
RealtimeCompLab2
Dependencies: mbed
Fork of PPP-Blinky by
sha1.h
00001 #ifndef SHA1_H 00002 #define SHA1_H 00003 00004 /* 00005 SHA-1 in C 00006 By Steve Reid <steve@edmweb.com> 00007 100% Public Domain 00008 */ 00009 00010 #include "stdint.h" 00011 00012 typedef struct 00013 { 00014 uint32_t state[5]; 00015 uint32_t count[2]; 00016 unsigned char buffer[64]; 00017 } SHA1_CTX; 00018 00019 void SHA1Transform( 00020 uint32_t state[5], 00021 const unsigned char buffer[64] 00022 ); 00023 00024 void SHA1Init( 00025 SHA1_CTX * context 00026 ); 00027 00028 void SHA1Update( 00029 SHA1_CTX * context, 00030 const unsigned char *data, 00031 uint32_t len 00032 ); 00033 00034 void SHA1Final( 00035 unsigned char digest[20], 00036 SHA1_CTX * context 00037 ); 00038 00039 void sha1( 00040 char *hash_out, 00041 const char *str, 00042 int len); 00043 00044 #endif /* SHA1_H */
Generated on Wed Jul 13 2022 22:24:08 by
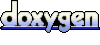