Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdec_register.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdec_register.c 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDEC driver register setup processing 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include "r_vdec.h" 00035 #include "r_vdec_user.h" 00036 #include "r_vdec_register.h" 00037 00038 00039 /****************************************************************************** 00040 Macro definitions 00041 ******************************************************************************/ 00042 /* shift value */ 00043 #define VEDC_REG_SHIFT_15 (15u) 00044 #define VEDC_REG_SHIFT_14 (14u) 00045 #define VEDC_REG_SHIFT_13 (13u) 00046 #define VEDC_REG_SHIFT_12 (12u) 00047 #define VEDC_REG_SHIFT_11 (11u) 00048 #define VEDC_REG_SHIFT_10 (10u) 00049 #define VEDC_REG_SHIFT_9 (9u) 00050 #define VEDC_REG_SHIFT_8 (8u) 00051 #define VEDC_REG_SHIFT_6 (6u) 00052 #define VEDC_REG_SHIFT_5 (5u) 00053 #define VEDC_REG_SHIFT_4 (4u) 00054 #define VEDC_REG_SHIFT_2 (2u) 00055 #define VEDC_REG_SHIFT_1 (1u) 00056 00057 /* bit set pattern */ 00058 #define VDEC_REG_SET_0X8000 (0x8000u) 00059 #define VDEC_REG_SET_0X4000 (0x4000u) 00060 #define VDEC_REG_SET_0X2000 (0x2000u) 00061 #define VDEC_REG_SET_0X1000 (0x1000u) 00062 #define VDEC_REG_SET_0X0800 (0x0800u) 00063 #define VDEC_REG_SET_0X0100 (0x0100u) 00064 #define VDEC_REG_SET_0X0080 (0x0080u) 00065 #define VDEC_REG_SET_0X0020 (0x0020u) 00066 #define VDEC_REG_SET_0X0010 (0x0010u) 00067 #define VDEC_REG_SET_0X0008 (0x0008u) 00068 #define VDEC_REG_SET_0X0004 (0x0004u) 00069 #define VDEC_REG_SET_0X0002 (0x0002u) 00070 #define VDEC_REG_SET_0X0001 (0x0001u) 00071 00072 /* bit mask pattern */ 00073 #define VDEC_REG_BIT_MASK_0X8000 (0x8000u) 00074 #define VDEC_REG_BIT_MASK_0X4000 (0x4000u) 00075 #define VDEC_REG_BIT_MASK_0X2000 (0x2000u) 00076 #define VDEC_REG_BIT_MASK_0X1000 (0x1000u) 00077 #define VDEC_REG_BIT_MASK_0X0800 (0x0800u) 00078 #define VDEC_REG_BIT_MASK_0X0400 (0x0400u) 00079 #define VDEC_REG_BIT_MASK_0X0200 (0x0200u) 00080 #define VDEC_REG_BIT_MASK_0X0100 (0x0100u) 00081 00082 /* register mask value */ 00083 #define VDEC_REG_MASK_0X03FF (0x03FFu) /* mask vdec_reg->syncssr */ 00084 #define VDEC_REG_MASK_0XFF1F (0xFF1Fu) /* mask vdec_reg->ycscr7 */ 00085 #define VDEC_REG_MASK_0XF3FF (0xF3FFu) /* mask vdec_reg->hafccr1 */ 00086 #define VDEC_REG_MASK_0X83FF (0x83FFu) /* mask vdec_reg->dcpcr1 */ 00087 #define VDEC_REG_MASK_0X01FF (0x01FFu) /* mask vdec_reg->ycscr11 */ 00088 #define VDEC_REG_MASK_0X1C00 (0x1C00u) /* mask vdec_reg->dcpcr9 */ 00089 #define VDEC_REG_MASK_0X1F00 (0x1F00u) /* mask vdec_reg->pgacr */ 00090 #define VDEC_REG_MASK_0X3F00 (0x3F00u) /* mask vdec_reg->agccr2 */ 00091 #define VDEC_REG_MASK_0X0007 (0x0007u) /* mask vdec_reg->rgorcr7 */ 00092 #define VDEC_REG_MASK_0XF800 (0xF800u) /* mask vdec_reg->ycscr8 */ 00093 #define VDEC_REG_MASK_0XFC00 (0xFC00u) /* mask vdec_reg->synscr1 */ 00094 #define VDEC_REG_MASK_0X00FF (0x00FFu) /* mask vdec_reg->synscr1 */ 00095 #define VDEC_REG_MASK_0X0003 (0x0003u) /* mask vdec_reg->cromasr1 */ 00096 00097 /****************************************************************************** 00098 Typedef definitions 00099 ******************************************************************************/ 00100 00101 /****************************************************************************** 00102 Private global variables and functions 00103 ******************************************************************************/ 00104 static void NoiseReductionLPF( 00105 const vdec_reg_address_t * const vdec_reg, 00106 const vdec_noise_rd_lpf_t * const p_noise_rd_lpf); 00107 static void SyncSlicer(const vdec_reg_address_t * const vdec_reg, const vdec_sync_slicer_t * const p_sync_slicer); 00108 static void HorizontalAFC( 00109 const vdec_reg_address_t * const vdec_reg, 00110 const vdec_horizontal_afc_t * const p_horizontal_afc); 00111 static void VerticalCountdown( 00112 const vdec_reg_address_t * const vdec_reg, 00113 const vdec_vcount_down_t * const p_vcount_down); 00114 static void AgcPga(const vdec_reg_address_t * const vdec_reg, const vdec_agc_t * const p_agc); 00115 static void PeakLimiterControl( 00116 const vdec_reg_address_t * const vdec_reg, 00117 const vdec_peak_limiter_t * const p_peak_limiter); 00118 static void OverRangeControl(const vdec_reg_address_t * const vdec_reg, const vdec_over_range_t * const p_over_range); 00119 static void YcSeparationControl( 00120 const vdec_reg_address_t * const vdec_reg, 00121 const vdec_yc_sep_ctrl_t * const p_yc_sep_ctrl); 00122 static void FilterTAPsCoefficient( 00123 volatile uint16_t * const * fil_reg_address, 00124 const vdec_chrfil_tap_t * const fil2_2d); 00125 00126 00127 /**************************************************************************//** 00128 * @brief Sets registers for initialization 00129 * @param[in] ch : Channel 00130 * @param[in] vinsel : Input pin control 00131 * @retval None 00132 *****************************************************************************/ 00133 void VDEC_Initialize (const vdec_channel_t ch, const vdec_adc_vinsel_t vinsel) 00134 { 00135 const vdec_reg_address_t * vdec_reg; 00136 uint16_t reg_data; 00137 00138 vdec_reg = &vdec_reg_address[ch]; 00139 00140 /* Input pin control */ 00141 if (vinsel == VDEC_ADC_VINSEL_VIN1 ) { 00142 reg_data = (uint16_t)((uint32_t)*(vdec_reg->adccr2) & (~0x0001u)); 00143 *(vdec_reg->adccr2) = reg_data; 00144 } else { 00145 reg_data = (uint16_t)((uint32_t)*(vdec_reg->adccr2) | (0x0001u)); 00146 *(vdec_reg->adccr2) = reg_data; 00147 } 00148 return; 00149 } /* End of function VDEC_Initialize() */ 00150 00151 /**************************************************************************//** 00152 * @brief Sets registers for active image period 00153 * @param[in] ch : Channel 00154 * @param[in] param : Active image period parameter 00155 * @retval None 00156 *****************************************************************************/ 00157 void VDEC_ActivePeriod (const vdec_channel_t ch, const vdec_active_period_t * const param) 00158 { 00159 const vdec_reg_address_t * vdec_reg; 00160 00161 vdec_reg = &vdec_reg_address[ch]; 00162 00163 /* Left end of input video signal capturing area */ 00164 *(vdec_reg->tgcr1) = param->srcleft ; 00165 /* Top end of input video signal capturing area 00166 and height of input video signal capturing area */ 00167 *(vdec_reg->tgcr2) = (uint16_t)(((uint32_t)param->srctop << VEDC_REG_SHIFT_10) | (uint32_t)param->srcheight ); 00168 /* Width of input video signal capturing area */ 00169 *(vdec_reg->tgcr3) = param->srcwidth ; 00170 00171 return; 00172 } /* End of function VDEC_ActivePeriod() */ 00173 00174 /**************************************************************************//** 00175 * @brief Sets registers for sync separation 00176 * @param[in] ch : Channel 00177 * @param[in] param : Sync separation parameter 00178 * @retval None 00179 *****************************************************************************/ 00180 void VDEC_SyncSeparation (const vdec_channel_t ch, const vdec_sync_separation_t * const param) 00181 { 00182 const vdec_reg_address_t * vdec_reg; 00183 00184 vdec_reg = &vdec_reg_address[ch]; 00185 00186 /* Noise reduction LPF */ 00187 NoiseReductionLPF(vdec_reg, param->noise_rd_lpf ); 00188 /* Auto level control sync slicer */ 00189 SyncSlicer(vdec_reg, param->sync_slicer ); 00190 /* Horizontal AFC */ 00191 HorizontalAFC(vdec_reg, param->horizontal_afc ); 00192 /* Vertical count-down */ 00193 VerticalCountdown(vdec_reg, param->vcount_down ); 00194 /* AGC/PGA */ 00195 AgcPga(vdec_reg, param->agc ); 00196 /* Peak limiter control */ 00197 PeakLimiterControl(vdec_reg, param->peak_limiter ); 00198 00199 return; 00200 } /* End of function VDEC_SyncSeparation() */ 00201 00202 /**************************************************************************//** 00203 * @brief Sets registers for Y/C separation 00204 * @param[in] ch : Channel 00205 * @param[in] param : Y/C separation parameter 00206 * @retval None 00207 *****************************************************************************/ 00208 void VDEC_YcSeparation (const vdec_channel_t ch, const vdec_yc_separation_t * const param) 00209 { 00210 const vdec_reg_address_t * vdec_reg; 00211 00212 vdec_reg = &vdec_reg_address[ch]; 00213 00214 /* Over-range control */ 00215 OverRangeControl(vdec_reg, param->over_range ); 00216 /* Y/C separation control */ 00217 YcSeparationControl(vdec_reg, param->yc_sep_ctrl ); 00218 00219 /* Two-dimensional cascade broadband (3.58/4.43/SECAM-DR)/TAKE-OFF filter TAP coefficient */ 00220 FilterTAPsCoefficient(vdec_filter_reg_address[ch].yctwa_f, param->fil2_2d_wa ); 00221 /* Two-dimensional cascade broadband (SECAM-DB) filter TAP coefficient */ 00222 FilterTAPsCoefficient(vdec_filter_reg_address[ch].yctwb_f, param->fil2_2d_wb ); 00223 /* Two-dimensional cascade narrowband (3.58/4.43/SECAM-DR) filter TAP coefficient */ 00224 FilterTAPsCoefficient(vdec_filter_reg_address[ch].yctna_f, param->fil2_2d_na ); 00225 /* Two-dimensional cascade narrowband (SECAMDB) filter TAP coefficient */ 00226 FilterTAPsCoefficient(vdec_filter_reg_address[ch].yctnb_f, param->fil2_2d_nb ); 00227 00228 return; 00229 } /* End of function VDEC_YcSeparation() */ 00230 00231 /**************************************************************************//** 00232 * @brief Sets registers for chroma decoding 00233 * @param[in] ch : Channel 00234 * @param[in] param : Chroma decoding parameter 00235 * @retval None 00236 *****************************************************************************/ 00237 void VDEC_ChromaDecoding (const vdec_channel_t ch, const vdec_chroma_decoding_t * const param) 00238 { 00239 const vdec_reg_address_t * vdec_reg; 00240 vdec_chrmdec_ctrl_t * p_chrmdec_ctrl; 00241 vdec_burst_lock_t * p_burst_lock; 00242 vdec_acc_t * p_acc; 00243 vdec_tint_ry_t * p_tint_ry; 00244 uint32_t reg_data; 00245 00246 vdec_reg = &vdec_reg_address[ch]; 00247 p_chrmdec_ctrl = param->chrmdec_ctrl ; 00248 p_burst_lock = param->burst_lock ; 00249 p_acc = param->acc ; 00250 p_tint_ry = param->tint_ry ; 00251 00252 /* Color system detection */ 00253 if (p_chrmdec_ctrl != NULL) { 00254 reg_data = (uint32_t)*(vdec_reg->btlcr) & (uint32_t)(~VDEC_REG_MASK_0X00FF); 00255 /* Default color system */ 00256 reg_data |= (uint32_t)p_chrmdec_ctrl->defaultsys << VEDC_REG_SHIFT_6; 00257 /* NTSC-M detection control */ 00258 reg_data |= (p_chrmdec_ctrl->nontsc358_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X0020 : (uint32_t)0x0000u; 00259 /* NTSC-4.43 detection control */ 00260 reg_data |= (p_chrmdec_ctrl->nontsc443_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X0010 : (uint32_t)0x0000u; 00261 /* PAL-M detection control */ 00262 reg_data |= (p_chrmdec_ctrl->nopalm_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X0008 : (uint32_t)0x0000u; 00263 /* PAL-N detection control */ 00264 reg_data |= (p_chrmdec_ctrl->nopaln_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X0004 : (uint32_t)0x0000u; 00265 /* PAL-B, G, H, I, D detection control */ 00266 reg_data |= (p_chrmdec_ctrl->nopal443_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X0002 : (uint32_t)0x0000u; 00267 /* SECAM detection control */ 00268 reg_data |= (p_chrmdec_ctrl->nosecam_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X0001 : (uint32_t)0x0000u; 00269 