
Hello World for RGA(Renesas Graphics Architecture). RGA is the Graphics Library of RZ/A1. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependencies: GR-PEACH_video GraphicsFramework R_BSP LCD_shield_config
main.cpp
00001 /* 00002 Permission is hereby granted, free of charge, to any person obtaining a copy 00003 of this software and associated documentation files (the "Software"), to deal 00004 in the Software without restriction, including without limitation the rights 00005 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00006 copies of the Software, and to permit persons to whom the Software is 00007 furnished to do so, subject to the following conditions: 00008 00009 The above copyright notice and this permission notice shall be included in 00010 all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00013 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00014 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00015 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00016 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00018 THE SOFTWARE. 00019 */ 00020 00021 #include "mbed.h" 00022 #include "rga_func.h" 00023 #include "DisplayBace.h" 00024 #include "rtos.h" 00025 #include "LCD_shield_config_7_1inch.h" 00026 00027 #define GRAPHICS_FORMAT (DisplayBase::GRAPHICS_FORMAT_RGB565) 00028 #define WR_RD_WRSWA (DisplayBase::WR_RD_WRSWA_32_16BIT) 00029 #define TOUCH_NUM (1u) 00030 00031 /* FRAME BUFFER Parameter */ 00032 #define FRAME_BUFFER_BYTE_PER_PIXEL (2) 00033 #define FRAME_BUFFER_STRIDE (((LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL) + 31u) & ~31u) 00034 00035 #define DRAW_RECTANGLE_CNT_MAX (4) 00036 00037 typedef enum { 00038 RGA_FUNC_NON, 00039 RGA_FUNC_DRAW_RECTANGLE, 00040 RGA_FUNC_DRAW_IMAGE, 00041 RGA_FUNC_DISSOLVE, 00042 RGA_FUNC_SCROLL, 00043 RGA_FUNC_ZOOM, 00044 RGA_FUNC_ROTATION, 00045 RGA_FUNC_ACCELERATE, 00046 RGA_FUNC_ANIME_EASE, 00047 RGA_FUNC_ANIME_LINEAR, 00048 RGA_FUNC_ANIME_EASE_IN, 00049 RGA_FUNC_ANIME_EASE_OUT, 00050 RGA_FUNC_ANIME_EASE_IN_OUT, 00051 RGA_FUNC_RETURN, 00052 RGA_FUNC_END 00053 } func_code_t; 00054 00055 DigitalOut lcd_pwon(P7_15); 00056 DigitalOut lcd_blon(P8_1); 00057 PwmOut lcd_cntrst(P8_15); 00058 DisplayBase Display; 00059 00060 typedef struct { 00061 uint32_t pic_pos_x; /* X position of the key picture. */ 00062 uint32_t pic_pos_y; /* Y position of the key picture. */ 00063 uint32_t pic_width; /* Width of the key picture. */ 00064 uint32_t pic_height; /* Height of the key picture. */ 00065 func_code_t func_code; /* func code of the key picture. */ 00066 } key_pic_info_t; 00067 00068 #if defined(__ICCARM__) 00069 #pragma data_alignment=32 00070 static uint8_t user_frame_buffer1[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00071 #pragma data_alignment=32 00072 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]; 00073 #else 00074 static uint8_t user_frame_buffer1[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); /* 32 bytes aligned */ 00075 static uint8_t user_frame_buffer2[FRAME_BUFFER_STRIDE * LCD_PIXEL_HEIGHT]__attribute((aligned(32))); /* 32 bytes aligned */ 00076 #endif 00077 static frame_buffer_t frame_buffer_info; 00078 static volatile int32_t vsync_count = 0; 00079 00080 static const key_pic_info_t top_screen_key_tbl[] = { 00081 /* X Y Width Height Func code */ 00082 { 50, 350, 