ERROR; 00104 } 00105 } 00106 00107 if (!_esp->config_soft_ap(_own_ssid, _own_pass, _own_ch, (uint8_t)_own_sec)) { 00108 return NSAPI_ERROR_DEVICE_ERROR; 00109 } 00110 00111 _connection_status = NSAPI_STATUS_GLOBAL_UP; 00112 if (_connection_status_cb) { 00113 _connection_status_cb(NSAPI_EVENT_CONNECTION_STATUS_CHANGE, _connection_status); 00114 } 00115 00116 return NSAPI_ERROR_OK; 00117 } 00118 00119 int ESP32InterfaceAP::set_credentials(const char *ssid, const char *pass, nsapi_security_t security) 00120 { 00121 switch (security) { 00122 case NSAPI_SECURITY_NONE: 00123 case NSAPI_SECURITY_WPA: 00124 case NSAPI_SECURITY_WPA2: 00125 case NSAPI_SECURITY_WPA_WPA2: 00126 _own_sec = security; 00127 break; 00128 case NSAPI_SECURITY_UNKNOWN: 00129 case NSAPI_SECURITY_WEP: 00130 default: 00131 return NSAPI_ERROR_UNSUPPORTED; 00132 } 00133 00134 memset(_own_ssid, 0, sizeof(_own_ssid)); 00135 strncpy(_own_ssid, ssid, sizeof(_own_ssid)); 00136 00137 memset(_own_pass, 0, sizeof(_own_pass)); 00138 strncpy(_own_pass, pass, sizeof(_own_pass)); 00139 00140 return 0; 00141 } 00142 00143 int ESP32InterfaceAP::set_channel(uint8_t channel) 00144 { 00145 if (channel != 0) { 00146 _own_ch = channel; 00147 } 00148 00149 return 0; 00150 } 00151 00152 int ESP32InterfaceAP::disconnect() 00153 { 00154 if (!_esp->set_mode(ESP32::WIFIMODE_STATION)) { 00155 return NSAPI_ERROR_DEVICE_ERROR; 00156 } 00157 00158 _connection_status = NSAPI_STATUS_DISCONNECTED; 00159 if (_connection_status_cb) { 00160 _connection_status_cb(NSAPI_EVENT_CONNECTION_STATUS_CHANGE, _connection_status); 00161 } 00162 00163 return NSAPI_ERROR_OK; 00164 } 00165 00166 const char *ESP32InterfaceAP::get_ip_address() 00167 { 00168 return _esp->getIPAddress_ap(); 00169 } 00170 00171 const char *ESP32InterfaceAP::get_mac_address() 00172 { 00173 return _esp->getMACAddress_ap(); 00174 } 00175 00176 const char *ESP32InterfaceAP::get_gateway() 00177 { 00178 return _esp->getGateway_ap(); 00179 } 00180 00181 const char *ESP32InterfaceAP::get_netmask() 00182 { 00183 return _esp->getNetmask_ap(); 00184 } 00185 00186 int8_t ESP32InterfaceAP::get_rssi() 00187 { 00188 return 0; 00189 } 00190 00191 int ESP32InterfaceAP::scan(WiFiAccessPoint *res, unsigned count) 00192 { 00193 return _esp->scan(res, count); 00194 } 00195 00196 void ESP32InterfaceAP::attach(mbed::Callback<void(nsapi_event_t, intptr_t)> status_cb) 00197 { 00198 _connection_status_cb = status_cb; 00199 } 00200 00201 nsapi_connection_status_t ESP32InterfaceAP::get_connection_status() const 00202 { 00203 return _connection_status; 00204 } 00205
Generated on Wed Jul 13 2022 08:56:28 by
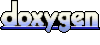