Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdec_check_parameter.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdec_check_parameter.c 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDEC driver parameter check processing 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include "r_vdec.h" 00035 #include "r_vdec_user.h" 00036 #include "r_vdec_check_parameter.h" 00037 00038 00039 #ifdef R_VDEC_CHECK_PARAMETERS 00040 /****************************************************************************** 00041 Macro definitions 00042 ******************************************************************************/ 00043 /* Valid bit range */ 00044 #define VDEC_CPARA_RANGE_0X00000001 (0x00000001u) 00045 #define VDEC_CPARA_RANGE_0X00000003 (0x00000003u) 00046 #define VDEC_CPARA_RANGE_0X00000007 (0x00000007u) 00047 #define VDEC_CPARA_RANGE_0X0000000F (0x0000000Fu) 00048 #define VDEC_CPARA_RANGE_0X0000001F (0x0000001Fu) 00049 #define VDEC_CPARA_RANGE_0X0000003F (0x0000003Fu) 00050 #define VDEC_CPARA_RANGE_0X0000007F (0x0000007Fu) 00051 #define VDEC_CPARA_RANGE_0X000000FF (0x000000FFu) 00052 #define VDEC_CPARA_RANGE_0X000001FF (0x000001FFu) 00053 #define VDEC_CPARA_RANGE_0X000003FF (0x000003FFu) 00054 #define VDEC_CPARA_RANGE_0X000007FF (0x000007FFu) 00055 #define VDEC_CPARA_RANGE_0X00001FFF (0x00001FFFu) 00056 00057 /****************************************************************************** 00058 Typedef definitions 00059 ******************************************************************************/ 00060 00061 /****************************************************************************** 00062 Private global variables and functions 00063 ******************************************************************************/ 00064 static vdec_error_t NoiseReductionLPFCheckParam(const vdec_noise_rd_lpf_t * const p_noise_rd_lpf); 00065 static vdec_error_t SyncSlicerCheckParam(const vdec_sync_slicer_t * const p_sync_slicer); 00066 static vdec_error_t HorizontalAFCCheckParam(const vdec_horizontal_afc_t * const p_horizontal_afc); 00067 static vdec_error_t VerticalCountdownCheckParam(const vdec_vcount_down_t * const p_vcount_down); 00068 static vdec_error_t AgcPgaCheckParam(const vdec_agc_t * const p_agc); 00069 static vdec_error_t PeakLimiterControlCheckParam(const vdec_peak_limiter_t * const p_peak_limiter); 00070 static vdec_error_t OverRangeControlCheckParam(const vdec_over_range_t * const p_over_range); 00071 static vdec_error_t YcSeparationControlCheckParam(const vdec_yc_sep_ctrl_t * const p_yc_sep_ctrl); 00072 static vdec_error_t FilterTAPsCoefficientCheckParam(const vdec_chrfil_tap_t * const fil2_2d); 00073 static vdec_error_t ChromaDecodingControlCheckParam(const vdec_chrmdec_ctrl_t * const p_chrmdec_ctrl); 00074 static vdec_error_t BurstLockCheckParam(const vdec_burst_lock_t * const p_burst_lock); 00075 static vdec_error_t AccColorKillerCheckParam(const vdec_acc_t * const p_acc); 00076 00077 00078 /**************************************************************************//** 00079 * @brief Checks on input pin control 00080 * @param[in] vinsel : Input pin control 00081 * @retval Error code 00082 *****************************************************************************/ 00083 vdec_error_t VDEC_InitializeCheckParam (const vdec_adc_vinsel_t vinsel) 00084 { 00085 vdec_error_t vdec_error; 00086 00087 vdec_error = VDEC_OK ; 00088 00089 if ((vinsel != VDEC_ADC_VINSEL_VIN1 ) && (vinsel != VDEC_ADC_VINSEL_VIN2 )) { 00090 vdec_error = VDEC_ERR_PARAM ; 00091 goto END; 00092 } 00093 END: 00094 return vdec_error; 00095 } /* End of function VDEC_InitializeCheckParam() */ 00096 00097 /**************************************************************************//** 00098 * @brief Checks on active image period parameter 00099 * @param[in] param : Active image period parameter 00100 * @retval Error code 00101 *****************************************************************************/ 00102 vdec_error_t VDEC_ActivePeriodCheckParam (const vdec_active_period_t * const param) 00103 { 00104 vdec_error_t vdec_error; 00105 00106 vdec_error = VDEC_OK ; 00107 00108 if (param == NULL) { 00109 vdec_error = VDEC_ERR_PARAM ; 00110 goto END; 00111 } 00112 /* Left end of input video signal capturing area */ 00113 if (((uint32_t)param->srcleft & (uint32_t)(~VDEC_CPARA_RANGE_0X000001FF)) != 0u) { 00114 vdec_error = VDEC_ERR_PARAM ; 00115 goto END; 00116 } 00117 /* Top end of input video signal capturing area */ 00118 if (((uint32_t)param->srctop & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00119 vdec_error = VDEC_ERR_PARAM ; 00120 goto END; 00121 } 00122 /* Height of input video signal capturing area */ 00123 if (((uint32_t)param->srcheight & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00124 vdec_error = VDEC_ERR_PARAM ; 00125 goto END; 00126 } 00127 /* Width of input video signal capturing area */ 00128 if (((uint32_t)param->srcwidth & (uint32_t)(~VDEC_CPARA_RANGE_0X000007FF)) != 0u) { 00129 vdec_error = VDEC_ERR_PARAM ; 00130 goto END; 00131 } 00132 END: 00133 return vdec_error; 00134 } /* End of function VDEC_ActivePeriodCheckParam() */ 00135 00136 /**************************************************************************//** 00137 * @brief Checks on sync separation parameter 00138 * @param[in] param : Sync separation parameter 00139 * @retval Error code 00140 *****************************************************************************/ 00141 vdec_error_t VDEC_SyncSeparationCheckParam (const vdec_sync_separation_t * const param) 00142 { 00143 vdec_error_t vdec_error; 00144 00145 vdec_error = VDEC_OK ; 00146 00147 if (param == NULL) { 00148 vdec_error = VDEC_ERR_PARAM ; 00149 goto END; 00150 } 00151 /* Noise reduction LPF */ 00152 vdec_error = NoiseReductionLPFCheckParam(param->noise_rd_lpf ); 00153 if (vdec_error != VDEC_OK ) { 00154 goto END; 00155 } 00156 /* Sync slicer */ 00157 vdec_error = SyncSlicerCheckParam(param->sync_slicer ); 00158 if (vdec_error != VDEC_OK ) { 00159 goto END; 00160 } 00161 /* Horizontal AFC */ 00162 vdec_error = HorizontalAFCCheckParam(param->horizontal_afc ); 00163 if (vdec_error != VDEC_OK ) { 00164 goto END; 00165 } 00166 /* Vertical countdown */ 00167 vdec_error = VerticalCountdownCheckParam(param->vcount_down ); 00168 if (vdec_error != VDEC_OK ) { 00169 goto END; 00170 } 00171 /* AGC / PGA */ 00172 vdec_error = AgcPgaCheckParam(param->agc ); 00173 if (vdec_error != VDEC_OK ) { 00174 goto END; 00175 } 00176 /* Peak limiter control */ 00177 vdec_error = PeakLimiterControlCheckParam(param->peak_limiter ); 00178 if (vdec_error != VDEC_OK ) { 00179 goto END; 00180 } 00181 END: 00182 return vdec_error; 00183 } /* End of function VDEC_SyncSeparationCheckParam() */ 00184 00185 /**************************************************************************//** 00186 * @brief Checks on Y/C separation parameter 00187 * @param[in] param : Y/C separation parameter 00188 * @retval Error code 00189 *****************************************************************************/ 00190 vdec_error_t VDEC_YcSeparationCheckParam (const vdec_yc_separation_t * const param) 00191 { 00192 vdec_error_t vdec_error; 00193 00194 vdec_error = VDEC_OK ; 00195 00196 if (param == NULL) { 00197 vdec_error = VDEC_ERR_PARAM ; 00198 goto END; 00199 } 00200 /* Over-range control */ 00201 vdec_error = OverRangeControlCheckParam(param->over_range ); 00202 if (vdec_error != VDEC_OK ) { 00203 goto END; 00204 } 00205 /* Y/C separation control */ 00206 vdec_error = YcSeparationControlCheckParam(param->yc_sep_ctrl ); 00207 if (vdec_error != VDEC_OK ) { 00208 goto END; 00209 } 00210 /* Two-dimensional cascade broadband (3.58/4.43/SECAM-DR)/TAKE-OFF filter TAP coefficient */ 00211 vdec_error = FilterTAPsCoefficientCheckParam(param->fil2_2d_wa ); 00212 if (vdec_error != VDEC_OK ) { 00213 goto END; 00214 } 00215 /* Two-dimensional cascade broadband (SECAM-DB) filter TAP coefficient */ 00216 vdec_error = FilterTAPsCoefficientCheckParam(param->fil2_2d_wb ); 00217 if (vdec_error != VDEC_OK ) { 00218 goto END; 00219 } 00220 /* Two-dimensional cascade narrowband (3.58/4.43/SECAM-DR) filter TAP coefficient */ 00221 vdec_error = FilterTAPsCoefficientCheckParam(param->fil2_2d_na ); 00222 if (vdec_error != VDEC_OK ) { 00223 goto END; 00224 } 00225 /* Two-dimensional cascade narrowband (SECAMDB) filter TAP coefficient */ 00226 vdec_error = FilterTAPsCoefficientCheckParam(param->fil2_2d_nb ); 00227 if (vdec_error != VDEC_OK ) { 00228 goto END; 00229 } 00230 END: 00231 return vdec_error; 00232 } /* End of function VDEC_YcSeparationCheckParam() */ 00233 00234 /**************************************************************************//** 00235 * @brief Checks on chroma decoding parameter 00236 * @param[in] param : Chroma decoding parameter 00237 * @retval Error code 00238 *****************************************************************************/ 00239 vdec_error_t VDEC_ChromaDecodingCheckParam (const vdec_chroma_decoding_t * const param) 00240 { 00241 vdec_tint_ry_t * p_tint_ry; 00242 vdec_error_t vdec_error; 00243 00244 vdec_error = VDEC_OK ; 00245 00246 if (param == NULL) { 00247 vdec_error = VDEC_ERR_PARAM ; 00248 goto END; 00249 } 00250 /* Chroma decoding control */ 00251 vdec_error = ChromaDecodingControlCheckParam(param->chrmdec_ctrl ); 00252 if (vdec_error != VDEC_OK ) { 00253 goto END; 00254 } 00255 /* Burst lock */ 00256 vdec_error = BurstLockCheckParam(param->burst_lock ); 00257 if (vdec_error != VDEC_OK ) { 00258 goto END; 00259 } 00260 /* ACC/color killer */ 00261 vdec_error = AccColorKillerCheckParam(param->acc ); 00262 if (vdec_error != VDEC_OK ) { 00263 goto END; 00264 } 00265 /* TINT/R-Y axis