Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdec.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdec.h 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDEC driver API definitions 00029 ******************************************************************************/ 00030 00031 #ifndef R_VDEC_H 00032 #define R_VDEC_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 #include "r_vdec_user.h" 00038 00039 00040 #ifdef __cplusplus 00041 extern "C" 00042 { 00043 #endif /* __cplusplus */ 00044 00045 00046 /****************************************************************************** 00047 Macro definitions 00048 ******************************************************************************/ 00049 /*! The number of chroma filter TAP coefficient values for Y/C separation */ 00050 #define VDEC_CHRFIL_TAPCOEF_NUM (9) 00051 00052 00053 /****************************************************************************** 00054 Typedef definitions 00055 ******************************************************************************/ 00056 /*! Error codes of the VDEC driver */ 00057 typedef enum { 00058 VDEC_OK = 0, /*!< Normal termination */ 00059 VDEC_ERR_CHANNEL , /*!< Invalid channel error */ 00060 VDEC_ERR_PARAM , /*!< Parameter error */ 00061 VDEC_ERR_NUM /*!< The number of the error codes */ 00062 } vdec_error_t ; 00063 00064 /*! VDEC channel */ 00065 typedef enum { 00066 VDEC_CHANNEL_0 = 0, /*!< Channel 0 */ 00067 VDEC_CHANNEL_1 , /*!< Channel 1 */ 00068 VDEC_CHANNEL_NUM /*!< The number of channels */ 00069 } vdec_channel_t ; 00070 00071 /*! On/Off */ 00072 typedef enum { 00073 VDEC_OFF = 0, /*!< Off */ 00074 VDEC_ON = 1 /*!< On */ 00075 } vdec_onoff_t ; 00076 00077 /*********************** For R_VDEC_Initialize ***********************/ 00078 /*! Input pin control */ 00079 typedef enum { 00080 VDEC_ADC_VINSEL_VIN1 = 0, /*!< VIN1 input */ 00081 VDEC_ADC_VINSEL_VIN2 , /*!< VIN2 input */ 00082 VDEC_ADC_VINSEL_NUM /*!< The number of input pins */ 00083 } vdec_adc_vinsel_t ; 00084 00085 /*********************** For R_VDEC_ActivePeriod ***********************/ 00086 /*! Active image period parameter */ 00087 typedef struct { 00088 uint16_t srcleft ; /*!< Left end of input video signal capturing area */ 00089 uint16_t srctop ; /*!< Top end of input video signal capturing area */ 00090 uint16_t srcheight ; /*!< Height of input video signal capturing area */ 00091 uint16_t srcwidth ; /*!< Width of input video signal capturing area */ 00092 } vdec_active_period_t ; 00093 00094 /*********************** For R_VDEC_SyncSeparation ***********************/ 00095 /*! LPF cutoff frequency before vertical sync separation */ 00096 typedef enum { 00097 VDEC_LPF_VSYNC_NONE = 0, /*!< None */ 00098 VDEC_LPF_VSYNC_0_94 , /*!< 0.94 MHz */ 00099 VDEC_LPF_VSYNC_0_67 , /*!< 0.67 MHz */ 00100 VDEC_LPF_VSYNC_0_54 , /*!< 0.54 MHz */ 00101 VDEC_LPF_VSYNC_0_47 , /*!< 0.47 MHz */ 00102 VDEC_LPF_VSYNC_0_34 , /*!< 0.34 MHz */ 00103 VDEC_LPF_VSYNC_0_27 , /*!< 0.27 MHz */ 00104 VDEC_LPF_VSYNC_0_23 , /*!< 0.23 MHz */ 00105 VDEC_LPF_VSYNC_NUM 00106 } vdec_lpfvsync_t ; 00107 /*! LPF cutoff frequency before horizontal sync separation */ 00108 typedef enum { 00109 VDEC_LPF_HSYNC_NONE = 0, /*!< None */ 00110 VDEC_LPF_HSYNC_2_15 , /*!< 2.15 MHz */ 00111 VDEC_LPF_HSYNC_1_88 , /*!< 1.88 MHz */ 00112 VDEC_LPF_HSYNC_1_34 , /*!< 1.34 MHz */ 00113 VDEC_LPF_HSYNC_1_07 , /*!< 1.07 MHz */ 00114 VDEC_LPF_HSYNC_0_94 , /*!< 0.94 MHz */ 00115 VDEC_LPF_HSYNC_0_67 , /*!< 0.67 MHz */ 00116 VDEC_LPF_HSYNC_0_54 , /*!< 0.54 MHz */ 00117 VDEC_LPF_HSYNC_NUM 00118 } vdec_lpfhsync_t ; 00119 /*! Noise reduction LPF parameter */ 00120 typedef struct { 00121 vdec_lpfvsync_t lpfvsync ; /*!< LPF cutoff frequency before vertical sync separation */ 00122 vdec_lpfhsync_t lpfhsync ; /*!