120, 52, RGA_FUNC_DRAW_RECTANGLE }, /* RGA Func1 */ 00083 { 230, 350, 120, 52, RGA_FUNC_DRAW_IMAGE }, /* RGA Func2 */ 00084 { 410, 350, 120, 52, RGA_FUNC_DISSOLVE }, /* RGA Func3 */ 00085 { 50, 420, 120, 52, RGA_FUNC_SCROLL }, /* RGA Func4 */ 00086 { 230, 420, 120, 52, RGA_FUNC_ZOOM }, /* RGA Func5 */ 00087 { 410, 420, 120, 52, RGA_FUNC_ROTATION }, /* RGA Func6 */ 00088 { 615, 420, 120, 52, RGA_FUNC_ACCELERATE }, /* RGA Func7 */ 00089 { 0, 0, 0, 0, RGA_FUNC_END } /* table end */ 00090 }; 00091 00092 static const key_pic_info_t return_key_tbl[] = { 00093 /* X Y Width Height Func code */ 00094 { 640, 10, 150, 84, RGA_FUNC_RETURN }, /* Return Top Screen */ 00095 { 0, 0, 0, 0, RGA_FUNC_END } /* table end */ 00096 }; 00097 00098 static const key_pic_info_t animetion_timing_key_tbl[] = { 00099 /* X Y Width Height Func code */ 00100 { 640, 10, 150, 84, RGA_FUNC_RETURN }, /* Return Top Screen */ 00101 { 17, 372, 136, 50, RGA_FUNC_ANIME_EASE }, /* ease */ 00102 { 173, 372, 136, 50, RGA_FUNC_ANIME_LINEAR }, /* linear */ 00103 { 330, 372, 136, 50, RGA_FUNC_ANIME_EASE_IN }, /* ease-in */ 00104 { 487, 372, 136, 50, RGA_FUNC_ANIME_EASE_OUT }, /* ease-out */ 00105 { 644, 372, 136, 50, RGA_FUNC_ANIME_EASE_IN_OUT }, /* ease-in-out */ 00106 { 0, 0, 0, 0, RGA_FUNC_END } /* table end */ 00107 }; 00108 00109 /****** LCD ******/ 00110 static void IntCallbackFunc_LoVsync(DisplayBase::int_type_t int_type) { 00111 /* Interrupt callback function for Vsync interruption */ 00112 if (vsync_count > 0) { 00113 vsync_count--; 00114 } 00115 } 00116 00117 static void Wait_Vsync(const int32_t wait_count) { 00118 /* Wait for the specified number of times Vsync occurs */ 00119 vsync_count = wait_count; 00120 while (vsync_count > 0) { 00121 /* Do nothing */ 00122 } 00123 } 00124 00125 static void Init_LCD_Display(void) { 00126 DisplayBase::graphics_error_t error; 00127 DisplayBase::lcd_config_t lcd_config; 00128 PinName lvds_pin[8] = { 00129 /* data pin */ 00130 P5_7, P5_6, P5_5, P5_4, P5_3, P5_2, P5_1, P5_0 00131 }; 00132 00133 lcd_pwon = 0; 00134 lcd_blon = 0; 00135 Thread::wait(100); 00136 lcd_pwon = 1; 00137 lcd_blon = 1; 00138 00139 Display.Graphics_Lvds_Port_Init(lvds_pin, 8); 00140 00141 /* Graphics initialization process */ 00142 lcd_config = LcdCfgTbl_LCD_shield; 00143 error = Display.Graphics_init(&lcd_config); 00144 if (error != DisplayBase::GRAPHICS_OK) { 00145 printf("Line %d, error %d\n", __LINE__, error); 00146 mbed_die(); 00147 } 00148 00149 /* Interrupt callback function setting (Vsync signal output from scaler 0) */ 00150 error = Display.Graphics_Irq_Handler_Set(DisplayBase::INT_TYPE_S0_LO_VSYNC, 0, IntCallbackFunc_LoVsync); 00151 if (error != DisplayBase::GRAPHICS_OK) { 00152 printf("Line %d, error %d\n", __LINE__, error); 00153 mbed_die(); 00154 } 00155 } 00156 00157 static void Start_LCD_Display(uint8_t * p_buf) { 00158 DisplayBase::rect_t rect; 00159 00160 rect.vs = 0; 00161 rect.vw = LCD_PIXEL_HEIGHT; 00162 rect.hs = 0; 00163 rect.hw = LCD_PIXEL_WIDTH; 00164 Display.Graphics_Read_Setting( 00165 DisplayBase::GRAPHICS_LAYER_0, 00166 (void *)p_buf, 00167 FRAME_BUFFER_STRIDE, 00168 GRAPHICS_FORMAT, 00169 WR_RD_WRSWA, 00170 &rect 00171 ); 00172 Display.Graphics_Start(DisplayBase::GRAPHICS_LAYER_0); 00173 } 00174 00175 static void Update_LCD_Display(frame_buffer_t * frmbuf_info) { 00176 