RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
dma.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2013 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 /**************************************************************************//** 00025 * @file dma.c 00026 * $Rev: 1674 $ 00027 * $Date:: 2015-05-29 16:35:57 +0900#$ 00028 * @brief DMA Driver internal functions 00029 ******************************************************************************/ 00030 00031 /***************************************************************************** 00032 * History : DD.MM.YYYY Version Description 00033 * : 15.01.2013 1.00 First Release 00034 ******************************************************************************/ 00035 00036 /******************************************************************************* 00037 Includes <System Includes>, "Project Includes" 00038 *******************************************************************************/ 00039 00040 #include "dma.h" 00041 #include "aioif.h" 00042 #include "iodefine.h" 00043 #include "gic.h" 00044 00045 /****************************************************************************** 00046 Private global driver management information 00047 ******************************************************************************/ 00048 00049 /* driver management infrmation */ 00050 static dma_info_drv_t gb_info_drv; 00051 00052 /****************************************************************************** 00053 Private function define (interrupt handler) 00054 ******************************************************************************/ 00055 00056 static void R_DMA_End0InterruptHandler(void); 00057 static void R_DMA_End1InterruptHandler(void); 00058 static void R_DMA_End2InterruptHandler(void); 00059 static void R_DMA_End3InterruptHandler(void); 00060 static void R_DMA_End4InterruptHandler(void); 00061 static void R_DMA_End5InterruptHandler(void); 00062 static void R_DMA_End6InterruptHandler(void); 00063 static void R_DMA_End7InterruptHandler(void); 00064 static void R_DMA_End8InterruptHandler(void); 00065 static void R_DMA_End9InterruptHandler(void); 00066 static void R_DMA_End10InterruptHandler(void); 00067 static void R_DMA_End11InterruptHandler(void); 00068 static void R_DMA_End12InterruptHandler(void); 00069 static void R_DMA_End13InterruptHandler(void); 00070 static void R_DMA_End14InterruptHandler(void); 00071 static void R_DMA_End15InterruptHandler(void); 00072 static void R_DMA_ErrInterruptHandler(void); 00073 static void R_DMA_EndHandlerProcess(const int_t channel); 00074 00075 /****************************************************************************** 00076 Function prototypes 00077 *****************************************************************************/ 00078 00079 static void DMA_OpenChannel(const int_t channel); 00080 00081 /****************************************************************************** 00082 * Function Name: DMA_GetDrvInstance 00083 * Description : Get pointer of gb_info_drv. 00084 * Arguments : *p_dma_info_drv - 00085 * Pointer of gb_info_drv is returned. 00086 * Return Value : None 00087 ******************************************************************************/ 00088 00089 dma_info_drv_t *DMA_GetDrvInstance(void) 00090 { 00091 00092 return &gb_info_drv; 00093 } 00094 00095 /****************************************************************************** 00096 End of function DMA_GetDrv_Instance 00097 ******************************************************************************/ 00098 00099 /****************************************************************************** 00100 * Function Name: DMA_GetDrvChInfo 00101 * Description : Get pointer of gb_info_drv.info_ch[channel]. 00102 * Arguments : *p_dma_info_drv - 00103 * Pointer of gb_info_drv is returned. 00104 * Return Value : None 00105 ******************************************************************************/ 00106 00107 dma_info_ch_t *DMA_GetDrvChInfo(const int_t channel) 00108 { 00109 00110 return &gb_info_drv.info_ch[channel]; 00111 } 00112 00113 /****************************************************************************** 00114 End of function DMA_GetDrvChInfo 00115 ******************************************************************************/ 00116 00117 /****************************************************************************** 00118 * Function Name: DMA_Initialize 00119 * Description : Initialize DMA driver. 00120 * Arguments : *p_dma_init_param - 00121 * Pointer of init parameters. 00122 * Return Value : ESUCCESS - 00123 * Operation successful. 00124 * OS error num - 00125 * Registering handler failed. 00126 ******************************************************************************/ 00127 00128 int_t DMA_Initialize(const dma_drv_init_t * const p_dma_init_param) 00129 { 00130 int_t retval = ESUCCESS; 00131 int_t ch_count; 00132 uint32_t error_code; 00133 bool_t init_check_flag; 00134 00135 /* ->MISRA 11.3, 11.4, IPA R3.6.2 This cast is needed for register access. */ 00136 /* address table of register set for each channel */ 00137 static volatile struct st_dmac_n *gb_dma_ch_register_addr_table[DMA_CH_NUM] = 00138 { &DMAC0, 00139 &DMAC1, 00140 &DMAC2, 00141 &DMAC3, 00142 &DMAC4, 00143 &DMAC5, 00144 &DMAC6, 00145 &DMAC7, 00146 &DMAC8, 00147 &DMAC9, 00148 &DMAC10, 00149 &DMAC11, 00150 &DMAC12, 00151 &DMAC13, 00152 &DMAC14, 00153 &DMAC15 00154 }; 00155 /* <-MISRA 11.3, 11.4, IPA R3.6.2*/ 00156 00157 /* ->MISRA 11.3, 11.4, IPA R3.6.2 This cast is needed for register access. */ 00158 /* address table of register set for common register */ 00159 static volatile struct st_dmaccommon_n *gb_dma_common_register_addr_table[DMA_CH_NUM] = 00160 { &DMAC07, 00161 &DMAC07, 00162 &DMAC07, 00163 &DMAC07, 00164 &DMAC07, 00165 &DMAC07, 00166 &DMAC07, 00167 &DMAC07, 00168 &DMAC815, 00169 &DMAC815, 00170 &DMAC815, 00171 &DMAC815, 00172 &DMAC815, 00173 &DMAC815, 00174 &DMAC815, 00175 &DMAC815 00176 }; 00177 /* <-MISRA 11.3, 11.4, IPA R3.6.2*/ 00178 00179 /* ->MISRA 11.3, 11.4 This cast is needed for register access. */ 00180 /* address table of register set for DMARS */ 00181 static volatile uint32_t *gb_dmars_register_addr_table[DMA_CH_NUM] = 00182 { &DMACDMARS0, 00183 &DMACDMARS0, 00184 &DMACDMARS1, 00185 &DMACDMARS1, 00186 &DMACDMARS2, 00187 &DMACDMARS2, 00188 &DMACDMARS3, 00189 &DMACDMARS3, 00190 &DMACDMARS4, 00191 &DMACDMARS4, 00192 &DMACDMARS5, 00193 &DMACDMARS5, 00194 &DMACDMARS6, 00195 &DMACDMARS6, 00196 &DMACDMARS7, 00197 &DMACDMARS7 00198 }; 00199 /* <-MISRA 11.3, 11.4 */ 00200 00201 /* Interrpt handlers table */ 00202 static const IRQHandler gb_dma_int_handler_table[DMA_CH_NUM] = 00203 { &R_DMA_End0InterruptHandler, /* DMA end interrupt for ch0 - ch15 */ 00204 &R_DMA_End1InterruptHandler, 00205 &R_DMA_End2InterruptHandler, 00206 &R_DMA_End3InterruptHandler, 00207 &R_DMA_End4InterruptHandler, 00208 &R_DMA_End5InterruptHandler, 00209 &R_DMA_End6InterruptHandler, 00210 &R_DMA_End7InterruptHandler, 00211 &R_DMA_End8InterruptHandler, 