< LPF cutoff frequency before horizontal sync separation */ 00123 } vdec_noise_rd_lpf_t ; 00124 /*! Reference level operation speed control for composite sync separation (for Hsync signal) */ 00125 typedef enum { 00126 VDEC_VELOCITY_SHIFT_1 = 0, /*!< x1 */ 00127 VDEC_VELOCITY_SHIFT_2 , /*!< x2 */ 00128 VDEC_VELOCITY_SHIFT_4 , /*!< x4 */ 00129 VDEC_VELOCITY_SHIFT_8 , /*!< x8 */ 00130 VDEC_VELOCITY_SHIFT_16 , /*!< x16 */ 00131 VDEC_VELOCITY_SHIFT_32 , /*!< x32 */ 00132 VDEC_VELOCITY_SHIFT_64 , /*!< x64 */ 00133 VDEC_VELOCITY_SHIFT_128 , /*!< x128 */ 00134 VDEC_VELOCITY_SHIFT_256 , /*!< x256 */ 00135 VDEC_VELOCITY_SHIFT_NUM 00136 } vdec_velocityshift_h_t ; 00137 /*! Auto-slice level setting for composite sync separation circuit */ 00138 typedef enum { 00139 VDEC_SLICE_MODE_MANULAL = 0, /*!< Manual setting */ 00140 VDEC_SLICE_MODE_AUTO_25 , /*!< 25% of sync depth (Auto) */ 00141 VDEC_SLICE_MODE_AUTO_50 , /*!< 50% of sync depth (Auto) */ 00142 VDEC_SLICE_MODE_AUTO_75 , /*!< 75% of sync depth (Auto) */ 00143 VDEC_SLICE_MODE_NUM 00144 } vdec_slicermode_t ; 00145 /*! Clipping level */ 00146 typedef enum { 00147 VDEC_CLIP_LV_512 = 0, /*!< 512 */ 00148 VDEC_CLIP_LV_546 , /*!< 546 */ 00149 VDEC_CLIP_LV_580 , /*!< 580 */ 00150 VDEC_CLIP_LV_614 , /*!< 614 */ 00151 VDEC_CLIP_LV_648 , /*!< 648 */ 00152 VDEC_CLIP_LV_682 , /*!< 682 */ 00153 VDEC_CLIP_LV_716 , /*!< 716 */ 00154 VDEC_CLIP_LV_750 , /*!< 750 */ 00155 VDEC_CLIP_LV_785 , /*!< 785 */ 00156 VDEC_CLIP_LV_819 , /*!< 819 */ 00157 VDEC_CLIP_LV_853 , /*!< 853 */ 00158 VDEC_CLIP_LV_887 , /*!< 887 */ 00159 VDEC_CLIP_LV_921 , /*!< 921 */ 00160 VDEC_CLIP_LV_955 , /*!< 955 */ 00161 VDEC_CLIP_LV_989 , /*!< 989 */ 00162 VDEC_CLIP_LV_1023 , /*!< 1023 */ 00163 VDEC_CLIP_LV_NUM 00164 } vdec_ssclipsel_t ; 00165 /*! Sync slicer */ 00166 typedef struct { 00167 vdec_velocityshift_h_t velocityshift_h; /*!< Reference level operation speed control for 00168 composite sync separation (for Hsync signal) */ 00169 vdec_slicermode_t slicermode_h; /*!< Auto-slice level setting for composite sync separation circuit 00170 (for Hsync signal) */ 00171 vdec_slicermode_t slicermode_v; /*!< Auto-slice level setting for composite sync separation circuit 00172 (for Vsync signal) */ 00173 uint16_t syncmaxduty_h; /*!< Max ratio of horizontal cycle 00174 to horizontal sync signal pulse width */ 00175 uint16_t syncminduty_h; /*!< Min ratio of horizontal cycle 00176 to horizontal sync signal pulse width */ 00177 vdec_ssclipsel_t ssclipsel ; /*!< Clipping level */ 00178 uint16_t csyncslice_h; /*!< Slice level for composite sync signal separation 00179 (for Hsync signal) */ 00180 uint16_t syncmaxduty_v; /*!< Max ratio of horizontal cycle 00181 to vertical sync signal pulse width */ 00182 uint16_t syncminduty_v; /*!< Min ratio of horizontal cycle 00183 to horizontal sync signal pulse width */ 00184 vdec_onoff_t vsyncdelay; /*!< Delays the separated vertical sync signal 00185 for 1/4 horizontal cycle */ 00186 uint16_t vsyncslice ; /*!< Threshold for vertical sync separation */ 00187 uint16_t csyncslice_v; /*!< Slice level for composite sync signal separation 00188 (for Vsync signal) */ 00189 } vdec_sync_slicer_t ; 00190 /*! Horizontal AFC VBI period operating mode */ 00191 typedef enum { 00192 VDEC_HAFCMD_FIX_PHST = 0, /*!< Loop gain is fixed and phase comparison is stopped 00193 during VBI period */ 00194 VDEC_HAFCMD_FIX_LGRD , /*!< Loop gain is fixed and loop gain is reduced during VBI period */ 00195 VDEC_HAFCMD_AUTO_PHST , /*!< Loop gain is automatically controlled and 00196 phase comparison is stopped during VBI period */ 00197 VDEC_HAFCMD_AUTO_LGRD , /*!< Loop gain is automatically controlled and loop gain is reduced 00198 during VBI period */ 00199 VDEC_HAFCMD_NUM 00200 } vdec_hafcmode_t ; 00201 /*! Phase comparator feedback adjust for low sync signal lock stability */ 00202 typedef enum { 00203 VDEC_PHDET_DIV_1_1 = 0, /*!