RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
ssif_if.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2013-2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 /****************************************************************************** 00025 * File Name : ssif_if.h 00026 * $Rev: 1032 $ 00027 * $Date:: 2014-08-06 09:04:50 +0900#$ 00028 * Description : SSIF Driver API header 00029 ******************************************************************************/ 00030 00031 #ifndef SSIF_IF_H 00032 #define SSIF_IF_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 00038 #include "cmsis_os.h" 00039 00040 #include "r_typedefs.h" 00041 #include "r_errno.h" 00042 #if(1) /* mbed */ 00043 #include "misratypes.h" 00044 #include "aioif.h" 00045 #include "R_BSP_mbed_fns.h" 00046 #include "R_BSP_SsifDef.h" 00047 #else /* not mbed */ 00048 #include "ioif_public.h" 00049 #endif /* end mbed */ 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 #if(1) /* mbed */ 00056 /****************************************************************************** 00057 Function Prototypes 00058 *****************************************************************************/ 00059 00060 extern RBSP_MBED_FNS* R_SSIF_MakeCbTbl_mbed(void); 00061 #else /* not mbed */ 00062 /****************************************************************************** 00063 Defines 00064 *****************************************************************************/ 00065 00066 /****************************************************************************** 00067 Constant Macros 00068 *****************************************************************************/ 00069 #define SSIF_NUM_CHANS (6u) /**< Number of SSIF channels */ 00070 00071 #define SSIF_CFG_DISABLE_ROMDEC_DIRECT (0x0u) /* Disable SSIRDR->STRMDIN0 route */ 00072 #define SSIF_CFG_ENABLE_ROMDEC_DIRECT (0xDEC0DEC1u) /* Enable SSIRDR->STRMDIN0 route */ 00073 00074 /****************************************************************************** 00075 Function Macros 00076 *****************************************************************************/ 00077 00078 /****************************************************************************** 00079 Enumerated Types 00080 *****************************************************************************/ 00081 00082 /** SSICR:CKS(Clock source for oversampling) */ 00083 typedef enum 00084 { 00085 SSIF_CFG_CKS_AUDIO_X1 = 0, /**< select AUDIO_X1 */ 00086 SSIF_CFG_CKS_AUDIO_CLK = 1 /**< select AUIDIO_CLK */ 00087 } ssif_chcfg_cks_t; 00088 00089 /** SSICR:CHNL(Audio channels per system word) */ 00090 typedef enum 00091 { 00092 SSIF_CFG_MULTI_CH_1 = 0, /**< 1ch within systemword (on tdm=0) */ 00093 SSIF_CFG_MULTI_CH_2 = 1, /**< 2ch within systemword (on tdm=0) */ 00094 SSIF_CFG_MULTI_CH_3 = 2, /**< 3ch within systemword (on tdm=0) */ 00095 SSIF_CFG_MULTI_CH_4 = 3 /**< 4ch within systemword (on tdm=0) */ 00096 } ssif_chcfg_multi_ch_t; 00097 00098 /** SSICR:DWL(Data word length) */ 00099 typedef enum 00100 { 00101 SSIF_CFG_DATA_WORD_8 = 0, /**< Data word length 8 */ 00102 SSIF_CFG_DATA_WORD_16 = 1, /**< Data word length 16 */ 00103 SSIF_CFG_DATA_WORD_18 = 2, /**< Data word length 18 */ 00104 SSIF_CFG_DATA_WORD_20 = 3, /**< Data word length 20 */ 00105 SSIF_CFG_DATA_WORD_22 = 4, /**< Data