
I messed up the merge, so pushing it over to another repo so I don't lose it. Will tidy up and remove later
Dependencies: BufferedSerial FatFileSystemCpp mbed
VIPSSerialProtocol.h
00001 #ifndef __VIPSSERIALPROTOCOL_H__ 00002 #define __VIPSSERIALPROTOCOL_H__ 00003 00004 #include "mbed.h" 00005 #include "position.h" 00006 #include "BufferedSerial.h" 00007 #include "LTCApp.h" 00008 #include "LowPassFilter.h" 00009 00010 #define MaxBuffSize 272 //The Get Unit Info LPC command (G N) returns 266 bytes 00011 00012 00013 00014 extern const char* VIPSStatusMessages[]; 00015 struct UserSettings_s; 00016 00017 class VIPSSerial 00018 { 00019 00020 public: 00021 00022 VIPSSerial(const PinName Tx, const PinName Rx); 00023 void run(void); 00024 bool setFilters(struct UserSettings_s *settings); 00025 00026 // send all position outputs rather than just when requested. 00027 void sendAllUpdated(bool enable); 00028 00029 // send a position output for the requested time. Times are based on the global TimeSinceLastFrame timer. 00030 position* sendPositionForTime(uint32_t timeValue); 00031 position* getWaitingPostion() 00032 { 00033 position *ptr = outputPtr; 00034 outputPtr=NULL; 00035 return ptr; 00036 } 00037 00038 static void getCRC(void *data, int len, void *checksum); 00039 00040 // void setOutMask(uint32_t outputMask) {_outputMask = outputMask;}; 00041 00042 bool EnableSmoothing(bool enabled) 00043 { 00044 hyperSmoothEnabled = enabled; 00045 return hyperSmoothEnabled; 00046 }; 00047 bool SmoothingEnabled(void) 00048 { 00049 return hyperSmoothEnabled; 00050 }; 00051 bool ForceSmoothing(bool enabled) 00052 { 00053 forcedHyperSmooth = enabled; 00054 hyperSmoothEnabled = enabled; 00055 return hyperSmoothEnabled; 00056 }; 00057 bool ForceSmoothingEnabled(bool enabled) 00058 { 00059 return forcedHyperSmooth; 00060 }; 00061 int GetSmoothLevel(void) 00062 { 00063 return SmoothBy; 00064 }; 00065 bool SetSmoothLevel (const int newSmooth) 00066 { 00067 if (newSmooth == SmoothBy) return false; 00068 SmoothBy = newSmooth; 00069 SmoothRunning = false; 00070 return true; 00071 }; 00072 void EnableBypass(bool enable) 00073 { 00074 BypassMode = enable; 00075 }; 00076 void bypassTx(char byte); 00077 void sendQueued(void); 00078 bool EnableDirectTX(bool enabled) 00079 { 00080 directTx = enabled; 00081 return directTx; 00082 }; 00083 void sendDirectTX(unsigned char* data, int dataLen); 00084 int getWaitingBuffer(unsigned char **TXBuffer, int *bytesToSend); 00085 void sendQuiet(void); 00086 00087 private: 00088 00089 struct posAndTime_s { 00090 uint32_t time; 00091 position pos; 00092 }; 00093 00094 void smoothOutputPacket(position *posPtr); 00095 void onSerialRx(void); 00096 void processRxMessage(); 00097 bool checkCRC(unsigned char* data); 00098 void sendResponse(unsigned char function, unsigned char* data, int dataLen); 00099 void queueResponse(unsigned char function, unsigned char* data, int dataLen); 00100 void sendAck(unsigned char function); 00101 void sendNack(unsigned char function); 00102 void sendVBOXTime(); 00103 void parsePostionInput_legacy(); 00104 void parsePostionInput_mocap(); 00105 void onTxTimeout(); 00106 bool checkNewPacketRC(unsigned char* data); 00107 RawSerial _port; 00108 unsigned char messageInBuffer[128]; 00109 unsigned char messageOutBuffer[16]; 00110 unsigned char TXBuffer1[MaxBuffSize]; 00111 unsigned char TXBuffer2[MaxBuffSize]; 00112 unsigned char *txBuf; 00113 int waitingBytes; 00114 #define posHistoryLen 3 00115 struct posAndTime_s lastPositions[posHistoryLen]; 00116 int nextPosition; 00117 struct posAndTime_s lastPos; // the most recent position received 00118 struct posAndTime_s prevPos; // the most last but one position received 00119 00120 position outputPosition; 00121 position *outputPtr; 00122 00123 int messagePrt; 00124 int messageLength; 00125 int statusMessage; 00126 bool enableAllUpdates; 00127 bool newFormatMsg; 00128 bool hyperSmoothEnabled; 00129 bool forcedHyperSmooth; 00130 bool directTx; 00131 uint32_t pointCount; 00132 uint32_t _outputMask; 00133 00134 int queueLen; 00135 int SmoothBy; 00136 // total as a float we would start to see rounding errors at valuses of ~20m 00137 double XSmoothTotal; 00138 double YSmoothTotal; 00139 double ZSmoothTotal; 00140 bool SmoothRunning; 00141 bool BypassMode; 00142 LowPassFilter yFilter; 00143 LowPassFilter xFilter; 00144 LowPassFilter zFilter; 00145 LowPassFilter rollFilter; 00146 LowPassFilter pitchFilter; 00147 LowPassFilter yawFilter; 00148 00149 Timeout TxTimeout; 00150 bool TransmitFinished; 00151 //VIPSSerial* self; 00152 }; 00153 00154 #endif
Generated on Thu Dec 15 2022 06:07:05 by
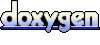