< 1/1 */ 00204 VDEC_PHDET_DIV_1_2 , /*!< 1/2 */ 00205 VDEC_PHDET_DIV_1_4 , /*!< 1/4 */ 00206 VDEC_PHDET_DIV_1_8 , /*!< 1/8 */ 00207 VDEC_PHDET_DIV_1_16 , /*!< 1/16 */ 00208 VDEC_PHDET_DIV_1_32 , /*!< 1/32 */ 00209 VDEC_PHDET_DIV_NUM 00210 } vdec_phdet_div_t ; 00211 /*! Horizontal AFC parameter */ 00212 typedef struct { 00213 uint16_t hafcgain ; /*!< Horizontal AFC loop gain */ 00214 uint16_t hafctyp ; /*!< Horizontal AFC center oscillation frequency */ 00215 uint16_t hafcstart; /*!< Start line of horizontal AFC normal operation 00216 (VBI process end line) */ 00217 vdec_onoff_t nox2hosc ; /*!< Disable of horizontal AFC double speed detection */ 00218 uint16_t hafcmax ; /*!< Maximum oscillation frequency of horizontal AFC */ 00219 uint16_t hafcend; /*!< End line of horizontal AFC normal operation 00220 (VBI process start line) */ 00221 vdec_hafcmode_t hafcmode ; /*!< Horizontal AFC VBI period operating mode */ 00222 uint16_t hafcmin ; /*!< Min oscillation frequency of horizontal AFC */ 00223 vdec_onoff_t phdet_fix ; /*!< Forcible or LOWGAIN control */ 00224 vdec_phdet_div_t phdet_div ; /*!< Phase comparator feedback adjust for low sync signal lock stability */ 00225 } vdec_horizontal_afc_t ; 00226 /*! Vertical countdown center oscillation frequency */ 00227 typedef enum { 00228 VDEC_VCD_FRQ_AUTO = 0, /*!< Auto-detection */ 00229 VDEC_VCD_FRQ_50HZ , /*!< 50.00 Hz */ 00230 VDEC_VCD_FRQ_59_94HZ , /*!< 59.94 Hz */ 00231 VDEC_VCD_FRQ_60HZ , /*!< 60.00 Hz */ 00232 VDEC_VCD_FRQ_NUM 00233 } vdec_vcddefault_t ; 00234 /*! Vertical count-down parameter */ 00235 typedef struct { 00236 vdec_onoff_t novcd50_ ; /*!< Vertical countdown 50-Hz oscillation mode */ 00237 vdec_onoff_t novcd60_ ; /*!< Vertical countdown 60-Hz (59.94-Hz) oscillation mode */ 00238 vdec_vcddefault_t vcddefault ; /*!< Vertical countdown center oscillation frequency */ 00239 uint16_t vcdwindow ; /*!< Vertical countdown sync area */ 00240 uint16_t vcdoffset ; /*!< Vertical countdown minimum oscillation frequency */ 00241 } vdec_vcount_down_t ; 00242 /*! AGC/PGA parameter */ 00243 typedef struct { 00244 vdec_onoff_t agcmode ; /*!< A/D converter AGC ON/OFF control & PGA switch */ 00245 vdec_onoff_t doreduce ; /*!< Manual control of sync signal amplitude detection during VBI period */ 00246 vdec_onoff_t noreduce_ ; /*!< Control of sync signal amplitude detection during VBI period */ 00247 uint16_t agcresponse ; /*!< AGC response speed */ 00248 uint16_t agclevel ; /*!< Sync signal reference amplitude */ 00249 uint16_t agcprecis ; /*!< AGC gain adjustment accuracy */ 00250 uint16_t pga_gain ; /*!< PGA gain */ 00251 } vdec_agc_t ; 00252 /*! Peak luminance value to operate peak limiter */ 00253 typedef enum { 00254 VDEC_PEAKLV_LIM_OFF =0, /*!< Limiter OFF */ 00255 VDEC_PEAKLV_1008 , /*!< 1008 LSB */ 00256 VDEC_PEAKLV_992 , /*!< 992 LSB */ 00257 VDEC_PEAKLV_960 , /*!< 960 LSB */ 00258 VDEC_PEAKLV_NUM 00259 } vdec_peaklevel_t ; 00260 /*! Maximum compression rate of peak limiter */ 00261 typedef enum { 00262 VDEC_PEAKRATIO_50 = 0, /*!< Compressed up to 50% */ 00263 VDEC_PEAKRATIO_25 , /*!< Compressed up to 25% */ 00264 VDEC_PEAKRATIO_12_5 , /*!< Compressed up to 12.5% */ 00265 VDEC_PEAKRATIO_0 , /*!< Compressed up to 0% */ 00266 VDEC_PEAKRATIO_NUM 00267 } vdec_peakratio_t ; 00268 /*! Peak limiter control parameter */ 00269 typedef struct { 00270 vdec_peaklevel_t peaklevel ; /*!< Peak luminance value limited by peak limiter (video signal level) */ 00271 uint16_t peakattack ; /*!< Response speed with peak limiter gain decreased */ 00272 uint16_t peakrelease ; /*!< Response speed with peak limiter gain increased */ 00273 vdec_peakratio_t peakratio ; /*!< Maximum compression rate of peak limiter */ 00274 uint16_t maxpeaksamples ; /*!