RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
posix_types.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2009,2012 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 /****************************************************************************** 00025 * 00026 * V. 2.03.00 00027 * $Rev: 1560 $ 00028 * $Date:: 2015-03-16 10:39:54 +0900#$ 00029 * 00030 * Description : POSIX types 00031 * 00032 *****************************************************************************/ 00033 00034 #ifndef POSIXTYPES_H_INCLUDED 00035 #define POSIXTYPES_H_INCLUDED 00036 00037 /*********************************************************************************** 00038 System Includes 00039 ***********************************************************************************/ 00040 00041 00042 /*********************************************************************************** 00043 User Includes 00044 ***********************************************************************************/ 00045 00046 00047 /*********************************************************************************** 00048 Defines 00049 ***********************************************************************************/ 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 /* From <unistd.h> */ 00055 #ifndef SEEK_SET 00056 #define SEEK_SET 0 /* from the biginning of file */ 00057 #define SEEK_CUR 1 /* from the current position in file */ 00058 #define SEEK_END 2 /* from the end of file */ 00059 #endif 00060 00061 /* <unistd.h> POSIX Standard: 2.10 file descriptors for stdin, stdout, 00062 stderr not included with SHC. Therefore defined below. */ 00063 #ifndef STDIN_FILENO 00064 #define STDIN_FILENO (0) 00065 #endif 00066 00067 #ifndef STDOUT_FILENO 00068 #define STDOUT_FILENO (1) 00069 #endif 00070 00071 #ifndef STDERR_FILENO 00072 #define STDERR_FILENO (2) 00073 #endif 00074 00075 00076 /* These values must be as defined under open in the compiler manual 00077 * otherwise the buffered file functions fopen() etc. will not work. 00078 */ 00079 #if(1) /* mbed */ 00080 #define O_ACCMODE 7 00081 00082 #if defined(__ARMCC_VERSION) || defined(__ICCARM__) 00083 # define O_RDONLY 0 00084 # define O_WRONLY 1 00085 # define O_RDWR 2 00086 # define O_CREAT 0x0200 00087 # define O_EXCL 0x0300 00088 # define O_TRUNC 0x0400 00089 # define O_APPEND 0x0008 00090 # define O_NONBLOCK 0x4000 00091 # define O_SYNC 0x8000 00092 00093 #else 00094 # include <sys/fcntl.h> 00095 # include <sys/types.h> 00096 # include <sys/syslimits.h> 00097 #endif 00098 00099 #else /* not mbed */ 00100 #define O_ACCMODE 00000007 00101 #define O_RDONLY 00000001 00102 #define O_WRONLY 00000002 00103 #define O_RDWR 00000004 00104 #define O_CREAT 00000010 00105 #define O_EXCL 00000020 00106 #define O_TRUNC 00000040 00107 #define O_APPEND 00002000 00108 #define O_NONBLOCK 00004000 00109 #define O_SYNC 00010000 00110 #endif /* end mbed */ 00111 00112 /*********************************************************************************** 00113 Constant Macros 00114 ***********************************************************************************/ 00115 00116 00117 /*********************************************************************************** 00118 Function Macros 00119 ***********************************************************************************/ 00120 00121 00122 /*********************************************************************************** 00123 Typedefs and Structures 00124 ***********************************************************************************/ 00125 00126 /* The POSIX standard states that:- 00127 mode_t shall be an integer type. 00128 nlink_t, uid_t, gid_t, and id_t shall be integer types. 00129 blkcnt_t and off_t shall be signed integer types. 00130 fsblkcnt_t, fsfilcnt_t, and ino_t shall be defined as unsigned integer types. 00131 size_t shall be an unsigned integer type. 00132 blksize_t, pid_t, and ssize_t shall be signed integer types. 00133 time_t and clock_t shall be integer or real-floating types. 00134 */ 00135 00136 #if(1) /* mbed */ 00137 #ifndef off_t 00138 typedef long off_t; 00139 #endif 00140 #ifndef ssize_t 00141 typedef int ssize_t; 00142 #endif 00143 #else /* not mbed */ 00144 typedef int fsid_t; 00145 typedef long time_t; /* Time in seconds. */ 00146 typedef long long off_t; 00147 typedef unsigned int mode_t; 00148 typedef unsigned int ino_t; 00149 typedef int nlink_t; 00150 typedef int uid_t; 00151 typedef int gid_t; 00152 typedef int dev_t; 00153 typedef int ssize_t; 00154 #endif /* end mbed */ 00155 00156 #if !defined(__cplusplus) && defined(__STRICT_ANSI__) 00157 #if defined (__GNUC__) 00158 typedef unsigned int size_t; 00159 #else /*__GNUC__*/ 00160 typedef unsigned long size_t; 00161 #endif/*__GNUC__*/ 00162 #endif 00163 00164 #ifdef __cplusplus 00165 } 00166 #endif 00167 00168 #endif
Generated on Tue Jul 12 2022 20:43:58 by
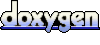