RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
dma_if.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2013 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 /**************************************************************************//** 00025 * @file dma_if.c 00026 * $Rev: 1674 $ 00027 * $Date:: 2015-05-29 16:35:57 +0900#$ 00028 * @brief DMA Driver interface functions 00029 ******************************************************************************/ 00030 00031 /***************************************************************************** 00032 * History : DD.MM.YYYY Version Description 00033 * : 15.01.2013 1.00 First Release 00034 ******************************************************************************/ 00035 00036 /******************************************************************************* 00037 Includes <System Includes>, "Project Includes" 00038 *******************************************************************************/ 00039 00040 #include "dma.h" 00041 00042 /****************************************************************************** 00043 Private global tables 00044 ******************************************************************************/ 00045 00046 /**************************************************************************//** 00047 * Function Name: R_DMA_Init 00048 * @brie Init DMA driver. 00049 * Check parameter in this function. 00050 * 00051 * Description:<br> 00052 * 00053 * @param[in] p_dma_init_param :Point of driver init parameter. 00054 * @param[in,out] p_errno :Pointer of error code. 00055 * When pointer is NULL, it isn't set error code. 00056 * error code - 00057 * OS error num : Registering handler failed. 00058 * EPERM : Pointer of callback function which called in DMA 00059 * error interrupt handler is NULL. 00060 * EFAULT : dma_init_param is NULL. 00061 * @retval ESUCCESS - 00062 * Operation successful. 00063 * EERROR - 00064 * Error occured. 00065 ******************************************************************************/ 00066 00067 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00068 int_t R_DMA_Init(const dma_drv_init_t * const p_dma_init_param, int32_t * const p_errno) 00069 /* <-IPA M1.1.1 */ 00070 { 00071 int_t retval = ESUCCESS; 00072 int_t result_init; 00073 int_t was_masked; 00074 00075 DMA_SetErrCode(ESUCCESS, p_errno); 00076 00077 if (NULL == p_dma_init_param) 00078 { 00079 /* set error return value */ 00080 retval = (EERROR); 00081 DMA_SetErrCode(EFAULT, p_errno); 00082 } 00083 00084 if (ESUCCESS == retval) 00085 { 00086 /* ->MISRA 1.2 It is confirming in advance whether to be NULL or not. */ 00087 if (NULL == p_dma_init_param->p_aio) 00088 /* <-MISRA 1.2 */ 00089 { 00090 /* set error return value */ 00091 retval = (EERROR); 00092 DMA_SetErrCode(EPERM, p_errno); 00093 } 00094 } 00095 00096 /* disable all irq */ 00097 #if defined (__ICCARM__) 00098 was_masked = __disable_irq_iar(); 00099 #else 00100 was_masked = __disable_irq(); 00101 #endif 00102 00103 if (ESUCCESS == retval) 00104 { 00105 result_init = DMA_Initialize(p_dma_init_param); 00106 if (ESUCCESS != result_init) 00107 { 00108 /* set error return value */ 00109 retval = (EERROR); 00110 DMA_SetErrCode(result_init, p_errno); 00111 } 00112 } 00113 00114 if (0 == was_masked) 00115 { 00116 __enable_irq(); 00117 } 00118 00119 return retval; 00120 } 00121 00122 /****************************************************************************** 00123 End of function R_DMA_Init 00124 ******************************************************************************/ 00125 00126 /**************************************************************************//** 00127 * Function Name: R_DMA_UnInit 00128 * @brie UnInit DMA driver. 00129 * Check parameter in this function. 00130 * 00131 * Description:<br> 00132 * 00133 * @param[in,out] p_errno :Pointer of error code 00134 * When pointer is NULL, it isn't set error code. 00135 * error code - 00136 * OS error num : Unegistering handler failed. 00137 * EACCES : Driver status isn't DMA_DRV_INIT. 00138 * EBUSY : It has been allocated already in channel. 00139 * EFAULT : Channel status is besides the status definded in dma_stat_ch_t. 00140 * @retval ESUCCESS - 00141 * Operation successful. 00142 * EERROR - 00143 * Error occured. 