correction (only valid for NTSC/PAL) */ 00266 p_tint_ry = param->tint_ry ; 00267 if (p_tint_ry != NULL) { 00268 /* Fine adjustment of R-Y demodulation axis and hue adjustment level */ 00269 if ((((uint32_t)p_tint_ry->tintsub & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) || 00270 (((uint32_t)p_tint_ry->tintmain & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u)) { 00271 vdec_error = VDEC_ERR_PARAM ; 00272 goto END; 00273 } 00274 } 00275 END: 00276 return vdec_error; 00277 } /* End of function VDEC_ChromaDecodingCheckParam() */ 00278 00279 /**************************************************************************//** 00280 * @brief Checks on digital clamp parameter 00281 * @param[in] param : Digital clamp parameter 00282 * @retval Error code 00283 *****************************************************************************/ 00284 vdec_error_t VDEC_DigitalClampCheckParam (const vdec_degital_clamp_t * const param) 00285 { 00286 vdec_pedestal_clamp_t * p_pedestal_clamp; 00287 vdec_center_clamp_t * p_center_clamp; 00288 vdec_noise_det_t * p_noise_det; 00289 vdec_error_t vdec_error; 00290 00291 vdec_error = VDEC_OK ; 00292 00293 if (param == NULL) { 00294 vdec_error = VDEC_ERR_PARAM ; 00295 goto END; 00296 } 00297 /* Digital clamp response speed */ 00298 if (((uint32_t)param->dcpresponse & (uint32_t)(~VDEC_CPARA_RANGE_0X00000007)) != 0u) { 00299 vdec_error = VDEC_ERR_PARAM ; 00300 goto END; 00301 } 00302 /* Digital clamp start line (in 1-line units) */ 00303 if (((uint32_t)param->dcpstart & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00304 vdec_error = VDEC_ERR_PARAM ; 00305 goto END; 00306 } 00307 /* Digital clamp end line (in 1-line units) */ 00308 if (((uint32_t)param->dcpend & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00309 vdec_error = VDEC_ERR_PARAM ; 00310 goto END; 00311 } 00312 /* Digital clamp pulse width */ 00313 if (((uint32_t)param->dcpwidth & (uint32_t)(~VDEC_CPARA_RANGE_0X0000007F)) != 0u) { 00314 vdec_error = VDEC_ERR_PARAM ; 00315 goto END; 00316 } 00317 00318 /* Pedestal clamp */ 00319 p_pedestal_clamp = param->pedestal_clamp ; 00320 if (p_pedestal_clamp != NULL) { 00321 /* Clamp offset level (Y signal) */ 00322 if (((uint32_t)p_pedestal_clamp->blanklevel_y & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00323 vdec_error = VDEC_ERR_PARAM ; 00324 goto END; 00325 } 00326 /* Digital clamp pulse horizontal start position (Y signal) */ 00327 if (((uint32_t)p_pedestal_clamp->dcppos_y & (uint32_t)(~VDEC_CPARA_RANGE_0X000000FF)) != 0u) { 00328 vdec_error = VDEC_ERR_PARAM ; 00329 goto END; 00330 } 00331 } 00332 /* Center clamp */ 00333 p_center_clamp = param->center_clamp ; 00334 if (p_center_clamp != NULL) { 00335 /* Clamp offset level (Cb signal) */ 00336 if (((uint32_t)p_center_clamp->blanklevel_cb & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00337 vdec_error = VDEC_ERR_PARAM ; 00338 goto END; 00339 } 00340 /* Clamp offset level (Cr signal) */ 00341 if (((uint32_t)p_center_clamp->blanklevel_cr & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00342 vdec_error = VDEC_ERR_PARAM ; 00343 goto END; 00344 } 00345 /* Digital clamp pulse horizontal start position (Cb/Cr signal) */ 00346 if (((uint32_t)p_center_clamp->dcppos_c & (uint32_t)(~VDEC_CPARA_RANGE_0X000000FF)) != 0u) { 00347 vdec_error = VDEC_ERR_PARAM ; 00348 goto END; 00349 } 00350 } 00351 /* Noise detection */ 00352 p_noise_det = param->noise_det ; 00353 if (p_noise_det != NULL) { 00354 /* Video signal for autocorrelation function */ 00355 if (p_noise_det->acfinput >= VDEC_ACFINPUT_NUM) { 00356 vdec_error = VDEC_ERR_PARAM ; 00357 goto END; 00358 } 00359 /* Delay time for autocorrelation function calculation */ 00360 if (((uint32_t)p_noise_det->acflagtime & (uint32_t)(~VDEC_CPARA_RANGE_0X0000001F)) != 0u) { 00361 vdec_error = VDEC_ERR_PARAM ; 00362 goto END; 00363 } 00364 /* Smoothing parameter of autocorrelation function data */ 00365 if (((uint32_t)p_noise_det->acffilter & (uint32_t)(~VDEC_CPARA_RANGE_0X00000003)) != 0u) { 00366 vdec_error = VDEC_ERR_PARAM ; 00367 goto END; 00368 } 00369 } 00370 END: 00371 return vdec_error; 00372 } /* End of function VDEC_DigitalClampCheckParam() */ 00373 00374 /**************************************************************************//** 00375 * @brief Checks on output adjustment parameter 00376 * @param[in] param : Output adjustment parameter 00377 * @retval Error code 00378 *****************************************************************************/ 00379 vdec_error_t VDEC_OutputCheckParam (const vdec_output_t * const param) 00380 { 00381 vdec_error_t vdec_error; 00382 00383 vdec_error = VDEC_OK ; 00384 00385 if (param == NULL) { 00386 vdec_error = VDEC_ERR_PARAM ; 00387 goto END; 00388 } 00389 /* Y signal gain coefficient */ 00390 if (((uint32_t)param->y_gain2 