00212 &R_DMA_End9InterruptHandler, 00213 &R_DMA_End10InterruptHandler, 00214 &R_DMA_End11InterruptHandler, 00215 &R_DMA_End12InterruptHandler, 00216 &R_DMA_End13InterruptHandler, 00217 &R_DMA_End14InterruptHandler, 00218 &R_DMA_End15InterruptHandler 00219 }; 00220 00221 /* Interrupt numbers table */ 00222 static const IRQn_Type gb_dma_int_num_table[DMA_CH_NUM] = 00223 { DMAINT0_IRQn, /* DMA end interrupt for ch0 - ch15 */ 00224 DMAINT1_IRQn, 00225 DMAINT2_IRQn, 00226 DMAINT3_IRQn, 00227 DMAINT4_IRQn, 00228 DMAINT5_IRQn, 00229 DMAINT6_IRQn, 00230 DMAINT7_IRQn, 00231 DMAINT8_IRQn, 00232 DMAINT9_IRQn, 00233 DMAINT10_IRQn, 00234 DMAINT11_IRQn, 00235 DMAINT12_IRQn, 00236 DMAINT13_IRQn, 00237 DMAINT14_IRQn, 00238 DMAINT15_IRQn 00239 }; 00240 00241 /* element of p_dma_init_param is copied to element of gb_info_drv */ 00242 gb_info_drv.p_err_aio = p_dma_init_param->p_aio; 00243 00244 /* set DMA error interrupt number */ 00245 gb_info_drv.err_irq_num = DMAERR_IRQn; 00246 00247 /* init channel management information */ 00248 for (ch_count = 0; ch_count < DMA_CH_NUM; ch_count++) 00249 { 00250 /* set channel number */ 00251 gb_info_drv.info_ch[ch_count].ch = ch_count; 00252 00253 /* set DMA end interrupt number */ 00254 gb_info_drv.info_ch[ch_count].end_irq_num = gb_dma_int_num_table[ch_count]; 00255 00256 /* init next DMA setting flag */ 00257 gb_info_drv.info_ch[ch_count].next_dma_flag = false; 00258 00259 if (1U == ((uint32_t)ch_count & CHECK_ODD_EVEN_MASK)) 00260 { 00261 /* set shift number when channel is odd value */ 00262 gb_info_drv.info_ch[ch_count].shift_dmars = SHIFT_DMARS_ODD_CH; 00263 /* set mask value when channel is odd value */ 00264 gb_info_drv.info_ch[ch_count].mask_dmars = MASK_DMARS_ODD_CH; 00265 } 00266 else 00267 { 00268 /* set shift number when channel is even value */ 00269 gb_info_drv.info_ch[ch_count].shift_dmars = SHIFT_DMARS_EVEN_CH; 00270 /* set mask value when channel is even value */ 00271 gb_info_drv.info_ch[ch_count].mask_dmars = MASK_DMARS_EVEN_CH; 00272 } 00273 00274 /* init DMA setup flag */ 00275 gb_info_drv.info_ch[ch_count].setup_flag = false; 00276 00277 /* ->MISRA 11.4 This cast is needed for register access. */ 00278 /* set DMA register address for each channel */ 00279 gb_info_drv.info_ch[ch_count].p_dma_ch_reg = gb_dma_ch_register_addr_table[ch_count]; 00280 /* set common resgiter for channel 0 - 7 */ 00281 gb_info_drv.info_ch[ch_count].p_dma_common_reg = gb_dma_common_register_addr_table[ch_count]; 00282 /* <-MISRA 11.4 */ 00283 00284 /* set DMARS register for each channel */ 00285 gb_info_drv.info_ch[ch_count].p_dma_dmars_reg = gb_dmars_register_addr_table[ch_count]; 00286 } 00287 00288 /* init DMA registers */ 00289 for (ch_count = 0; ch_count < DMA_CH_NUM; ch_count++) 00290 { 00291 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N0SA_n = N0SA_INIT_VALUE; 00292 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N1SA_n = N1SA_INIT_VALUE; 00293 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N0DA_n = N0DA_INIT_VALUE; 00294 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N1DA_n = N1DA_INIT_VALUE; 00295 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N0TB_n = N0TB_INIT_VALUE; 00296 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N1TB_n = N1TB_INIT_VALUE; 00297 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHCTRL_n = CHCTRL_INIT_VALUE; 00298 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHCFG_n = CHCFG_INIT_VALUE; 00299 /* set DMA interval = 0 */ 00300 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHITVL_n = CHITVL_INIT_VALUE; 00301 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHEXT_n = CHEXT_INIT_VALUE; 00302 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->NXLA_n = NXLA_INIT_VALUE; 00303 *(gb_info_drv.info_ch[ch_count].p_dma_dmars_reg) = DMARS_INIT_VALUE; 00304 } 00305 /* init common resgiter for channel 0 - 7 */ 00306 /* set interrupt output : pulse, 00307 set round robin mode */ 00308 gb_info_drv.info_ch[DMA_CH_0].p_dma_common_reg->DCTRL_0_7 = DCTRL_INIT_VALUE; 00309 /* init common resgiter for channel 8 - 15 */ 00310 /* set interrupt output : pulse, 00311 set round robin mode */ 00312 gb_info_drv.info_ch[HIGH_COMMON_REG_OFFSET].p_dma_common_reg->DCTRL_0_7 = DCTRL_INIT_VALUE; 00313 00314 if (ESUCCESS == retval) 00315 { 00316 /* DMA end interrupt handler register */ 00317 init_check_flag = false; 00318 ch_count = 0; 00319 while (false == init_check_flag) 00320 { 00321 error_code = InterruptHandlerRegister(gb_info_drv.info_ch[ch_count].end_irq_num, 00322 gb_dma_int_handler_table[ch_count] 00323 ); 00324 /* 0 is no error on InterruptHandlerRegister() */ 00325 if (0U != error_code) 00326 { 00327 retval = (int_t)error_code; 00328 init_check_flag = true; 00329 } 00330 if ((DMA_CH_NUM - 1) == ch_count) 00331 { 00332 init_check_flag = true; 00333 } 00334 ch_count++; 00335 } 00336 00337 if (ESUCCESS == retval) 00338 { 00339 /* DMA error interrupt handler register */ 00340 error_code = InterruptHandlerRegister(gb_info_drv.err_irq_num, 00341 &R_DMA_ErrInterruptHandler 00342 ); 00343 /* 0 is no error on InterruptHandlerRegister() */ 00344 if (0U != error_code) 00345 { 00346 retval = (int_t)error_code; 00347 } 00348 } 00349 } 00350 00351 if (ESUCCESS == retval) 00352 { 00353 /* set DMA end interrupt level & priority */ 00354 for (ch_count = 0; ch_count < DMA_CH_NUM; ch_count++) 00355 { 00356 /* set interrupt level (set edge trigger, 1-N model) */ 00357 GIC_SetLevelModel(gb_info_drv.info_ch[ch_count].end_irq_num, 1, 1); 00358 } 00359 /* set DMA error interrupt level (set edge trgger, 1-N model) */ 00360 GIC_SetLevelModel(gb_info_drv.err_irq_num, 1, 1); 00361 /* DMA error interrupt enable */ 00362 GIC_EnableIRQ(gb_info_drv.err_irq_num); 00363 } 00364 00365 if (ESUCCESS == retval) 00366 { 00367 /* set channel status */ 00368 for (ch_count = 0; ch_count < DMA_CH_NUM; ch_count++) 00369 { 00370 if ((bool_t)false != p_dma_init_param->channel[ch_count]) 00371 { 00372 gb_info_drv.info_ch[ch_count].ch_stat = DMA_CH_INIT; 00373 } 00374 else 00375 { 00376 gb_info_drv.info_ch[ch_count].ch_stat = DMA_CH_UNINIT; 00377 } 00378 } 00379 /* set driver status to DMA_DRV_INIT */ 00380 gb_info_drv.drv_stat = DMA_DRV_INIT; 00381 } 00382 00383 return retval; 00384 } 00385 00386 /****************************************************************************** 00387 End of function DMA_Initialize 00388 ******************************************************************************/ 00389 00390 /****************************************************************************** 00391 * Function Name: DMA_UnInitialize 00392 * Description : UnInitialize DMA driver. 00393 * Arguments : None. 00394 * Return Value : ESUCCESS - 00395 * Operation successful. 