RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
ssif_api.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "ssif_api.h" 00025 00026 static const PinMap PinMap_SSIF_SCK[] = { 00027 // pin ch func 00028 {P2_8 , 0 , 4}, 00029 {P4_4 , 0 , 5}, 00030 {P3_4 , 1 , 3}, 00031 {P7_1 , 1 , 6}, 00032 {P10_12 , 1 , 2}, 00033 {P7_5 , 2 , 6}, 00034 {P9_5 , 2 , 3}, 00035 {P4_12 , 3 , 6}, 00036 {P7_8 , 3 , 2}, 00037 {P7_12 , 4 , 2}, 00038 {P8_12 , 4 , 8}, 00039 {P11_4 , 4 , 3}, 00040 {P2_4 , 5 , 4}, 00041 {P4_8 , 5 , 5}, 00042 {P8_8 , 5 , 8}, 00043 {NC , NC , 0} 00044 }; 00045 00046 static const PinMap PinMap_SSIF_WS[] = { 00047 // pin ch func 00048 {P2_9 , 0 , 4}, 00049 {P4_5 , 0 , 5}, 00050 {P3_5 , 1 , 3}, 00051 {P7_2 , 1 , 6}, 00052 {P10_13 , 1 , 2}, 00053 {P7_6 , 2 , 6}, 00054 {P9_6 , 2 , 3}, 00055 {P4_13 , 3 , 6}, 00056 {P7_9 , 3 , 2}, 00057 {P7_13 , 4 , 2}, 00058 {P8_13 , 4 , 8}, 00059 {P11_5 , 4 , 3}, 00060 {P2_5 , 5 , 4}, 00061 {P4_9 , 5 , 5}, 00062 {P8_9 , 5 , 8}, 00063 {NC , NC , 0} 00064 }; 00065 00066 static const PinMap PinMap_SSIF_TxD[] = { 00067 // pin ch func 00068 {P2_11 , 0 , 4}, 00069 {P4_7 , 0 , 5}, 00070 {P7_4 , 1 , 6}, 00071 {P10_15 , 1 , 2}, 00072 {P7_7 , 2 , 6}, /* SSIDATA2 */ 00073 {P9_7 , 2 , 3}, /* SSIDATA2 */ 00074 {P4_15 , 3 , 6}, 00075 {P7_11 , 3 , 2}, 00076 {P6_1 , 4 , 6}, /* SSIDATA4 */ 00077 {P7_14 , 4 , 2}, /* SSIDATA4 */ 00078 {P8_14 , 4 , 8}, /* SSIDATA4 */ 00079 {P11_6 , 4 , 3}, /* SSIDATA4 */ 00080 {P2_7 , 5 , 4}, 00081 {P4_11 , 5 , 5}, 00082 {P8_10 , 5 , 8}, 00083 {NC , NC , 0} 00084 }; 00085 00086 static const PinMap PinMap_SSIF_RxD[] = { 00087 // pin ch func 00088 {P2_10 , 0 , 4}, 00089 {P4_6 , 0 , 5}, 00090 {P3_7 , 1 , 3}, 00091 {P7_3 , 1 , 6}, 00092 {P10_14 , 1 , 2}, 00093 {P7_7 , 2 , 6}, /* SSIDATA2 */ 00094 {P9_7 , 2 , 3}, /* SSIDATA2 */ 00095 {P4_14 , 3 , 6}, 00096 {P7_10 , 3 , 2}, 00097 {P6_1 , 4 , 6}, /* SSIDATA4 */ 00098 {P7_14 , 4 , 2}, /* SSIDATA4 */ 00099 {P8_14 , 4 , 8}, /* SSIDATA4 */ 00100 {P11_6 , 4 , 3}, /* SSIDATA4 */ 00101 {P2_6 , 5 , 4}, 00102 {P4_10 , 5 , 5}, 00103 {NC , NC , 0} 00104 }; 00105 00106 static void ssif_power_enable(uint32_t ssif_ch) { 00107 volatile uint8_t dummy; 00108 00109 switch (ssif_ch) { 00110 case 0: 00111 CPGSTBCR11 &= ~(0x20); 00112 break; 00113 case 1: 00114 CPGSTBCR11 &= ~(0x10); 00115 break; 00116 case 2: 00117 CPGSTBCR11 &= ~(0x08); 00118 break; 00119 case 3: 00120 CPGSTBCR11 &= ~(0x04); 00121 break; 00122 case 4: 00123 CPGSTBCR11 &= ~(0x02); 00124 break; 00125 case 5: 00126 CPGSTBCR11 &= ~(0x01); 00127 break; 00128 } 00129 dummy = CPGSTBCR11; 00130 } 00131 00132 int32_t ssif_init(PinName sck, PinName ws, PinName tx, PinName rx) { 00133 /* determine the ssif to use */ 00134 uint32_t ssif_sck = pinmap_peripheral(sck, PinMap_SSIF_SCK); 00135 uint32_t ssif_ws = pinmap_peripheral(ws, PinMap_SSIF_WS); 00136 uint32_t ssif_tx = pinmap_peripheral(tx, PinMap_SSIF_TxD); 00137 uint32_t ssif_rx = pinmap_peripheral(rx, PinMap_SSIF_RxD); 00138 uint32_t ssif_ch = pinmap_merge(ssif_tx, ssif_rx); 00139 00140 if ((ssif_ch == ssif_sck) && (ssif_ch == ssif_ws)) { 00141 ssif_power_enable(ssif_ch); 00142 pinmap_pinout(sck, PinMap_SSIF_SCK); 00143 pinmap_pinout(ws, PinMap_SSIF_WS); 00144 pinmap_pinout(tx, PinMap_SSIF_TxD); 00145 pinmap_pinout(rx, PinMap_SSIF_RxD); 00146 } else { 00147 ssif_ch = (uint32_t)NC; 00148 } 00149 00150 return (int32_t)ssif_ch; 00151 } 00152
Generated on Tue Jul 12 2022 20:43:58 by
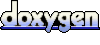