< Allowable number of overflowing pixels */ 00275 } vdec_peak_limiter_t ; 00276 /*! Sync separation parameter */ 00277 typedef struct { 00278 vdec_noise_rd_lpf_t * noise_rd_lpf ; /*!< Noise reduction LPF parameter */ 00279 vdec_sync_slicer_t * sync_slicer ; /*!< Auto level control sync slicer parameter */ 00280 vdec_horizontal_afc_t * horizontal_afc ; /*!< Horizontal AFC parameter */ 00281 vdec_vcount_down_t * vcount_down ; /*!< Vertical count-down parameter */ 00282 vdec_agc_t * agc ; /*!< AGC/PGA parameter */ 00283 vdec_peak_limiter_t * peak_limiter ; /*!< Peak limiter control parameter */ 00284 } vdec_sync_separation_t ; 00285 00286 /*********************** For R_VDEC_YcSeparation ***********************/ 00287 /*! Over-range control parameter */ 00288 typedef struct { 00289 uint16_t radj_o_level0 ; /*!< A/D over-threshold level (between levels 0 and 1) */ 00290 uint16_t radj_u_level0 ; /*!< A/D under-threshold level (between levels 2 and 3) */ 00291 uint16_t radj_o_level1 ; /*!< A/D over-threshold level (between levels 1 and 2) */ 00292 uint16_t radj_u_level1 ; /*!< A/D under-threshold level (between levels 1 and 2) */ 00293 uint16_t radj_o_level2 ; /*!< A/D over-threshold level (between levels 2 and 3) */ 00294 uint16_t radj_u_level2 ; /*!< A/D under-threshold level (between levels 0 and 1) */ 00295 vdec_onoff_t ucmp_sw ; /*!< Over-range detection enable */ 00296 vdec_onoff_t dcmp_sw ; /*!< Under-range detection enable */ 00297 vdec_onoff_t hwide_sw ; /*!< Horizontal enlargement of over/under-range level */ 00298 } vdec_over_range_t ; 00299 /*! Latter-stage BPF select */ 00300 typedef enum { 00301 VDEC_LSTG_BPFSEL_BYPASS = 0, /*!< Bypass */ 00302 VDEC_LSTG_BPFSEL_17TAP = 1 /*!< 17 TAP */ 00303 } vdec_lstg_bpfsel_t ; 00304 /*! Former-stage BPF select */ 00305 typedef enum { 00306 VDEC_FSTG_BPFSEL_17TAP = 0, /*!< 17 TAP */ 00307 VDEC_FSTG_BPFSEL_9TAP = 1 /*!< 9 TAP */ 00308 } vdec_fstg_bpfsel_t ; 00309 /*! Horizontal filter and horizontal/vertical filter bandwidth switch signal */ 00310 typedef enum { 00311 VDEC_HFIL_TAP_SEL_17TAP = 0, /*!< 17 TAP */ 00312 VDEC_HFIL_TAP_SEL_9TAP = 1 /*!< 9 TAP */ 00313 } vdec_hfil_tap_sel_t ; 00314 /*! Mixing ratio of signals after passing filters */ 00315 typedef enum { 00316 VDEC_FILMIX_RATIO_0 = 0, /*!< 0% */ 00317 VDEC_FILMIX_RATIO_12_5 , /*!< 12.5% */ 00318 VDEC_FILMIX_RATIO_25 , /*!< 25% */ 00319 VDEC_FILMIX_RATIO_37_5 , /*!< 37.5% */ 00320 VDEC_FILMIX_RATIO_50 , /*!< 50% */ 00321 VDEC_FILMIX_RATIO_62_5 , /*!< 62.5% */ 00322 VDEC_FILMIX_RATIO_75 , /*!< 75% */ 00323 VDEC_FILMIX_RATIO_87_5 , /*!< 87.5% */ 00324 VDEC_FILMIX_RATIO_100 , /*!< 100% */ 00325 VDEC_FILMIX_RATIO_NUM 00326 } vdec_filmix_ratio_t ; 00327 /*! Two-dimensional cascade/TAKE-OFF filter mode select */ 00328 typedef enum { 00329 VDEC_2DFIL_MDSEL_BYPASS = 0, /*!< Bypass */ 00330 VDEC_2DFIL_MDSEL_CASCADE , /*!< Cascade filter */ 00331 VDEC_2DFIL_MDSEL_TAKEOFF , /*!< TAKE-OFF filter */ 00332 VDEC_2DFIL_MDSEL_NUM 00333 } vdec_2dfil_mdsel_t ; 00334 /*! Two-dimensional cascade filter select */ 00335 typedef enum { 00336 VDEC_2D_FIL_SEL_BYPASS = 0, /*!< Bypass */ 00337 VDEC_2D_FIL_SEL_17TAP = 1 /*!< 17 TAP */ 00338 } vdec_2d_fil_sel_t ; 00339 /*! Y/C separation control parameter */ 00340 typedef struct { 00341 uint16_t k15 ; /*!< Two-dimensional Y/C separation filter select coefficient, K15 */ 00342 uint16_t k13 ; /*!< Two-dimensional Y/C separation filter select coefficient, K13 */ 00343 uint16_t k11 ; /*!< Two-dimensional Y/C separation filter select coefficient, K11 */ 00344 uint16_t k16 ; /*!< Two-dimensional Y/C separation filter select coefficient, K16 */ 00345 uint16_t k14 ; /*!< Two-dimensional Y/C separation filter select coefficient, K14 */ 00346 uint16_t k12 ; /*!< Two-dimensional Y/C separation filter select coefficient, K12 */ 00347 uint16_t k22a ; /*!< Two-dimensional Y/C separation filter select coefficient, K22A */ 00348 uint16_t k21a ; /*!