TLV320_RBSP class, defined on the I2C master bus.
Dependents: MovPlayer GR-PEACH_Audio_Playback_Sample GR-PEACH_Audio_Playback_7InchLCD_Sample RGA-MJPEG_VideoDemo ... more
Fork of TLV320_RBSP by
TLV320_RBSP.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #ifndef MBED_TLV320_RBSP_H 00025 #define MBED_TLV320_RBSP_H 00026 00027 #include "mbed.h" 00028 #include "R_BSP_Ssif.h" 00029 00030 /** TLV320_RBSP class, defined on the I2C master bus 00031 * 00032 */ 00033 class TLV320_RBSP { 00034 public: 00035 00036 /** Create a TLV320_RBSP object defined on the I2C port 00037 * 00038 * @param cs Control port input latch/address select (codec pin) 00039 * @param sda I2C data line pin 00040 * @param scl I2C clock line pin 00041 * @param sck SSIF serial bit clock 00042 * @param ws SSIF word selection 00043 * @param tx SSIF serial data output 00044 * @param rx SSIF serial data input 00045 * @param int_level Interupt priority (SSIF) 00046 * @param max_write_num The upper limit of write buffer (SSIF) 00047 * @param max_read_num The upper limit of read buffer (SSIF) 00048 */ 00049 TLV320_RBSP(PinName cs, PinName sda, PinName scl, PinName sck, PinName ws, PinName tx, PinName rx, uint8_t int_level = 0x80, int32_t max_write_num = 16, int32_t max_read_num = 16); 00050 00051 /** Overloaded power() function default = 0x80, record requires 0x02 00052 * 00053 * @param device Call individual devices to power up/down 00054 * Device power 0x00 = On 0x80 = Off 00055 * Clock 0x00 = On 0x40 = Off 00056 * Oscillator 0x00 = On 0x20 = Off 00057 * Outputs 0x00 = On 0x10 = Off 00058 * DAC 0x00 = On 0x08 = Off 00059 * ADC 0x00 = On 0x04 = Off 00060 * Microphone input 0x00 = On 0x02 = Off 00061 * Line input 0x00 = On 0x01 = Off 00062 */ 00063 void power(int device = 0x07); 00064 00065 /** Set I2S interface bit length and mode 00066 * 00067 * @param length Set bit length to 16, 20, 24 or 32 bits 00068 * @return true = success, false = failure 00069 */ 00070 bool format(char length); 00071 00072 /** Set sample frequency 00073 * 00074 * @param frequency Sample frequency of data in Hz 00075 * @return true = success, false = failure 00076 * 00077 * The TLV320 supports the following frequencies: 8kHz, 8.021kHz, 32kHz, 44.1kHz, 48kHz, 88.2kHz, 96kHz 00078 * Default is 44.1kHz 00079 */ 00080 bool frequency(int hz); 00081 00082 /** Reset TLV320 00083 * 00084 */ 00085 void reset(void); 00086 00087 /** Get a value of SSIF channel number 00088 * 00089 * @return SSIF channel number 00090 */ 00091 int32_t GetSsifChNo(void) { 00092 return mI2s_.GetSsifChNo(); 00093 }; 00094 00095 /** Enqueue asynchronous write request 00096 * 00097 * @param p_data Location of the data 00098 * @param data_size Number of bytes to write 00099 * @param p_data_conf Asynchronous control block structure 00100 * @return Number of bytes written on success. negative number on error. 00101 */ 00102 int write(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL) { 00103 return mI2s_.write(p_data, data_size, p_data_conf); 00104 }; 00105 00106 /** Enqueue asynchronous read request 00107 * 00108 * @param p_data Location of the data 00109 * @param data_size Number of bytes to read 00110 * @param p_data_conf Asynchronous control block structure 00111 * @return Number of bytes read on success. negative number on error. 00112 */ 00113 int read(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf = NULL) { 00114 return mI2s_.read(p_data, data_size, p_data_conf); 00115 }; 00116 00117 /** Line in volume control i.e. record volume 00118 * 00119 * @param leftVolumeIn Left line-in volume 00120 * @param rightVolumeIn Right line-in volume 00121 * @return Returns "true" for success, "false" if parameters are out of range 00122 * Parameters accept a value, where 0.0 < parameter < 1.0 and where 0.0 maps to -34.5dB 00123 * and 1.0 maps to +12dB (0.74 = 0 dB default). 00124 */ 00125 bool inputVolume(float leftVolumeIn, float rightVolumeIn); 00126 00127 /** Headphone out volume control 00128 * 00129 * @param leftVolumeOut Left headphone-out volume 00130 * @param rightVolumeOut Right headphone-out volume 00131 * @return Returns "true" for success, "false" if parameters are out of range 00132 * Parameters accept a value, where 0.0 < parameter < 1.0 and where 0.0 maps to -73dB (mute) 00133 * and 1.0 maps to +6dB (0.5 = default) 00134 */ 00135 bool outputVolume(float leftVolumeOut, float rightVolumeOut); 00136 00137 /** Analog audio path control (Bypass) function default = false 00138 * 00139 * @param bypassVar Route analogue audio direct from line in to headphone out 00140 */ 00141 void bypass(bool bypassVar); 00142 00143 /** Analog audio path control (Input select for ADC) function default = false 00144 * 00145 * @param micVar Input select for ADC. true : Microphone , false : Line 00146 */ 00147 void mic(bool micVar); 00148 00149 /** Microphone volume 00150 * 00151 * @param mute Microphone mute. true : mute , false : normal 00152 * @param boost Microphone boost. true : 20dB , false : 0dB 00153 */ 00154 void micVolume(bool mute, bool boost = false); 00155 00156 /** Digital audio path control 00157 * 00158 * @param softMute Mute output 00159 */ 00160 void mute(bool softMute); 00161 00162 protected: 00163 char cmd[2]; // the address and command for TLV320 internal registers 00164 int mAddr; // register write address 00165 private: 00166 DigitalOut audio_cs_; 00167 I2C mI2c_; // MUST use the I2C port 00168 R_BSP_Ssif mI2s_; 00169 ssif_channel_cfg_t ssif_cfg; 00170 char audio_path_control; 00171 /** Digital interface activation 00172 * 00173 */ 00174 void activateDigitalInterface_(void); 00175 00176 // TLV320AIC23B register addresses as defined in the TLV320AIC23B datasheet 00177 #define LEFT_LINE_INPUT_CHANNEL_VOLUME_CONTROL (0x00 << 1) 00178 #define RIGHT_LINE_INPUT_CHANNEL_VOLUME_CONTROL (0x01 << 1) 00179 #define LEFT_CHANNEL_HEADPHONE_VOLUME_CONTROL (0x02 << 1) 00180 #define RIGHT_CHANNEL_HEADPHONE_VOLUME_CONTROL (0x03 << 1) 00181 #define ANALOG_AUDIO_PATH_CONTROL (0x04 << 1) 00182 #define DIGITAL_AUDIO_PATH_CONTROL (0x05 << 1) 00183 #define POWER_DOWN_CONTROL (0x06 << 1) 00184 #define DIGITAL_AUDIO_INTERFACE_FORMAT (0x07 << 1) 00185 #define SAMPLE_RATE_CONTROL (0x08 << 1) 00186 #define DIGITAL_INTERFACE_ACTIVATION (0x09 << 1) 00187 #define RESET_REGISTER (0x0F << 1) 00188 }; 00189 00190 #endif
Generated on Sat Jul 16 2022 00:40:10 by
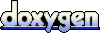