Display.Graphics_Read_Change(DisplayBase::GRAPHICS_LAYER_0, 00177 (void *)frmbuf_info->buffer_address[frmbuf_info->draw_buffer_index]); 00178 Wait_Vsync(1); 00179 } 00180 00181 static void Swap_FrameBuffer(frame_buffer_t * frmbuf_info) { 00182 if (frmbuf_info->draw_buffer_index == 1) { 00183 frmbuf_info->draw_buffer_index = 0; 00184 } else { 00185 frmbuf_info->draw_buffer_index = 1; 00186 } 00187 } 00188 00189 /****** Touch ******/ 00190 static func_code_t Scan_Key(const key_pic_info_t * key_tbl, const uint32_t pos_x, const uint32_t pos_y) { 00191 func_code_t ret = RGA_FUNC_NON; 00192 00193 while (ret == RGA_FUNC_NON) { 00194 if (key_tbl->func_code == RGA_FUNC_END) { 00195 break; 00196 } 00197 /* Check the range of the X position */ 00198 if ((pos_x >= key_tbl->pic_pos_x) && (pos_x <= (key_tbl->pic_pos_x + key_tbl->pic_width))) { 00199 /* Check the range of the Y position */ 00200 if ((pos_y >= key_tbl->pic_pos_y) && (pos_y <= (key_tbl->pic_pos_y + key_tbl->pic_height))) { 00201 /* Decide the func code. */ 00202 ret = key_tbl->func_code; 00203 } 00204 } 00205 key_tbl++; 00206 } 00207 00208 return ret; 00209 } 00210 00211 /****** Efect ******/ 00212 static void Exe_RGA_Func(func_code_t func_name, frame_buffer_t* frmbuf_info, TouckKey_LCD_shield * p_touch) { 00213 uint8_t touch_num = 0; 00214 TouchKey::touch_pos_t touch_pos[TOUCH_NUM]; 00215 00216 switch (func_name) { 00217 case RGA_FUNC_DRAW_RECTANGLE: 00218 { 00219 bool key_on = false; 00220 int cnt; 00221 int color_cnt = 0; 00222 int x_0 = 0; 00223 int y_0 = 0; 00224 draw_rectangle_pos_t pos_tbl[DRAW_RECTANGLE_CNT_MAX] = {0}; 00225 00226 pos_tbl[0].style = "#FF0000"; /* red */ 00227 pos_tbl[1].style = "#00FF00"; /* green */ 00228 pos_tbl[2].style = "#0000FF"; /* blue */ 00229 pos_tbl[3].style = "#000000"; /* black */ 00230 00231 while (1) { 00232 /* Get coordinates */ 00233 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00234 if (touch_num != 0) { 00235 if (Scan_Key(return_key_tbl, touch_pos[0].x, touch_pos[0].y) == RGA_FUNC_RETURN) { 00236 break; 00237 } 00238 if (key_on == false) { 00239 key_on = true; 00240 if (color_cnt == 0) { 00241 for (cnt = 0; cnt < DRAW_RECTANGLE_CNT_MAX; cnt++) { 00242 pos_tbl[cnt].x = 0; 00243 pos_tbl[cnt].y = 0; 00244 pos_tbl[cnt].w = 0; 00245 pos_tbl[cnt].h = 0; 00246 } 00247 } 00248 x_0 = touch_pos[0].x; 00249 y_0 = touch_pos[0].y; 00250 } 00251 if (x_0 < touch_pos[0].x) { 00252 pos_tbl[color_cnt].x = x_0; 00253 pos_tbl[color_cnt].w = touch_pos[0].x - x_0; 00254 } else { 00255 pos_tbl[color_cnt].x = touch_pos[0].x; 00256 pos_tbl[color_cnt].w = x_0 - touch_pos[0].x; 00257 } 00258 if (y_0 < touch_pos[0].y) { 00259 pos_tbl[color_cnt].y = y_0; 00260 pos_tbl[color_cnt].h = touch_pos[0].y - y_0; 00261 } else { 00262 pos_tbl[color_cnt].y = touch_pos[0].y; 00263 pos_tbl[color_cnt].h = y_0 - touch_pos[0].y; 00264 } 00265 } else { 00266 if (key_on != false) { 00267 color_cnt++; 00268 if (color_cnt == DRAW_RECTANGLE_CNT_MAX) { 00269 color_cnt = 0; 00270 } 00271 } 00272 key_on = false; 00273 } 00274 /* Draw screen */ 00275 Swap_FrameBuffer(frmbuf_info); 00276 RGA_Func_DrawRectangle(frmbuf_info, pos_tbl, DRAW_RECTANGLE_CNT_MAX); 00277 Update_LCD_Display(frmbuf_info); 00278 } 00279 } 00280 break; 00281 case RGA_FUNC_DRAW_IMAGE: 00282 { 00283 int center_pos_x = 320; 00284 int center_pos_y = 110; 00285 while (1) { 