< Two-dimensional Y/C separation filter select coefficient, K21A */ 00349 uint16_t k22b ; /*!< Two-dimensional Y/C separation filter select coefficient, K22B */ 00350 uint16_t k21b ; /*!< Two-dimensional Y/C separation filter select coefficient, K21B */ 00351 uint16_t k23b ; /*!< Two-dimensional Y/C separation filter select coefficient, K23B */ 00352 uint16_t k23a ; /*!< Two-dimensional Y/C separation filter select coefficient, K23A */ 00353 uint16_t k24 ; /*!< Two-dimensional Y/C separation filter select coefficient, K24 */ 00354 vdec_lstg_bpfsel_t hbpf_narrow ; /*!< Latter-stage horizontal BPF select */ 00355 vdec_lstg_bpfsel_t hvbpf_narrow ; /*!< Latter-stage horizontal/vertical BPF select */ 00356 vdec_fstg_bpfsel_t hbpf1_9tap_on ; /*!< Former-stage horizontal BPF select */ 00357 vdec_fstg_bpfsel_t hvbpf1_9tap_on ; /*!< Former-stage horizontal/vertical BPF select */ 00358 vdec_hfil_tap_sel_t hfil_tap_sel; /*!< Horizontal filter and horizontal/vertical filter 00359 bandwidth switch signal */ 00360 vdec_onoff_t det2_on ; /*!< Two-dimensional filter mixing select */ 00361 vdec_filmix_ratio_t hsel_mix_y; /*!< Mixing ratio of signal after passing horizontal filter 00362 to signal after passing former-stage horizontal filter */ 00363 vdec_filmix_ratio_t vsel_mix_y; /*!< Mixing ratio of signal after passing vertical filter 00364 to signal after passing former-stage horizontal/vertical filter */ 00365 vdec_filmix_ratio_t hvsel_mix_y; /*!< Mixing ratio of signal after passing horizontal/vertical filter 00366 to signal after passing former-stage horizontal/vertical filter */ 00367 uint16_t v_y_level ; /*!< Vertical luminance detection level for correlation detection filter */ 00368 vdec_filmix_ratio_t det2_mix_c; /*!< Mixing ratio of C signal after passing horizontal/vertical adaptive filter 00369 to signal after passing correlation detection filter */ 00370 vdec_filmix_ratio_t det2_mix_y; /*!< Mixing ratio of C signal for Y generation after passing 00371 horizontal/vertical adaptive filter to signal 00372 after passing correlation detection filter */ 00373 vdec_2dfil_mdsel_t fil2_mode_2d ; /*!< Two-dimensional cascade/TAKE-OFF filter mode select */ 00374 vdec_2d_fil_sel_t fil2_narrow_2d ; /*!< Two-dimensional cascade filter select */ 00375 } vdec_yc_sep_ctrl_t ; 00376 /*! Chroma filter TAP coefficient for Y/C separation */ 00377 typedef struct { 00378 uint16_t fil2_2d_f[VDEC_CHRFIL_TAPCOEF_NUM]; /*!< Chroma filter TAP coefficient 0 to 8 */ 00379 } vdec_chrfil_tap_t ; 00380 /*! Y/C separation parameter */ 00381 typedef struct { 00382 vdec_over_range_t * over_range ; /*!< Over-range control parameter */ 00383 vdec_yc_sep_ctrl_t * yc_sep_ctrl ; /*!< Y/C separation control parameter */ 00384 const vdec_chrfil_tap_t * fil2_2d_wa; /*!< Two-dimensional cascade broadband (3.58/4.43/SECAM-DR)/TAKE-OFF 00385 filter TAP coefficient */ 00386 const vdec_chrfil_tap_t * fil2_2d_wb; /*!< Two-dimensional cascade broadband (SECAM-DB) filter 00387 TAP coefficient */ 00388 const vdec_chrfil_tap_t * fil2_2d_na; /*!< Two-dimensional cascade narrowband (3.58/4.43/SECAM-DR) filter 00389 TAP coefficient */ 00390 const vdec_chrfil_tap_t * fil2_2d_nb; /*!< Two-dimensional cascade narrowband (SECAMDB) filter 00391 TAP coefficient */ 00392 } vdec_yc_separation_t ; 00393 00394 /*********************** For R_VDEC_ChromaDecoding ***********************/ 00395 /*! Color system */ 00396 typedef enum { 00397 VDEC_COL_SYS_NTSC = 0, /*!< NTSC */ 00398 VDEC_COL_SYS_PAL , /*!< PAL */ 00399 VDEC_COL_SYS_SECAM , /*!< SECAM */ 00400 VDEC_COL_SYS_NON , /*!< Not specified/undetectable */ 00401 VDEC_COL_SYS_NUM /*!< The number of color system settings */ 00402 } vdec_color_sys_t ; 00403 /*! Averaging processing for pre-demodulated line */ 00404 typedef enum { 00405 VDEC_DEMOD_MD_NO = 0, /*!