00396 * OS error num - 00397 * Unregistering handler failed 00398 ******************************************************************************/ 00399 00400 int_t DMA_UnInitialize(void) 00401 { 00402 int_t retval = ESUCCESS; 00403 int_t ch_count; 00404 uint32_t error_code; 00405 bool_t uninit_check_flag; 00406 00407 /* init DMA registers */ 00408 for (ch_count = 0; ch_count < DMA_CH_NUM; ch_count++) 00409 { 00410 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHCTRL_n = 0; 00411 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHCFG_n = 0; 00412 *(gb_info_drv.info_ch[ch_count].p_dma_dmars_reg) = 0; 00413 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N0SA_n = 0; 00414 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N1SA_n = 0; 00415 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N0DA_n = 0; 00416 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N1DA_n = 0; 00417 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N0TB_n = 0; 00418 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->N1TB_n = 0; 00419 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHITVL_n = 0; 00420 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->CHEXT_n = 0; 00421 gb_info_drv.info_ch[ch_count].p_dma_ch_reg->NXLA_n = 0; 00422 } 00423 /* init common resgiter for channel 0 - 7 */ 00424 gb_info_drv.info_ch[DMA_CH_0].p_dma_common_reg->DCTRL_0_7 = 0; 00425 /* init common resgiter for channel 8 - 15 */ 00426 gb_info_drv.info_ch[HIGH_COMMON_REG_OFFSET].p_dma_common_reg->DCTRL_0_7 = 0; 00427 00428 /* uninit DMA interrupt */ 00429 ch_count = 0; 00430 uninit_check_flag = false; 00431 while (false == uninit_check_flag) 00432 { 00433 /* disable DMA end interrupt */ 00434 GIC_DisableIRQ(gb_info_drv.info_ch[ch_count].end_irq_num); 00435 00436 /* unregister DMA end interrupt handler */ 00437 error_code = InterruptHandlerUnregister(gb_info_drv.info_ch[ch_count].end_irq_num); 00438 /* 0 is no error on InterruptHandlerUnRegister() */ 00439 if (0U != error_code) 00440 { 00441 retval = (int_t)error_code; 00442 uninit_check_flag = true; 00443 } 00444 if ((DMA_CH_NUM - 1) == ch_count) 00445 { 00446 uninit_check_flag = true; 00447 } 00448 ch_count++; 00449 } 00450 if (ESUCCESS == retval) 00451 { 00452 /* disable DMA error interrupt */ 00453 GIC_DisableIRQ(gb_info_drv.err_irq_num); 00454 00455 /* unregister DMA interrupt error handler */ 00456 error_code = InterruptHandlerUnregister(gb_info_drv.err_irq_num); 00457 /* 0 is no error on InterruptHandlerUnRegister() */ 00458 if (0U != error_code) 00459 { 00460 retval = (int_t)error_code; 00461 } 00462 } 00463 00464 if (ESUCCESS == retval) 00465 { 00466 /* set channel status to DMA_CH_UNINIT */ 00467 for (ch_count = 0; ch_count < DMA_CH_NUM; ch_count++) 00468 { 00469 gb_info_drv.info_ch[ch_count].ch_stat = DMA_CH_UNINIT; 00470 } 00471 /* set driver status to DMA_DRV_UNINIT*/ 00472 gb_info_drv.drv_stat = DMA_DRV_UNINIT; 00473 } 00474 00475 return retval; 00476 } 00477 00478 /****************************************************************************** 00479 End of function DMA_UnInitialize 00480 ******************************************************************************/ 00481 00482 /****************************************************************************** 00483 * Function Name: DMA_OpenChannel 00484 * Description : DMA channel open. 00485 * Set DMA channel status to DMA_CH_OPEN. 00486 * Arguments : channel - 00487 * Open channel number. 00488 * Return Value : None. 00489 ******************************************************************************/ 00490 00491 static void DMA_OpenChannel(const int_t channel) 00492 { 00493 /* set channel status to DMA_CH_OPEN */ 00494 gb_info_drv.info_ch[channel].ch_stat = DMA_CH_OPEN; 00495 00496 return; 00497 } 00498 00499 /****************************************************************************** 00500 End of function DMA_OpenChannel 00501 ******************************************************************************/ 00502 00503 /****************************************************************************** 00504 * Function Name: DMA_GetFreeChannel 00505 * Description : Find free DMA channel and Get DMA channel. 00506 * Arguments : None 00507 * Return Value : channel - 00508 * Open channel number. 00509 * error code - 00510 * EMFILE : When looking for a free channel, but a free 00511 * channel didn't exist. 00512 ******************************************************************************/ 00513 00514 int_t DMA_GetFreeChannel(void) 00515 { 00516 int_t retval = EFAULT; 00517 dma_info_ch_t *dma_info_ch; 00518 int_t ch_alloc; 00519 bool_t ch_stat_check_flag; 00520 00521 /* looking for free channel */ 00522 ch_stat_check_flag = false; 00523 ch_alloc = 0; 00524 while (false == ch_stat_check_flag) 00525 { 00526 dma_info_ch = DMA_GetDrvChInfo(ch_alloc); 00527 00528 if (false == ch_stat_check_flag) 00529 { 00530 if (DMA_CH_INIT == dma_info_ch->ch_stat) 00531 { 00532 DMA_OpenChannel(ch_alloc); 00533 retval = ch_alloc; 00534 ch_stat_check_flag = true; 00535 } 00536 if (false == ch_stat_check_flag) 00537 { 00538 ch_alloc++; 00539 /* not detected free channel */ 00540 if (DMA_CH_NUM == ch_alloc) 00541 { 00542 /* set error return value */ 00543 retval = EMFILE; 00544 ch_stat_check_flag = true; 00545 } 00546 } 00547 } 00548 } 00549 00550 return retval; 00551 } 00552 00553 /****************************************************************************** 00554 End of function DMA_GetFreeChannel 00555 ******************************************************************************/ 00556 00557 /****************************************************************************** 00558 * Function Name: DMA_GetFixedChannel 00559 * Description : Get specified DMA channel number. 00560 * Arguments : channel - 00561 * Open channel number. 00562 * Return Value : channel - 00563 * Open channel number. 00564 * error code - 00565 * EBUSY : It has been allocated already in channel. 00566 * ENOTSUP : Channel status is DMA_CH_UNINIT. 00567 * EFAULT: Channel status is besides the status definded in 00568 * dma_stat_ch_t. 00569 ******************************************************************************/ 00570 00571 int_t DMA_GetFixedChannel(const int_t channel) 00572 { 00573 int_t retval = ESUCCESS; 00574 dma_info_ch_t *dma_info_ch; 00575 00576 /* allocate the specified number */ 00577 dma_info_ch = DMA_GetDrvChInfo(channel); 00578 00579 if (ESUCCESS == retval) 00580 { 00581 if (DMA_CH_INIT == dma_info_ch->ch_stat) 00582 { 00583 DMA_OpenChannel(channel); 00584 /* return alloc channel number */ 00585 retval = channel; 00586 } 00587 else 00588 { 00589 /* set error return value */ 00590 switch (dma_info_ch->ch_stat) 00591 { 00592 case DMA_CH_UNINIT: 00593 retval = ENOTSUP; 00594 break; 00595 /* These 2 cases are intentionally combined. */ 00596 case DMA_CH_OPEN: 00597 case DMA_CH_TRANSFER: 00598 retval = EBUSY; 00599 break; 00600 00601 default: 00602 retval = EFAULT; 00603 break; 00604 00605 } 00606 } 00607 } 00608 00609 return retval; 00610 } 00611 00612 /****************************************************************************** 00613 End of function DMA_GetFixedChannel 00614 ******************************************************************************/ 00615 00616 /****************************************************************************** 00617 * Function Name: DMA_CloseChannel 00618 * Description : DMA channel close. 