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00391 vdec_error = VDEC_ERR_PARAM ; 00392 goto END; 00393 } 00394 /* Cb signal gain coefficient */ 00395 if (((uint32_t)param->cb_gain2 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00396 vdec_error = VDEC_ERR_PARAM ; 00397 goto END; 00398 } 00399 /* Cr signal gain coefficient */ 00400 if (((uint32_t)param->cr_gain2 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00401 vdec_error = VDEC_ERR_PARAM ; 00402 goto END; 00403 } 00404 END: 00405 return vdec_error; 00406 } /* End of function VDEC_OutputCheckParam() */ 00407 00408 /**************************************************************************//** 00409 * @brief Checks on noise reduction LPF parameter 00410 * @param[in] p_noise_rd_lpf : Noise reduction LPF parameter 00411 * @retval Error code 00412 *****************************************************************************/ 00413 static vdec_error_t NoiseReductionLPFCheckParam (const vdec_noise_rd_lpf_t * const p_noise_rd_lpf) 00414 { 00415 vdec_error_t vdec_error; 00416 00417 vdec_error = VDEC_OK ; 00418 00419 /* Noise reduction LPF */ 00420 if (p_noise_rd_lpf != NULL) { 00421 /* LPF cutoff frequency before vertical sync separation */ 00422 if (p_noise_rd_lpf->lpfvsync >= VDEC_LPF_VSYNC_NUM) { 00423 vdec_error = VDEC_ERR_PARAM ; 00424 goto END; 00425 } 00426 /* LPF cutoff frequency before horizontal sync separation */ 00427 if (p_noise_rd_lpf->lpfhsync >= VDEC_LPF_HSYNC_NUM) { 00428 vdec_error = VDEC_ERR_PARAM ; 00429 goto END; 00430 } 00431 } 00432 END: 00433 return vdec_error; 00434 } /* End of function NoiseReductionLPFCheckParam() */ 00435 00436 /**************************************************************************//** 00437 * @brief Checks on auto level control sync slicer parameter 00438 * @param[in] p_sync_slicer : Auto level control sync slicer parameter 00439 * @retval Error code 00440 *****************************************************************************/ 00441 static vdec_error_t SyncSlicerCheckParam (const vdec_sync_slicer_t * const p_sync_slicer) 00442 { 00443 vdec_error_t vdec_error; 00444 00445 vdec_error = VDEC_OK ; 00446 00447 /* Sync slicer */ 00448 if (p_sync_slicer != NULL) { 00449 /* Reference level operation speed control for composite sync separation (for Hsync signal) */ 00450 if (p_sync_slicer->velocityshift_h >= VDEC_VELOCITY_SHIFT_NUM) { 00451 vdec_error = VDEC_ERR_PARAM ; 00452 goto END; 00453 } 00454 /* Auto-slice level setting for composite sync separator circuit (for Hsync signal) */ 00455 if (p_sync_slicer->slicermode_h >= VDEC_SLICE_MODE_NUM) { 00456 vdec_error = VDEC_ERR_PARAM ; 00457 goto END; 00458 } 00459 /* Auto-slice level setting for composite sync separation circuit (for Vsync signal) */ 00460 if (p_sync_slicer->slicermode_v >= VDEC_SLICE_MODE_NUM) { 00461 vdec_error = VDEC_ERR_PARAM ; 00462 goto END; 00463 } 00464 /* Max ratio of horizontal cycle to horizontal sync signal pulse width 00465 and min ratio of horizontal cycle to horizontal sync signal pulse width (for Hsync signal) */ 00466 if ((((uint32_t)p_sync_slicer->syncmaxduty_h & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) || 00467 (((uint32_t)p_sync_slicer->syncminduty_h & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u)) { 00468 vdec_error = VDEC_ERR_PARAM ; 00469 goto END; 00470 } 00471 /* Clipping level */ 00472 if (p_sync_slicer->ssclipsel >= VDEC_CLIP_LV_NUM) { 00473 vdec_error = VDEC_ERR_PARAM ; 00474 goto END; 00475 } 00476 /* Slice level for composite sync signal separation (for Hsync signal) */ 00477 if (((uint32_t)p_sync_slicer->csyncslice_h & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00478 vdec_error = VDEC_ERR_PARAM ; 00479 goto END; 00480 } 00481 /* Max ratio of horizontal cycle to horizontal sync signal pulse width 00482 and min ratio of horizontal cycle to horizontal sync signal pulse width (for Vsync signal) */ 00483 if ((((uint32_t)p_sync_slicer->syncmaxduty_v & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) || 00484 (((uint32_t)p_sync_slicer->syncminduty_v & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u)) { 00485 vdec_error = VDEC_ERR_PARAM ; 00486 goto END; 00487 } 00488 /* Threshold for vertical sync separation */ 00489 if (((uint32_t)p_sync_slicer->vsyncslice & (uint32_t)(~VDEC_CPARA_RANGE_0X0000001F)) != 0u) { 00490 vdec_error = VDEC_ERR_PARAM ; 00491 goto END; 00492 } 00493 /* Slice level for composite sync signal separation (for Vsync signal) */ 00494 if (((uint32_t)p_sync_slicer->csyncslice_v & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00495 vdec_error = VDEC_ERR_PARAM ; 00496 goto END; 00497 } 00498 } 00499 END: 00500 return vdec_error; 00501 } /* End of function SyncSlicerCheckParam() */ 00502 00503 /**************************************************************************//** 00504 * @brief Checks on horizontal