00144 ******************************************************************************/ 00145 00146 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00147 int_t R_DMA_UnInit(int32_t * const p_errno) 00148 /* <-IPA M1.1.1 */ 00149 { 00150 int_t retval = ESUCCESS; 00151 int_t result_uninit; 00152 dma_info_drv_t *dma_info_drv; 00153 dma_info_ch_t *dma_info_ch; 00154 int_t ch_count; 00155 bool_t ch_stat_check_flag; 00156 int_t was_masked; 00157 00158 DMA_SetErrCode(ESUCCESS, p_errno); 00159 00160 dma_info_drv = DMA_GetDrvInstance(); 00161 00162 /* disable all irq */ 00163 #if defined (__ICCARM__) 00164 was_masked = __disable_irq_iar(); 00165 #else 00166 was_masked = __disable_irq(); 00167 #endif 00168 00169 /* check driver status */ 00170 if (ESUCCESS == retval) 00171 { 00172 if (DMA_DRV_INIT != dma_info_drv->drv_stat) 00173 { 00174 /* set error return value */ 00175 retval = EERROR; 00176 DMA_SetErrCode(EACCES, p_errno); 00177 } 00178 else 00179 { 00180 ch_stat_check_flag = false; 00181 ch_count = 0; 00182 while (false == ch_stat_check_flag) 00183 { 00184 /* check channel status */ 00185 dma_info_ch = DMA_GetDrvChInfo(ch_count); 00186 if ((DMA_CH_UNINIT != dma_info_ch->ch_stat) && 00187 (DMA_CH_INIT != dma_info_ch->ch_stat)) 00188 { 00189 /* set error return value */ 00190 retval = EERROR; 00191 /* check channel status is busy */ 00192 switch (dma_info_ch->ch_stat) 00193 { 00194 /* These 2 cases are intentionally combined. */ 00195 case DMA_CH_OPEN: 00196 case DMA_CH_TRANSFER: 00197 DMA_SetErrCode(EBUSY, p_errno); 00198 break; 00199 00200 default: 00201 DMA_SetErrCode(EFAULT, p_errno); 00202 break; 00203 } 00204 } 00205 00206 if ((DMA_CH_NUM - 1) == ch_count) 00207 { 00208 /* channel status check end */ 00209 ch_stat_check_flag = true; 00210 } 00211 ch_count++; 00212 } 00213 } 00214 /* uninitialize DMA */ 00215 if (ESUCCESS == retval) 00216 { 00217 result_uninit = DMA_UnInitialize(); 00218 if (ESUCCESS != result_uninit) 00219 { 00220 /* set error return value */ 00221 retval = EERROR; 00222 DMA_SetErrCode(result_uninit, p_errno); 00223 } 00224 } 00225 } 00226 00227 if (0 == was_masked) 00228 { 00229 __enable_irq(); 00230 } 00231 00232 return retval; 00233 } 00234 00235 /****************************************************************************** 00236 End of function R_DMA_UnInit 00237 ******************************************************************************/ 00238 00239 /**************************************************************************//** 00240 * Function Name: R_DMA_Alloc 00241 * @brie Open DMA channel. 00242 * Check parameter in this function mainly. 00243 * 00244 * Description:<br> 00245 * 00246 * @param[in] channel :Open channel number. 00247 * If channel is (-1), it looking for free chanel and allocate. 00248 * @param[in,out] p_errno :Pointer of error code 00249 * When pointer is NULL, it isn't set error code. 00250 * error code - 00251 * EINVAL : Value of the ch is outside the range of DMA_ALLOC_CH(-1) <= ch < DMA_CH_NUM. 00252 * EACCES : Driver status isn't DMA_DRV_INIT. 00253 * EBUSY : It has been allocated already in channel. 00254 * EMFILE : When looking for a free channel, but a free channel didn't exist. 00255 * ENOTSUP : Channel status is DMA_CH_UNINIT. 00256 * EFAULT: Channel status is besides the status definded in dma_stat_ch_t. 00257 * @retval ESUCCESS - 00258 * Operation successful. 00259 * EERROR - 00260 * Error occured. 00261 ******************************************************************************/ 00262 00263 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00264 int_t R_DMA_Alloc(const int_t channel, int32_t * const p_errno) 00265 /* <-IPA M1.1.1 */ 00266 { 00267 int_t retval = ESUCCESS; 00268 int_t ercd = ESUCCESS; 00269 int_t get_ch_num; 00270 dma_info_drv_t *dma_info_drv; 00271 int_t was_masked; 00272 00273 DMA_SetErrCode(ESUCCESS, p_errno); 00274 00275 /* check driver status */ 00276 dma_info_drv = DMA_GetDrvInstance(); 00277 00278 /* disable all irq */ 00279 #if defined (__ICCARM__) 00280 was_masked = __disable_irq_iar(); 00281 #else 00282 was_masked = __disable_irq(); 00283 #endif 00284 00285 if (ESUCCESS == ercd) 00286 { 00287 if (DMA_DRV_INIT != dma_info_drv->drv_stat) 00288 { 00289 /* set error return value */ 00290 ercd = EACCES; 00291 } 00292 else 00293 { 00294 /* check channel of argment */ 00295 if ((DMA_ALLOC_CH <= channel) && (channel < DMA_CH_NUM)) 00296 { 00297 if (DMA_ALLOC_CH == channel) 00298 { 00299 get_ch_num = DMA_GetFreeChannel(); 00300 } 00301 else 00302 { 00303 get_ch_num = DMA_GetFixedChannel(channel); 00304 } 00305 00306 /* check return number or error number */ 00307 if ((DMA_ALLOC_CH < get_ch_num) && (get_ch_num < DMA_CH_NUM)) 00308 { 00309 /* set channel number to return value */ 00310 retval = get_ch_num; 00311 } 00312 else 00313 { 00314 /* set error code to error value */ 00315 ercd = get_ch_num; 00316 } 