00619 * Set DMA channel status to DMA_CH_INIT. 00620 * Arguments : channel - 00621 * Close channel number. 00622 * Return Value : None. 00623 ******************************************************************************/ 00624 00625 void DMA_CloseChannel(const int_t channel) 00626 { 00627 /* clear DMARS register */ 00628 *(gb_info_drv.info_ch[channel].p_dma_dmars_reg) &= gb_info_drv.info_ch[channel].mask_dmars; 00629 00630 /* set channel status to DMA_CH_INIT */ 00631 gb_info_drv.info_ch[channel].ch_stat = DMA_CH_INIT; 00632 00633 return; 00634 } 00635 00636 /****************************************************************************** 00637 End of function DMA_CloseChannel 00638 ******************************************************************************/ 00639 00640 /****************************************************************************** 00641 * Function Name: DMA_Setparam 00642 * Description : Set DMA transfer parameter to Register. 00643 * Arguments : channel - 00644 * Set up channel number. 00645 * *p_ch_setup - 00646 * Set up parameters. 00647 * *p_ch_cfg - 00648 * DMA channel config table parameters. 00649 * *reqd 00650 * set vaule for REQD bit on CHCFG 00651 * Return Value : None. 00652 ******************************************************************************/ 00653 00654 void DMA_SetParam(const int_t channel, const dma_ch_setup_t *const p_ch_setup, 00655 const dma_ch_cfg_t * const p_ch_cfg, const uint32_t reqd) 00656 { 00657 uint32_t chcfg_sel; 00658 uint32_t value_dmars; 00659 00660 /* set DMA transfer parameter to DMA channel infomation */ 00661 gb_info_drv.info_ch[channel].resource = p_ch_setup->resource; 00662 gb_info_drv.info_ch[channel].direction = p_ch_setup->direction; 00663 gb_info_drv.info_ch[channel].src_width = p_ch_setup->src_width; 00664 gb_info_drv.info_ch[channel].src_cnt = p_ch_setup->src_cnt; 00665 gb_info_drv.info_ch[channel].dst_width = p_ch_setup->dst_width; 00666 gb_info_drv.info_ch[channel].dst_cnt = p_ch_setup->dst_cnt; 00667 gb_info_drv.info_ch[channel].p_end_aio = p_ch_setup->p_aio; 00668 00669 /* set DMARS value and protect non change bit */ 00670 value_dmars = *(gb_info_drv.info_ch[channel].p_dma_dmars_reg); 00671 value_dmars = ((value_dmars & gb_info_drv.info_ch[channel].mask_dmars) | 00672 (uint32_t)(p_ch_cfg->dmars << gb_info_drv.info_ch[channel].shift_dmars)); 00673 /* set DMARS register value */ 00674 *(gb_info_drv.info_ch[channel].p_dma_dmars_reg) = value_dmars; 00675 00676 /* set CHCFG regsiter */ 00677 if (channel < HIGH_COMMON_REG_OFFSET) 00678 { 00679 chcfg_sel = (uint32_t)channel; 00680 } 00681 else 00682 { 00683 chcfg_sel = (uint32_t)(channel - HIGH_COMMON_REG_OFFSET); 00684 } 00685 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n 00686 = ((uint32_t)CHCFG_FIXED_VALUE | 00687 /* ->MISRA 21.1 ,IPA R2.4.1 The value of every parameter won't be minus. 00688 and the value after a shift will be less than 0x80000000 certainly. 00689 */ 00690 (((uint32_t)p_ch_setup->dst_cnt << CHCFG_SHIFT_DAD) & CHCFG_MASK_DAD) | 00691 (((uint32_t)p_ch_setup->src_cnt << CHCFG_SHIFT_SAD) & CHCFG_MASK_SAD) | 00692 (((uint32_t)p_ch_setup->dst_width << CHCFG_SHIFT_DDS) & CHCFG_MASK_DDS) | 00693 (((uint32_t)p_ch_setup->src_width << CHCFG_SHIFT_SDS) & CHCFG_MASK_SDS) | 00694 /* <-MISRA 21.1, IPA R2.4.1 */ 00695 p_ch_cfg->tm | 00696 p_ch_cfg->lvl | 00697 reqd | 00698 chcfg_sel); 00699 00700 /* set setup flag */ 00701 gb_info_drv.info_ch[channel].setup_flag = true; 00702 00703 return; 00704 } 00705 00706 /****************************************************************************** 00707 End of function DMA_SetParam 00708 ******************************************************************************/ 00709 00710 /****************************************************************************** 00711 * Function Name: DMA_BusParam 00712 * Description : Set bus parameter for DMA. 00713 * Arguments : channel - 00714 * Set address channel number. 00715 * *p_dma_data - 00716 * DMA transfer address parameter set. 00717 * Return Value : None. 00718 ******************************************************************************/ 00719 00720 void DMA_BusParam(const int_t channel, const dma_trans_data_t * const p_dma_data) 00721 { 00722 uint32_t src_bus_addr = (uint32_t)p_dma_data->src_addr; 00723 uint32_t dst_bus_addr = (uint32_t)p_dma_data->dst_addr; 00724 uint32_t chext_value = (CHEXT_SET_DPR_NON_SECURE | CHEXT_SET_SPR_NON_SECURE); 00725 00726 /* set bus parameter for SRC */ 00727 if ((DMA_EXTERNAL_BUS_END >= src_bus_addr) || 00728 ((DMA_EXTERNAL_BUS_MIRROR_START <= src_bus_addr) && 00729 (DMA_EXTERNAL_BUS_MIRROR_END >= src_bus_addr))) 00730 00731 { 00732 chext_value |= CHEXT_SET_SCA_NORMAL; 00733 } 00734 else 00735 { 00736 chext_value |= CHEXT_SET_SCA_STRONG; 00737 } 00738 00739 /* set bus parameter for DST */ 00740 if ((DMA_EXTERNAL_BUS_END >= dst_bus_addr) || 00741 ((DMA_EXTERNAL_BUS_MIRROR_START <= dst_bus_addr) && 00742 (DMA_EXTERNAL_BUS_MIRROR_END >= dst_bus_addr))) 00743 00744 { 00745 chext_value |= CHEXT_SET_DCA_NORMAL; 00746 } 00747 else 00748 { 00749 chext_value |= CHEXT_SET_DCA_STRONG; 00750 } 00751 00752 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHEXT_n = chext_value; 00753 00754 return; 00755 } 00756 00757 /****************************************************************************** 00758 End of function DMA_BusParam 00759 ******************************************************************************/ 00760 00761 /****************************************************************************** 00762 * Function Name: DMA_SetData 00763 * Description : Set DMA transfer address to Register. 00764 * Arguments : channel - 00765 * Set address channel number. 00766 * *p_dma_data - 00767 * DMA transfer address parameter set. 00768 * next_register_set - 00769 * Number of next register set. 00770 * Return Value : None. 00771 ******************************************************************************/ 00772 00773 void DMA_SetData(const int_t channel, const dma_trans_data_t * const p_dma_data, 00774 const uint32_t next_register_set) 00775 { 00776 if (0U == next_register_set) 00777 { 00778 /* set DMA transfer address parameters to next register set0 */ 00779 gb_info_drv.info_ch[channel].src_addr0 = p_dma_data->src_addr; 00780 gb_info_drv.info_ch[channel].dst_addr0 = p_dma_data->dst_addr; 00781 gb_info_drv.info_ch[channel].count0 = p_dma_data->count; 00782 00783 /* ->MISRA 11.3 This cast is needed for setting address to register. */ 00784 /* set DAM transfer addres to register */ 00785 gb_info_drv.info_ch[channel].p_dma_ch_reg->N0SA_n = (uint32_t)p_dma_data->src_addr; 00786 gb_info_drv.info_ch[channel].p_dma_ch_reg->N0DA_n = (uint32_t)p_dma_data->dst_addr; 00787 /* <-MISRA 11.3 */ 00788 gb_info_drv.info_ch[channel].p_dma_ch_reg->N0TB_n = p_dma_data->count; 00789 } 00790 else 00791 { 00792 /* set DMA transfer address parameters to next regiter set1 */ 00793 gb_info_drv.info_ch[channel].src_addr1 = p_dma_data->src_addr; 00794 gb_info_drv.info_ch[channel].dst_addr1 = p_dma_data->dst_addr; 00795 gb_info_drv.info_ch[channel].count1 = p_dma_data->count; 00796 00797 /* ->MISRA 11.3 This cast is needed for setting address to register. */ 00798 /* set DAM transfer addres to register */ 00799 gb_info_drv.info_ch[channel].p_dma_ch_reg->N1SA_n = (uint32_t)p_dma_data->src_addr; 00800 gb_info_drv.info_ch[channel].p_dma_ch_reg->N1DA_n = (uint32_t)p_dma_data->dst_addr; 00801 /* <-MISRA 11.3 */ 00802 gb_info_drv.info_ch[channel].p_dma_ch_reg->N1TB_n = p_dma_data->count; 00803 } 00804 00805 return; 00806 } 00807 00808 /****************************************************************************** 00809 End of function DMA_SetData 00810 ******************************************************************************/ 00811 00812 /****************************************************************************** 00813 * Function Name: DMA_SetNextData 00814 * Description : Set continuous DMA transfer setting. 