RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
R_BSP_Aio.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "cmsis_os.h" 00025 #include "r_typedefs.h" 00026 #include "r_errno.h" 00027 #include "misratypes.h" 00028 #include "aioif.h" 00029 #include "R_BSP_Aio.h" 00030 00031 typedef int32_t (*rbsp_read_write_a_func_t)(void* const p_fd, AIOCB* const p_aio, int32_t* const p_errno); 00032 00033 R_BSP_Aio::R_BSP_Aio() { 00034 } 00035 00036 R_BSP_Aio::~R_BSP_Aio() { 00037 if (write_ctl.MaxNum != 0) { 00038 delete [] (rbsp_sival_t *)write_ctl.p_sival_top; 00039 delete [] (AIOCB *)write_ctl.p_aio_top; 00040 delete write_ctl.p_sem_ctl; 00041 } 00042 if (read_ctl.MaxNum != 0) { 00043 delete [] (rbsp_sival_t *)read_ctl.p_sival_top; 00044 delete [] (AIOCB *)read_ctl.p_aio_top; 00045 delete read_ctl.p_sem_ctl; 00046 } 00047 } 00048 00049 void R_BSP_Aio::init(rbsp_serial_ctl_t * p_ctl, void * handle, void * p_func_a, int32_t max_buff_num) { 00050 if (handle != NULL) { 00051 p_ctl->ch_handle = handle; 00052 p_ctl->MaxNum = max_buff_num; 00053 p_ctl->p_aio_top = NULL; 00054 p_ctl->index = 0; 00055 p_ctl->p_async_func = p_func_a; 00056 if (p_ctl->MaxNum != 0) { 00057 p_ctl->p_sem_ctl = new Semaphore(p_ctl->MaxNum); 00058 p_ctl->p_aio_top = new AIOCB[p_ctl->MaxNum]; 00059 p_ctl->p_sival_top = new rbsp_sival_t[p_ctl->MaxNum]; 00060 } 00061 } 00062 } 00063 00064 int32_t R_BSP_Aio::write(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf) { 00065 if (p_data_conf == NULL) { 00066 return sync_trans(&write_ctl, p_data, data_size); 00067 } else { 00068 return aio_trans(&write_ctl, p_data, data_size, p_data_conf); 00069 } 00070 } 00071 00072 int32_t R_BSP_Aio::read(void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf) { 00073 if (p_data_conf == NULL) { 00074 return sync_trans(&read_ctl, p_data, data_size); 00075 } else { 00076 return aio_trans(&read_ctl, p_data, data_size, p_data_conf); 00077 } 00078 } 00079 00080 /* static */ int32_t R_BSP_Aio::sync_trans(rbsp_serial_ctl_t * p_ctl, void * const p_data, uint32_t data_size) { 00081 rbsp_sync_t sync_info; 00082 rbsp_data_conf_t data_conf; 00083 Semaphore * p_sem_wait = new Semaphore(0); 00084 00085 sync_info.result = -1; 00086 if (p_sem_wait != NULL) { 00087 sync_info.p_sem = p_sem_wait; 00088 00089 data_conf.p_notify_func = &callback_sync_trans; 00090 data_conf.p_app_data = &sync_info; 00091 00092 if (aio_trans(p_ctl, p_data, data_size, &data_conf) == ESUCCESS) { 00093 p_sem_wait->wait(osWaitForever); 00094 } 00095 delete p_sem_wait; 00096 } 00097 00098 return sync_info.result; 00099 } 00100 00101 /* static */ void R_BSP_Aio::callback_sync_trans(void * p_data, int32_t result, void * p_app_data) { 00102 rbsp_sync_t * p_sync_info = (rbsp_sync_t *)p_app_data; 00103 00104 p_sync_info->result = result; 00105 p_sync_info->p_sem->release(); 00106 } 00107 00108 /* static */ int32_t R_BSP_Aio::aio_trans(rbsp_serial_ctl_t * const p_ctl, 00109 void * const p_data, uint32_t data_size, const rbsp_data_conf_t * const p_data_conf) { 00110 int32_t wk_errno; 00111 AIOCB * p_rbsp_aio; 00112 rbsp_sival_t * p_sival; 00113 rbsp_read_write_a_func_t p_func = (rbsp_read_write_a_func_t)p_ctl->p_async_func; 00114 00115 if ((p_data_conf == NULL) || (p_data == NULL)) { 00116 wk_errno = ENOSPC; 00117 } else if (p_ctl->p_sem_ctl->wait(osWaitForever) == -1) { 00118 wk_errno = EIO; 00119 } else { 00120 p_rbsp_aio = (AIOCB *)p_ctl->p_aio_top + p_ctl->index; 00121 p_sival = p_ctl->p_sival_top + p_ctl->index; 00122 00123 p_sival->p_cb_func = p_data_conf->p_notify_func; 00124 p_sival->p_cb_data = p_data_conf->p_app_data; 00125 p_sival->p_sem = p_ctl->p_sem_ctl; 00126 p_sival->p_aio = p_rbsp_aio; 00127 00128 p_rbsp_aio->aio_fildes = 0; 00129 p_rbsp_aio->aio_buf = p_data; 00130 p_rbsp_aio->aio_nbytes = data_size; 00131 p_rbsp_aio->aio_offset = 0; 00132 p_rbsp_aio->aio_sigevent.sigev_notify = SIGEV_THREAD; 00133 p_rbsp_aio->aio_sigevent.sigev_value.sival_ptr = (void*)p_sival; 00134 p_rbsp_aio->aio_sigevent.sigev_notify_function = &callback_aio_trans; 00135 p_func(p_ctl->ch_handle, p_rbsp_aio, &wk_errno); 00136 00137 if (wk_errno == ESUCCESS) { 00138 if ((p_ctl->index + 1) >= p_ctl->MaxNum) { 00139 p_ctl->index = 0; 00140 } else { 00141 p_ctl->index++; 00142 } 00143 } else { 00144 p_ctl->p_sem_ctl->release(); 00145 } 00146 } 00147 00148 return wk_errno; 00149 } 00150 00151 /* static */ void R_BSP_Aio::callback_aio_trans(union sigval signo) { 00152 rbsp_sival_t * p_sival = (rbsp_sival_t *)signo.sival_ptr; 00153 AIOCB * p_aio_result = (AIOCB *)p_sival->p_aio; 00154 00155 if ((p_sival->p_cb_func != NULL) && (p_aio_result != NULL)) { 00156 p_sival->p_cb_func((void *)p_aio_result->aio_buf, p_aio_result->aio_return, p_sival->p_cb_data); 00157 } 00158 p_sival->p_sem->release(); 00159 }
Generated on Tue Jul 12 2022 20:43:58 by
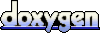