00317 } 00318 else 00319 { 00320 /* set error return value */ 00321 ercd = EINVAL; 00322 } 00323 } 00324 } 00325 00326 /* occured error check */ 00327 if (ESUCCESS != ercd) 00328 { 00329 retval = EERROR; 00330 DMA_SetErrCode(ercd, p_errno); 00331 } 00332 00333 if (0 == was_masked) 00334 { 00335 __enable_irq(); 00336 } 00337 00338 return retval; 00339 } 00340 00341 /****************************************************************************** 00342 End of function R_DMA_Alloc 00343 ******************************************************************************/ 00344 00345 /**************************************************************************//** 00346 * Function Name: R_DMA_Free 00347 * @brie Close DMA channel. 00348 * Check parameter in this function mainly. 00349 * 00350 * Description:<br> 00351 * 00352 * @param[in] channel :Close channel number. 00353 * @param[in,out] p_errno :Pointer of error code 00354 * When pointer is NULL, it isn't set error code. 00355 * error code - 00356 * EBADF : Channel status is DMA_CH_INIT. 00357 * EINVAL : Value of the ch is outside the range of (-1) < ch < (DMA_CH_NUM + 1). 00358 * EACCES : Driver status isn't DMA_DRV_INIT. 00359 * EBUSY : It has been start DMA transfer in channel. 00360 * ENOTSUP : Channel status is DMA_CH_UNINIT. 00361 * EFAULT: Channel status is besides the status definded in dma_stat_ch_t. 00362 * @retval ESUCCESS - 00363 * Operation successful. 00364 * EERROR - 00365 * Error occured. 00366 ******************************************************************************/ 00367 00368 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00369 int_t R_DMA_Free(const int_t channel, int32_t *const p_errno) 00370 /* <-IPA M1.1.1 */ 00371 { 00372 int_t retval = ESUCCESS; 00373 dma_info_drv_t *dma_info_drv; 00374 dma_info_ch_t *dma_info_ch; 00375 int_t error_code; 00376 int_t was_masked; 00377 00378 DMA_SetErrCode(ESUCCESS, p_errno); 00379 00380 /* disable all irq */ 00381 #if defined (__ICCARM__) 00382 was_masked = __disable_irq_iar(); 00383 #else 00384 was_masked = __disable_irq(); 00385 #endif 00386 00387 /* check channel of argument */ 00388 if ((0 <= channel) && (channel < DMA_CH_NUM)) 00389 { 00390 /* check driver status */ 00391 dma_info_drv = DMA_GetDrvInstance(); 00392 00393 if (ESUCCESS == retval) 00394 { 00395 /* check driver status */ 00396 if (DMA_DRV_INIT == dma_info_drv->drv_stat) 00397 { 00398 dma_info_ch = DMA_GetDrvChInfo(channel); 00399 00400 if (ESUCCESS == retval) 00401 { 00402 if (DMA_CH_OPEN == dma_info_ch->ch_stat) 00403 { 00404 DMA_CloseChannel(channel); 00405 } 00406 else 00407 { 00408 /* set error return value */ 00409 retval = EERROR; 00410 switch (dma_info_ch->ch_stat) 00411 { 00412 case DMA_CH_UNINIT: 00413 error_code = ENOTSUP; 00414 break; 00415 00416 case DMA_CH_INIT: 00417 error_code = EBADF; 00418 break; 00419 00420 case DMA_CH_TRANSFER: 00421 error_code = EBUSY; 00422 break; 00423 00424 default: 00425 error_code = EFAULT; 00426 break; 00427 } 00428 DMA_SetErrCode(error_code, p_errno); 00429 } 00430 } 00431 } 00432 else 00433 { 00434 /* set error return value */ 00435 retval = EERROR; 00436 DMA_SetErrCode(EACCES, p_errno); 00437 00438 } 00439 } 00440 } 00441 else 00442 { 00443 /* set error return value */ 00444 retval = EERROR; 00445 DMA_SetErrCode(EINVAL, p_errno); 00446 } 00447 00448 if (0 == was_masked) 00449 { 00450 __enable_irq(); 00451 } 00452 00453 return retval; 00454 } 00455 00456 /****************************************************************************** 00457 End of function R_DMA_Free 00458 ******************************************************************************/ 00459 00460 /**************************************************************************//** 00461 * Function Name: R_DMA_Setup 00462 * @brie Setup DMA transfer parameter. 00463 * Check parameter in this function mainly. 00464 * 00465 * Description:<br> 00466 * 00467 * @param[in] channel :Setup channel number. 00468 * @param[in] p_ch_setup:Set up parameters. 00469 * @param[in,out] p_errno :Pointer of error code 00470 * When pointer is NULL, it isn't set error code. 00471 * error code - 00472 * EBADF : Channel status is DMA_CH_INIT. 00473 * EINVAL : Value of the ch is outside the range of (-1) < ch < (DMA_CH_NUM + 1). 00474 * EBUSY : It has been start DMA transfer in channel. 00475 * ENOTSUP : Channel status is DMA_CH_UNINIT. 00476 * EPERM : The value in p_ch_setup isn't in the right range. 00477 * EFAULT: Channel status is besides the status definded in dma_stat_ch_t. p_ch_setup is NULL. 00478 * @retval ESUCCESS - 00479 * Operation successful. 