00815 * Arguments : channel - 00816 * Set continuous DMA transfer channel number. 00817 * *p_dma_data - 00818 * DMA transfer address parameter set. 00819 * Return Value : None. 00820 ******************************************************************************/ 00821 00822 void DMA_SetNextData(const int_t channel, const dma_trans_data_t * const p_dma_data) 00823 { 00824 uint32_t next_register_set; 00825 00826 /* check number of next register set for next DMA transfer */ 00827 /* The reverse number in current number is set in next regsiter set of next DMA. */ 00828 if (0U == (gb_info_drv.info_ch[channel].p_dma_ch_reg->CHSTAT_n & CHSTAT_MASK_SR)) 00829 { 00830 next_register_set = 1U; 00831 } 00832 else 00833 { 00834 next_register_set = 0U; 00835 } 00836 00837 /* set DMA transfer address for next DMA */ 00838 DMA_SetData(channel, p_dma_data, next_register_set); 00839 00840 /* start setting for next DMA */ 00841 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n |= (uint32_t)(CHCFG_SET_REN | CHCFG_SET_RSW); 00842 00843 /* set flag wich indicates that next DMA transfer set already */ 00844 gb_info_drv.info_ch[channel].next_dma_flag = true; 00845 00846 /* auto restart continous DMA */ 00847 if ((0U == (gb_info_drv.info_ch[channel].p_dma_ch_reg->CHSTAT_n & CHSTAT_MASK_EN)) && 00848 (false == gb_info_drv.info_ch[channel].setup_flag)) 00849 { 00850 /* auto restart DMA */ 00851 DMA_SetData(channel, p_dma_data, 0); 00852 DMA_Start(channel, true); 00853 } 00854 00855 return; 00856 } 00857 00858 /****************************************************************************** 00859 End of function DMA_Nextdata 00860 ******************************************************************************/ 00861 00862 /****************************************************************************** 00863 * Function Name: DMA_Start 00864 * Description : Start DMA transfer. 00865 * Arguments : channel - 00866 * DMA transfer start channel number. 00867 * :restart_flag - 00868 * Flag of DMA continous transfer auto restart. 00869 * Return Value : None. 00870 ******************************************************************************/ 00871 00872 void DMA_Start(const int_t channel, const bool_t restart_flag) 00873 { 00874 if (false != restart_flag) 00875 { 00876 /* clear continous DMA setting */ 00877 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n &= 00878 ~(uint32_t)(CHCFG_SET_RSW | CHCFG_SET_RSEL | CHCFG_SET_REN); 00879 gb_info_drv.info_ch[channel].next_dma_flag = false; 00880 } 00881 00882 /* clear setup flag */ 00883 gb_info_drv.info_ch[channel].setup_flag = false; 00884 00885 /* reset DMA */ 00886 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCTRL_n = CHCTRL_SET_SWRST; 00887 00888 /* clear mask of DMA transfer end */ 00889 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n &= ~((uint32_t)CHCFG_SET_DEM); 00890 00891 GIC_EnableIRQ(gb_info_drv.info_ch[channel].end_irq_num); 00892 00893 /* start DMA transfer */ 00894 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCTRL_n = CHCTRL_SET_SETEN; 00895 00896 /* set channel status to DMA_CH_TRANSFER */ 00897 gb_info_drv.info_ch[channel].ch_stat = DMA_CH_TRANSFER; 00898 00899 return; 00900 } 00901 00902 /****************************************************************************** 00903 End of function DMA_Start 00904 ******************************************************************************/ 00905 00906 /****************************************************************************** 00907 * Function Name: DMA_Stop 00908 * Description : Stop DMA transfer. 00909 * Arguments : channel - 00910 * DMA transfer start channel number. 00911 * *p_remain - 00912 * Remain data size of DMA transfer. 00913 * Return Value : None. 00914 ******************************************************************************/ 00915 00916 void DMA_Stop(const int_t channel, uint32_t * const p_remain) 00917 { 00918 uint32_t stop_wait_cnt; 00919 00920 /* disable DMA end interrupt */ 00921 GIC_DisableIRQ(gb_info_drv.info_ch[channel].end_irq_num); 00922 00923 /* stop DMA transfer */ 00924 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCTRL_n = CHCTRL_SET_CLREN; 00925 00926 /* wait DMA stop */ 00927 stop_wait_cnt = 0; 00928 while ((0 != (gb_info_drv.info_ch[channel].p_dma_ch_reg->CHSTAT_n & CHSTAT_MASK_TACT)) && 00929 (DMA_STOP_WAIT_MAX_CNT > stop_wait_cnt)) 00930 { 00931 stop_wait_cnt++; 00932 } 00933 00934 if (DMA_STOP_WAIT_MAX_CNT <= stop_wait_cnt) 00935 { 00936 /* NON_NOTICE_ASSERT: wait count is abnormal value (usually, a count is set to 0 or 1) */ 00937 } 00938 00939 /* get remain data size */ 00940 *p_remain = gb_info_drv.info_ch[channel].p_dma_ch_reg->CRTB_n; 00941 00942 /* set mask of DMA transfer end */ 00943 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n |= (uint32_t)CHCFG_SET_DEM; 00944 00945 /* clear setting of continuous DMA */ 00946 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n &= ~(uint32_t)(CHCFG_SET_RSW | CHCFG_SET_RSEL); 00947 00948 /* clear TC, END bit */ 00949 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCTRL_n = (CHCTRL_SET_CLRTC | CHCTRL_SET_CLREND); 00950 00951 /* clear flag wich indicates that next DMA transfer set already */ 00952 gb_info_drv.info_ch[channel].next_dma_flag = false; 00953 00954 /* interrupt clear, if interrupt occured already */ 00955 GIC_ClearPendingIRQ(gb_info_drv.info_ch[channel].end_irq_num); 00956 00957 /* set channel status to DMA_CH_OPEN */ 00958 gb_info_drv.info_ch[channel].ch_stat = DMA_CH_OPEN; 00959 00960 return; 00961 } 00962 00963 /****************************************************************************** 00964 End of function DMA_Stop 00965 ******************************************************************************/ 00966 00967 /****************************************************************************** 00968 * Function Name: DMA_SetErrCode 00969 * Description : Set error code to error code pointer. 