AFC parameter 00505 * @param[in] p_horizontal_afc : Horizontal AFC parameter 00506 * @retval Error code 00507 *****************************************************************************/ 00508 static vdec_error_t HorizontalAFCCheckParam (const vdec_horizontal_afc_t * const p_horizontal_afc) 00509 { 00510 vdec_error_t vdec_error; 00511 00512 vdec_error = VDEC_OK ; 00513 00514 /* Horizontal AFC */ 00515 if (p_horizontal_afc != NULL) { 00516 /* Horizontal AFC loop gain */ 00517 if (((uint32_t)p_horizontal_afc->hafcgain & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) { 00518 vdec_error = VDEC_ERR_PARAM ; 00519 goto END; 00520 } 00521 /* Horizontal AFC center oscillation frequency */ 00522 if (((uint32_t)p_horizontal_afc->hafcgain & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00523 vdec_error = VDEC_ERR_PARAM ; 00524 goto END; 00525 } 00526 /* Start line of horizontal AFC normal operation */ 00527 if (((uint32_t)p_horizontal_afc->hafcstart & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) { 00528 vdec_error = VDEC_ERR_PARAM ; 00529 goto END; 00530 } 00531 /* Maximum oscillation frequency of horizontal AFC */ 00532 if (((uint32_t)p_horizontal_afc->hafcmax & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00533 vdec_error = VDEC_ERR_PARAM ; 00534 goto END; 00535 } 00536 /* End line of horizontal AFC normal operation */ 00537 if (((uint32_t)p_horizontal_afc->hafcend & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) { 00538 vdec_error = VDEC_ERR_PARAM ; 00539 goto END; 00540 } 00541 /* Horizontal AFC VBI period operating mode */ 00542 if (p_horizontal_afc->hafcmode >= VDEC_HAFCMD_NUM) { 00543 vdec_error = VDEC_ERR_PARAM ; 00544 goto END; 00545 } 00546 /* Min oscillation frequency of horizontal AFC */ 00547 if (((uint32_t)p_horizontal_afc->hafcmin & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00548 vdec_error = VDEC_ERR_PARAM ; 00549 goto END; 00550 } 00551 /* Phase comparator feedback adjust for low sync signal lock stability */ 00552 if (p_horizontal_afc->phdet_div >= VDEC_PHDET_DIV_NUM) { 00553 vdec_error = VDEC_ERR_PARAM ; 00554 goto END; 00555 } 00556 } 00557 END: 00558 return vdec_error; 00559 } /* End of function HorizontalAFCCheckParam() */ 00560 00561 /**************************************************************************//** 00562 * @brief Checks on vertical count-down parameter 00563 * @param[in] p_vcount_down : Vertical count-down parameter 00564 * @retval Error code 00565 *****************************************************************************/ 00566 static vdec_error_t VerticalCountdownCheckParam (const vdec_vcount_down_t * const p_vcount_down) 00567 { 00568 vdec_error_t vdec_error; 00569 00570 vdec_error = VDEC_OK ; 00571 00572 /* Vertical countdown */ 00573 if (p_vcount_down != NULL) { 00574 /* Vertical countdown center oscillation frequency */ 00575 if (p_vcount_down->vcddefault >= VDEC_VCD_FRQ_NUM) { 00576 vdec_error = VDEC_ERR_PARAM ; 00577 goto END; 00578 } 00579 /* Vertical countdown sync area */ 00580 if (((uint32_t)p_vcount_down->vcdwindow & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00581 vdec_error = VDEC_ERR_PARAM ; 00582 goto END; 00583 } 00584 /* Vertical countdown minimum oscillation frequency */ 00585 if (((uint32_t)p_vcount_down->vcdoffset & (uint32_t)(~VDEC_CPARA_RANGE_0X0000001F)) != 0u) { 00586 vdec_error = VDEC_ERR_PARAM ; 00587 goto END; 00588 } 00589 } 00590 END: 00591 return vdec_error; 00592 } /* End of function VerticalCountdownCheckParam() */ 00593 00594 /**************************************************************************//** 00595 * @brief Checks on AGC/PGA parameter 00596 * @param[in] p_agc : AGC/PGA parameter 00597 * @retval Error code 00598 *****************************************************************************/ 00599 static vdec_error_t AgcPgaCheckParam (const vdec_agc_t * const p_agc) 00600 { 00601 vdec_error_t vdec_error; 00602 00603 vdec_error = VDEC_OK ; 00604 00605 /* AGC / PGA */ 00606 if (p_agc != NULL) { 00607 /* AGC response speed */ 00608 if (((uint32_t)p_agc->agcresponse & (uint32_t)(~VDEC_CPARA_RANGE_0X00000007)) != 0u) { 00609 vdec_error = VDEC_ERR_PARAM ; 00610 goto END; 00611 } 00612 /* Sync signal reference amplitude */ 00613 if (((uint32_t)p_agc->agclevel & (uint32_t)(~VDEC_CPARA_RANGE_0X000001FF)) != 0u) { 00614 vdec_error = VDEC_ERR_PARAM ; 00615 goto END; 00616 } 00617 /* AGC gain adjustment accuracy */ 00618 if (((uint32_t)p_agc->agcprecis & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00619 vdec_error = VDEC_ERR_PARAM ; 00620 goto END; 00621 } 00622 /* PGA gain */ 00623 if (((uint32_t)p_agc->pga_gain & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00624 vdec_error = VDEC_ERR_PARAM ; 00625 goto END; 00626 } 00627 } 00628 END: 00629 return vdec_error; 00630 } /* End of function AgcPgaCheckParam() */ 00631 