00480 * EERROR - 00481 * Error occured. 00482 ******************************************************************************/ 00483 00484 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00485 int_t R_DMA_Setup(const int_t channel, const dma_ch_setup_t * const p_ch_setup, 00486 int32_t * const p_errno) 00487 /* <-IPA M1.1.1 */ 00488 { 00489 int_t retval = ESUCCESS; 00490 dma_info_ch_t *dma_info_ch; 00491 int_t error_code; 00492 uint32_t cfg_table_count; 00493 dma_ch_cfg_t ch_cfg_set_table; 00494 uint32_t set_reqd; 00495 bool_t check_table_flag; 00496 int_t was_masked; 00497 00498 /* Resouce Configure Set Table */ 00499 static const dma_ch_cfg_t ch_cfg_table[DMA_CH_CONFIG_TABLE_NUM] = 00500 { 00501 {DMA_RS_OSTIM0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00502 {DMA_RS_OSTIM1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00503 {DMA_RS_TGI0A, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00504 {DMA_RS_TGI1A, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00505 {DMA_RS_TGI2A, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00506 {DMA_RS_TGI3A, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00507 {DMA_RS_TGI4A, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00508 {DMA_RS_TXI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00509 {DMA_RS_RXI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00510 {DMA_RS_TXI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00511 {DMA_RS_RXI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00512 {DMA_RS_TXI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00513 {DMA_RS_RXI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00514 {DMA_RS_TXI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00515 {DMA_RS_RXI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00516 {DMA_RS_TXI4, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00517 {DMA_RS_RXI4, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00518 {DMA_RS_TXI5, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00519 {DMA_RS_RXI5, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00520 {DMA_RS_TXI6, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00521 {DMA_RS_RXI6, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00522 {DMA_RS_TXI7, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00523 {DMA_RS_RXI7, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00524 {DMA_RS_USB0_DMA0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00525 {DMA_RS_USB0_DMA1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00526 {DMA_RS_USB1_DMA0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00527 {DMA_RS_USB1_DMA1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00528 {DMA_RS_ADEND, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00529 {DMA_RS_IEBBTD, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00530 {DMA_RS_IEBBTV, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00531 {DMA_RS_IREADY, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00532 {DMA_RS_FLDT, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00533 {DMA_RS_SDHI_0T, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00534 {DMA_RS_SDHI_0R, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00535 {DMA_RS_SDHI_1T, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00536 {DMA_RS_SDHI_1R, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00537 {DMA_RS_MMCT, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00538 {DMA_RS_MMCR, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00539 {DMA_RS_SSITXI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00540 {DMA_RS_SSIRXI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00541 {DMA_RS_SSITXI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00542 {DMA_RS_SSIRXI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00543 {DMA_RS_SSIRTI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00544 {DMA_RS_SSITXI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00545 {DMA_RS_SSIRXI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00546 {DMA_RS_SSIRTI4, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00547 {DMA_RS_SSITXI5, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00548 {DMA_RS_SSIRXI5, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00549 {DMA_RS_SCUTXI0, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00550 {DMA_RS_SCURXI0, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00551 {DMA_RS_SCUTXI1, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00552 {DMA_RS_SCURXI1, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00553 {DMA_RS_SCUTXI2, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00554 {DMA_RS_SCURXI2, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00555 {DMA_RS_SCUTXI3, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00556 {DMA_RS_SCURXI3, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00557 {DMA_RS_SPTI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00558 {DMA_RS_SPRI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00559 {DMA_RS_SPTI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00560 {DMA_RS_SPRI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00561 {DMA_RS_SPTI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00562 {DMA_RS_SPRI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00563 {DMA_RS_SPTI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00564 {DMA_RS_SPRI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00565 {DMA_RS_SPTI4, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00566 {DMA_RS_SPRI4, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00567 {DMA_RS_SPDIFTXI, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00568 {DMA_RS_SPDIFRXI, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00569 {DMA_RS_CMI1, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00570 {DMA_RS_CMI2, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00571 {DMA_RS_MLBCI, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_REQD_UNDEFINED}, 00572 {DMA_RS_SGDEI0, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00573 {DMA_RS_SGDEI1, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00574 {DMA_RS_SGDEI2, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00575 {DMA_RS_SGDEI3, CHCFG_SET_AM_LEVEL, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00576 {DMA_RS_SCUTXI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00577 {DMA_RS_SCURXI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00578 {DMA_RS_SCUTXI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00579 {DMA_RS_SCURXI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00580 {DMA_RS_TI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00581 {DMA_RS_RI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00582 {DMA_RS_TI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00583 {DMA_RS_RI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00584 {DMA_RS_TI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00585 {DMA_RS_RI2, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00586 {DMA_RS_TI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00587 {DMA_RS_RI3, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00588 {DMA_RS_LIN0_INT_T, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00589 {DMA_RS_LIN0_INT_R, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00590 {DMA_RS_LIN1_INT_T, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_DST }, 00591 {DMA_RS_LIN1_INT_R, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_EDGE, CHCFG_SET_REQD_SRC }, 00592 {DMA_RS_IFEI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00593 {DMA_RS_OFFI0, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC }, 00594 {DMA_RS_IFEI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_DST }, 00595 {DMA_RS_OFFI1, CHCFG_SET_AM_BUS_CYCLE, CHCFG_SET_LVL_LEVEL, CHCFG_SET_REQD_SRC } 00596 }; 00597 00598 DMA_SetErrCode(ESUCCESS, p_errno); 00599 /* dummy init set_reqd */ 00600 set_reqd = CHCFG_REQD_UNDEFINED; 00601 ch_cfg_set_table = ch_cfg_table[0]; 00602 00603 /* check channel of argument */ 00604 if ((0 <= channel) && (channel < DMA_CH_NUM)) 00605 { 00606 if (NULL != p_ch_setup) 00607 { 00608 /* check setup parameter */ 00609 /* check AIOCB pointer */ 00610 if (NULL == p_ch_setup->p_aio) 00611 { 00612 /* set error return value */ 00613 retval = EERROR; 00614 DMA_SetErrCode(EPERM, p_errno); 00615 } 00616 00617 if (ESUCCESS == retval) 00618 { 00619 /* check DMA transfer unit size for destination */ 00620 if (((int_t)p_ch_setup->dst_width <= DMA_UNIT_MIN) || 00621 ((int_t)p_ch_setup->dst_width >= DMA_UNIT_MAX)) 00622 { 00623 /* set error return value */ 00624 retval = EERROR; 00625 DMA_SetErrCode(EPERM, p_errno); 00626 } 00627 } 00628 00629 if (ESUCCESS == retval) 00630 { 00631 /* check DMA transfer unit size for source */ 00632 if (((int_t)p_ch_setup->src_width <= DMA_UNIT_MIN) || 00633 ((int_t)p_ch_setup->src_width >= DMA_UNIT_MAX)) 00634 { 00635 /* set error return value */ 00636 retval = EERROR; 00637 DMA_SetErrCode(EPERM, p_errno); 00638 } 00639 } 00640 00641 if (ESUCCESS == retval) 00642 { 00643 /* check DMA address count direction for destination */ 00644 if (((int_t)p_ch_setup->dst_cnt <= DMA_ADDR_MIN) || 00645 ((int_t)p_ch_setup->dst_cnt >= DMA_ADDR_MAX)) 