word length 22 */ 00106 SSIF_CFG_DATA_WORD_24 = 5, /**< Data word length 24 */ 00107 SSIF_CFG_DATA_WORD_32 = 6 /**< Data word length 32 */ 00108 } ssif_chcfg_data_word_t; 00109 00110 /** SSICR:SWL(System word length) */ 00111 typedef enum 00112 { 00113 SSIF_CFG_SYSTEM_WORD_8 = 0, /**< System word length 8 */ 00114 SSIF_CFG_SYSTEM_WORD_16 = 1, /**< System word length 16 */ 00115 SSIF_CFG_SYSTEM_WORD_24 = 2, /**< System word length 24 */ 00116 SSIF_CFG_SYSTEM_WORD_32 = 3, /**< System word length 32 */ 00117 SSIF_CFG_SYSTEM_WORD_48 = 4, /**< System word length 48 */ 00118 SSIF_CFG_SYSTEM_WORD_64 = 5, /**< System word length 64 */ 00119 SSIF_CFG_SYSTEM_WORD_128 = 6, /**< System word length 128 */ 00120 SSIF_CFG_SYSTEM_WORD_256 = 7 /**< System word length 256 */ 00121 } ssif_chcfg_system_word_t; 00122 00123 /** SSICR:SCKP(Clock polarity) */ 00124 typedef enum 00125 { 00126 SSIF_CFG_FALLING = 0, /**< Falling edge */ 00127 SSIF_CFG_RISING = 1 /**< Rising edge */ 00128 } ssif_chcfg_clock_pol_t; 00129 00130 /** SSICR:SWSP(Word select polarity) */ 00131 typedef enum 00132 { 00133 SSIF_CFG_WS_LOW = 0, /**< Low for ther 1st channel(not TDM) */ 00134 SSIF_CFG_WS_HIGH = 1 /**< High for the 1st channel(not TDM) */ 00135 } ssif_chcfg_ws_pol_t; 00136 00137 /** SSICR:SPDP(Serial padding polarity) */ 00138 typedef enum 00139 { 00140 SSIF_CFG_PADDING_LOW = 0, /**< Padding bits are low */ 00141 SSIF_CFG_PADDING_HIGH = 1 /**< Padding bits are high */ 00142 } ssif_chcfg_padding_pol_t; 00143 00144 /** SSICR:SDTA(Serial data alignment) */ 00145 typedef enum 00146 { 00147 SSIF_CFG_DATA_FIRST = 0, /**< Data first */ 00148 SSIF_CFG_PADDING_FIRST = 1 /**< Padding bits first */ 00149 } ssif_chcfg_serial_alignment_t; 00150 00151 /** SSICR:PDTA(Parallel data alignment) */ 00152 typedef enum 00153 { 00154 SSIF_CFG_LEFT = 0, /**< Left aligned */ 00155 SSIF_CFG_RIGHT = 1 /**< Right aligned */ 00156 } ssif_chcfg_parallel_alignment_t; 00157 00158 /** SSICR:DEL(Serial data delay) */ 00159 typedef enum 00160 { 00161 SSIF_CFG_DELAY = 0, /**< 1 clock delay */ 00162 SSIF_CFG_NO_DELAY = 1 /**< No delay */ 00163 } ssif_chcfg_ws_delay_t; 00164 00165 /** SSICR:CKDV(Serial oversampling clock division ratio) */ 00166 typedef enum 00167 { 00168 SSIF_CFG_CKDV_BITS_1 = 0, 00169 SSIF_CFG_CKDV_BITS_2 = 1, 00170 SSIF_CFG_CKDV_BITS_4 = 2, 00171 SSIF_CFG_CKDV_BITS_8 = 3, 00172 SSIF_CFG_CKDV_BITS_16 = 4, 00173 SSIF_CFG_CKDV_BITS_32 = 5, 00174 SSIF_CFG_CKDV_BITS_64 = 6, 00175 SSIF_CFG_CKDV_BITS_128 = 7, 00176 SSIF_CFG_CKDV_BITS_6 = 8, 00177 SSIF_CFG_CKDV_BITS_12 = 9, 00178 SSIF_CFG_CKDV_BITS_24 = 10, 00179 SSIF_CFG_CKDV_BITS_48 = 11, 00180 SSIF_CFG_CKDV_BITS_96 = 12 00181 } ssif_chcfg_ckdv_t; 00182 00183 00184 /** SNCR:SSIxNL(Serial sound interface channel x noise canceler enable) */ 00185 typedef enum 00186 { 00187 SSIF_CFG_DISABLE_NOISE_CANCEL = 0, /**< Not use noise cancel function */ 00188 SSIF_CFG_ENABLE_NOISE_CANCEL = 1 /**< Use noise cancel function */ 00189 } ssif_chcfg_noise_cancel_t; 00190 00191 00192 /** SSITDMR:TDM(TDM mode) */ 00193 typedef enum 00194 { 00195 SSIF_CFG_DISABLE_TDM = 0, /**< not TDM mode */ 00196 SSIF_CFG_ENABLE_TDM = 1 /**< set TDM mode */ 00197 } ssif_chcfg_tdm_t; 00198 00199 /****************************************************************************** 00200 Structures 