00270 *(vdec_reg->btlcr) = (uint16_t)reg_data; 00271 00272 /* Luminance signal delay adjustment */ 00273 reg_data = (uint32_t)p_chrmdec_ctrl->lumadelay << VEDC_REG_SHIFT_4; 00274 /* LPF for demodulated chroma */ 00275 reg_data |= (p_chrmdec_ctrl->chromalpf == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0004; 00276 /* Averaging processing for pre-demodulated line */ 00277 reg_data |= (uint32_t)p_chrmdec_ctrl->demodmode ; 00278 00279 *(vdec_reg->ycdcr) = (uint16_t)reg_data; 00280 } 00281 /* BCO */ 00282 if (p_burst_lock != NULL) { 00283 reg_data = (uint32_t)*(vdec_reg->btlcr) & (uint32_t)(~VDEC_REG_MASK_0XFC00); 00284 /* Burst lock PLL lock range */ 00285 reg_data |= (uint32_t)p_burst_lock->lockrange << VEDC_REG_SHIFT_14; 00286 /* Burst lock PLL loop gain */ 00287 reg_data |= (uint32_t)p_burst_lock->loopgain << VEDC_REG_SHIFT_12; 00288 /* Level for burst lock PLL to re-search free-run frequency */ 00289 reg_data |= (uint32_t)p_burst_lock->locklimit << VEDC_REG_SHIFT_10; 00290 00291 *(vdec_reg->btlcr) = (uint16_t)reg_data; 00292 00293 /* burst gate pulse position check */ 00294 reg_data = (uint32_t)p_burst_lock->bgpcheck << VEDC_REG_SHIFT_15; 00295 /* burst gate pulse width */ 00296 reg_data |= (uint32_t)p_burst_lock->bgpwidth << VEDC_REG_SHIFT_8; 00297 /* burst gate pulse start position */ 00298 reg_data |= (uint32_t)p_burst_lock->bgpstart ; 00299 00300 *(vdec_reg->btgpcr) = (uint16_t)reg_data; 00301 } 00302 /* ACC and color killer */ 00303 if (p_acc != NULL) { 00304 /* ACC operating mode */ 00305 reg_data = (p_acc->accmode == VDEC_ACC_MD_AUTO ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0800; 00306 /* Maximum ACC Gain */ 00307 reg_data |= (uint32_t)p_acc->accmaxgain << VEDC_REG_SHIFT_9; 00308 /* ACC reference color burst amplitude */ 00309 reg_data |= (uint32_t)p_acc->acclevel ; 00310 /* Color killer offset */ 00311 reg_data |= (uint32_t)p_acc->killeroffset << VEDC_REG_SHIFT_12; 00312 00313 *(vdec_reg->acccr1) = (uint16_t)reg_data; 00314 00315 /* Chroma manual gain (sub) */ 00316 reg_data = (uint32_t)p_acc->chromasubgain << VEDC_REG_SHIFT_9; 00317 /* Chroma manual gain (main) */ 00318 reg_data |= (uint32_t)p_acc->chromamaingain ; 00319 00320 *(vdec_reg->acccr2) = (uint16_t)reg_data; 00321 00322 /* ACC response speed */ 00323 reg_data = (uint32_t)p_acc->accresponse << VEDC_REG_SHIFT_14; 00324 /* ACC gain adjustment accuracy */ 00325 reg_data |= (uint32_t)p_acc->accprecis << VEDC_REG_SHIFT_8; 00326 /* Forced color killer mode ON/OFF */ 00327 reg_data |= (p_acc->killermode == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0080; 00328 /* Color killer operation start point */ 00329 reg_data |= (uint32_t)p_acc->killerlevel << VEDC_REG_SHIFT_1; 00330 00331 *(vdec_reg->acccr3) = (uint16_t)reg_data; 00332 } 00333 /* TINT correction/R-Y axis correction (only valid for NTSC/PAL) */ 00334 if (p_tint_ry != NULL) { 00335 /* Fine adjustment of R-Y demodulation axis and hue adjustment level */ 00336 reg_data = (uint32_t)p_tint_ry->tintsub << VEDC_REG_SHIFT_10; 00337 reg_data |= (uint32_t)p_tint_ry->tintmain ; 00338 00339 *(vdec_reg->tintcr) = (uint16_t)reg_data; 00340 } 00341 return; 00342 } /* End of function VDEC_ChromaDecoding() */ 00343 00344 /**************************************************************************//** 00345 * @brief Sets registers for digital clamp 00346 * @param[in] ch : Channel 00347 * @param[in] param : Digital clamp parameter 00348 * @retval None 00349 *****************************************************************************/ 00350 void VDEC_DigitalClamp (const vdec_channel_t ch, const vdec_degital_clamp_t * const param) 00351 { 00352 const vdec_reg_address_t * vdec_reg; 00353 vdec_pedestal_clamp_t * p_pedestal_clamp; 00354 vdec_center_clamp_t * p_center_clamp; 00355 vdec_noise_det_t * p_noise_det; 00356 uint32_t reg_data; 00357 00358 vdec_reg = &vdec_reg_address[ch]; 00359 p_pedestal_clamp = param->pedestal_clamp ; 00360 p_center_clamp = param->center_clamp ; 00361 p_noise_det = param->noise_det ; 00362 00363 /* Digital clamp pulse position check */ 00364 reg_data = (uint32_t)*(vdec_reg->dcpcr1); 00365 if (param->dcpcheck == VDEC_OFF ) { 00366 reg_data &= (uint32_t)(~VDEC_REG_SET_0X0800); 00367 } else { 00368 reg_data |= (uint32_t)VDEC_REG_SET_0X0800; 00369 } 00370 *(vdec_reg->dcpcr1) = (uint16_t)reg_data; 00371 00372 /* Digital clamp response speed */ 00373 *(vdec_reg->dcpcr3) = (uint16_t)((uint32_t)param->dcpresponse << VEDC_REG_SHIFT_12); 00374 /* Digital clamp start line */ 00375 *(vdec_reg->dcpcr4) = (uint16_t)((uint32_t)param->dcpstart << VEDC_REG_SHIFT_10); 00376 /* Digital clamp end line */ 00377 *(vdec_reg->dcpcr5) = (uint16_t)((uint32_t)param->dcpend << VEDC_REG_SHIFT_10); 00378 /* Digital clamp pulse width */ 00379 *(vdec_reg->dcpcr6) = (uint16_t)((uint32_t)param->dcpwidth << VEDC_REG_SHIFT_8); 00380 00381 /* Pedestal clamp */ 00382 if (p_pedestal_clamp != NULL) { 00383 reg_data = (uint32_t)*(vdec_reg->dcpcr1) & (uint32_t)(~VDEC_REG_MASK_0X83FF); 00384 /* Clamp level setting mode (Y signal) */ 00385 reg_data |= (p_pedestal_clamp->dcpmode_y == VDEC_DCPMODE_MANUAL ) ? (uint32_t)0x0000u : 00386 (uint32_t)VDEC_REG_SET_0X8000; 00387 /* Clamp offset level (Y signal) */ 00388 reg_data |= (uint32_t)p_pedestal_clamp->blanklevel_y ; 00389 00390 *(vdec_reg->dcpcr1) = (uint16_t)reg_data; 00391 00392 /* Digital clamp pulse horizontal start position (Y signal) */ 00393 *(vdec_reg->dcpcr7) = (uint16_t)((uint32_t)p_pedestal_clamp->dcppos_y << VEDC_REG_SHIFT_8); 00394 } 00395 /* Center clamp */ 00396 if (p_center_clamp != NULL) { 00397 /* Clamp level setting mode (Cb/Cr signal) */ 00398 reg_data = (p_center_clamp->dcpmode_c == VDEC_DCPMODE_MANUAL ) ? (uint32_t)0x0000u : 00399 (uint32_t)VDEC_REG_SET_0X8000; 00400 /* Clamp offset level (Cb signal) */ 00401 reg_data |= (uint32_t)p_center_clamp->blanklevel_cb << VEDC_REG_SHIFT_6; 00402 /* Clamp offset level (Cr signal) */ 00403 reg_data |= (uint32_t)p_center_clamp->blanklevel_cr ; 00404 00405 *(vdec_reg->dcpcr2) = (uint16_t)reg_data; 00406 00407 /* Digital clamp pulse horizontal start position (Cb/Cr signal) */ 00408 *(vdec_reg->dcpcr8) = (uint16_t)((uint32_t)p_center_clamp->dcppos_c << VEDC_REG_SHIFT_8); 00409 } 00410 /* Noise detection */ 00411 if (p_noise_det != NULL) { 00412 /* Video signal for autocorrelation function */ 00413 reg_data = (uint32_t)p_noise_det->acfinput << VEDC_REG_SHIFT_12; 00414 /* Delay time for autocorrelation function calculation */ 00415 reg_data |= (uint32_t)p_noise_det->acflagtime << VEDC_REG_SHIFT_4; 00416 /* Smoothing parameter of autocorrelation function data */ 00417 reg_data |= (uint32_t)p_noise_det->acffilter ; 00418 00419 *(vdec_reg->nsdcr) = (uint16_t)reg_data; 00420 } 00421 /* Clamp data hold processing (Y, Cb, Cr) OFF */ 00422 reg_data = (uint32_t)*(vdec_reg->dcpcr9) & (uint32_t)(~VDEC_REG_MASK_0X1C00); 00423 *(vdec_reg->dcpcr9) = (uint16_t)reg_data; 00424 00425 return; 00426 } /* End of function VDEC_DigitalClamp() */ 00427 00428 /**************************************************************************//** 00429 * @brief Sets registers for output adjustment 00430 * @param[in] ch : Channel 00431 * @param[in] param : Output adjustment parameter 00432 * @retval None 00433 *****************************************************************************/ 00434 void VDEC_Output (const vdec_channel_t ch, const vdec_output_t * const param) 00435 { 00436 const vdec_reg_address_t * vdec_reg; 00437 00438 vdec_reg = &vdec_reg_address[ch]; 00439 00440 /* Y, Cb and Cr signal gain coefficient */ 00441 *(vdec_reg->ygaincr) = param->y_gain2 ; 00442 *(vdec_reg->cbgaincr) = param->cb_gain2 ; 00443 *(vdec_reg->crgaincr) = param->cr_gain2 ; 00444 00445 return; 00446 } /* End of function VDEC_Output() */ 00447 00448 /**************************************************************************//** 00449 * @brief Query VDEC parameters 00450 * @param[in] ch : Channel 00451 * @param[out] q_sync_sep : Sync separation parameters 00452 * @param[out] q_agc : Agc parameters 00453 * @param[out] q_chroma_dec : Chroma decoding parameters 00454 * @param[out] q_digital_clamp : Digital clamp parameters 00455 * @retval None 00456 *****************************************************************************/ 00457 void VDEC_Query ( 00458 const vdec_channel_t ch, 00459 vdec_q_sync_sep_t * const q_sync_sep, 00460 vdec_q_agc_t * const q_agc, 00461 vdec_q_chroma_dec_t * const q_chroma_dec, 00462 vdec_q_digital_clamp_t * const q_digital_clamp) 00463 { 00464 const vdec_reg_address_t * vdec_reg; 00465 uint32_t reg_value; 00466 00467 vdec_reg = &vdec_reg_address[ch]; 00468 00469 /* Sync separation */ 00470 if (q_sync_sep != NULL) { 00471 reg_value = (uint32_t)*(vdec_reg->vsyncsr); 00472 /* Horizontal AFC lock detection result */ 00473 q_sync_sep->fhlock = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X4000) == 0u) ? VDEC_UNLOCK : VDEC_LOCK ; 00474 /* Detection result of low S/N signal by sync separation */ 00475 q_sync_sep->isnoisy = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X2000) == 0u) ? VDEC_NO : VDEC_YES ; 00476 /* Speed detection result */ 00477 q_sync_sep->fhmode = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X1000) == 0u) ? VDEC_FHMD_NORMAL : 00478 VDEC_FHMD_MULTIPLIED ; 00479 /* No-signal detection result */ 00480 q_sync_sep->nosignal_ = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0800) == 0u) ? VDEC_YES : VDEC_NO ; 00481 /* Vertical countdown lock detection result */ 00482 q_sync_sep->fvlock = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0400) == 0u) ? VDEC_UNLOCK : VDEC_LOCK ; 00483 /* Vertical countdown oscillation mode */ 00484 q_sync_sep->fvmode = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0200) == 0u) ? VDEC_FVMD_50HZ : 00485 VDEC_FVMD_60HZ ; 00486 /* Interlace detection result */ 00487 q_sync_sep->interlaced = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0100) == 0u) ? VDEC_NO : VDEC_YES ; 00488 /* Vertical cycle measurement result */ 00489 q_sync_sep->fvcount = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X00FF); 00490 /* Horizontal AFC oscillation cycle */ 00491 q_sync_sep->fhcount = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X8000) == 0u) ? 0x0000u : (uint32_t)0x0001u; 00492 q_sync_sep->fhcount |= (uint32_t)*(vdec_reg->hsyncsr) << VEDC_REG_SHIFT_1; 00493 00494 reg_value = (uint32_t)*(vdec_reg->syncssr); 00495 /* Sync amplitude detection result during VBI period */ 00496 q_sync_sep->isreduced = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X1000) == 0u) ? VDEC_NO : VDEC_YES ; 00497 /* Sync pulse amplitude detection result */ 00498 q_sync_sep->syncdepth = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X03FF); 00499 } 00500 /* Agc */ 00501 if (q_agc != NULL) { 00502 reg_value = (uint32_t)*(vdec_reg->agccsr1); 00503 /* Number of pixels which have larger luminance value than peak luminance limited by peak limiter */ 00504 q_agc->highsamples = (uint16_t)(reg_value >> VEDC_REG_SHIFT_8); 00505 /* Number of overflowing pixels */ 00506 q_agc->peaksamples = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X00FF); 00507 00508 reg_value = (uint32_t)*(vdec_reg->agccsr2); 00509 /* AGC convergence detection result */ 00510 if ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0100) == 0u) { 00511 q_agc->agcconverge = (uint16_t)0x0000u; 00512 } else { 00513 q_agc->agcconverge = (uint16_t)0x0001u; 00514 } 00515 /* Current AGC gain value */ 00516 q_agc->agcgain = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X00FF); 00517 } 00518 /* Chroma decoding */ 00519 if (q_chroma_dec != NULL) { 00520 reg_value = (uint32_t)*(vdec_reg->cromasr1); 00521 /* Color system detection result */ 00522 q_chroma_dec->colorsys = (vdec_color_sys_t )(reg_value >> VEDC_REG_SHIFT_14); 00523 /* Color sub-carrier frequency detection result */ 00524 q_chroma_dec->fscmode = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X2000) == 0u) ? VDEC_FSCMD_3_58 : 00525 VDEC_FSCMD_4_43 ; 00526 /* Burst lock PLL lock state detection result */ 00527 q_chroma_dec->fsclock = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X1000) == 0u) ? VDEC_UNLOCK : VDEC_LOCK ; 00528 /* Color burst detection result */ 00529 q_chroma_dec->noburst_ = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0800) == 0u) ? VDEC_YES : VDEC_NO ; 00530 /* Current ACC gain value (sub) */ 00531 q_chroma_dec->accsubgain = (vdec_chrm_subgain_t )((reg_value >> VEDC_REG_SHIFT_9) & 00532 (uint32_t)VDEC_REG_MASK_0X0003); 00533 /* Current ACC gain value (main) */ 00534 q_chroma_dec->accmaingain = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X01FF); 00535 00536 reg_value = (uint32_t)*(vdec_reg->cromasr2); 00537 /* SECAM detection result */ 00538 q_chroma_dec->issecam = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X1000) == 0u) ? VDEC_NO : VDEC_YES ; 00539 /* PAL detection result */ 00540 q_chroma_dec->ispal = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0800) == 0u) ? VDEC_NO : VDEC_YES ; 00541 /* NTSC detection result */ 00542 q_chroma_dec->isntsc = ((reg_value & (uint32_t)VDEC_REG_BIT_MASK_0X0400) == 0u) ? VDEC_NO : VDEC_YES ; 00543 /* Low S/N signal detection result by burst lock PLL */ 00544 q_chroma_dec->locklevel = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X00FF); 00545 } 00546 /* Digital clamp */ 00547 if (q_digital_clamp != NULL) { 00548 reg_value = (uint32_t)*(vdec_reg->dcpsr1); 00549 /* Digital clamp subtraction value (Cb signal) */ 00550 q_digital_clamp->clamplevel_cb = (uint16_t)(reg_value >> VEDC_REG_SHIFT_10); 00551 /* Digital clamp subtraction value (Y signal) */ 00552 q_digital_clamp->clamplevel_y = (uint16_t)(reg_value & (uint32_t)VDEC_REG_MASK_0X03FF); 00553 00554 /* Digital clamp subtraction value (Cr signal) */ 00555 q_digital_clamp->clamplevel_cr = (uint16_t)((uint32_t)*(vdec_reg->dcpsr2) >> VEDC_REG_SHIFT_10); 00556 00557 /* Noise autocorrelation strength at digital clamp pulse position */ 00558 q_digital_clamp->acfstrength = *(vdec_reg->nsdsr); 00559 } 00560 return; 00561 } /* End of function VDEC_Query() */ 00562 00563 /**************************************************************************//** 00564 * @brief Sets registers for noise reduction LPF 00565 * @param[in] vdec_reg : VDEC registers 00566 * @param[in] p_noise_rd_lpf : Noise reduction LPF parameter 00567 * @retval None 00568 *****************************************************************************/ 00569 static void NoiseReductionLPF ( 00570 const vdec_reg_address_t * const vdec_reg, 00571 const vdec_noise_rd_lpf_t * const p_noise_rd_lpf) 00572 { 00573 uint32_t reg_data; 00574 00575 /* Noise reduction LPF */ 00576 if (p_noise_rd_lpf != NULL) { 00577 reg_data = (uint32_t)*(vdec_reg->synscr1) & (uint32_t)(~VDEC_REG_MASK_0XFC00); 00578 /* LPF cutoff frequency before vertical sync separation */ 00579 reg_data |= (uint32_t)p_noise_rd_lpf->lpfvsync << VEDC_REG_SHIFT_13; 00580 /* LPF cutoff frequency before horizontal sync separation */ 00581 reg_data |= (uint32_t)p_noise_rd_lpf->lpfhsync << VEDC_REG_SHIFT_10; 00582 00583 *(vdec_reg->synscr1) = (uint16_t)reg_data; 00584 } 00585 return; 00586 } /* End of function NoiseReductionLPF() */ 00587 00588 /**************************************************************************//** 00589 * @brief Sets registers for auto level control sync slicer 00590 * @param[in] vdec_reg : VDEC registers 00591 * @param[in] p_sync_slicer : Auto level control sync slicer parameter 00592 * @retval None 00593 *****************************************************************************/ 00594 static void SyncSlicer (const vdec_reg_address_t * const vdec_reg, const vdec_sync_slicer_t * const p_sync_slicer) 00595 { 00596 uint32_t reg_data; 00597 00598 /* Auto level control sync slicer */ 00599 if (p_sync_slicer != NULL) { 00600 reg_data = (uint32_t)*(vdec_reg->synscr1) & (uint32_t)(~VDEC_REG_MASK_0X00FF); 00601 /* Reference level operation speed control for composite sync separation (for Hsync signal) */ 00602 reg_data |= (uint32_t)p_sync_slicer->velocityshift_h << VEDC_REG_SHIFT_4; 00603 /* Auto-slice level setting for composite sync separation circuit (for Hsync signal) */ 00604 reg_data |= (uint32_t)p_sync_slicer->slicermode_h << VEDC_REG_SHIFT_2; 00605 /* Auto-slice level setting for composite sync separation circuit (for Vsync signal) */ 00606 reg_data |= (uint32_t)p_sync_slicer->slicermode_v ; 00607 00608 *(vdec_reg->synscr1) = (uint16_t)reg_data; 00609 00610 /* Max ratio of horizontal cycle to horizontal sync signal pulse width 00611 and min ratio of horizontal cycle to horizontal sync signal pulse width (for Hsync signal) */ 00612 reg_data = (uint32_t)p_sync_slicer->syncmaxduty_h << VEDC_REG_SHIFT_6; 00613 reg_data |= (uint32_t)p_sync_slicer->syncminduty_h ; 00614 00615 *(vdec_reg->synscr2) = (uint16_t)reg_data; 00616 00617 /* Clipping level and slice level for composite sync signal separation (for Hsync signal) */ 00618 reg_data = (uint32_t)p_sync_slicer->ssclipsel << VEDC_REG_SHIFT_10; 00619 reg_data |= (uint32_t)p_sync_slicer->csyncslice_h ; 00620 00621 *(vdec_reg->synscr3) = (uint16_t)reg_data; 00622 00623 /* Max