00646 { 00647 /* set error return value */ 00648 retval = EERROR; 00649 DMA_SetErrCode(EPERM, p_errno); 00650 } 00651 } 00652 00653 if (ESUCCESS == retval) 00654 { 00655 /* check DMA address count direction for source */ 00656 if (((int_t)(p_ch_setup->src_cnt) <= DMA_ADDR_MIN) || 00657 ((int_t)p_ch_setup->src_cnt >= DMA_ADDR_MAX)) 00658 { 00659 /* set error return value */ 00660 retval = EERROR; 00661 DMA_SetErrCode(EPERM, p_errno); 00662 } 00663 } 00664 00665 if (ESUCCESS == retval) 00666 { 00667 /* check DMA transfer direction */ 00668 if (((int_t)p_ch_setup->direction <= DMA_REQ_MIN) || 00669 ((int_t)p_ch_setup->direction >= DMA_REQ_MAX)) 00670 { 00671 /* set error return value */ 00672 retval = EERROR; 00673 DMA_SetErrCode(EPERM, p_errno); 00674 } 00675 } 00676 00677 if (ESUCCESS == retval) 00678 { 00679 /* check DMA transfer resouce */ 00680 check_table_flag = false; 00681 cfg_table_count = 0; 00682 while (false == check_table_flag) 00683 { 00684 if (p_ch_setup->resource == ch_cfg_table[cfg_table_count].dmars) 00685 { 00686 /* check reqd is undefined */ 00687 if (CHCFG_REQD_UNDEFINED == ch_cfg_table[cfg_table_count].reqd) 00688 { 00689 /* set reqd value on fixed value */ 00690 if (DMA_REQ_SRC == p_ch_setup->direction) 00691 { 00692 set_reqd = CHCFG_SET_REQD_SRC; 00693 } 00694 else 00695 { 00696 set_reqd = CHCFG_SET_REQD_DST; 00697 } 00698 } 00699 else 00700 { 00701 /* set reqd value in channel config table */ 00702 set_reqd = ch_cfg_table[cfg_table_count].reqd; 00703 } 00704 /* set channel config table address for DMA_SetParam() */ 00705 ch_cfg_set_table = ch_cfg_table[cfg_table_count]; 00706 check_table_flag = true; 00707 } 00708 if (false == check_table_flag) 00709 { 00710 /* resource value did not exist in channel config table */ 00711 if ((uint32_t)((sizeof(ch_cfg_table)/sizeof(dma_ch_cfg_t)) - 1U) == cfg_table_count) 00712 { 00713 /* set error return value */ 00714 retval = EERROR; 00715 DMA_SetErrCode(EPERM, p_errno); 00716 check_table_flag = true; 00717 } 00718 cfg_table_count++; 00719 } 00720 } 00721 } 00722 00723 /* disable all irq */ 00724 #if defined (__ICCARM__) 00725 was_masked = __disable_irq_iar(); 00726 #else 00727 was_masked = __disable_irq(); 00728 #endif 00729 00730 if (ESUCCESS == retval) 00731 { 00732 dma_info_ch = DMA_GetDrvChInfo(channel); 00733 00734 if (ESUCCESS == retval) 00735 { 00736 if (DMA_CH_OPEN == dma_info_ch->ch_stat) 00737 { 00738 /* set up parameter */ 00739 DMA_SetParam(channel, p_ch_setup, &ch_cfg_set_table, set_reqd); 00740 } 00741 else 00742 { 00743 /* set error return value */ 00744 retval = EERROR; 00745 switch (dma_info_ch->ch_stat) 00746 { 00747 case DMA_CH_UNINIT: 00748 error_code = ENOTSUP; 00749 break; 00750 00751 case DMA_CH_INIT: 00752 error_code = EBADF; 00753 break; 00754 00755 case DMA_CH_TRANSFER: 00756 error_code = EBUSY; 00757 break; 00758 00759 default: 00760 error_code = EFAULT; 00761 break; 00762 } 00763 DMA_SetErrCode(error_code, p_errno); 00764 } 00765 } 00766 } 00767 00768 if (0 == was_masked) 00769 { 00770 __enable_irq(); 00771 } 00772 } 00773 else 00774 { 00775 /* set error return value */ 00776 retval = EERROR; 00777 DMA_SetErrCode(EFAULT, p_errno); 00778 } 00779 } 00780 else 00781 { 00782 /* set error return value */ 00783 retval = EERROR; 00784 DMA_SetErrCode(EINVAL, p_errno); 00785 } 00786 00787 return retval; 00788 } 00789 00790 /****************************************************************************** 00791 End of function R_DMA_SetParam 00792 ******************************************************************************/ 00793 00794 /**************************************************************************//** 00795 * Function Name: R_DMA_Start 00796 * @brie Start DMA transfer. 00797 * Check parameter in this function mainly. 00798 * 00799 * Description:<br> 00800 * 00801 * @param[in] channel :DMA start channel number. 00802 * @param[in] p_dma_data:DMA address parameters. 00803 * @param[in,out] p_errno :Pointer of error code 00804 * When pointer is NULL, it isn't set error code. 00805 * error code - 00806 * EBADF : Channel status is DMA_CH_INIT. 00807 * EINVAL : Value of the ch is outside the range of (-1) < ch < (DMA_CH_NUM + 1). 00808 * EBUSY : It has been start DMA transfer in channel. 00809 * ENOTSUP : Channel status is DMA_CH_UNINIT. 00810 * EPERM : The value in p_ch_setup isn't in the right range. 00811 * EFAULT: Channel status is besides the status definded in dma_stat_ch_t. p_dma_data is NULL. 00812 * @retval ESUCCESS - 00813 * Operation successful. 00814 * EERROR - 00815 * Error occured. 