00201 *****************************************************************************/ 00202 00203 typedef struct 00204 { 00205 uint32_t mode; /* Enable/Disable SSIRDR->STRMDIN0 route */ 00206 void (*p_cbfunc)(void); /* SSIF error callback function */ 00207 } ssif_chcfg_romdec_t; 00208 00209 /**< This structure contains the configuration settings */ 00210 typedef struct 00211 { 00212 bool_t enabled; /* The enable flag for the channel */ 00213 uint8_t int_level; /* Interrupt priority for the channel */ 00214 bool_t slave_mode; /* Mode of operation */ 00215 uint32_t sample_freq; /* Audio Sampling frequency(Hz) */ 00216 ssif_chcfg_cks_t clk_select; /* SSICR-CKS : Audio clock select */ 00217 ssif_chcfg_multi_ch_t multi_ch; /* SSICR-CHNL: Audio channels per system word */ 00218 ssif_chcfg_data_word_t data_word; /* SSICR-DWL : Data word length */ 00219 ssif_chcfg_system_word_t system_word; /* SSICR-SWL : System word length */ 00220 ssif_chcfg_clock_pol_t bclk_pol; /* SSICR-SCKP: Bit Clock polarity */ 00221 ssif_chcfg_ws_pol_t ws_pol; /* SSICR-SWSP: Word Clock polarity */ 00222 ssif_chcfg_padding_pol_t padding_pol; /* SSICR-SPDP: Padding polarity */ 00223 ssif_chcfg_serial_alignment_t serial_alignment; /* SSICR-SDTA: Serial data alignment */ 00224 ssif_chcfg_parallel_alignment_t parallel_alignment; /* SSICR-PDTA: Parallel data alignment */ 00225 ssif_chcfg_ws_delay_t ws_delay; /* SSICR-DEL : Serial clock delay */ 00226 ssif_chcfg_noise_cancel_t noise_cancel; /* GPIO-SNCR : Noise cancel */ 00227 ssif_chcfg_tdm_t tdm_mode; /* SSITDMR-TDM: TDM mode */ 00228 ssif_chcfg_romdec_t romdec_direct; /* DMA : SSIRDR->STRMDIN0 route settings */ 00229 } ssif_channel_cfg_t; 00230 00231 /****************************************************************************** 00232 IOCTLS 00233 *****************************************************************************/ 00234 00235 #define SSIF_CONFIG_CHANNEL (7) 00236 #define SSIF_GET_STATUS (13) 00237 00238 00239 /****************************************************************************** 00240 External Data 00241 *****************************************************************************/ 00242 00243 00244 /****************************************************************************** 00245 Function Prototypes 00246 *****************************************************************************/ 00247 /** 00248 * @ingroup API 00249 * 00250 * This function returns a pointer to the function table of the SSIF driver. 00251 * This is intended to be used as a parameter in the ioif_start_device function. 00252 * 00253 * @retval IOIF_DRV_API* - Pointer to the table of functions supported by the 00254 * driver. 00255 * 00256 */ 00257 extern IOIF_DRV_API *R_SSIF_MakeCbTbl(void); 00258 #endif /* end mbed */ 00259 00260 extern int_t R_SSIF_SWLtoLen(const ssif_chcfg_system_word_t ssicr_swl); 00261 00262 extern int_t R_SSIF_Userdef_InitPinMux(const uint32_t ssif_ch); 00263 extern int_t R_SSIF_Userdef_SetClockDiv(const ssif_channel_cfg_t* const p_ch_cfg, ssif_chcfg_ckdv_t* const p_clk_div); 00264 00265 extern uint16_t R_SSIF_GetVersion(void); 00266 00267 #ifdef __cplusplus 00268 } 00269 #endif 00270 00271 #endif /* SSIF_IF_H */ 00272 /*EOF*/ 00273
Generated on Tue Jul 12 2022 20:43:58 by
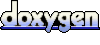