ratio of horizontal cycle to horizontal sync signal pulse width 00624 and min ratio of horizontal cycle to horizontal sync signal pulse width (for Vsync signal) */ 00625 reg_data = (uint32_t)p_sync_slicer->syncmaxduty_v << VEDC_REG_SHIFT_6; 00626 reg_data |= (uint32_t)p_sync_slicer->syncminduty_v ; 00627 00628 *(vdec_reg->synscr4) = (uint16_t)reg_data; 00629 00630 /* Delays the separated vertical sync signal for 1/4 horizontal cycle */ 00631 reg_data = (p_sync_slicer->vsyncdelay == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X8000; 00632 /* Threshold for vertical sync separation */ 00633 reg_data |= (uint32_t)p_sync_slicer->vsyncslice << VEDC_REG_SHIFT_10; 00634 /* Slice level for composite sync signal separation (for Vsync signal) */ 00635 reg_data |= (uint32_t)p_sync_slicer->csyncslice_v ; 00636 00637 *(vdec_reg->synscr5) = (uint16_t)reg_data; 00638 } 00639 return; 00640 } /* End of function SyncSlicer() */ 00641 00642 /**************************************************************************//** 00643 * @brief Sets registers for horizontal AFC 00644 * @param[in] vdec_reg : VDEC registers 00645 * @param[in] p_horizontal_afc : Horizontal AFC parameter 00646 * @retval None 00647 *****************************************************************************/ 00648 static void HorizontalAFC ( 00649 const vdec_reg_address_t * const vdec_reg, 00650 const vdec_horizontal_afc_t * const p_horizontal_afc) 00651 { 00652 uint32_t reg_data; 00653 00654 /* Horizontal AFC */ 00655 if (p_horizontal_afc != NULL) { 00656 reg_data = (uint32_t)*(vdec_reg->hafccr1) & (uint32_t)(~VDEC_REG_MASK_0XF3FF); 00657 /* Horizontal AFC loop gain */ 00658 reg_data |= (uint32_t)p_horizontal_afc->hafcgain << VEDC_REG_SHIFT_12; 00659 /* Horizontal AFC center oscillation frequency */ 00660 reg_data |= (uint32_t)p_horizontal_afc->hafctyp ; 00661 00662 *(vdec_reg->hafccr1) = (uint16_t)reg_data; 00663 00664 /* Start line of horizontal AFC normal operation 00665 and Horizontal AFC forced double-speed oscillation (DOX2HOSC = 0, auto control) */ 00666 reg_data = (uint32_t)p_horizontal_afc->hafcstart << VEDC_REG_SHIFT_12; 00667 /* Disable of horizontal AFC double speed detection */ 00668 reg_data |= (p_horizontal_afc->nox2hosc == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0800; 00669 /* Maximum oscillation frequency of horizontal AFC */ 00670 reg_data |= (uint32_t)p_horizontal_afc->hafcmax ; 00671 00672 *(vdec_reg->hafccr2) = (uint16_t)reg_data; 00673 00674 /* End line of horizontal AFC normal operation */ 00675 reg_data = (uint32_t)p_horizontal_afc->hafcend << VEDC_REG_SHIFT_12; 00676 /* Horizontal AFC VBI period operating mode */ 00677 reg_data |= (uint32_t)p_horizontal_afc->hafcmode << VEDC_REG_SHIFT_10; 00678 /* Min oscillation frequency of horizontal AFC */ 00679 reg_data |= (uint32_t)p_horizontal_afc->hafcmin ; 00680 00681 *(vdec_reg->hafccr3) = (uint16_t)reg_data; 00682 00683 /* Forcible or LOWGAIN control */ 00684 reg_data = (p_horizontal_afc->phdet_fix == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0010; 00685 /* Phase comparator feedback adjust for low sync signal lock stability */ 00686 reg_data |= (uint32_t)p_horizontal_afc->phdet_div ; 00687 00688 *(vdec_reg->afcpfcr) = (uint16_t)reg_data; 00689 } 00690 return; 00691 } /* End of function HorizontalAFC() */ 00692 00693 /**************************************************************************//** 00694 * @brief Sets registers for vertical count-down 00695 * @param[in] vdec_reg : VDEC registers 00696 * @param[in] p_vcount_down : Vertical count-down parameter 00697 * @retval None 00698 *****************************************************************************/ 00699 static void VerticalCountdown ( 00700 const vdec_reg_address_t * const vdec_reg, 00701 const vdec_vcount_down_t * const p_vcount_down) 00702 { 00703 uint32_t reg_data; 00704 00705 /* Vertical count-down */ 00706 if (p_vcount_down != NULL) { 00707 /* Vertical countdown 50-Hz oscillation mode 00708 and Vertical countdown free-run oscillation mode (VCDFREERUN = OFF) */ 00709 reg_data = (p_vcount_down->novcd50_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X4000 : (uint32_t)0x0000u; 00710 /* Vertical countdown 60-Hz (59.94-Hz) oscillation mode */ 00711 reg_data |= (p_vcount_down->novcd60_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X2000 : (uint32_t)0x0000u; 00712 /* Vertical countdown center oscillation frequency */ 00713 reg_data |= (uint32_t)p_vcount_down->vcddefault << VEDC_REG_SHIFT_11; 00714 /* Vertical countdown sync area */ 00715 reg_data |= (uint32_t)p_vcount_down->vcdwindow << VEDC_REG_SHIFT_5; 00716 /* Vertical countdown minimum oscillation frequency */ 00717 reg_data |= (uint32_t)p_vcount_down->vcdoffset ; 00718 00719 *(vdec_reg->vcdwcr1) = (uint16_t)reg_data; 00720 } 00721 return; 00722 } /* End of function VerticalCountdown() */ 00723 00724 /**************************************************************************//** 00725 * @brief Sets registers for AGC/PGA 00726 * @param[in] vdec_reg : VDEC registers 00727 * @param[in] p_agc : AGC/PGA parameter 00728 * @retval None 00729 *****************************************************************************/ 00730 static void AgcPga (const vdec_reg_address_t * const vdec_reg, const vdec_agc_t * const p_agc) 00731 { 00732 uint32_t reg_data; 00733 00734 /* AGC/PGA */ 00735 if (p_agc != NULL) { 00736 /* A/D converter AGC ON/OFF control & PGA switch */ 00737 if (p_agc->agcmode == VDEC_OFF ) { 00738 reg_data = (uint32_t)*(vdec_reg->pgacr) | (uint32_t)VDEC_REG_SET_0X2000; 00739 *(vdec_reg->pgacr) = (uint16_t)reg_data; 00740 *(vdec_reg->adccr1) = (uint16_t)0u; 00741 } else { 00742 *(vdec_reg->adccr1) = (uint16_t)VDEC_REG_SET_0X0100; 