< No processing */ 00406 VDEC_DEMOD_MD_PAL = 2 /*!< For PAL */ 00407 } vdec_demodmode_t ; 00408 /*! Color system detection parameter */ 00409 typedef struct { 00410 vdec_color_sys_t defaultsys ; /*!< Default color system */ 00411 vdec_onoff_t nontsc358_ ; /*!< NTSC-M detection control */ 00412 vdec_onoff_t nontsc443_ ; /*!< NTSC-4.43 detection control */ 00413 vdec_onoff_t nopalm_ ; /*!< PAL-M detection control */ 00414 vdec_onoff_t nopaln_ ; /*!< PAL-N detection control */ 00415 vdec_onoff_t nopal443_ ; /*!< PAL-B, G, H, I, D detection control */ 00416 vdec_onoff_t nosecam_ ; /*!< SECAM detection control */ 00417 uint16_t lumadelay ; /*!< Luminance signal delay adjustment */ 00418 vdec_onoff_t chromalpf ; /*!< LPF for demodulated chroma */ 00419 vdec_demodmode_t demodmode ; /*!< Averaging processing for pre-demodulated line */ 00420 } vdec_chrmdec_ctrl_t ; 00421 /*! Burst lock PLL lock range */ 00422 typedef enum { 00423 VDEC_LOCK_RANGE_400HZ = 0, /*!< +-400 Hz */ 00424 VDEC_LOCK_RANGE_800HZ , /*!< +-800 Hz */ 00425 VDEC_LOCK_RANGE_1200HZ , /*!< +-1200 Hz */ 00426 VDEC_LOCK_RANGE_1600HZ , /*!< +-1600 Hz */ 00427 VDEC_LOCK_RANGE_NUM 00428 } vdec_lockrange_t ; 00429 /*! BCO parameter */ 00430 typedef struct { 00431 vdec_lockrange_t lockrange ; /*!< Burst lock PLL lock range */ 00432 uint16_t loopgain ; /*!< Burst lock PLL loop gain */ 00433 uint16_t locklimit ; /*!< Level for burst lock PLL to re-search free-run frequency */ 00434 uint16_t bgpcheck ; /*!< burst gate pulse position check */ 00435 uint16_t bgpwidth ; /*!< burst gate pulse width */ 00436 uint16_t bgpstart ; /*!< burst gate pulse start position */ 00437 } vdec_burst_lock_t ; 00438 /*! ACC operating mode */ 00439 typedef enum { 00440 VDEC_ACC_MD_AUTO = 0, /*!< Auto gain */ 00441 VDEC_ACC_MD_MANUAL = 1 /*!< Manual gain */ 00442 } vdec_accmode_t ; 00443 /*! Maximum ACC gain */ 00444 typedef enum { 00445 VDEC_ACC_MAX_GAIN_6 = 0, /*!< 6 times */ 00446 VDEC_ACC_MAX_GAIN_8 , /*!< 8 times */ 00447 VDEC_ACC_MAX_GAIN_12 , /*!< 12 times */ 00448 VDEC_ACC_MAX_GAIN_16 , /*!< 16 times */ 00449 VDEC_ACC_MAX_GAIN_NUM 00450 } vdec_accmaxgain_t ; 00451 /*! Chroma manual gain (sub) */ 00452 typedef enum { 00453 VDEC_CHRM_SB_GAIN_1 = 0, /*!< 1 time */ 00454 VDEC_CHRM_SB_GAIN_2 , /*!< 2 times */ 00455 VDEC_CHRM_SB_GAIN_4 , /*!< 4 times */ 00456 VDEC_CHRM_SB_GAIN_8 , /*!< 8 times */ 00457 VDEC_CHRM_SB_GAIN_NUM 00458 } vdec_chrm_subgain_t ; 00459 /*! ACC and color killer parameter */ 00460 typedef struct { 00461 vdec_accmode_t accmode ; /*!< ACC operating mode */ 00462 vdec_accmaxgain_t accmaxgain ; /*!< Maximum ACC Gain */ 00463 uint16_t acclevel ; /*!< ACC reference color burst amplitude */ 00464 vdec_chrm_subgain_t chromasubgain ; /*!< Chroma manual gain (sub) */ 00465 uint16_t chromamaingain ; /*!< Chroma manual gain (main) */ 00466 uint16_t accresponse ; /*!< ACC response speed */ 00467 uint16_t accprecis ; /*!< ACC gain adjustment accuracy */ 00468 vdec_onoff_t killermode ; /*!< Forced color killer mode ON/OFF */ 00469 uint16_t killerlevel ; /*!< Color killer operation start point */ 00470 uint16_t killeroffset ; /*!< Color killer offset */ 00471 } vdec_acc_t ; 00472 /*! TINT correction/R-Y axis correction parameter (only valid for NTSC/PAL) */ 00473 typedef struct { 00474 uint16_t tintsub ; /*!< Fine adjustment of R-Y demodulation axis */ 00475 uint16_t tintmain ; /*!< Hue adjustment level */ 00476 } vdec_tint_ry_t ; 00477 /*! Chroma decoding parameter */ 00478 typedef struct { 00479 vdec_chrmdec_ctrl_t * chrmdec_ctrl ; /*!< Color system detection parameter */ 00480 vdec_burst_lock_t * burst_lock ; /*!< BCO parameter */ 00481 vdec_acc_t * acc ; /*!< ACC and color killer parameter */ 00482 vdec_tint_ry_t * tint_ry ; /*!