00286 /* Get coordinates */ 00287 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00288 if (touch_num != 0) { 00289 if (Scan_Key(return_key_tbl, touch_pos[0].x, touch_pos[0].y) == RGA_FUNC_RETURN) { 00290 break; 00291 } 00292 center_pos_x = touch_pos[0].x; 00293 center_pos_y = touch_pos[0].y; 00294 } 00295 /* Draw screen */ 00296 Swap_FrameBuffer(frmbuf_info); 00297 RGA_Func_DrawImage(frmbuf_info, center_pos_x, center_pos_y); 00298 Update_LCD_Display(frmbuf_info); 00299 } 00300 } 00301 break; 00302 case RGA_FUNC_DISSOLVE: 00303 { 00304 float32_t work_alpha = 0.0f; 00305 while (1) { 00306 /* Get coordinates */ 00307 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00308 if (touch_num != 0) { 00309 if (Scan_Key(return_key_tbl, touch_pos[0].x, touch_pos[0].y) == RGA_FUNC_RETURN) { 00310 break; 00311 } 00312 work_alpha = (float32_t)touch_pos[0].x / (float32_t)(LCD_PIXEL_WIDTH); 00313 } 00314 /* Draw screen */ 00315 Swap_FrameBuffer(frmbuf_info); 00316 RGA_Func_Dissolve(frmbuf_info, work_alpha); 00317 Update_LCD_Display(frmbuf_info); 00318 } 00319 } 00320 break; 00321 case RGA_FUNC_SCROLL: 00322 { 00323 int work_width_pos = 0; 00324 while (1) { 00325 /* Get coordinates */ 00326 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00327 if (touch_num != 0) { 00328 if (Scan_Key(return_key_tbl, touch_pos[0].x, touch_pos[0].y) == RGA_FUNC_RETURN) { 00329 break; 00330 } 00331 work_width_pos = SCROLL_MAX_NUM * ((float32_t)touch_pos[0].x / (float32_t)(LCD_PIXEL_WIDTH)); 00332 } 00333 /* Draw screen */ 00334 Swap_FrameBuffer(frmbuf_info); 00335 RGA_Func_Scroll(frmbuf_info, work_width_pos); 00336 Update_LCD_Display(frmbuf_info); 00337 } 00338 } 00339 break; 00340 case RGA_FUNC_ZOOM: 00341 { 00342 int work_height_pos = ZOOM_MAX_NUM; 00343 while (1) { 00344 /* Get coordinates */ 00345 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00346 if (touch_num != 0) { 00347 if (Scan_Key(return_key_tbl, touch_pos[0].x, touch_pos[0].y) == RGA_FUNC_RETURN) { 00348 break; 00349 } 00350 work_height_pos = ZOOM_MAX_NUM * ((float32_t)touch_pos[0].x / (float32_t)(LCD_PIXEL_WIDTH)); 00351 } 00352 /* Draw screen */ 00353 Swap_FrameBuffer(frmbuf_info); 00354 RGA_Func_Zoom(frmbuf_info, work_height_pos); 00355 Update_LCD_Display(frmbuf_info); 00356 } 00357 } 00358 break; 00359 case RGA_FUNC_ROTATION: 00360 { 00361 graphics_matrix_float_t work_angle = 0; 00362 while (1) { 00363 /* Get coordinates */ 00364 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00365 if (touch_num != 0) { 00366 if (Scan_Key(return_key_tbl, touch_pos[0].x, touch_pos[0].y) == RGA_FUNC_RETURN) { 00367 break; 00368 } 00369 work_angle = ROTATION_MAX_NUM * ((float32_t)touch_pos[0].x / (float32_t)(LCD_PIXEL_WIDTH)); 00370 } 00371 /* Draw screen */ 00372 Swap_FrameBuffer(frmbuf_info); 00373 RGA_Func_Rotation(frmbuf_info, work_angle); 00374 Update_LCD_Display(frmbuf_info); 00375 } 00376 } 00377 break; 00378 case RGA_FUNC_ACCELERATE: 00379 { 00380 int acce_frame_num = 0; 00381 int animation_timing = 0; 00382 float32_t work_relative_pos; 00383 while (1) { 00384 /* Get coordinates */ 00385 touch_num = p_touch->GetCoordinates(TOUCH_NUM, touch_pos); 00386 if (touch_num != 0) { 00387 func_code_t func_code; 00388 00389 func_code = Scan_Key(animetion_timing_key_tbl, touch_pos[0].x, touch_pos[0].y); 00390 if (func_code == RGA_FUNC_RETURN) { 