00743 reg_data = (uint32_t)*(vdec_reg->pgacr) & (uint32_t)(~VDEC_REG_SET_0X2000); 00744 *(vdec_reg->pgacr) = (uint16_t)reg_data; 00745 } 00746 /* PGA gain */ 00747 reg_data = (uint32_t)*(vdec_reg->pgacr) & (uint32_t)(~VDEC_REG_MASK_0X1F00); 00748 reg_data |= (uint32_t)p_agc->pga_gain << VEDC_REG_SHIFT_8; 00749 00750 *(vdec_reg->pgacr) = (uint16_t)reg_data; 00751 00752 /* PGA register update register */ 00753 *(vdec_reg->pga_update) = (uint16_t)1u; 00754 00755 /* Manual control of sync signal amplitude detection during VBI period */ 00756 reg_data = (p_agc->doreduce == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X2000; 00757 /* Control of sync signal amplitude detection during VBI period */ 00758 reg_data |= (p_agc->noreduce_ == VDEC_OFF ) ? (uint32_t)VDEC_REG_SET_0X1000 : (uint32_t)0x0000u; 00759 /* AGC response speed */ 00760 reg_data |= (uint32_t)p_agc->agcresponse << VEDC_REG_SHIFT_9; 00761 /* Sync signal reference amplitude */ 00762 reg_data |= (uint32_t)p_agc->agclevel ; 00763 00764 *(vdec_reg->agccr1) = (uint16_t)reg_data; 00765 00766 /* AGC gain adjustment accuracy */ 00767 reg_data = (uint32_t)*(vdec_reg->agccr2) & (uint32_t)(~VDEC_REG_MASK_0X3F00); 00768 reg_data |= (uint32_t)p_agc->agcprecis << VEDC_REG_SHIFT_8; 00769 00770 *(vdec_reg->agccr2) = (uint16_t)reg_data; 00771 } 00772 return; 00773 } /* End of function AgcPga() */ 00774 00775 /**************************************************************************//** 00776 * @brief Sets registers for peak limiter control 00777 * @param[in] vdec_reg : VDEC registers 00778 * @param[in] p_peak_limiter : Peak limiter control parameter 00779 * @retval None 00780 *****************************************************************************/ 00781 static void PeakLimiterControl ( 00782 const vdec_reg_address_t * const vdec_reg, 00783 const vdec_peak_limiter_t * const p_peak_limiter) 00784 { 00785 uint32_t reg_data; 00786 00787 /* Peak limiter control */ 00788 if (p_peak_limiter != NULL) { 00789 /* Peak luminance value limited by peak limiter */ 00790 reg_data = (uint32_t)p_peak_limiter->peaklevel << VEDC_REG_SHIFT_14; 00791 /* Response speed with peak limiter gain decreased */ 00792 reg_data |= (uint32_t)p_peak_limiter->peakattack << VEDC_REG_SHIFT_12; 00793 /* Response speed with peak limiter gain increased */ 00794 reg_data |= (uint32_t)p_peak_limiter->peakrelease << VEDC_REG_SHIFT_10; 00795 /* Maximum compression rate of peak limiter */ 00796 reg_data |= (uint32_t)p_peak_limiter->peakratio << VEDC_REG_SHIFT_8; 00797 /* Allowable number of overflowing pixels */ 00798 reg_data |= (uint32_t)p_peak_limiter->maxpeaksamples ; 00799 00800 *(vdec_reg->pklimitcr) = (uint16_t)reg_data; 00801 } 00802 return; 00803 } /* End of function PeakLimiterControl() */ 00804 00805 /**************************************************************************//** 00806 * @brief Sets registers for over-range control 00807 * @param[in] vdec_reg : VDEC registers 00808 * @param[in] p_over_range : Over-range control parameter 00809 * @retval None 00810 *****************************************************************************/ 00811 static void OverRangeControl (const vdec_reg_address_t * const vdec_reg, const vdec_over_range_t * const p_over_range) 00812 { 00813 uint32_t reg_data; 00814 00815 /* Over-range control */ 00816 if (p_over_range != NULL) { 00817 /* A/D over-threshold level (between levels 0 and 1) */ 00818 *(vdec_reg->rgorcr1) = p_over_range->radj_o_level0 ; 00819 /* A/D under-threshold level (between levels 2 and 3) */ 00820 *(vdec_reg->rgorcr2) = p_over_range->radj_u_level0 ; 00821 /* A/D over-threshold level (between levels 1 and 2) */ 00822 *(vdec_reg->rgorcr3) = p_over_range->radj_o_level1 ; 00823 /* A/D under-threshold level (between levels 1 and 2) */ 00824 *(vdec_reg->rgorcr4) = p_over_range->radj_u_level1 ; 00825 /* A/D over-threshold level (between levels 2 and 3) */ 00826 *(vdec_reg->rgorcr5) = p_over_range->radj_o_level2 ; 00827 /* A/D under-threshold level (between levels 0 and 1) */ 00828 *(vdec_reg->rgorcr6) = p_over_range->radj_u_level2 ; 00829 00830 reg_data = (uint32_t)*(vdec_reg->rgorcr7) & (uint32_t)(~VDEC_REG_MASK_0X0007); 00831 /* Over-range detection enable */ 00832 reg_data |= (p_over_range->ucmp_sw == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0004; 00833 /* Under-range detection enable */ 00834 reg_data |= (p_over_range->dcmp_sw == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X0002; 00835 /* Horizontal enlargement of over/under-range level */ 00836 reg_data |= (p_over_range->hwide_sw == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)0x0001u; 00837 00838 *(vdec_reg->rgorcr7) = (uint16_t)reg_data; 00839 } 00840 return; 00841 } /* End of function OverRangeControl() */ 00842 00843 /**************************************************************************//** 00844 * @brief Sets registers for Y/C separation control 00845 * @param[in] vdec_reg : VDEC registers 00846 * @param[in] p_yc_sep_ctrl : Y/C separation control parameter 00847 * @retval None 00848 *****************************************************************************/ 00849 static void YcSeparationControl ( 00850 const vdec_reg_address_t * const vdec_reg, 00851 const vdec_yc_sep_ctrl_t * const p_yc_sep_ctrl) 00852 { 00853 uint32_t reg_data; 00854 00855 /* Y/C separation control */ 00856 if (p_yc_sep_ctrl != NULL) { 00857 /* Two-dimensional Y/C separation filter select coefficient (K15, K13, and K11) */ 00858 reg_data = (uint32_t)p_yc_sep_ctrl->k15 << VEDC_REG_SHIFT_12; 00859 reg_data |= (uint32_t)p_yc_sep_ctrl->k13 << VEDC_REG_SHIFT_6; 00860 reg_data |= (uint32_t)p_yc_sep_ctrl->k11 ; 00861 00862 *(vdec_reg->ycscr3) = (uint16_t)reg_data; 00863 00864 /* Two-dimensional Y/C separation filter select coefficient (K16, K14, and K12) */ 00865 reg_data = (uint32_t)p_yc_sep_ctrl->k16 << VEDC_REG_SHIFT_12; 00866 reg_data |= (uint32_t)p_yc_sep_ctrl->k14 << VEDC_REG_SHIFT_6; 00867 reg_data |= (uint32_t)p_yc_sep_ctrl->k12 ; 00868 00869 *(vdec_reg->ycscr4) = (uint16_t)reg_data; 00870 00871 /* Two-dimensional Y/C separation filter select coefficient (K22A and K21A) */ 00872 reg_data = (uint32_t)p_yc_sep_ctrl->k22a << VEDC_REG_SHIFT_8; 00873 reg_data |= (uint32_t)p_yc_sep_ctrl->k21a ; 00874 00875 *(vdec_reg->ycscr5) = (uint16_t)reg_data; 00876 00877 /* Two-dimensional Y/C separation filter select coefficient (K22B and K21B) */ 00878 reg_data = (uint32_t)p_yc_sep_ctrl->k22b << VEDC_REG_SHIFT_8; 00879 reg_data |= (uint32_t)p_yc_sep_ctrl->k21b ; 00880 00881 *(vdec_reg->ycscr6) = (uint16_t)reg_data; 00882 00883 /* Two-dimensional Y/C separation filter select coefficient (K23B, K23A, and K24) */ 00884 reg_data = (uint32_t)*(vdec_reg->ycscr7) & (uint32_t)(~VDEC_REG_MASK_0XFF1F); 00885 reg_data |= (uint32_t)p_yc_sep_ctrl->k23b << VEDC_REG_SHIFT_12; 00886 reg_data |= (uint32_t)p_yc_sep_ctrl->k23a << VEDC_REG_SHIFT_8; 00887 reg_data |= (uint32_t)p_yc_sep_ctrl->k24 ; 00888 00889 *(vdec_reg->ycscr7) = (uint16_t)reg_data; 00890 00891 reg_data = (uint32_t)*(vdec_reg->ycscr8) & (uint32_t)(~VDEC_REG_MASK_0XF800); 00892 /* Latter-stage horizontal BPF select */ 00893 reg_data |= (p_yc_sep_ctrl->hbpf_narrow == VDEC_LSTG_BPFSEL_BYPASS ) ? (uint32_t)0x0000u : 00894 (uint32_t)VDEC_REG_SET_0X8000; 00895 /* Latter-stage horizontal/vertical BPF select */ 00896 reg_data |= (p_yc_sep_ctrl->hvbpf_narrow == VDEC_LSTG_BPFSEL_BYPASS ) ? (uint32_t)0x0000u : 00897 (uint32_t)VDEC_REG_SET_0X4000; 00898 /* Former-stage horizontal BPF select */ 00899 reg_data |= (p_yc_sep_ctrl->hbpf1_9tap_on == VDEC_FSTG_BPFSEL_17TAP ) ? (uint32_t)0x0000u : 00900 (uint32_t)VDEC_REG_SET_0X2000; 00901 /* Former-stage horizontal/vertical BPF select */ 00902 reg_data |= (p_yc_sep_ctrl->hvbpf1_9tap_on == VDEC_FSTG_BPFSEL_17TAP ) ? (uint32_t)0x0000u : 00903 (uint32_t)VDEC_REG_SET_0X1000; 00904 /* Horizontal filter and horizontal/vertical filter bandwidth switch signal */ 00905 reg_data |= (p_yc_sep_ctrl->hfil_tap_sel == VDEC_HFIL_TAP_SEL_17TAP ) ? (uint32_t)0x0000u : 00906 (uint32_t)VDEC_REG_SET_0X0800; 00907 00908 *(vdec_reg->ycscr8) = (uint16_t)reg_data; 00909 00910 /* Two-dimensional filter mixing select */ 00911 reg_data = (p_yc_sep_ctrl->det2_on == VDEC_OFF ) ? (uint32_t)0x0000u : (uint32_t)VDEC_REG_SET_0X8000; 00912 /* Mixing ratio of signal after passing horizontal filter 00913 to signal after passing former-stage horizontal filter */ 00914 reg_data |= (uint32_t)p_yc_sep_ctrl->hsel_mix_y << VEDC_REG_SHIFT_8; 00915 /* Mixing ratio of signal after passing vertical filter 00916 to signal after passing former-stage horizontal/vertical filter */ 00917 reg_data |= (uint32_t)p_yc_sep_ctrl->vsel_mix_y << VEDC_REG_SHIFT_4; 00918 /* Mixing ratio of signal after passing horizontal/vertical filter 00919 to signal after passing former-stage horizontal/vertical filter */ 00920 reg_data |= (uint32_t)p_yc_sep_ctrl->hvsel_mix_y ; 00921 00922 *(vdec_reg->ycscr9) = (uint16_t)reg_data; 00923 00924 /* Vertical luminance detection level for correlation detection filter */ 00925 reg_data = (uint32_t)*(vdec_reg->ycscr11) & (uint32_t)(~VDEC_REG_MASK_0X01FF); 00926 reg_data |= (uint32_t)p_yc_sep_ctrl->v_y_level ; 00927 00928 *(vdec_reg->ycscr11) = (uint16_t)reg_data; 00929 00930 if (p_yc_sep_ctrl->det2_on == VDEC_OFF ) { 00931 reg_data = ((uint32_t)VDEC_FILMIX_RATIO_0 << VEDC_REG_SHIFT_12) | 00932 ((uint32_t)VDEC_FILMIX_RATIO_0 << VEDC_REG_SHIFT_8); 00933 } else { 00934 /* Mixing ratio of C signal after passing horizontal/vertical adaptive filter 00935 to signal after passing correlation detection filter */ 00936 reg_data = (uint32_t)p_yc_sep_ctrl->det2_mix_c << VEDC_REG_SHIFT_12; 00937 /* Mixing ratio of C signal for Y generation after passing 00938 horizontal/vertical adaptive filter to signal after passing correlation */ 00939 reg_data |= (uint32_t)p_yc_sep_ctrl->det2_mix_y << VEDC_REG_SHIFT_8; 00940 } 00941 /* Two-dimensional cascade/TAKE-OFF filter mode select */ 00942 reg_data |= (uint32_t)p_yc_sep_ctrl->fil2_mode_2d << VEDC_REG_SHIFT_2; 00943 /* Two-dimensional cascade filter select */ 00944 reg_data |= (p_yc_sep_ctrl->fil2_narrow_2d == VDEC_2D_FIL_SEL_BYPASS ) ? (uint32_t)0x0000u : 00945 (uint32_t)0x0001u; 00946 00947 *(vdec_reg->ycscr12) = (uint16_t)reg_data; 00948 } 00949 return; 00950 } /* End of function YcSeparationControl() */ 00951 00952 /**************************************************************************//** 00953 * @brief Sets registers for chroma filter TAP coefficient 00954 * @param[in] fil_reg_address : 2D filter TAP coefficient registers 00955 * @param[in] fil2_2d : Chroma filter TAP coefficient for Y/C separation 00956 * @retval None 00957 *****************************************************************************/ 00958 static void FilterTAPsCoefficient ( 00959 volatile uint16_t * const * fil_reg_address, 00960 const vdec_chrfil_tap_t * const fil2_2d) 00961 { 00962 int32_t tap_coef; 00963 volatile uint16_t * fil_reg; 00964 const uint16_t * taps; 00965 00966 if (fil2_2d != NULL) { 00967 taps = fil2_2d->fil2_2d_f ; 00968 for (tap_coef = 0; tap_coef < VDEC_CHRFIL_TAPCOEF_NUM; tap_coef++) { 00969 fil_reg = *fil_reg_address; 00970 fil_reg_address++; 00971 *fil_reg = *taps; 00972 taps++; 00973 } 00974 } 00975 return; 00976 } /* End of function FilterTAPsCoefficient() */ 00977
Generated on Tue Jul 12 2022 15:08:46 by
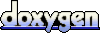