< TINT correction/R-Y axis correction parameter */ 00483 } vdec_chroma_decoding_t ; 00484 00485 /*********************** For R_VDEC_DigitalClamp ***********************/ 00486 /*! Clamp level setting mode */ 00487 typedef enum { 00488 VDEC_DCPMODE_MANUAL = 0, /*!< Manual clamp level setting */ 00489 VDEC_DCPMODE_AUTO = 1 /*!< Auto clamp level setting */ 00490 } vdec_dcpmode_t ; 00491 /*! Pedestal clamp parameter */ 00492 typedef struct { 00493 vdec_dcpmode_t dcpmode_y ; /*!< Clamp level setting mode (Y signal) */ 00494 uint16_t blanklevel_y ; /*!< Clamp offset level (Y signal) */ 00495 uint16_t dcppos_y ; /*!< Digital clamp pulse horizontal start position (Y signal) */ 00496 } vdec_pedestal_clamp_t ; 00497 /*! Center clamp parameter */ 00498 typedef struct { 00499 vdec_dcpmode_t dcpmode_c ; /*!< Clamp level setting mode (Cb/Cr signal) */ 00500 uint16_t blanklevel_cb ; /*!< Clamp offset level (Cb signal) */ 00501 uint16_t blanklevel_cr ; /*!< Clamp offset level (Cr signal) */ 00502 uint16_t dcppos_c ; /*!< Digital clamp pulse horizontal start position (Cb/Cr signal) */ 00503 } vdec_center_clamp_t ; 00504 /*! Video signal for autocorrelation function */ 00505 typedef enum { 00506 VDEC_ACFINPUT_Y = 0, /*!< Y signal */ 00507 VDEC_ACFINPUT_CB , /*!< Cb signal */ 00508 VDEC_ACFINPUT_CR , /*!< Cr signal */ 00509 VDEC_ACFINPUT_NUM 00510 } vdec_acfinput_t ; 00511 /*! Noise detection parameter */ 00512 typedef struct { 00513 vdec_acfinput_t acfinput ; /*!< Video signal for autocorrelation function */ 00514 uint16_t acflagtime ; /*!< Delay time for autocorrelation function calculation */ 00515 uint16_t acffilter ; /*!< Smoothing parameter of autocorrelation function data */ 00516 } vdec_noise_det_t ; 00517 /*! Digital clamp parameter */ 00518 typedef struct { 00519 vdec_onoff_t dcpcheck ; /*!< Digital clamp pulse position check */ 00520 uint16_t dcpresponse ; /*!< Digital clamp response speed */ 00521 uint16_t dcpstart ; /*!< Digital clamp start line */ 00522 uint16_t dcpend ; /*!< Digital clamp end line */ 00523 uint16_t dcpwidth ; /*!< Digital clamp pulse width */ 00524 vdec_pedestal_clamp_t * pedestal_clamp ; /*!< Pedestal clamp parameter */ 00525 vdec_center_clamp_t * center_clamp ; /*!< Center clamp parameter */ 00526 vdec_noise_det_t * noise_det ; /*!< Noise detection parameter */ 00527 } vdec_degital_clamp_t ; 00528 00529 /*********************** For R_VDEC_Output ***********************/ 00530 /*! Output adjustment parameter */ 00531 typedef struct { 00532 uint16_t y_gain2 ; /*!< Y signal gain coefficient */ 00533 uint16_t cb_gain2 ; /*!< Cb signal gain coefficient */ 00534 uint16_t cr_gain2 ; /*!< Cr signal gain coefficient */ 00535 } vdec_output_t ; 00536 00537 /*********************** For R_VDEC_Query ***********************/ 00538 /*! Answer */ 00539 typedef enum { 00540 VDEC_NO = 0, /*!< No */ 00541 VDEC_YES = 1 /*!< Yes */ 00542 } vdec_answer_t ; 00543 /*! Lock state */ 00544 typedef enum { 00545 VDEC_UNLOCK = 0, /*!< Unlocked */ 00546 VDEC_LOCK = 1 /*!< Locked */ 00547 } vdec_lock_t ; 00548 /*! Speed detection result */ 00549 typedef enum { 00550 VDEC_FHMD_NORMAL = 0, /*!< Normal speed */ 00551 VDEC_FHMD_MULTIPLIED = 1 /*!< Multiplied speed */ 00552 } vdec_fhmode_t ; 00553 /*! Vertical countdown oscillation mode */ 00554 typedef enum { 00555 VDEC_FVMD_50HZ = 0, /*!< 50 Hz */ 00556 VDEC_FVMD_60HZ = 1 /*!< 60 Hz */ 00557 } vdec_fvmode_t ; 00558 /*! Color sub-carrier frequency detection result */ 00559 typedef enum { 00560 VDEC_FSCMD_3_58 = 0, /*!< 3.58 MHz */ 00561 VDEC_FSCMD_4_43 = 1 /*!< 4.43 MHz */ 00562 } vdec_fscmode_t ; 00563 /*! Sync separation parameters (for query) */ 00564 typedef struct { 00565 vdec_lock_t fhlock ; /*!< Horizontal AFC lock detection result */ 00566 vdec_answer_t isnoisy ; /*!< Detection result of low S/N signal by sync separation */ 00567 vdec_fhmode_t fhmode ; /*!< Speed detection result */ 00568 vdec_answer_t nosignal_ ; /*!