00632 /**************************************************************************//** 00633 * @brief Checks on peak limiter control parameter 00634 * @param[in] p_peak_limiter : Peak limiter control parameter 00635 * @retval Error code 00636 *****************************************************************************/ 00637 static vdec_error_t PeakLimiterControlCheckParam (const vdec_peak_limiter_t * const p_peak_limiter) 00638 { 00639 vdec_error_t vdec_error; 00640 00641 vdec_error = VDEC_OK ; 00642 00643 /* Peak limiter control */ 00644 if (p_peak_limiter != NULL) { 00645 /* Peak luminance value to operate peak limiter */ 00646 if (p_peak_limiter->peaklevel >= VDEC_PEAKLV_NUM) { 00647 vdec_error = VDEC_ERR_PARAM ; 00648 goto END; 00649 } 00650 00651 /* Response speed with peak limiter gain decreased */ 00652 if (((uint32_t)p_peak_limiter->peakattack & (uint32_t)(~VDEC_CPARA_RANGE_0X00000003)) != 0u) { 00653 vdec_error = VDEC_ERR_PARAM ; 00654 goto END; 00655 } 00656 /* Response speed with peak limiter gain increased */ 00657 if (((uint32_t)p_peak_limiter->peakrelease & (uint32_t)(~VDEC_CPARA_RANGE_0X00000003)) != 0u) { 00658 vdec_error = VDEC_ERR_PARAM ; 00659 goto END; 00660 } 00661 /* Maximum compression rate of peak limiter */ 00662 if (p_peak_limiter->peakratio >= VDEC_PEAKRATIO_NUM) { 00663 vdec_error = VDEC_ERR_PARAM ; 00664 goto END; 00665 } 00666 /* Allowable number of overflowing pixels */ 00667 if (((uint32_t)p_peak_limiter->maxpeaksamples & (uint32_t)(~VDEC_CPARA_RANGE_0X000000FF)) != 0u) { 00668 vdec_error = VDEC_ERR_PARAM ; 00669 goto END; 00670 } 00671 } 00672 END: 00673 return vdec_error; 00674 } /* End of function PeakLimiterControlCheckParam() */ 00675 00676 /**************************************************************************//** 00677 * @brief Checks on over-range control parameter 00678 * @param[in] p_over_range : Over-range control parameter 00679 * @retval Error code 00680 *****************************************************************************/ 00681 static vdec_error_t OverRangeControlCheckParam (const vdec_over_range_t * const p_over_range) 00682 { 00683 vdec_error_t vdec_error; 00684 00685 vdec_error = VDEC_OK ; 00686 00687 /* Over-range control */ 00688 if (p_over_range != NULL) { 00689 /* A/D over-threshold level (between levels 0 and 1) */ 00690 if (((uint32_t)p_over_range->radj_o_level0 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00691 vdec_error = VDEC_ERR_PARAM ; 00692 goto END; 00693 } 00694 /* A/D under-threshold level (between levels 2 and 3) */ 00695 if (((uint32_t)p_over_range->radj_u_level0 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00696 vdec_error = VDEC_ERR_PARAM ; 00697 goto END; 00698 } 00699 /* A/D over-threshold level (between levels 1 and 2) */ 00700 if (((uint32_t)p_over_range->radj_o_level1 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00701 vdec_error = VDEC_ERR_PARAM ; 00702 goto END; 00703 } 00704 /* A/D under-threshold level (between levels 1 and 2) */ 00705 if (((uint32_t)p_over_range->radj_u_level1 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00706 vdec_error = VDEC_ERR_PARAM ; 00707 goto END; 00708 } 00709 /* A/D over-threshold level (between levels 2 and 3) */ 00710 if (((uint32_t)p_over_range->radj_o_level2 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00711 vdec_error = VDEC_ERR_PARAM ; 00712 goto END; 00713 } 00714 /* A/D under-threshold level (between levels 0 and 1) */ 00715 if (((uint32_t)p_over_range->radj_u_level2 & (uint32_t)(~VDEC_CPARA_RANGE_0X000003FF)) != 0u) { 00716 vdec_error = VDEC_ERR_PARAM ; 00717 goto END; 00718 } 00719 } 00720 END: 00721 return vdec_error; 00722 } /* End of function OverRangeControlCheckParam() */ 00723 00724 /**************************************************************************//** 00725 * @brief Checks on Y/C separation control parameter 00726 * @param[in] p_yc_sep_ctrl : Y/C separation control parameter 00727 * @retval Error code 00728 *****************************************************************************/ 00729 static vdec_error_t YcSeparationControlCheckParam (const vdec_yc_sep_ctrl_t * const p_yc_sep_ctrl) 00730 { 00731 vdec_error_t vdec_error; 00732 00733 vdec_error = VDEC_OK ; 00734 00735 /* Y/C separation control */ 00736 if (p_yc_sep_ctrl != NULL) { 00737 /* Two-dimensional Y/C separation filter select coefficient (K15, K13, and K11) */ 00738 if ((((uint32_t)p_yc_sep_ctrl->k15 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) || 00739 (((uint32_t)p_yc_sep_ctrl->k13 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) || 00740 (((uint32_t)p_yc_sep_ctrl->k11 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u)) { 00741 vdec_error = VDEC_ERR_PARAM ; 00742 goto END; 00743 } 00744 /* Two-dimensional Y/C separation filter select coefficient (K16, K14, and K12) */ 00745 