00970 * If error code pointer is NULL, nothing is done. 00971 * Arguments : error_code - 00972 * Error code. 00973 * *p_errno - 00974 * Pointer of set error code. 00975 * Return Value : None. 00976 ******************************************************************************/ 00977 00978 void DMA_SetErrCode(const int_t error_code, int32_t * const p_errno) 00979 { 00980 if (NULL != p_errno) 00981 { 00982 *p_errno = error_code; 00983 } 00984 00985 return; 00986 } 00987 00988 /****************************************************************************** 00989 End of function DMA_SetErrCode 00990 ******************************************************************************/ 00991 00992 /****************************************************************************** 00993 * Function Name: R_DMA_ErrInterruptHandler 00994 * Description : DMA error interrupt handler. 00995 * Notify error information to the module function which called DMA driver. 00996 * Arguments : None. 00997 * Return Value : None. 00998 * Note: store error code (EIO) to AIOCB member. 00999 ******************************************************************************/ 01000 01001 static void R_DMA_ErrInterruptHandler(void) 01002 { 01003 uint32_t dstat_er_0_7; 01004 uint32_t dstat_er_8_15; 01005 01006 if (NULL != gb_info_drv.p_err_aio) 01007 { 01008 /* get error channel number */ 01009 dstat_er_0_7 = gb_info_drv.info_ch[DMA_CH_0].p_dma_common_reg->DSTAT_ER_0_7; 01010 dstat_er_8_15 = gb_info_drv.info_ch[HIGH_COMMON_REG_OFFSET].p_dma_common_reg->DSTAT_ER_0_7; 01011 01012 /* set error infrmation */ 01013 gb_info_drv.p_err_aio->aio_sigevent.sigev_value.sival_int = (int_t)(dstat_er_0_7 | (dstat_er_8_15 << HIGH_COMMON_REG_OFFSET)); 01014 01015 /* set error code (EIO) */ 01016 gb_info_drv.p_err_aio->aio_return = EIO; 01017 01018 /* call back to the module function which called DMA driver */ 01019 ahf_complete(NULL, gb_info_drv.p_err_aio); 01020 } 01021 else 01022 { 01023 ; 01024 /* NON_NOTICE_ASSERT:<callback pointer is NULL> */ 01025 } 01026 01027 } 01028 01029 /****************************************************************************** 01030 End of function R_DMA_ErrInteruuptHandler 01031 ******************************************************************************/ 01032 01033 /****************************************************************************** 01034 * Function Name: R_DMA_End0InterruptHandler 01035 * Description : DMA end interrupt handler for channel 0. 01036 * It's processed to DMA complete and notify DMA transfer finished 01037 * to the module function which called DMA driver. 01038 * Arguments : None. 01039 * Return Value : None. 01040 * Note: store error code (EIO) to AIOCB. 01041 * ESUCCESS - 01042 * DMA transfer completed. 01043 * EIO - 01044 * DMA transfer don't stopped. 01045 ******************************************************************************/ 01046 01047 static void R_DMA_End0InterruptHandler(void) 01048 { 01049 01050 R_DMA_EndHandlerProcess(DMA_CH_0); 01051 01052 } 01053 01054 /****************************************************************************** 01055 End of function R_DMA_End0InterruptHandler 01056 ******************************************************************************/ 01057 01058 /****************************************************************************** 01059 * Function Name: R_DMA_End1InterruptHandler 01060 * Description : DMA end interrupt handler for channel 1. 01061 * It's processed to DMA complete and notify DMA transfer finished 01062 * to the module function which called DMA driver. 01063 * Arguments : None. 01064 * Return Value : None. 01065 * Note: store error code (EIO) to AIOCB. 01066 * ESUCCESS - 01067 * DMA transfer completed. 01068 * EIO - 01069 * DMA transfer don't stopped. 01070 ******************************************************************************/ 01071 01072 static void R_DMA_End1InterruptHandler(void) 01073 { 01074 01075 R_DMA_EndHandlerProcess(DMA_CH_1); 01076 01077 } 01078 01079 /****************************************************************************** 01080 End of function R_DMA_End1InterruptHandler 01081 ******************************************************************************/ 01082 01083 /****************************************************************************** 01084 * Function Name: R_DMA_End2InterruptHandler 01085 * Description : DMA end interrupt handler for channel 2. 01086 * It's processed to DMA complete and notify DMA transfer finished 01087 * to the module function which called DMA driver. 01088 * Arguments : None. 01089 * Return Value : None. 01090 * Note: store error code (EIO) to AIOCB. 01091 * ESUCCESS - 01092 * DMA transfer completed. 01093 * EIO - 01094 * DMA transfer don't stopped. 01095 ******************************************************************************/ 01096 01097 static void R_DMA_End2InterruptHandler(void) 01098 { 01099 01100 R_DMA_EndHandlerProcess(DMA_CH_2); 01101 01102 } 01103 01104 /****************************************************************************** 01105 End of function R_DMA_End2InterruptHandler 01106 ******************************************************************************/ 01107 01108 /****************************************************************************** 01109 * Function Name: R_DMA_End3InterruptHandler 01110 * Description : DMA end interrupt handler for channel 3. 01111 * It's processed to DMA complete and notify DMA transfer finished 01112 * to the module function which called DMA driver. 01113 * Arguments : None. 01114 * Return Value : None. 01115 * Note: store error code (EIO) to AIOCB. 01116 * ESUCCESS - 01117 * DMA transfer completed. 01118 * EIO - 01119 * DMA transfer don't stopped. 01120 ******************************************************************************/ 01121 01122 static void R_DMA_End3InterruptHandler(void) 01123 { 01124 01125 R_DMA_EndHandlerProcess(DMA_CH_3); 01126 01127 } 01128 01129 /****************************************************************************** 01130 End of function R_DMA_End3InterruptHandler 01131 ******************************************************************************/ 01132 01133 /****************************************************************************** 01134 * Function Name: R_DMA_End4InterruptHandler 01135 * Description : DMA end interrupt handler for channel 4. 01136 * It's processed to DMA complete and notify DMA transfer finished 01137 * to the module function which called DMA driver. 01138 * Arguments : None. 01139 * Return Value : None. 01140 * Note: store error code (EIO) to AIOCB. 01141 * ESUCCESS - 01142 * DMA transfer completed. 01143 * EIO - 01144 * DMA transfer don't stopped. 01145 ******************************************************************************/ 01146 01147 static void R_DMA_End4InterruptHandler(void) 01148 { 01149 01150 R_DMA_EndHandlerProcess(DMA_CH_4); 01151 01152 } 01153 01154 /****************************************************************************** 01155 End of function R_DMA_End4InterruptHandler 01156 ******************************************************************************/ 01157 01158 /****************************************************************************** 01159 * Function Name: R_DMA_End5InterruptHandler 01160 * Description : DMA end interrupt handler for channel 5. 