
Sample program for communicating with Fujitsuu IoT Platform using HTTP
Dependencies: AsciiFont GR-PEACH_video GraphicsFramework LCD_shield_config R_BSP USBHost_custom easy-connect-gr-peach mbed-http picojson BM1383GLV KX022 rohm-sensor-hal rohm-bh1745
BM1422AGMV.cpp
00001 /* Copyright (c) 2015 ARM Ltd., MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 */ 00019 00020 #include "mbed.h" 00021 #include "BM1422AGMV.h" 00022 00023 BM1422AGMV::BM1422AGMV(PinName sda, PinName scl, int addr) : m_i2c(sda, scl), m_addr(addr) 00024 { 00025 initialize(); 00026 } 00027 00028 BM1422AGMV::BM1422AGMV(I2C &i2c_obj, int addr) : m_i2c(i2c_obj), m_addr(addr) 00029 { 00030 initialize(); 00031 } 00032 00033 BM1422AGMV::~BM1422AGMV() 00034 { 00035 /* do nothing */ 00036 } 00037 00038 void BM1422AGMV::initialize(void) 00039 { 00040 uint8_t reg; 00041 uint8_t buf[3]; 00042 00043 _drdy_flg = 0; 00044 00045 DEBUG_PRINT("BM1422AGMV init started\n\r"); 00046 00047 readRegs(BM1422AGMV_WIA, ®, 1); 00048 00049 if ( reg != BM1422AGMV_WIA_VAL) { 00050 DEBUG_PRINT("BM1422AGMV initialization error. (WAI %d, not %d)\n\r", buf, BM1422AGMV_WIA); 00051 DEBUG_PRINT("Trying to config anyway, in case there is some compatible sensor connected.\n\r"); 00052 } 00053 00054 // Step1 00055 buf[0] = BM1422AGMV_CNTL1; 00056 buf[1] = BM1422AGMV_CNTL1_VAL; 00057 writeRegs(buf, 2); 00058 00059 // Check 12bit or 14bit 00060 reg = (BM1422AGMV_CNTL1_VAL & BM1422AGMV_CNTL1_OUT_BIT); 00061 if (reg == BM1422AGMV_CNTL1_OUT_BIT) { 00062 _sens = BM1422AGMV_14BIT_SENS; 00063 } else { 00064 _sens = BM1422AGMV_12BIT_SENS; 00065 } 00066 00067 wait(1); 00068 00069 buf[0] = BM1422AGMV_CNTL4; 00070 buf[1] = (BM1422AGMV_CNTL4_VAL >> 8) & 0xFF; 00071 buf[2] = (BM1422AGMV_CNTL4_VAL & 0xFF); 00072 writeRegs(buf, 3); 00073 00074 // Step2 00075 buf[0] = BM1422AGMV_CNTL2; 00076 buf[1] = BM1422AGMV_CNTL2_VAL; 00077 writeRegs(buf, 2); 00078 00079 // Step3 00080 00081 // Option 00082 buf[0] = BM1422AGMV_AVE_A; 00083 buf[1] = BM1422AGMV_AVE_A_VAL; 00084 writeRegs(buf, 2); 00085 } 00086 00087 void BM1422AGMV::get_rawval(unsigned char *data) 00088 { 00089 uint8_t reg; 00090 uint8_t buf[2]; 00091 00092 // Step 4 00093 buf[0] = BM1422AGMV_CNTL3; 00094 buf[1] = BM1422AGMV_CNTL3_VAL; 00095 writeRegs(buf, 2); 00096 00097 while(1) { 00098 readRegs(BM1422AGMV_STA1, ®, 1); 00099 if ( (reg>>6) == 1) { 00100 break; 00101 } 00102 wait_ms(100); 00103 } 00104 00105 readRegs(BM1422AGMV_DATAX, data, 6); 00106 } 00107 00108 void BM1422AGMV::get_val(float *data) 00109 { 00110 uint8_t val[6]; 00111 int16_t mag[3]; 00112 00113 get_rawval(val); 00114 00115 mag[0] = ((int16_t)val[1] << 8) | (int16_t)(val[0]); 00116 mag[1] = ((int16_t)val[3] << 8) | (int16_t)(val[2]); 00117 mag[2] = ((int16_t)val[5] << 8) | (int16_t)(val[4]); 00118 00119 convert_uT(mag, data); 00120 } 00121 00122 void BM1422AGMV::convert_uT(int16_t *rawdata, float *data) 00123 { 00124 // LSB to uT 00125 data[0] = (float)rawdata[0] / _sens; 00126 data[1] = (float)rawdata[1] / _sens; 00127 data[2] = (float)rawdata[2] / _sens; 00128 } 00129 00130 void BM1422AGMV::set_drdy_flg(void) 00131 { 00132 _drdy_flg = 1; 00133 } 00134 00135 void BM1422AGMV::readRegs(int addr, uint8_t * data, int len) 00136 { 00137 int read_nok; 00138 char t[1] = {addr}; 00139 00140 m_i2c.write(m_addr, t, 1, true); 00141 read_nok = m_i2c.read(m_addr, (char *)data, len); 00142 if (read_nok){ 00143 DEBUG_PRINT("Read fail\n\r"); 00144 } 00145 } 00146 00147 void BM1422AGMV::writeRegs(uint8_t * data, int len) 00148 { 00149 m_i2c.write(m_addr, (char *)data, len); 00150 }
Generated on Wed Jul 13 2022 06:45:29 by
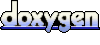