if ((((uint32_t)p_yc_sep_ctrl->k16 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) || 00746 (((uint32_t)p_yc_sep_ctrl->k14 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) || 00747 (((uint32_t)p_yc_sep_ctrl->k12 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u)) { 00748 vdec_error = VDEC_ERR_PARAM ; 00749 goto END; 00750 } 00751 /* Two-dimensional Y/C separation filter select coefficient (K22A and K21A) */ 00752 if ((((uint32_t)p_yc_sep_ctrl->k22a & (uint32_t)(~VDEC_CPARA_RANGE_0X000000FF)) != 0u) || 00753 (((uint32_t)p_yc_sep_ctrl->k21a & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u)) { 00754 vdec_error = VDEC_ERR_PARAM ; 00755 goto END; 00756 } 00757 /* Two-dimensional Y/C separation filter select coefficient (K22B and K21B) */ 00758 if ((((uint32_t)p_yc_sep_ctrl->k22b & (uint32_t)(~VDEC_CPARA_RANGE_0X000000FF)) != 0u) || 00759 (((uint32_t)p_yc_sep_ctrl->k21b & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u)) { 00760 vdec_error = VDEC_ERR_PARAM ; 00761 goto END; 00762 } 00763 /* Two-dimensional Y/C separation filter select coefficient (K23B, K23A, and K24) */ 00764 if ((((uint32_t)p_yc_sep_ctrl->k23b & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) || 00765 (((uint32_t)p_yc_sep_ctrl->k23a & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) || 00766 (((uint32_t)p_yc_sep_ctrl->k24 & (uint32_t)(~VDEC_CPARA_RANGE_0X0000001F)) != 0u)) { 00767 vdec_error = VDEC_ERR_PARAM ; 00768 goto END; 00769 } 00770 /* Mixing ratio of signal after passing horizontal filter 00771 to signal after passing former-stage horizontal filter */ 00772 if (p_yc_sep_ctrl->hsel_mix_y >= VDEC_FILMIX_RATIO_NUM) { 00773 vdec_error = VDEC_ERR_PARAM ; 00774 goto END; 00775 } 00776 /* Mixing ratio of signal after passing vertical filter 00777 to signal after passing former-stage horizontal/vertical filter */ 00778 if (p_yc_sep_ctrl->vsel_mix_y >= VDEC_FILMIX_RATIO_NUM) { 00779 vdec_error = VDEC_ERR_PARAM ; 00780 goto END; 00781 } 00782 /* Mixing ratio of signal after passing horizontal/vertical filter 00783 to signal after passing former-stage horizontal/vertical filter */ 00784 if (p_yc_sep_ctrl->hvsel_mix_y >= VDEC_FILMIX_RATIO_NUM) { 00785 vdec_error = VDEC_ERR_PARAM ; 00786 goto END; 00787 } 00788 /* Vertical luminance detection level for correlation detection filter */ 00789 if (((uint32_t)p_yc_sep_ctrl->v_y_level & (uint32_t)(~VDEC_CPARA_RANGE_0X000001FF)) != 0u) { 00790 vdec_error = VDEC_ERR_PARAM ; 00791 goto END; 00792 } 00793 00794 /* Mixing ratio of c signal after passing horizontal/vertical adaptive filter 00795 to signal after passing correlation detection filter */ 00796 if (p_yc_sep_ctrl->det2_mix_c >= VDEC_FILMIX_RATIO_NUM) { 00797 vdec_error = VDEC_ERR_PARAM ; 00798 goto END; 00799 } 00800 /* Mixing ratio of C signal for Y generation after passing 00801 horizontal/vertical adaptive filter to signal after passing correlation */ 00802 if (p_yc_sep_ctrl->det2_mix_y >= VDEC_FILMIX_RATIO_NUM) { 00803 vdec_error = VDEC_ERR_PARAM ; 00804 goto END; 00805 } 00806 /* Two-dimensional cascade/TAKE-OFF filter mode select */ 00807 if (p_yc_sep_ctrl->fil2_mode_2d >= VDEC_2DFIL_MDSEL_NUM) { 00808 vdec_error = VDEC_ERR_PARAM ; 00809 goto END; 00810 } 00811 } 00812 END: 00813 return vdec_error; 00814 } /* End of function YcSeparationControlCheckParam() */ 00815 00816 /**************************************************************************//** 00817 * @brief Checks on chroma filter TAP coefficient for Y/C separation 00818 * @param[in] fil2_2d : Chroma filter TAP coefficient for Y/C separation 00819 * @retval Error code 00820 *****************************************************************************/ 00821 static vdec_error_t FilterTAPsCoefficientCheckParam (const vdec_chrfil_tap_t * const fil2_2d) 00822 { 00823 int32_t tap_coef; 00824 const uint16_t * taps; 00825 vdec_error_t vdec_error; 00826 00827 vdec_error = VDEC_OK ; 00828 00829 if (fil2_2d != NULL) { 00830 taps = fil2_2d->fil2_2d_f ; 00831 for (tap_coef = 0; tap_coef < VDEC_CHRFIL_TAPCOEF_NUM; tap_coef++) { 00832 if (((uint32_t)*taps & (uint32_t)(~VDEC_CPARA_RANGE_0X00001FFF)) != 0u) { 00833 vdec_error = VDEC_ERR_PARAM ; 00834 goto END; 00835 } 00836 taps++; 00837 } 00838 } 00839 END: 00840 return vdec_error; 00841 } /* End of function FilterTAPsCoefficientCheckParam() */ 00842 00843 /**************************************************************************//** 00844 * @brief Checks on color system detection parameter 00845 * @param[in] p_chrmdec_ctrl : Color system detection parameter 00846 * @retval Error code 00847 *****************************************************************************/ 00848 static vdec_error_t ChromaDecodingControlCheckParam (const vdec_chrmdec_ctrl_t * const p_chrmdec_ctrl) 00849 { 00850 vdec_error_t vdec_error; 00851 00852 vdec_error = VDEC_OK ; 00853 00854 /* Chroma decoding control */ 00855 if (p_chrmdec_ctrl != NULL) { 00856 /* Default color system */ 00857 if (p_chrmdec_ctrl->defaultsys >= VDEC_COL_SYS_NUM ) { 00858 vdec_error = VDEC_ERR_PARAM ; 00859 goto END; 00860 } 00861 /* Luminance signal delay adjustment */ 00862 if (((uint32_t)p_chrmdec_ctrl->lumadelay & (uint32_t)(~VDEC_CPARA_RANGE_0X0000001F)) != 0u) { 00863 vdec_error = VDEC_ERR_PARAM ; 00864 goto END; 00865 } 00866 /* Averaging processing for pre-demodulated line */ 00867 if ((p_chrmdec_ctrl->demodmode != VDEC_DEMOD_MD_NO ) && (p_chrmdec_ctrl->demodmode != VDEC_DEMOD_MD_PAL )) { 00868 vdec_error = VDEC_ERR_PARAM ; 00869 goto END; 00870 } 00871 } 00872 END: 00873 return vdec_error; 00874 } /* End of function ChromaDecodingControlCheckParam() */ 00875 00876 /**************************************************************************//** 00877 * @brief Checks on BCO parameter 00878 * @param[in] p_burst_lock : BCO parameter 00879 * @retval Error code 00880 *****************************************************************************/ 00881 static vdec_error_t BurstLockCheckParam (const vdec_burst_lock_t * const p_burst_lock) 00882 { 00883 vdec_error_t vdec_error; 00884 00885 vdec_error = VDEC_OK ; 00886 00887 /* Burst lock */ 00888 if (p_burst_lock != NULL) { 00889 /* Burst lock PLL lock range */ 00890 if (p_burst_lock->lockrange >= VDEC_LOCK_RANGE_NUM) { 00891 vdec_error = VDEC_ERR_PARAM ; 00892 goto END; 00893 } 00894 /* Burst lock PLL loop gain */ 00895 if (((uint32_t)p_burst_lock->loopgain & (uint32_t)(~VDEC_CPARA_RANGE_0X00000003)) != 0u) { 00896 vdec_error = VDEC_ERR_PARAM ; 00897 goto END; 00898 } 00899 /* Level for burst lock PLL to re-search free-run frequency */ 00900 if (((uint32_t)p_burst_lock->locklimit & (uint32_t)(~VDEC_CPARA_RANGE_0X00000003)) != 0u) { 00901 vdec_error = VDEC_ERR_PARAM ; 00902 goto END; 00903 } 00904 /* burst gate pulse position check */ 00905 if (((uint32_t)p_burst_lock->bgpcheck & (uint32_t)(~VDEC_CPARA_RANGE_0X00000001)) != 0u) { 00906 vdec_error = VDEC_ERR_PARAM ; 00907 goto END; 00908 } 00909 /* burst gate pulse width */ 00910 if (((uint32_t)p_burst_lock->bgpwidth & (uint32_t)(~VDEC_CPARA_RANGE_0X0000007F)) != 0u) { 00911 vdec_error = VDEC_ERR_PARAM ; 00912 goto END; 00913 } 00914 /* burst gate pulse start position */ 00915 if (((uint32_t)p_burst_lock->bgpstart & (uint32_t)(~VDEC_CPARA_RANGE_0X000000FF)) != 0u) { 00916 vdec_error = VDEC_ERR_PARAM ; 00917 goto END; 00918 } 00919 } 00920 END: 00921 return vdec_error; 00922 } /* End of function BurstLockCheckParam() */ 00923 00924 /**************************************************************************//** 00925 * @brief Checks on ACC and color killer parameter 00926 * @param[in] p_acc : ACC and color killer parameter 00927 * @retval Error code 00928 *****************************************************************************/ 00929 static vdec_error_t AccColorKillerCheckParam (const vdec_acc_t * const p_acc) 00930 { 00931 vdec_error_t vdec_error; 00932 00933 vdec_error = VDEC_OK ; 00934 00935 /* ACC/color killer */ 00936 if (p_acc != NULL) { 00937 /* Maximum ACC Gain */ 00938 if (p_acc->accmaxgain >= VDEC_ACC_MAX_GAIN_NUM) { 00939 vdec_error = VDEC_ERR_PARAM ; 00940 goto END; 00941 } 00942 /* ACC reference color burst amplitude */ 00943 if (((uint32_t)p_acc->acclevel & (uint32_t)(~VDEC_CPARA_RANGE_0X000001FF)) != 0u) { 00944 vdec_error = VDEC_ERR_PARAM ; 00945 goto END; 00946 } 00947 /* Chroma manual gain (sub) */ 00948 if (p_acc->chromasubgain >= VDEC_CHRM_SB_GAIN_NUM) { 00949 vdec_error = VDEC_ERR_PARAM ; 00950 goto END; 00951 } 00952 /* Chroma manual gain (main) */ 00953 if (((uint32_t)p_acc->chromamaingain & (uint32_t)(~VDEC_CPARA_RANGE_0X000001FF)) != 0u) { 00954 vdec_error = VDEC_ERR_PARAM ; 00955 goto END; 00956 } 00957 /* ACC response speed */ 00958 if (((uint32_t)p_acc->accresponse & (uint32_t)(~VDEC_CPARA_RANGE_0X00000003)) != 0u) { 00959 vdec_error = VDEC_ERR_PARAM ; 00960 goto END; 00961 } 00962 /* ACC gain adjustment accuracy */ 00963 if (((uint32_t)p_acc->accprecis & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00964 vdec_error = VDEC_ERR_PARAM ; 00965 goto END; 00966 } 00967 /* Color killer operation start point */ 00968 if (((uint32_t)p_acc->killerlevel & (uint32_t)(~VDEC_CPARA_RANGE_0X0000003F)) != 0u) { 00969 vdec_error = VDEC_ERR_PARAM ; 00970 goto END; 00971 } 00972 /* The offset level to turn off the color killer */ 00973 if (((uint32_t)p_acc->killeroffset & (uint32_t)(~VDEC_CPARA_RANGE_0X0000000F)) != 0u) { 00974 vdec_error = VDEC_ERR_PARAM ; 00975 goto END; 00976 } 00977 } 00978 END: 00979 return vdec_error; 00980 } /* End of function AccColorKillerCheckParam() */ 00981 00982 #endif /* R_VDEC_CHECK_PARAMETERS */ 00983
Generated on Tue Jul 12 2022 15:08:46 by
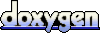