01161 * It's processed to DMA complete and notify DMA transfer finished 01162 * to the module function which called DMA driver. 01163 * Arguments : None. 01164 * Return Value : None. 01165 * Note: store error code (EIO) to AIOCB. 01166 * ESUCCESS - 01167 * DMA transfer completed. 01168 * EIO - 01169 * DMA transfer don't stopped. 01170 ******************************************************************************/ 01171 01172 static void R_DMA_End5InterruptHandler(void) 01173 { 01174 01175 R_DMA_EndHandlerProcess(DMA_CH_5); 01176 01177 } 01178 01179 /****************************************************************************** 01180 End of function R_DMA_End5InterruptHandler 01181 ******************************************************************************/ 01182 01183 /****************************************************************************** 01184 * Function Name: R_DMA_End6InterruptHandler 01185 * Description : DMA end interrupt handler for channel 6. 01186 * It's processed to DMA complete and notify DMA transfer finished 01187 * to the module function which called DMA driver. 01188 * Arguments : None. 01189 * Return Value : None. 01190 * Note: store error code (EIO) to AIOCB. 01191 * ESUCCESS - 01192 * DMA transfer completed. 01193 * EIO - 01194 * DMA transfer don't stopped. 01195 ******************************************************************************/ 01196 01197 static void R_DMA_End6InterruptHandler(void) 01198 { 01199 01200 R_DMA_EndHandlerProcess(DMA_CH_6); 01201 01202 } 01203 01204 /****************************************************************************** 01205 End of function R_DMA_End6InterruptHandler 01206 ******************************************************************************/ 01207 01208 /****************************************************************************** 01209 * Function Name: R_DMA_End7InterruptHandler 01210 * Description : DMA end interrupt handler for channel 7. 01211 * It's processed to DMA complete and notify DMA transfer finished 01212 * to the module function which called DMA driver. 01213 * Arguments : None. 01214 * Return Value : None. 01215 * Note: store error code (EIO) to AIOCB. 01216 * ESUCCESS - 01217 * DMA transfer completed. 01218 * EIO - 01219 * DMA transfer don't stopped. 01220 ******************************************************************************/ 01221 01222 static void R_DMA_End7InterruptHandler(void) 01223 { 01224 01225 R_DMA_EndHandlerProcess(DMA_CH_7); 01226 01227 } 01228 01229 /****************************************************************************** 01230 End of function R_DMA_End7InterruptHandler 01231 ******************************************************************************/ 01232 01233 /****************************************************************************** 01234 * Function Name: R_DMA_End8InterruptHandler 01235 * Description : DMA end interrupt handler for channel 8. 01236 * It's processed to DMA complete and notify DMA transfer finished 01237 * to the module function which called DMA driver. 01238 * Arguments : None. 01239 * Return Value : None. 01240 * Note: store error code (EIO) to AIOCB. 01241 * ESUCCESS - 01242 * DMA transfer completed. 01243 * EIO - 01244 * DMA transfer don't stopped. 01245 ******************************************************************************/ 01246 01247 static void R_DMA_End8InterruptHandler(void) 01248 { 01249 01250 R_DMA_EndHandlerProcess(DMA_CH_8); 01251 01252 } 01253 01254 /****************************************************************************** 01255 End of function R_DMA_End8InterruptHandler 01256 ******************************************************************************/ 01257 01258 /****************************************************************************** 01259 * Function Name: R_DMA_End9InterruptHandler 01260 * Description : DMA end interrupt handler for channel 9. 01261 * It's processed to DMA complete and notify DMA transfer finished 01262 * to the module function which called DMA driver. 01263 * Arguments : None. 01264 * Return Value : None. 01265 * Note: store error code (EIO) to AIOCB. 01266 * ESUCCESS - 01267 * DMA transfer completed. 01268 * EIO - 01269 * DMA transfer don't stopped. 01270 ******************************************************************************/ 01271 01272 static void R_DMA_End9InterruptHandler(void) 01273 { 01274 01275 R_DMA_EndHandlerProcess(DMA_CH_9); 01276 01277 } 01278 01279 /****************************************************************************** 01280 End of function R_DMA_End9InterruptHandler 01281 ******************************************************************************/ 01282 01283 /****************************************************************************** 01284 * Function Name: R_DMA_End10InterruptHandler 01285 * Description : DMA end interrupt handler for channel 10. 01286 * It's processed to DMA complete and notify DMA transfer finished 01287 * to the module function which called DMA driver. 01288 * Arguments : None. 01289 * Return Value : None. 01290 * Note: store error code (EIO) to AIOCB. 01291 * ESUCCESS - 01292 * DMA transfer completed. 01293 * EIO - 01294 * DMA transfer don't stopped. 01295 ******************************************************************************/ 01296 01297 static void R_DMA_End10InterruptHandler(void) 01298 { 01299 01300 R_DMA_EndHandlerProcess(DMA_CH_10); 01301 01302 } 01303 01304 /****************************************************************************** 01305 End of function R_DMA_End10InterruptHandler 01306 ******************************************************************************/ 01307 01308 /****************************************************************************** 01309 * Function Name: R_DMA_End11InterruptHandler 01310 * Description : DMA end interrupt handler for channel 11. 01311 * It's processed to DMA complete and notify DMA transfer finished 01312 * to the module function which called DMA driver. 01313 * Arguments : None. 01314 * Return Value : None. 01315 * Note: store error code (EIO) to AIOCB. 01316 * ESUCCESS - 01317 * DMA transfer completed. 01318 * EIO - 01319 * DMA transfer don't stopped. 01320 ******************************************************************************/ 01321 01322 static void R_DMA_End11InterruptHandler(void) 01323 { 01324 01325 R_DMA_EndHandlerProcess(DMA_CH_11); 01326 01327 } 01328 01329 /****************************************************************************** 01330 End of function R_DMA_End11InterruptHandler 01331 ******************************************************************************/ 01332 01333 /****************************************************************************** 01334 * Function Name: R_DMA_End12InterruptHandler 01335 * Description : DMA end interrupt handler for channel 12. 