< No-signal detection result */ 00569 vdec_lock_t fvlock ; /*!< Vertical countdown lock detection result */ 00570 vdec_fvmode_t fvmode ; /*!< Vertical countdown oscillation mode */ 00571 vdec_answer_t interlaced ; /*!< Interlace detection result */ 00572 uint16_t fvcount ; /*!< Vertical cycle measurement result */ 00573 uint32_t fhcount ; /*!< Horizontal AFC oscillation cycle */ 00574 vdec_answer_t isreduced ; /*!< Sync amplitude detection result during VBI period */ 00575 uint16_t syncdepth ; /*!< Sync pulse amplitude detection result */ 00576 } vdec_q_sync_sep_t ; 00577 /*! AGC parameters (for query) */ 00578 typedef struct { 00579 uint16_t highsamples; /*!< Number of pixels which have larger luminance value than peak luminance 00580 limited by peak limiter */ 00581 uint16_t peaksamples ; /*!< Number of overflowing pixels */ 00582 uint16_t agcconverge ; /*!< AGC convergence detection result */ 00583 uint16_t agcgain ; /*!< Current AGC gain value */ 00584 } vdec_q_agc_t ; 00585 /*! Chroma decoding parameters (for query) */ 00586 typedef struct { 00587 vdec_color_sys_t colorsys ; /*!< Color system detection result */ 00588 vdec_fscmode_t fscmode ; /*!< Color sub-carrier frequency detection result */ 00589 vdec_lock_t fsclock ; /*!< Burst lock PLL lock state detection result */ 00590 vdec_answer_t noburst_ ; /*!< Color burst detection result */ 00591 vdec_chrm_subgain_t accsubgain ; /*!< Current ACC gain value (sub) */ 00592 uint16_t accmaingain ;/*!< Current ACC gain value (main) */ 00593 vdec_answer_t issecam ; /*!< SECAM detection result */ 00594 vdec_answer_t ispal ; /*!< PAL detection result */ 00595 vdec_answer_t isntsc ; /*!< NTSC detection result */ 00596 uint16_t locklevel ; /*!< Low S/N signal detection result by burst lock PLL */ 00597 } vdec_q_chroma_dec_t ; 00598 /*! Digital clamp parameters (for query) */ 00599 typedef struct { 00600 uint16_t clamplevel_cb ; /*!< Digital clamp subtraction value (Cb signal) */ 00601 uint16_t clamplevel_y ; /*!< Digital clamp subtraction value (Y signal) */ 00602 uint16_t clamplevel_cr ; /*!< Digital clamp subtraction value (Cr signal) */ 00603 uint16_t acfstrength ; /*!< Noise autocorrelation strength at digital clamp pulse position */ 00604 } vdec_q_digital_clamp_t ; 00605 00606 00607 /****************************************************************************** 00608 Functions Prototypes 00609 ******************************************************************************/ 00610 vdec_error_t R_VDEC_Initialize( 00611 const vdec_channel_t ch, 00612 const vdec_adc_vinsel_t vinsel, 00613 void (* const init_func)(uint32_t), 00614 const uint32_t user_num); 00615 vdec_error_t R_VDEC_Terminate(const vdec_channel_t ch, void (* const quit_func)(uint32_t), const uint32_t user_num); 00616 vdec_error_t R_VDEC_ActivePeriod(const vdec_channel_t ch, const vdec_active_period_t * const param); 00617 vdec_error_t R_VDEC_SyncSeparation(const vdec_channel_t ch, const vdec_sync_separation_t * const param); 00618 vdec_error_t R_VDEC_YcSeparation(const vdec_channel_t ch, const vdec_yc_separation_t * const param); 00619 vdec_error_t R_VDEC_ChromaDecoding(const vdec_channel_t ch, const vdec_chroma_decoding_t * const param); 00620 vdec_error_t R_VDEC_DigitalClamp(const vdec_channel_t ch, const vdec_degital_clamp_t * const param); 00621 vdec_error_t R_VDEC_Output(const vdec_channel_t ch, const vdec_output_t * const param); 00622 vdec_error_t R_VDEC_Query( 00623 const vdec_channel_t ch, 00624 vdec_q_sync_sep_t * const q_sync_sep, 00625 vdec_q_agc_t * const q_agc, 00626 vdec_q_chroma_dec_t * const q_chroma_dec, 00627 vdec_q_digital_clamp_t * const q_digital_clamp); 00628 00629 00630 #ifdef __cplusplus 00631 } 00632 #endif /* __cplusplus */ 00633 00634 00635 #endif /* R_VDEC_H */ 00636
Generated on Tue Jul 12 2022 15:08:46 by
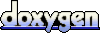