00391 break; 00392 } 00393 switch (func_code) { 00394 case RGA_FUNC_ANIME_EASE: 00395 animation_timing = 0; 00396 acce_frame_num = 0; 00397 break; 00398 case RGA_FUNC_ANIME_LINEAR: 00399 animation_timing = 1; 00400 acce_frame_num = 0; 00401 break; 00402 case RGA_FUNC_ANIME_EASE_IN: 00403 animation_timing = 2; 00404 acce_frame_num = 0; 00405 break; 00406 case RGA_FUNC_ANIME_EASE_OUT: 00407 animation_timing = 3; 00408 acce_frame_num = 0; 00409 break; 00410 case RGA_FUNC_ANIME_EASE_IN_OUT: 00411 animation_timing = 4; 00412 acce_frame_num = 0; 00413 break; 00414 default: 00415 /* Do Nothing */ 00416 break; 00417 } 00418 } 00419 work_relative_pos = acce_frame_num / (float32_t)ACCELERATE_MAX_NUM; 00420 acce_frame_num++; 00421 if (acce_frame_num > ACCELERATE_MAX_NUM) { 00422 acce_frame_num = 0; 00423 } 00424 /* Draw screen */ 00425 Swap_FrameBuffer(frmbuf_info); 00426 RGA_Func_Accelerate(frmbuf_info, animation_timing, work_relative_pos); 00427 Update_LCD_Display(frmbuf_info); 00428 } 00429 } 00430 break; 00431 default : 00432 /* Do nothing */ 00433 break; 00434 } 00435 } 00436 00437 int main(void) { 00438 func_code_t func_code; 00439 uint8_t touch_num = 0; 00440 TouchKey::touch_pos_t touch_pos[TOUCH_NUM]; 00441 00442 /* Initialization of LCD */ 00443 Init_LCD_Display(); /* When using LCD, please call before than Init_Video(). */ 00444 00445 memset(user_frame_buffer1, 0, sizeof(user_frame_buffer1)); 00446 memset(user_frame_buffer2, 0, sizeof(user_frame_buffer2)); 00447 frame_buffer_info.buffer_address[0] = user_frame_buffer1; 00448 frame_buffer_info.buffer_address[1] = user_frame_buffer2; 00449 frame_buffer_info.buffer_count = 2; 00450 frame_buffer_info.show_buffer_index = 0; 00451 frame_buffer_info.draw_buffer_index = 0; 00452 frame_buffer_info.width = LCD_PIXEL_WIDTH; 00453 frame_buffer_info.byte_per_pixel = FRAME_BUFFER_BYTE_PER_PIXEL; 00454 frame_buffer_info.stride = LCD_PIXEL_WIDTH * FRAME_BUFFER_BYTE_PER_PIXEL; 00455 frame_buffer_info.height = LCD_PIXEL_HEIGHT; 00456 frame_buffer_info.pixel_format = PIXEL_FORMAT_RGB565; 00457 00458 /* Display Top Screen */ 00459 Set_RGAObject(&frame_buffer_info); 00460 RGA_Func_DrawTopScreen(&frame_buffer_info); 00461 00462 /* Start of LCD */ 00463 Start_LCD_Display(frame_buffer_info.buffer_address[0]); 00464 00465 /* Backlight on */ 00466 Thread::wait(200); 00467 lcd_cntrst.write(1.0); 00468 00469 /* Reset touch IC */ 00470 TouckKey_LCD_shield touch(P4_0, P2_13, I2C_SDA, I2C_SCL); 00471 touch.Reset(); 00472 00473 while (1) { 00474 /* Get Coordinates */ 00475 touch_num = touch.GetCoordinates(TOUCH_NUM, touch_pos); 00476 if (touch_num != 0) { 00477 func_code = Scan_Key(top_screen_key_tbl, touch_pos[0].x, touch_pos[0].y); 00478 if (func_code != RGA_FUNC_NON) { 00479 /* Wait key off */ 00480 while (1) { 00481 touch_num = touch.GetCoordinates(TOUCH_NUM, touch_pos); 00482 if (touch_num == 0) { 00483 break; 00484 } 00485 Thread::wait(20); 00486 } 00487 00488 /* Execute RGA functions */ 00489 Exe_RGA_Func(func_code, &frame_buffer_info, &touch); 00490 00491 /* Return Top Screen */ 00492 Swap_FrameBuffer(&frame_buffer_info); 00493 RGA_Func_DrawTopScreen(&frame_buffer_info); 00494 Update_LCD_Display(&frame_buffer_info); 00495 } 00496 } 00497 Thread::wait(20); 00498 } 00499 }
Generated on Sat Jul 16 2022 00:32:54 by
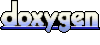