01336 * It's processed to DMA complete and notify DMA transfer finished 01337 * to the module function which called DMA driver. 01338 * Arguments : None. 01339 * Return Value : None. 01340 * Note: store error code (EIO) to AIOCB. 01341 * ESUCCESS - 01342 * DMA transfer completed. 01343 * EIO - 01344 * DMA transfer don't stopped. 01345 ******************************************************************************/ 01346 01347 static void R_DMA_End12InterruptHandler(void) 01348 { 01349 01350 R_DMA_EndHandlerProcess(DMA_CH_12); 01351 01352 } 01353 01354 /****************************************************************************** 01355 End of function R_DMA_End12InterruptHandler 01356 ******************************************************************************/ 01357 01358 /****************************************************************************** 01359 * Function Name: R_DMA_End13InterruptHandler 01360 * Description : DMA end interrupt handler for channel 13. 01361 * It's processed to DMA complete and notify DMA transfer finished 01362 * to the module function which called DMA driver. 01363 * Arguments : None. 01364 * Return Value : None. 01365 * Note: store error code (EIO) to AIOCB. 01366 * ESUCCESS - 01367 * DMA transfer completed. 01368 * EIO - 01369 * DMA transfer don't stopped. 01370 ******************************************************************************/ 01371 01372 static void R_DMA_End13InterruptHandler(void) 01373 { 01374 01375 R_DMA_EndHandlerProcess(DMA_CH_13); 01376 01377 } 01378 01379 /****************************************************************************** 01380 End of function R_DMA_End13InterruptHandler 01381 ******************************************************************************/ 01382 01383 /****************************************************************************** 01384 * Function Name: R_DMA_End14InterruptHandler 01385 * Description : DMA end interrupt handler for channel 14. 01386 * It's processed to DMA complete and notify DMA transfer finished 01387 * to the module function which called DMA driver. 01388 * Arguments : None. 01389 * Return Value : None. 01390 * Note: store error code (EIO) to AIOCB. 01391 * ESUCCESS - 01392 * DMA transfer completed. 01393 * EIO - 01394 * DMA transfer don't stopped. 01395 ******************************************************************************/ 01396 01397 static void R_DMA_End14InterruptHandler(void) 01398 { 01399 01400 R_DMA_EndHandlerProcess(DMA_CH_14); 01401 01402 } 01403 01404 /****************************************************************************** 01405 End of function R_DMA_End14InterruptHandler 01406 ******************************************************************************/ 01407 01408 /****************************************************************************** 01409 * Function Name: R_DMA_End15InterruptHandler 01410 * Description : DMA end interrupt handler for channel 15. 01411 * It's processed to DMA complete and notify DMA transfer finished 01412 * to the module function which called DMA driver. 01413 * Arguments : None. 01414 * Return Value : None. 01415 * Note: store error code (EIO) to AIOCB. 01416 * ESUCCESS - 01417 * DMA transfer completed. 01418 * EIO - 01419 * DMA transfer don't stopped. 01420 ******************************************************************************/ 01421 01422 static void R_DMA_End15InterruptHandler(void) 01423 { 01424 01425 R_DMA_EndHandlerProcess(DMA_CH_15); 01426 01427 } 01428 01429 /****************************************************************************** 01430 End of function R_DMA_End15InterruptHandler 01431 ******************************************************************************/ 01432 01433 /****************************************************************************** 01434 * Function Name: R_DMA_EndHandlerProcess 01435 * Description : DMA end interrupt handler process carry out. 01436 * It's processed to DMA complete and notify DMA transfer finished 01437 * to the module function which called DMA driver. 01438 * Arguments : channel - 01439 * Open channel number. 01440 * Return Value : None. 01441 * Note: store error code (EIO) to AIOCB. 01442 * ESUCCESS - 01443 * DMA transfer completed. 01444 * EIO - 01445 * DMA transfer don't stopped. 01446 ******************************************************************************/ 01447 01448 __inline static void R_DMA_EndHandlerProcess(const int_t channel) 01449 { 01450 bool_t store_next_dma_flag; 01451 int_t was_masked; 01452 01453 if (NULL != gb_info_drv.info_ch[channel].p_end_aio) 01454 { 01455 /* disable all irq */ 01456 #if defined (__ICCARM__) 01457 was_masked = __disable_irq_iar(); 01458 #else 01459 was_masked = __disable_irq(); 01460 #endif 01461 01462 /* store next_dma_flag */ 01463 store_next_dma_flag = gb_info_drv.info_ch[channel].next_dma_flag; 01464 01465 /* clear flag wich indicates that next DMA transfer set already */ 01466 gb_info_drv.info_ch[channel].next_dma_flag = false; 01467 01468 if (false == store_next_dma_flag) 01469 { 01470 /* DMA transfer complete */ 01471 /* mask DMA end interrupt */ 01472 GIC_DisableIRQ(gb_info_drv.info_ch[channel].end_irq_num); 01473 01474 /* set channel status to DMA_CH_OPEN */ 01475 gb_info_drv.info_ch[channel].ch_stat = DMA_CH_OPEN; 01476 01477 /* set mask of DMA transfer end */ 01478 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n |= (uint32_t)CHCFG_SET_DEM; 01479 01480 /* clear setting of continuous DMA */ 01481 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCFG_n &= ~(uint32_t)(CHCFG_SET_RSW | CHCFG_SET_RSEL); 01482 01483 /* check EN bit is clear */ 01484 if (0U == (gb_info_drv.info_ch[channel].p_dma_ch_reg->CHSTAT_n & CHSTAT_MASK_EN)) 01485 { 01486 /* set error code (ESUCCESS) */ 01487 gb_info_drv.info_ch[channel].p_end_aio->aio_return = ESUCCESS; 01488 } 01489 else 01490 { 01491 /* set error code (EIO) */ 01492 gb_info_drv.info_ch[channel].p_end_aio->aio_return = EIO; 01493 } 01494 } 01495 else 01496 { 01497 /* set next DMA already */ 01498 /* set error code (ESUCCESS) */ 01499 gb_info_drv.info_ch[channel].p_end_aio->aio_return = ESUCCESS; 01500 } 01501 01502 /* clear TC, END bit */ 01503 gb_info_drv.info_ch[channel].p_dma_ch_reg->CHCTRL_n = (CHCTRL_SET_CLRTC | CHCTRL_SET_CLREND); 01504 01505 if (0 == was_masked) 01506 { 01507 __enable_irq(); 01508 } 01509 01510 /* call back to the module function which called DMA driver */ 01511 ahf_complete(NULL, gb_info_drv.info_ch[channel].p_end_aio); 01512 } 01513 else 01514 { 01515 ; 01516 /* NON_NOTICE_ASSERT:<callback pointer is NULL> */ 01517 } 01518 01519 return; 01520 } 01521 01522 /****************************************************************************** 01523 End of function R_DMA_EndHandlerProcess 01524 ******************************************************************************/ 01525
Generated on Tue Jul 12 2022 20:43:58 by
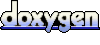