00816 ******************************************************************************/ 00817 00818 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00819 int_t R_DMA_Start(const int_t channel, const dma_trans_data_t * const p_dma_data, 00820 int32_t * const p_errno) 00821 /* <-IPA M1.1.1 */ 00822 { 00823 int_t retval = ESUCCESS; 00824 dma_info_ch_t *dma_info_ch; 00825 int_t error_code; 00826 int_t was_masked; 00827 00828 DMA_SetErrCode(ESUCCESS, p_errno); 00829 00830 /* disable all irq */ 00831 #if defined (__ICCARM__) 00832 was_masked = __disable_irq_iar(); 00833 #else 00834 was_masked = __disable_irq(); 00835 #endif 00836 00837 /* check channel of argument */ 00838 if ((0 <= channel) && (channel < DMA_CH_NUM)) 00839 { 00840 if (NULL != p_dma_data) 00841 { 00842 /* check address parameter */ 00843 /* check DMA transfer count destination address is 0 */ 00844 if (0U == p_dma_data->count) 00845 { 00846 /* set error return value */ 00847 retval = EERROR; 00848 DMA_SetErrCode(EPERM, p_errno); 00849 } 00850 00851 if (ESUCCESS == retval) 00852 { 00853 dma_info_ch = DMA_GetDrvChInfo(channel); 00854 00855 if (ESUCCESS == retval) 00856 { 00857 if (DMA_CH_OPEN == dma_info_ch->ch_stat) 00858 { 00859 /* set bus paramter for DMA */ 00860 DMA_BusParam(channel, p_dma_data); 00861 /* set up address parameter */ 00862 /* Next register set is 0 */ 00863 DMA_SetData(channel, p_dma_data, 0); 00864 /* DMA transfer start */ 00865 DMA_Start(channel, false); 00866 } 00867 else 00868 { 00869 /* set error return value */ 00870 retval = EERROR; 00871 switch (dma_info_ch->ch_stat) 00872 { 00873 case DMA_CH_UNINIT: 00874 error_code = ENOTSUP; 00875 break; 00876 00877 case DMA_CH_INIT: 00878 error_code = EBADF; 00879 break; 00880 00881 case DMA_CH_TRANSFER: 00882 error_code = EBUSY; 00883 break; 00884 00885 default: 00886 error_code = EFAULT; 00887 break; 00888 } 00889 DMA_SetErrCode(error_code, p_errno); 00890 } 00891 } 00892 } 00893 } 00894 else 00895 { 00896 /* set error return value */ 00897 retval = EERROR; 00898 DMA_SetErrCode(EFAULT, p_errno); 00899 } 00900 } 00901 else 00902 { 00903 /* set error return value */ 00904 retval = EERROR; 00905 DMA_SetErrCode(EINVAL, p_errno); 00906 } 00907 00908 if (0 == was_masked) 00909 { 00910 __enable_irq(); 00911 } 00912 00913 return retval; 00914 } 00915 00916 /****************************************************************************** 00917 End of function R_DMA_Start 00918 ******************************************************************************/ 00919 00920 /**************************************************************************//** 00921 * Function Name: R_DMA_NextData 00922 * @brie Set continous DMA mode. 00923 * Check parameter in this function mainly. 00924 * 00925 * Description:<br> 00926 * 00927 * @param[in] channel :Continuous DMA channel number. 00928 * @param[in,out] p_dma_data:DMA address parameters. 00929 * @param[in,out] p_errno :Pointer of error code 00930 * When pointer is NULL, it isn't set error code. 00931 * error code - 00932 * EBADF : Channel status is DMA_CH_INIT. 00933 * EINVAL : Value of the ch is outside the range of (-1) < ch < (DMA_CH_NUM + 1). 00934 * EBUSY : It has been set continous DMA transfer. 00935 * ENOTSUP : Channel status is DMA_CH_UNINIT. 00936 * EPERM : The value in p_ch_setup isn't in the right range. 00937 * EFAULT: Channel status is besides the status definded in dma_stat_ch_t. p_dma_data is NULL. 00938 * @retval ESUCCESS - 00939 * Operation successful. 00940 * EERROR - 00941 * Error occured. 00942 ******************************************************************************/ 00943 00944 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 00945 int_t R_DMA_NextData(const int_t channel, const dma_trans_data_t * const p_dma_data, 00946 int32_t * const p_errno) 00947 /* <-IPA M1.1.1 */ 00948 { 00949 int_t retval = ESUCCESS; 00950 dma_info_ch_t *dma_info_ch; 00951 int_t error_code; 00952 int_t was_masked; 00953 00954 DMA_SetErrCode(ESUCCESS, p_errno); 00955 00956 /* disable all irq */ 00957 #if defined (__ICCARM__) 00958 was_masked = __disable_irq_iar(); 00959 #else 00960 was_masked = __disable_irq(); 00961 #endif 00962 00963 /* check channel of argument */ 00964 if ((0 <= channel) && (channel < DMA_CH_NUM)) 00965 { 00966 if (NULL != p_dma_data) 00967 { 00968 /* check address parameter */ 00969 /* check DMA transfer count destination address is 0 */ 00970 if (0U == p_dma_data->count) 00971 { 00972 /* set error return value */ 00973 retval = EERROR; 00974 DMA_SetErrCode(EPERM, p_errno); 00975 } 00976 00977 if (ESUCCESS == retval) 00978 { 00979 dma_info_ch = DMA_GetDrvChInfo(channel); 00980 00981 if (ESUCCESS == retval) 00982 { 00983 if ((DMA_CH_OPEN == dma_info_ch->ch_stat) || 00984 (DMA_CH_TRANSFER == dma_info_ch->ch_stat)) 00985 { 00986 if (false == dma_info_ch->next_dma_flag) 00987 { 00988 /* set up address parameter for continous DMA*/ 00989 DMA_SetNextData(channel, p_dma_data); 00990 } 00991 else 00992 { 00993 /* set error return value */ 00994 retval = EERROR; 00995 DMA_SetErrCode(EBUSY, p_errno); 00996 } 00997 } 00998 else 00999 { 01000 /* set error return value */ 01001 retval = EERROR; 01002 switch (dma_info_ch->ch_stat) 01003 { 01004 case DMA_CH_UNINIT: 01005 error_code = ENOTSUP; 01006 break; 01007 01008 case DMA_CH_INIT: 01009 error_code = EBADF; 01010 break; 01011 01012 default: 01013 error_code = EFAULT; 01014 break; 01015 } 01016 DMA_SetErrCode(error_code, p_errno); 01017 } 01018 } 01019 } 01020 } 01021 else 01022 { 01023 /* set error return value */ 01024 retval = EERROR; 01025 DMA_SetErrCode(EFAULT, p_errno); 01026 } 01027 } 01028 else 01029 { 01030 /* set error return value */ 01031 retval = EERROR; 01032 DMA_SetErrCode(EINVAL, p_errno); 01033 } 01034 01035 if (0 == was_masked) 01036 { 01037 __enable_irq(); 01038 } 01039 01040 return retval; 01041 } 01042 01043 /****************************************************************************** 01044 End of function R_DMA_NextData 01045 ******************************************************************************/ 01046 01047 /**************************************************************************//** 01048 * Function Name: R_DMA_Cancel 01049 * @brie Cancel DMA transfer. 01050 * Check parameter in this function mainly. 01051 * 01052 * Description:<br> 01053 * 01054 * @param[in] channel :Cancel DMA channel number. 01055 * @param[in] p_remain :Remain data size of DMA transfer when it stopping. 01056 * @param[in,out] p_errno :Pointer of error code 01057 * When pointer is NULL, it isn't set error code. 01058 * error code - 01059 * EBADF : Channel status is DMA_CH_INIT or DMA_CH_OPEN. (DMA stopped) 01060 * EINVAL : Value of the ch is outside the range of (-1) < ch < (DMA_CH_NUM + 1). 01061 * ENOTSUP : Channel status is DMA_CH_UNINIT. 01062 * EFAULT: Channel status is besides the status definded in dma_stat_ch_t. p_remain is NULL. 01063 * @retval ESUCCESS - 01064 * Operation successful. 01065 * EERROR - 01066 * Error occured. 01067 ******************************************************************************/ 01068 01069 /* ->IPA M1.1.1 If this function is the whole system, it will be called. */ 01070 int_t R_DMA_Cancel(const int_t channel, uint32_t * const p_remain, int32_t * const p_errno) 01071 /* <-IPA M1.1.1 */ 01072 { 01073 int_t retval = ESUCCESS; 01074 dma_info_ch_t *dma_info_ch; 01075 int_t error_code; 01076 int_t was_masked; 01077 01078 DMA_SetErrCode(ESUCCESS, p_errno); 01079 01080 /* disable all irq */ 01081 #if defined (__ICCARM__) 01082 was_masked = __disable_irq_iar(); 01083 #else 01084 was_masked = __disable_irq(); 01085 #endif 01086 01087 /* check channel of argument */ 01088 if ((0 <= channel) && (channel < DMA_CH_NUM)) 01089 { 01090 /* check whether p_remain is NULL */ 01091 if (NULL != p_remain) 01092 { 01093 dma_info_ch = DMA_GetDrvChInfo(channel); 01094 01095 if (ESUCCESS == retval) 01096 { 01097 if (DMA_CH_TRANSFER == dma_info_ch->ch_stat) 01098 { 01099 /* set up address parameter for continous DMA*/ 01100 DMA_Stop(channel, p_remain); 01101 } 01102 else 01103 { 01104 /* set error return value */ 01105 retval = EERROR; 01106 switch (dma_info_ch->ch_stat) 01107 { 01108 case DMA_CH_UNINIT: 01109 error_code = ENOTSUP; 01110 break; 01111 01112 case DMA_CH_INIT: 01113 error_code = EBADF; 01114 break; 01115 01116 case DMA_CH_OPEN: 01117 error_code = EBADF; 01118 break; 01119 01120 default: 01121 error_code = EFAULT; 01122 break; 01123 } 01124 DMA_SetErrCode(error_code, p_errno); 01125 } 01126 } 01127 } 01128 else 01129 { 01130 /* set error return value */ 01131 retval = EERROR; 01132 DMA_SetErrCode(EFAULT, p_errno); 01133 } 01134 } 01135 else 01136 { 01137 /* set error return value */ 01138 retval = EERROR; 01139 DMA_SetErrCode(EINVAL, p_errno); 01140 } 01141 01142 if (0 == was_masked) 01143 { 01144 __enable_irq(); 01145 } 01146 01147 return retval; 01148 } 01149 01150 /****************************************************************************** 01151 End of function R_DMA_Cancel 01152 ******************************************************************************/ 01153
Generated on Tue Jul 12 2022 20:43:58 by
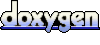